Using the Postgres API to Create or Update Records (with PHP examples)
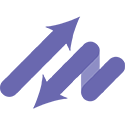
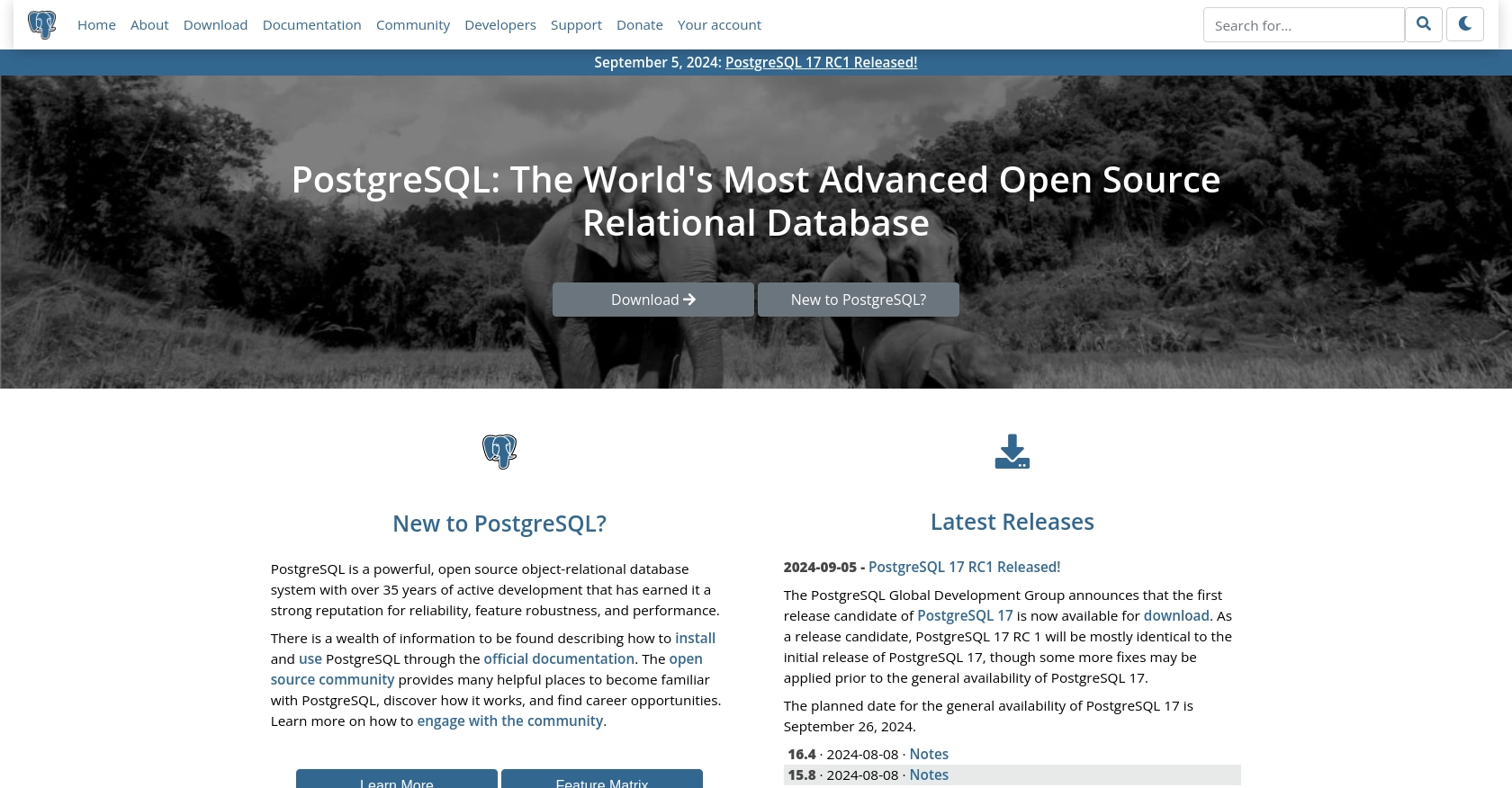
Introduction to PostgreSQL API Integration
PostgreSQL, often referred to as Postgres, is a powerful, open-source relational database management system known for its robustness, scalability, and compliance with SQL standards. It is widely used by developers and organizations to manage complex data workloads efficiently.
Integrating with the PostgreSQL API allows developers to automate database operations such as creating or updating records programmatically. This can be particularly useful in scenarios where applications need to interact with the database dynamically. For example, a developer might want to update user information in a PostgreSQL database whenever a user updates their profile on a web application.
