Using the Salesloft API to Create or Update Accounts (with Python examples)
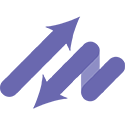
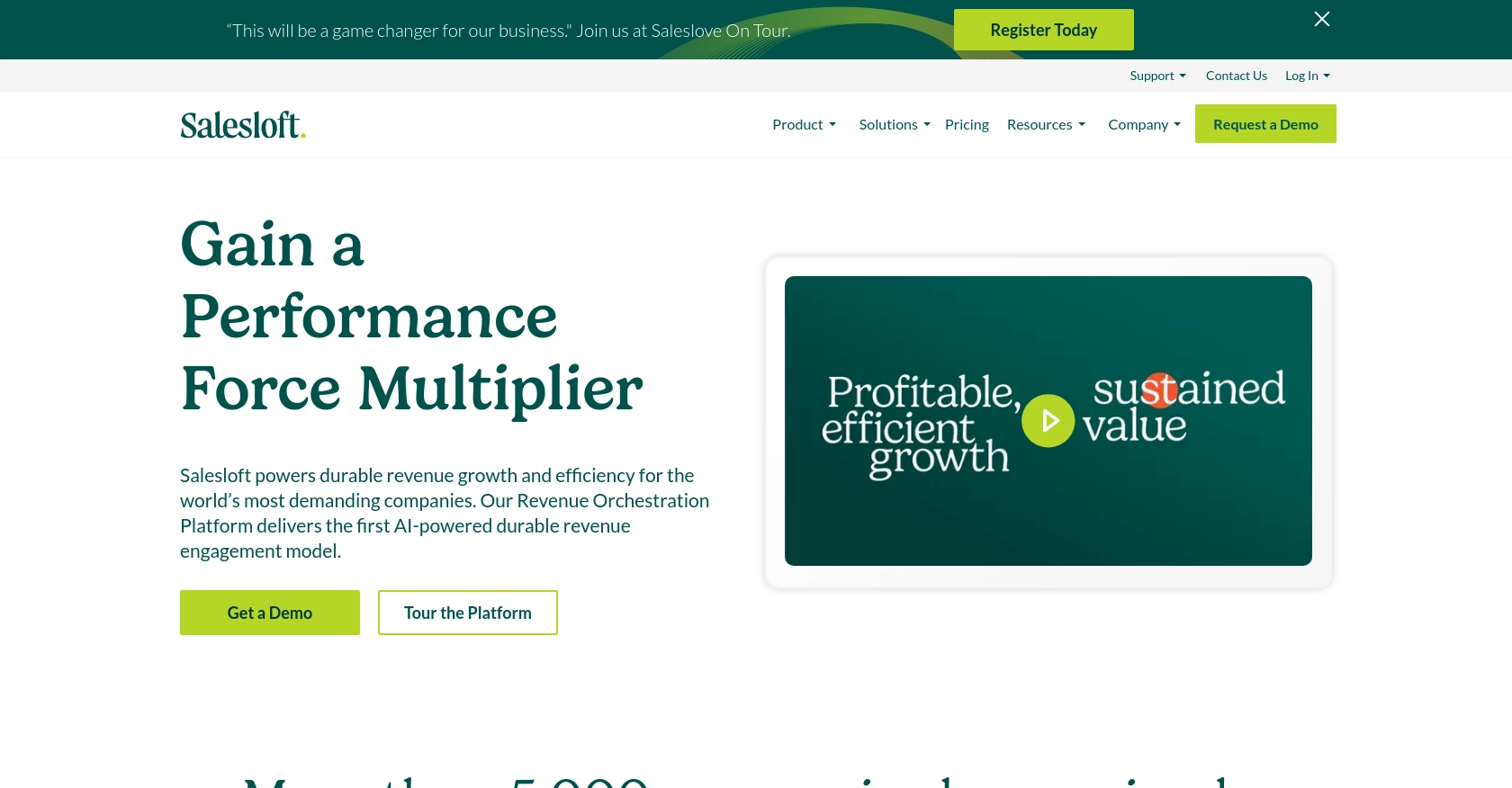
Introduction to Salesloft API Integration
Salesloft is a premier sales engagement platform that empowers sales teams to enhance their communication strategies and streamline their workflows. By providing a suite of tools for managing customer interactions, Salesloft helps businesses increase their sales efficiency and effectiveness.
Developers may want to integrate with the Salesloft API to automate and optimize account management processes. For example, using the Salesloft API, a developer can create or update account information directly from their application, ensuring that sales teams have access to the most current data without manual entry.
This article will guide you through using Python to interact with the Salesloft API, specifically focusing on creating or updating accounts. By following this tutorial, you will learn how to efficiently manage account data within the Salesloft platform using Python code examples.
