Using the Cloze API to Get Companies in Javascript
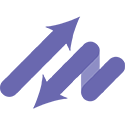
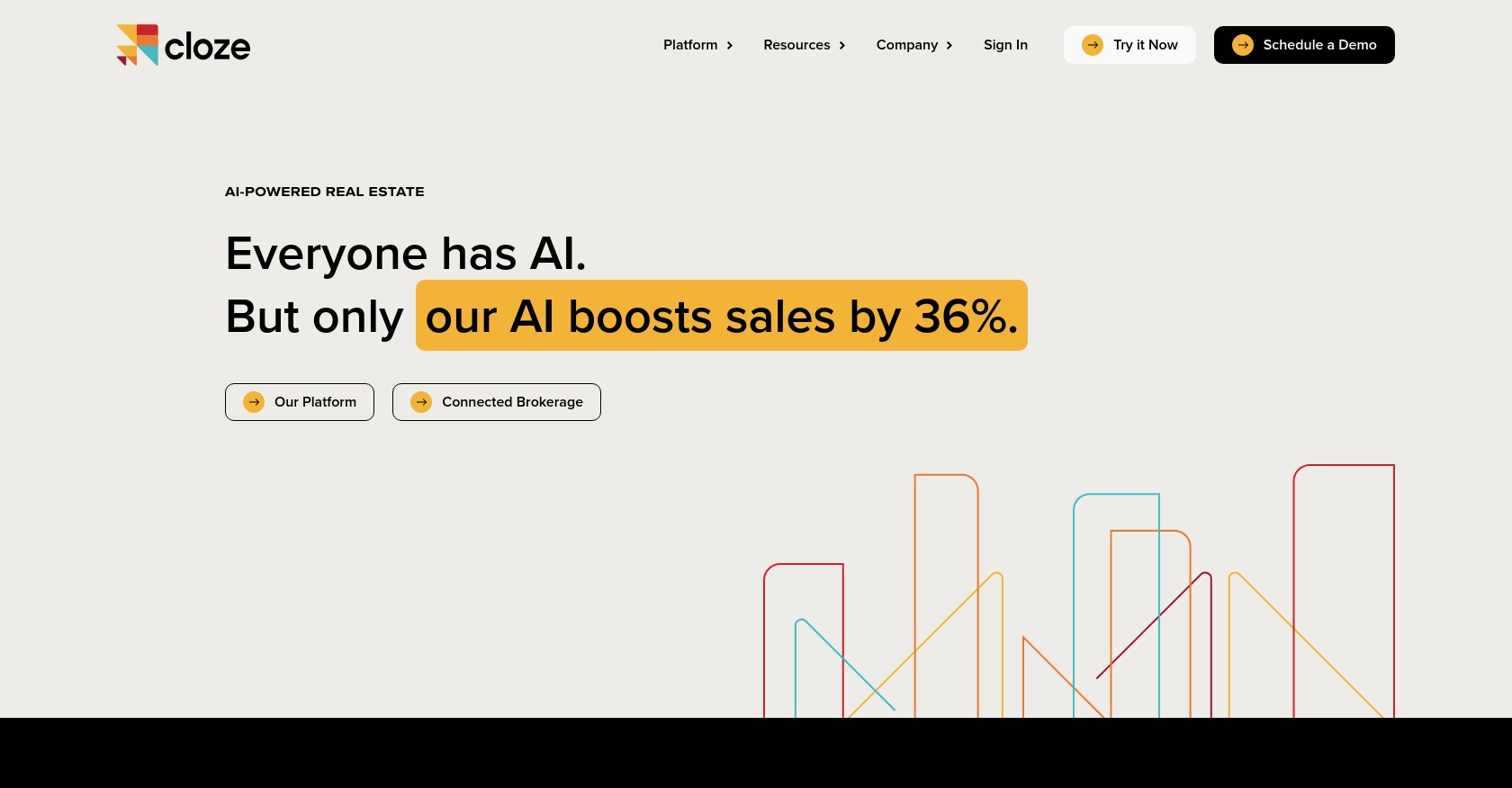
Introduction to Cloze API
Cloze is a powerful relationship management platform designed to help businesses manage their interactions with clients, partners, and prospects. With its comprehensive suite of tools, Cloze offers features for tracking communications, managing projects, and analyzing engagement, making it an essential tool for businesses aiming to optimize their relationship management strategies.