This article will guide you through the process of using PHP to interact with the PostgreSQL API, focusing on creating and updating records. By the end of this tutorial, you will have a clear understanding of how to leverage PHP to perform these operations seamlessly with PostgreSQL.
Setting Up a PostgreSQL Test Environment
Before diving into the integration process, it's essential to set up a PostgreSQL test environment. This will allow you to safely experiment with creating and updating records without affecting your production data.
Installing PostgreSQL Locally
To begin, you need to install PostgreSQL on your local machine. Follow these steps:
- Visit the PostgreSQL download page and choose the appropriate version for your operating system.
- Follow the installation instructions provided for your OS to complete the setup.
- Once installed, use the PostgreSQL command-line tool,
psql
, to interact with your database.
Creating a Test Database
After installing PostgreSQL, create a test database to work with:
CREATE DATABASE test_db;
Use the following command in psql
to create a new database named test_db
.
Setting Up User Authentication
PostgreSQL uses role-based authentication. Create a new user role for your test environment:
CREATE USER test_user WITH PASSWORD 'securepassword';
GRANT ALL PRIVILEGES ON DATABASE test_db TO test_user;
This creates a user test_user
with access to test_db
.
Configuring PHP for PostgreSQL
Ensure PHP is configured to connect to PostgreSQL:
- Install the PHP PostgreSQL extension by running
sudo apt-get install php-pgsql
on Linux or using the appropriate package manager for your OS. - Verify the extension is enabled by checking your
php.ini
file.
With your PostgreSQL test environment set up, you're ready to start integrating with the database using PHP.
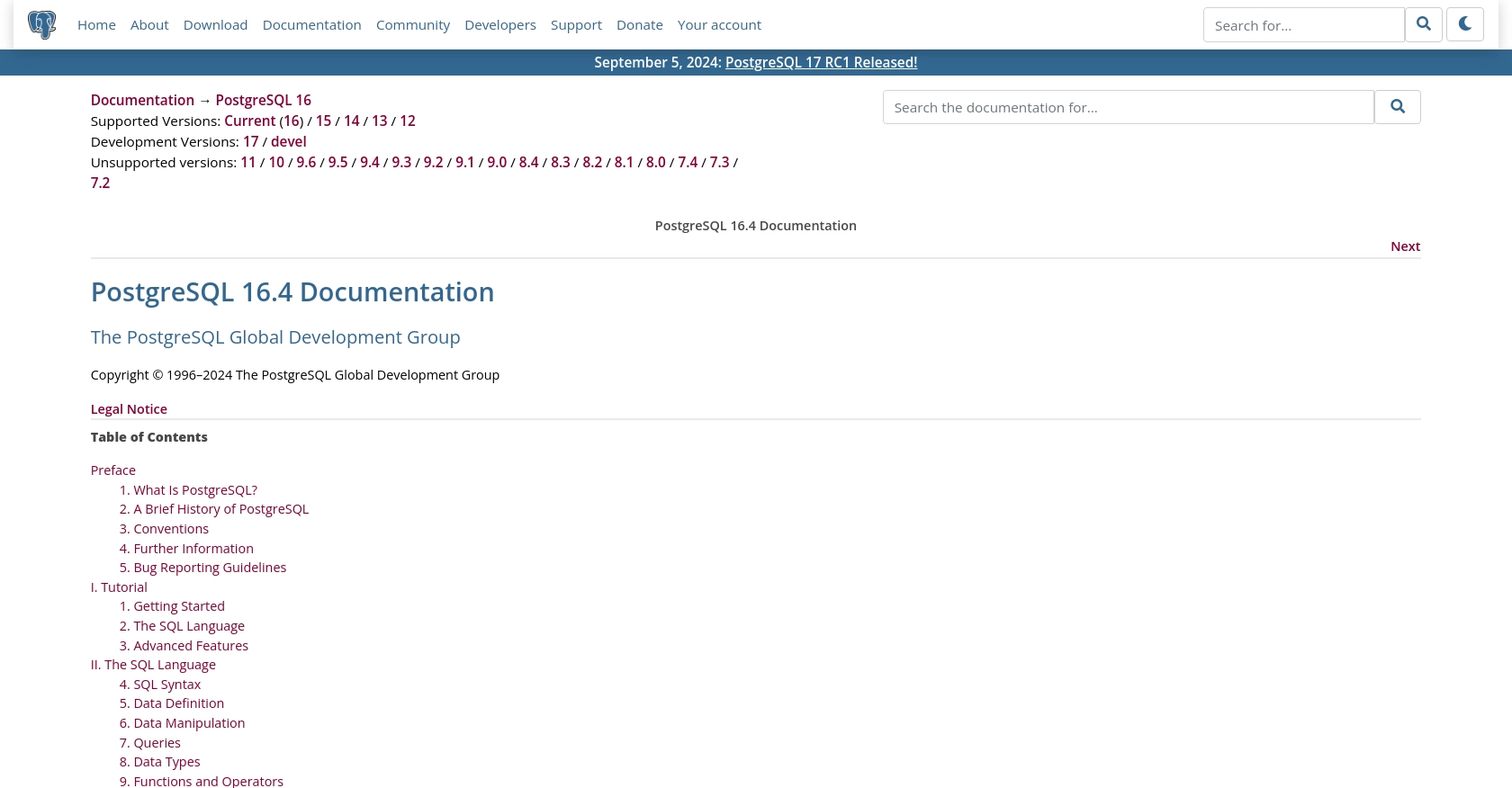
sbb-itb-96038d7
Executing API Calls to Create or Update Records in PostgreSQL Using PHP
To interact with PostgreSQL databases programmatically, PHP provides a robust set of functions that allow developers to execute SQL queries directly from their applications. This section will guide you through the process of creating and updating records in a PostgreSQL database using PHP.
Prerequisites for PHP and PostgreSQL Integration
Before proceeding, ensure you have the following prerequisites:
- PHP 7.4 or higher installed on your system.
- The PHP PostgreSQL extension enabled, which allows PHP to communicate with PostgreSQL databases.
- A PostgreSQL database and user set up as described in the previous section.
Connecting to a PostgreSQL Database Using PHP
To begin, establish a connection to your PostgreSQL database using PHP's pg_connect
function. This function requires a connection string that includes the host, database name, user, and password.
This code snippet connects to the test_db
database using the credentials provided. Ensure that the connection is successful before proceeding with any database operations.
Creating Records in PostgreSQL Using PHP
To create a new record in a PostgreSQL table, use the pg_query