Setting Up Your Salesloft Test/Sandbox Account
Before you can start integrating with the Salesloft API, you need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data.
Creating a Salesloft Account
If you don't already have a Salesloft account, you can sign up for a free trial or demo account on the Salesloft website. Follow the instructions to complete the registration process. Once your account is created, log in to access the Salesloft dashboard.
Generating OAuth Credentials for Salesloft API
Salesloft uses OAuth 2.0 for authentication, which requires you to create an OAuth app to obtain the necessary credentials.
- Navigate to your Salesloft account settings.
- Go to Your Applications and select OAuth Applications.
- Click on Create New to set up a new OAuth application.
- Fill in the required fields and save your application.
- After saving, you will receive your Client ID, Client Secret, and Redirect URI. Make sure to store these credentials securely, as they will be needed for API authentication.
Authorizing Your Application
To authorize your application and obtain an access token, follow these steps:
- Generate a request to the Salesloft authorization endpoint using your Client ID and Redirect URI:
- Authorize the application when prompted. Upon approval, you will receive an authorization code.
- Exchange the authorization code for an access token by making a POST request to the token endpoint:
- Store the received access token and refresh token securely for future API requests.
https://accounts.salesloft.com/oauth/authorize?client_id=YOUR_CLIENT_ID&redirect_uri=YOUR_REDIRECT_URI&response_type=code
POST https://accounts.salesloft.com/oauth/token
{
"client_id": "YOUR_CLIENT_ID",
"client_secret": "YOUR_CLIENT_SECRET",
"code": "AUTHORIZATION_CODE",
"grant_type": "authorization_code",
"redirect_uri": "YOUR_REDIRECT_URI"
}
With your Salesloft account and OAuth credentials set up, you are now ready to make API calls to create or update accounts using Python.
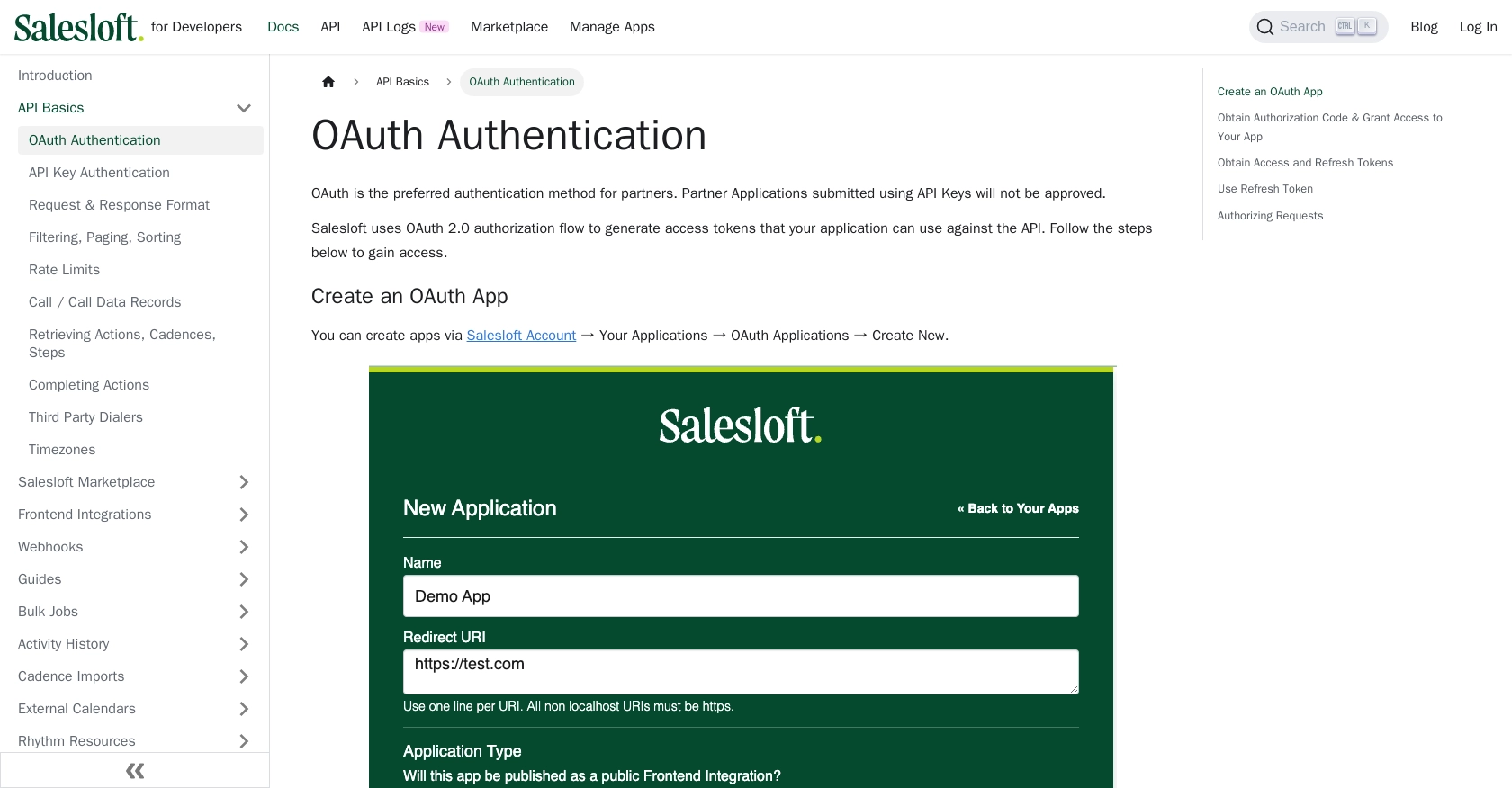
sbb-itb-96038d7
Making API Calls to Create or Update Accounts in Salesloft Using Python
With your Salesloft OAuth credentials ready, you can now proceed to make API calls to create or update accounts. This section will guide you through the necessary steps and provide Python code examples to interact with the Salesloft API effectively.