Integrating with the Cloze API allows developers to access and manage company data efficiently. For example, you might want to retrieve a list of companies from Cloze to analyze their engagement metrics or to synchronize data with another system. This integration can streamline workflows and enhance data-driven decision-making processes.
Setting Up Your Cloze API Test Account
Before you can start integrating with the Cloze API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data.
Creating a Cloze Account for API Testing
If you don't already have a Cloze account, you can sign up for a free trial on the Cloze website. This will provide you with access to the necessary tools and features to begin testing the API.
- Visit the Cloze website and click on the "Sign Up" button.
- Follow the on-screen instructions to create your account.
- Once your account is set up, log in to access the dashboard.
Generating an API Key for Cloze API Access
To authenticate your API requests, you'll need an API key. Follow these steps to generate one:
- Navigate to the "Settings" section in your Cloze dashboard.
- Under "Integrations," select "API Keys."
- Click on "Create API Key" and provide a name for your key.
- Copy the generated API key and store it securely, as you'll need it for API calls.
For more detailed instructions, refer to the Cloze API documentation.
Configuring OAuth for Public Integrations
If you're planning to build a public integration with Cloze, you'll need to use OAuth for authentication. Here's how to get started:
- Contact Cloze support at support@cloze.com to enable OAuth for your account.
- Once enabled, follow the instructions provided by Cloze support to configure your OAuth settings.
Using OAuth allows you to authenticate users securely and is recommended for integrations intended for external users.
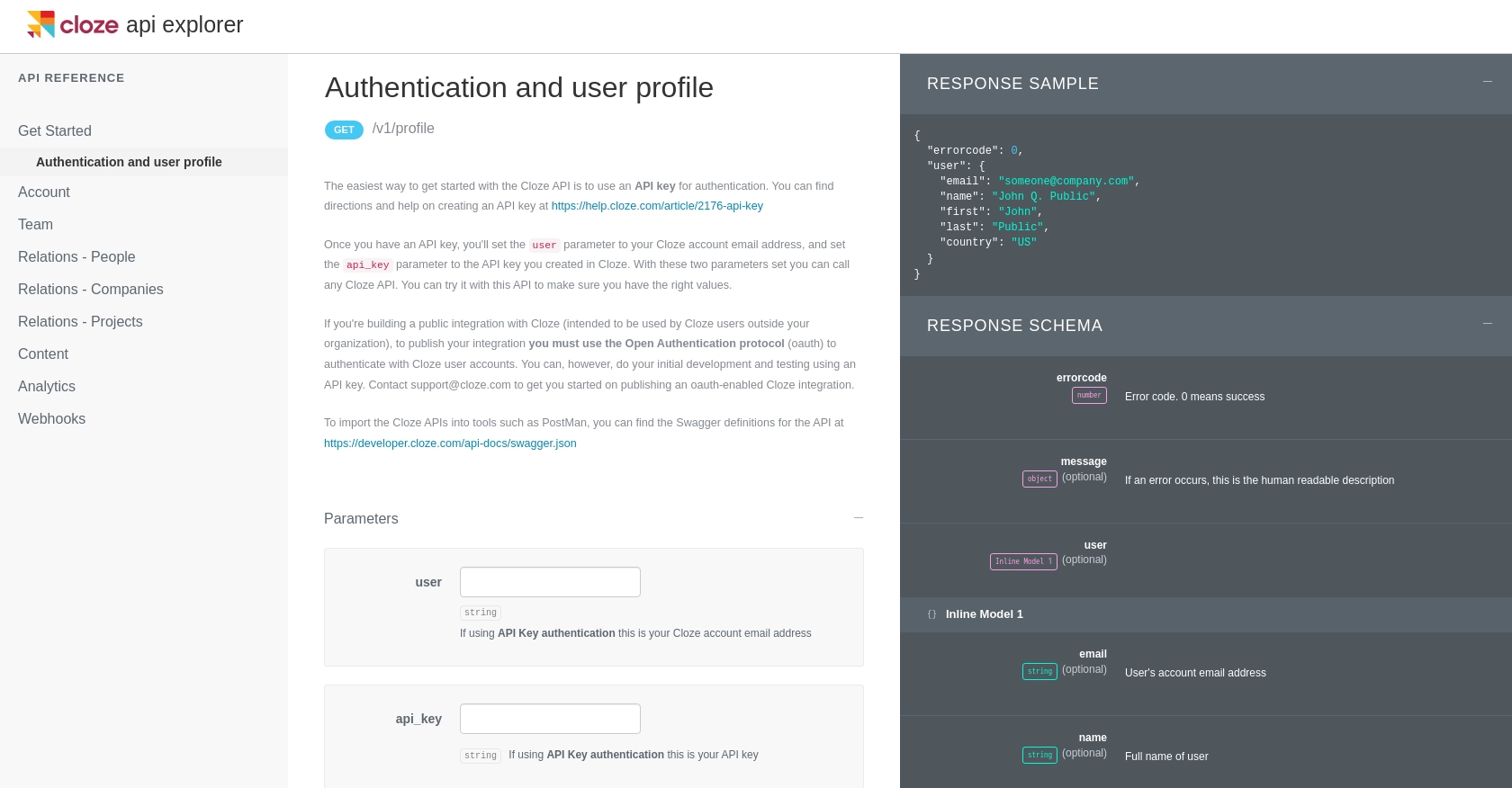
sbb-itb-96038d7
Making API Calls to Retrieve Companies Using Cloze API in JavaScript
To interact with the Cloze API and retrieve company data, you'll need to use JavaScript to make HTTP requests. This section will guide you through setting up your environment and executing the necessary API calls.
