Using the Apollo API to Get Contacts (with Python examples)
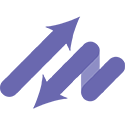
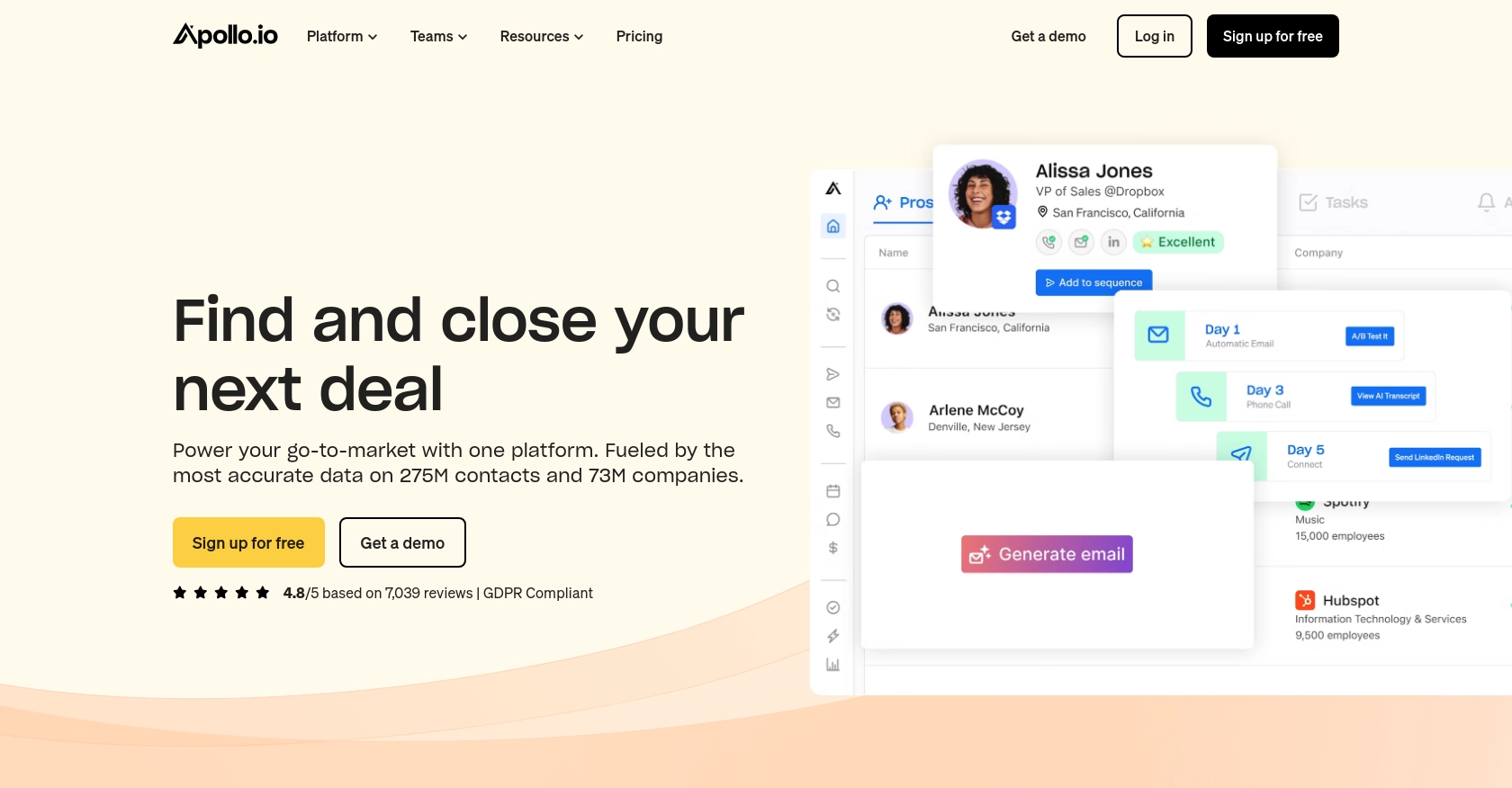
Introduction to Apollo API for Contact Management
Apollo is a powerful sales intelligence and engagement platform that helps businesses streamline their sales processes by providing access to a vast database of contacts and companies. With Apollo, sales teams can enhance their outreach efforts, improve lead generation, and optimize their sales strategies.