Setting Up Your Python Environment for Salesloft API Integration
Before making API calls, ensure you have Python 3.11.1 and the requests
library installed. You can install the requests
library using pip:
pip install requests
Creating a New Account in Salesloft
To create a new account in Salesloft, you need to make a POST request to the Salesloft API endpoint. Below is a Python example demonstrating how to create an account:
import requests
# Define the API endpoint and headers
url = "https://api.salesloft.com/v2/accounts"
headers = {
"Authorization": "Bearer YOUR_ACCESS_TOKEN",
"Content-Type": "application/json"
}
# Define the account data
account_data = {
"name": "Example Company",
"domain": "example.com",
"description": "A sample company for demonstration purposes",
"phone": "+1234567890",
"website": "https://example.com"
}
# Make the POST request to create the account
response = requests.post(url, json=account_data, headers=headers)
# Check if the request was successful
if response.status_code == 200:
print("Account created successfully:", response.json())
else:
print("Failed to create account:", response.status_code, response.json())
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth process. The code above sends a POST request to the Salesloft API to create a new account with the specified details. If successful, it prints the account information returned by the API.
Updating an Existing Account in Salesloft
To update an existing account, you need to make a PUT request to the Salesloft API. Here's how you can update an account using Python:
import requests
# Define the API endpoint and headers
account_id = "ACCOUNT_ID_TO_UPDATE"
url = f"https://api.salesloft.com/v2/accounts/{account_id}"
headers = {
"Authorization": "Bearer YOUR_ACCESS_TOKEN",
"Content-Type": "application/json"
}
# Define the updated account data
updated_data = {
"description": "Updated description for the account"
}
# Make the PUT request to update the account
response = requests.put(url, json=updated_data, headers=headers)
# Check if the request was successful
if response.status_code == 200:
print("Account updated successfully:", response.json())
else:
print("Failed to update account:", response.status_code, response.json())
Replace ACCOUNT_ID_TO_UPDATE
with the ID of the account you wish to update. The code sends a PUT request to update the account's description. If successful, it prints the updated account details.
Handling Errors and Verifying API Requests
When making API calls, it's crucial to handle potential errors. The Salesloft API may return various status codes indicating success or failure. For instance, a 200 status code indicates success, while a 422 status code may indicate validation errors. Always check the response status code and handle errors appropriately.
To verify that your API requests are successful, you can log in to your Salesloft sandbox account and check the accounts list to see if the new or updated account appears as expected.
For more detailed information on error codes and handling, refer to the Salesloft API documentation.