Setting Up Your JavaScript Environment for Cloze API Integration
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine. You can download it from the official Node.js website.
- A text editor or IDE such as Visual Studio Code.
Once Node.js is installed, you can use npm (Node Package Manager) to install the axios
library, which simplifies making HTTP requests:
npm install axios
Executing the API Call to Get Companies from Cloze
With your environment set up, you can now write a script to retrieve companies from the Cloze API. Create a new JavaScript file, for example, getCompanies.js
, and add the following code:
const axios = require('axios');
// Replace with your Cloze API key and email
const apiKey = 'YOUR_API_KEY';
const userEmail = 'YOUR_EMAIL';
const getCompanies = async () => {
try {
const response = await axios.get('https://api.cloze.com/v1/companies/find', {
params: {
user: userEmail,
api_key: apiKey,
pagesize: 10,
pagenumber: 1
}
});
if (response.data.errorcode === 0) {
console.log('Companies retrieved successfully:', response.data.companies);
} else {
console.error('Error retrieving companies:', response.data.message);
}
} catch (error) {
console.error('API call failed:', error);
}
};
getCompanies();
In this script, we use axios
to send a GET request to the Cloze API endpoint for retrieving companies. Ensure you replace YOUR_API_KEY
and YOUR_EMAIL
with your actual Cloze API key and email address.
Running and Verifying Your JavaScript Code
To execute the script, run the following command in your terminal:
node getCompanies.js
If successful, the console will display the list of companies retrieved from the Cloze API. Verify the output by checking the company data against your Cloze test account.
Handling Errors and Troubleshooting API Calls
When making API calls, it's essential to handle potential errors gracefully. The script above includes basic error handling, logging any issues encountered during the API request. For more detailed error information, refer to the Cloze API documentation.