Developers might want to integrate with Apollo's API to automate and manage contact data efficiently. By leveraging the Apollo API, you can retrieve detailed contact information, enabling personalized communication and targeted marketing campaigns. For example, a developer could use the Apollo API to fetch contact details and integrate them into a CRM system, ensuring that sales teams have up-to-date information at their fingertips.
Setting Up Your Apollo API Test Account
Before you can start using the Apollo API to manage contacts, you'll need to set up a test account. This involves obtaining an API key, which will allow you to authenticate your requests and access Apollo's vast database of contacts.
Creating an Apollo Account
If you don't already have an Apollo account, you can sign up for a free trial on the Apollo website. This will give you access to the platform's features and allow you to explore its capabilities.
- Visit the Apollo website and click on "Sign Up."
- Follow the prompts to create your account, providing the necessary information.
- Once your account is set up, log in to access the Apollo dashboard.
Generating an Apollo API Key
To authenticate your API requests, you'll need to generate an API key. Follow these steps to obtain your key:
- Log in to your Apollo account and navigate to the API settings section.
- Click on "Generate API Key" to create a new key for your application.
- Copy the generated API key and store it securely, as you'll need it for authentication in your API requests.
Configuring API Key Authentication
With your API key in hand, you can now configure your requests to authenticate with Apollo's API. The API key should be included in the headers of your requests as follows:
import requests
url = "https://api.apollo.io/v1/contacts"
headers = {
'Cache-Control': 'no-cache',
'Content-Type': 'application/json',
'X-Api-Key': 'YOUR_API_KEY_HERE'
}
response = requests.get(url, headers=headers)
print(response.text)
Replace YOUR_API_KEY_HERE
with the API key you generated. This setup will allow you to authenticate your requests and interact with the Apollo API.
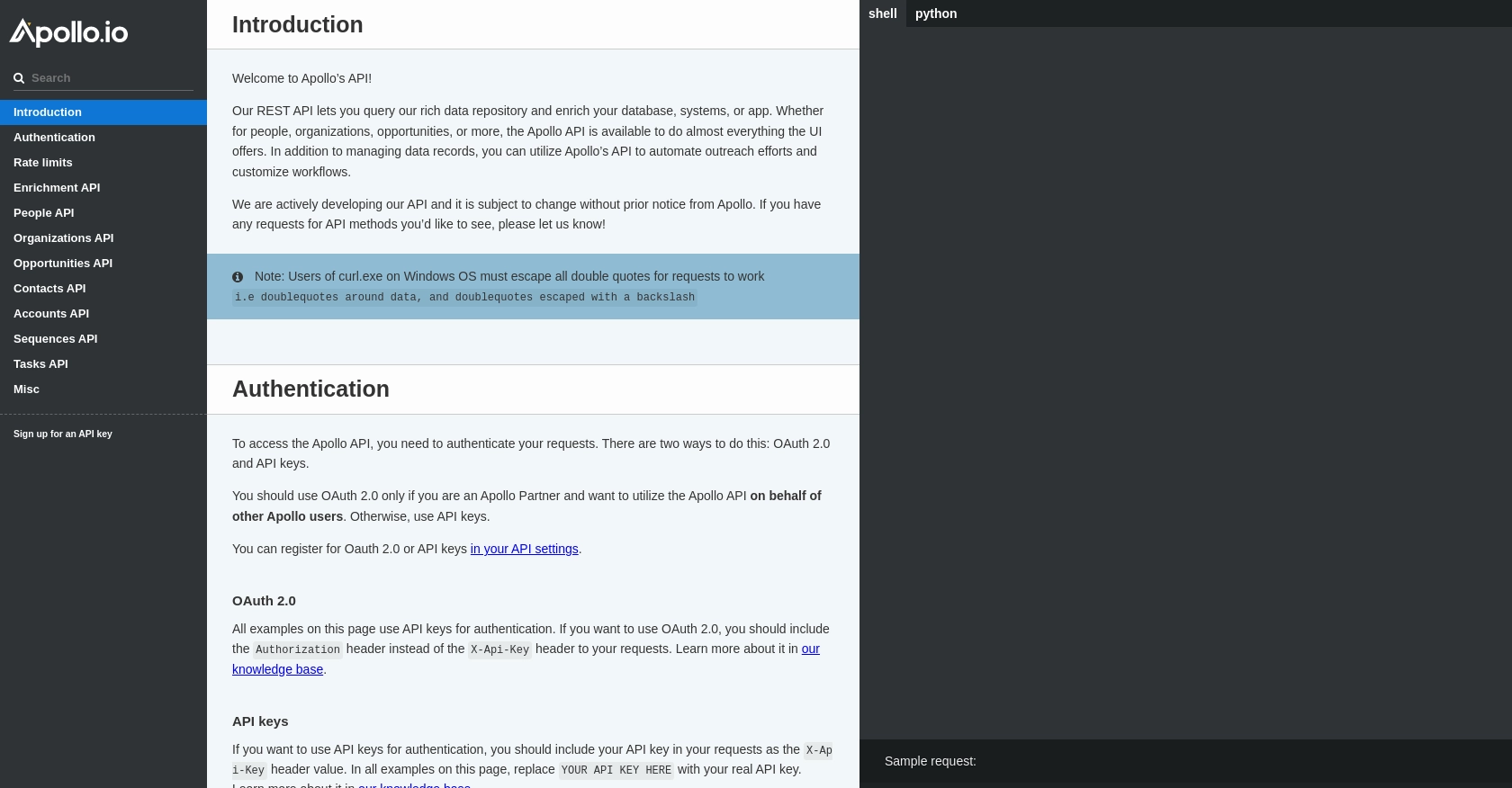
sbb-itb-96038d7
Making API Calls to Retrieve Contacts Using Apollo API with Python