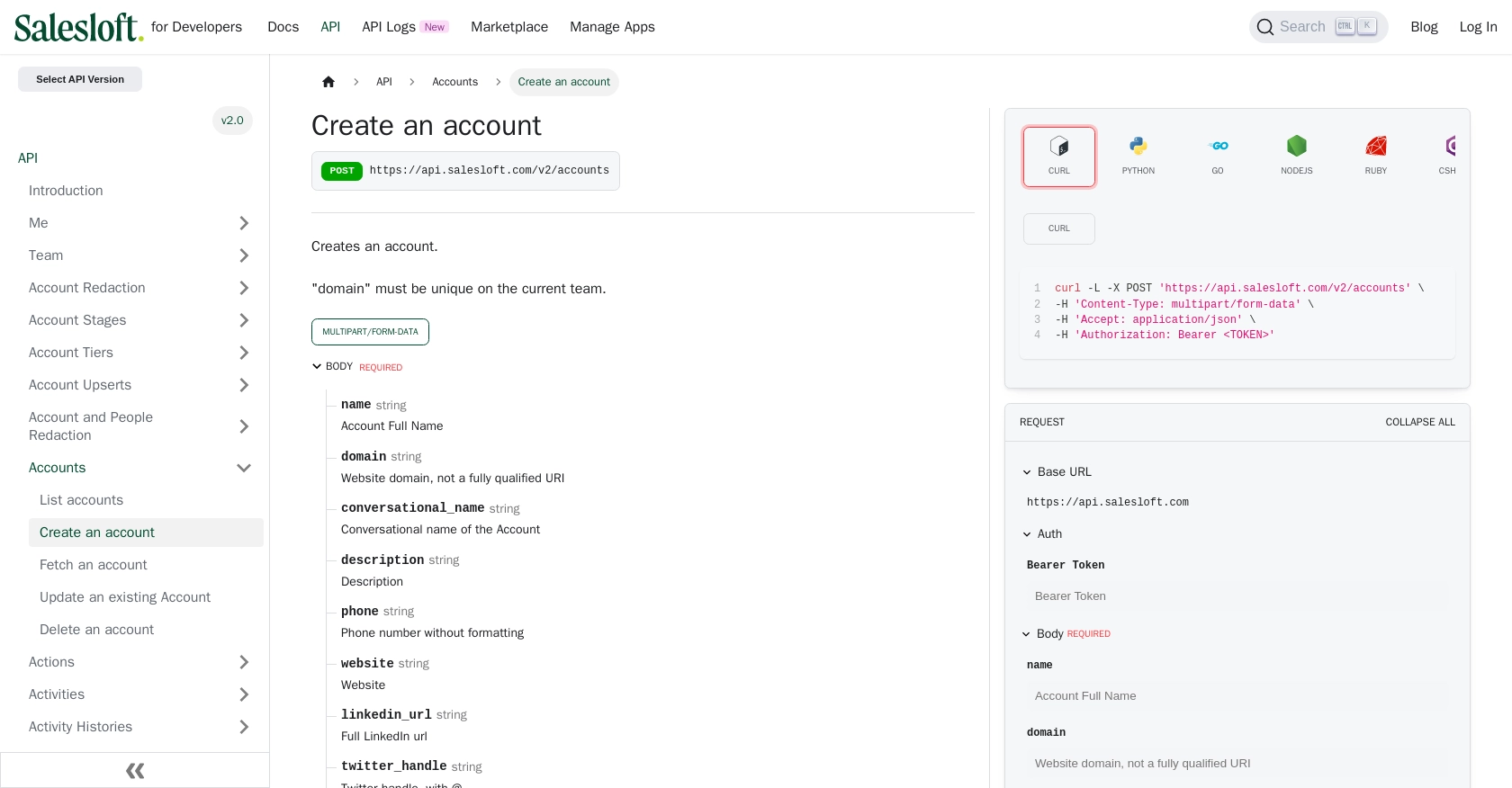
Conclusion and Best Practices for Salesloft API Integration
Integrating with the Salesloft API using Python provides a powerful way to automate account management and streamline sales processes. By following the steps outlined in this guide, developers can efficiently create and update account information, ensuring that sales teams have access to the most current data.
Best Practices for Secure and Efficient Salesloft API Usage
- Secure Storage of Credentials: Always store your OAuth credentials, including access and refresh tokens, securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handling Rate Limits: Be mindful of the Salesloft API rate limits, which are set at 600 cost per minute. Implement logic to handle rate limit responses and consider using exponential backoff strategies to retry requests when limits are reached. For more details, refer to the Salesloft API rate limits documentation.
- Error Handling: Implement robust error handling to manage various HTTP status codes returned by the API. This includes checking for validation errors (422) and unauthorized access (401).
- Data Standardization: Ensure that data fields are standardized and validated before sending them to the API to prevent errors and maintain data integrity.
Enhancing Integration Capabilities with Endgrate
While integrating with Salesloft directly can be effective, using a tool like Endgrate can further simplify the process. Endgrate offers a unified API endpoint that connects to multiple platforms, including Salesloft, allowing developers to manage integrations more efficiently. By leveraging Endgrate, you can save time and resources, focusing on your core product development while ensuring a seamless integration experience for your customers.
Explore how Endgrate can enhance your integration capabilities by visiting Endgrate and discover how it can help streamline your development workflow.
Read More
- https://endgrate.com/provider/salesloft
- https://developers.salesloft.com/docs/platform/api-basics/oauth-authentication/
- https://developers.salesloft.com/docs/platform/api-basics/request-response-format/
- https://developers.salesloft.com/docs/platform/api-basics/filtering-paging-sorting/
- https://developers.salesloft.com/docs/platform/api-basics/rate-limits/
- https://developers.salesloft.com/docs/api/accounts-create/
Ready to get started?