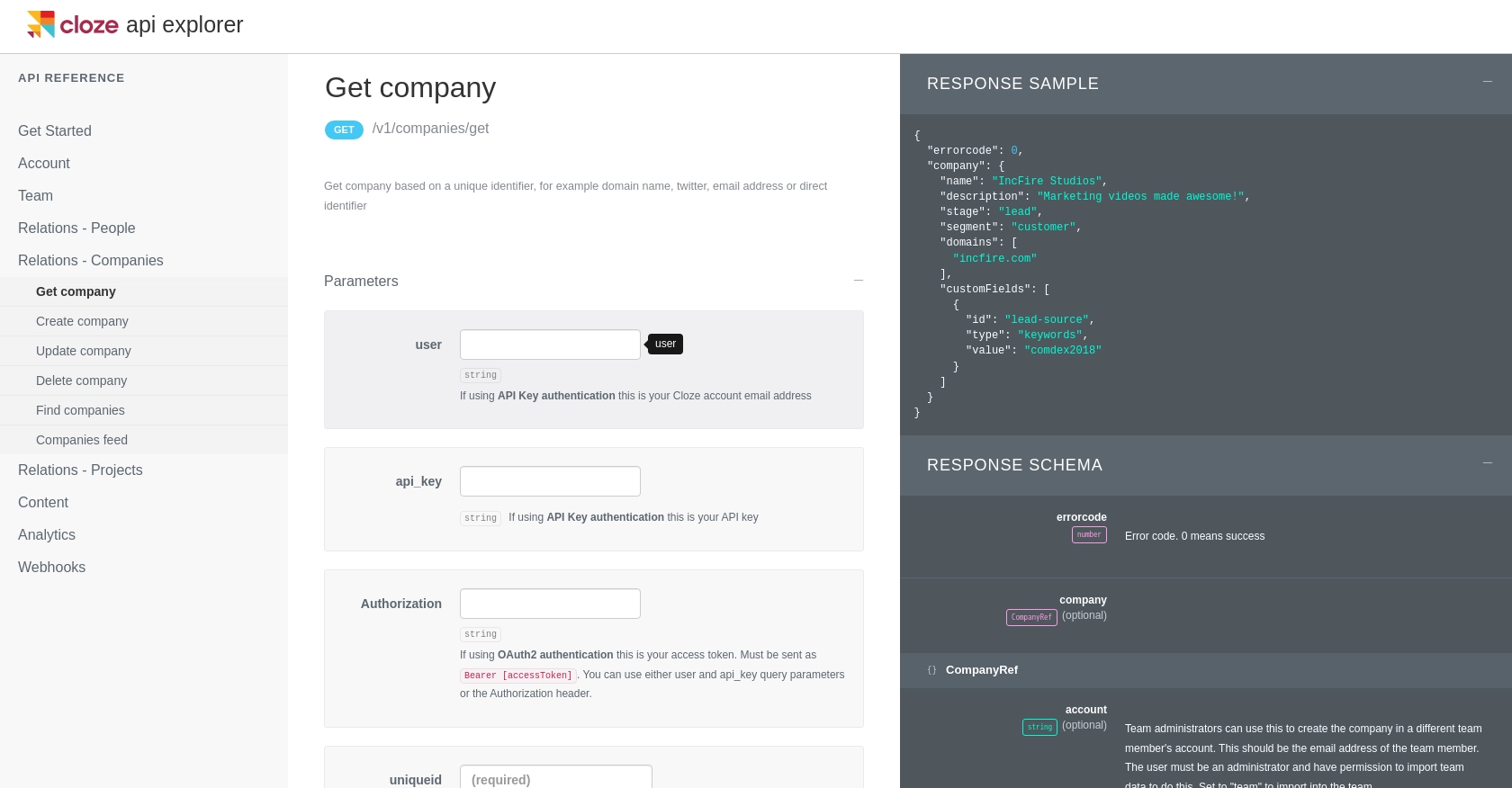
Conclusion and Best Practices for Using Cloze API in JavaScript
Integrating with the Cloze API using JavaScript can significantly enhance your ability to manage and analyze company data. By following the steps outlined in this guide, you can efficiently retrieve and utilize company information to drive data-driven decisions and streamline your workflows.
Best Practices for Secure and Efficient API Integration
- Secure API Key Storage: Always store your API keys securely. Avoid hardcoding them in your scripts. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of the API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that the data retrieved from Cloze is standardized and transformed as needed to fit your application’s requirements. This can help maintain data consistency across systems.
- Error Handling: Implement robust error handling to manage API call failures. Log errors for troubleshooting and provide user-friendly messages where applicable.
Enhancing Your Integration Experience with Endgrate
While integrating with the Cloze API can be straightforward, managing multiple integrations can become complex. Endgrate offers a unified API solution that simplifies integration processes across various platforms, including Cloze. By leveraging Endgrate, you can:
- Save time and resources by outsourcing integration management, allowing you to focus on your core product development.
- Build once for each use case, reducing the need for multiple integrations for different platforms.
- Provide an intuitive and seamless integration experience for your customers.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate.
Read More
Ready to get started?