function to execute an SQL INSERT
statement. Below is an example of how to insert a new user record into a table named users
.
This code inserts a new user with the username newuser
and email newuser@example.com
into the users
table. Check the result of the query to ensure the operation was successful.
Updating Records in PostgreSQL Using PHP
To update an existing record, use the pg_query
function with an SQL UPDATE
statement. The following example updates the email of a user in the users
table.
This code updates the email address of the user with the username newuser
. As with the insert operation, verify the result to confirm the update was successful.
Handling Errors and Verifying Operations
It's crucial to handle potential errors when interacting with the database. Use the pg_last_error
function to retrieve error messages if a query fails.
Additionally, verify the changes in your PostgreSQL test environment to ensure the records have been created or updated as expected.
Conclusion: Best Practices for PostgreSQL API Integration with PHP
Integrating with the PostgreSQL API using PHP offers a powerful way to automate database operations, enhancing the efficiency and functionality of your applications. By following the steps outlined in this article, you can create and update records in your PostgreSQL database seamlessly.
Best Practices for Secure and Efficient PostgreSQL Integration
- Secure User Credentials: Always store database credentials securely. Use environment variables or secure vaults to manage sensitive information.
- Handle Errors Gracefully: Implement robust error handling using
pg_last_error
to capture and log errors, ensuring smooth application performance. - Optimize Queries: Regularly review and optimize your SQL queries to improve performance and reduce load on the database.
- Manage Connections: Use connection pooling to efficiently manage database connections, reducing overhead and improving response times.
- Data Validation: Validate and sanitize all inputs to prevent SQL injection and ensure data integrity.
Rate Limiting and Performance Considerations
While PostgreSQL does not impose strict rate limits, it's important to consider the performance impact of frequent API calls. Implement caching strategies and batch processing to minimize database load and enhance application responsiveness.
Transforming and Standardizing Data
Ensure that data is consistently formatted and standardized across your application. This practice simplifies data manipulation and enhances compatibility with other systems.
Streamlining Integrations with Endgrate
For developers looking to simplify and scale their integration efforts, consider leveraging Endgrate. By providing a unified API endpoint, Endgrate allows you to connect with multiple platforms, including PostgreSQL, with ease. This approach saves time and resources, enabling you to focus on your core product development.
Explore how Endgrate can transform your integration process by visiting Endgrate and discover a more efficient way to manage your integrations.
Read More
Ready to get started?