To interact with the Apollo API and retrieve contact information, you'll need to set up your Python environment and make HTTP requests to the appropriate endpoints. This section will guide you through the process of making API calls using Python, ensuring you can efficiently access and manage contact data.
Setting Up Your Python Environment for Apollo API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests. Install it using pip:
pip install requests
Example Code to Retrieve Contacts from Apollo API
With your environment ready, you can now write a Python script to fetch contacts from Apollo. Create a file named get_apollo_contacts.py
and add the following code:
import requests
# Define the API endpoint and headers
url = "https://api.apollo.io/v1/contacts"
headers = {
'Cache-Control': 'no-cache',
'Content-Type': 'application/json',
'X-Api-Key': 'YOUR_API_KEY_HERE'
}
# Make a GET request to the API
response = requests.get(url, headers=headers)
# Check if the request was successful
if response.status_code == 200:
data = response.json()
for contact in data.get("contacts", []):
print(contact)
else:
print(f"Failed to retrieve contacts: {response.status_code}")
Replace YOUR_API_KEY_HERE
with your actual API key. This script sends a GET request to the Apollo API to retrieve contact data. If successful, it prints out the contact details.
Handling API Response and Errors
It's crucial to handle potential errors when making API requests. The above code checks the response status code to ensure the request was successful. If not, it prints an error message. You can further enhance error handling by checking for specific error codes and implementing retries or alternative actions as needed.
Verifying API Call Success in Apollo Dashboard
After running your script, verify the retrieved contacts by checking your Apollo dashboard. This ensures that the data fetched matches the records in your Apollo account.
Understanding Apollo API Rate Limits
Be mindful of Apollo's API rate limits to avoid exceeding the allowed number of requests. According to Apollo's documentation, the rate limits are as follows:
- 50 requests per minute
- 100 requests per hour
- 300 requests per day
Monitor your API usage and implement rate limiting strategies to stay within these limits. For more details, refer to the Apollo API documentation.
Conclusion and Best Practices for Using Apollo API with Python
Integrating with the Apollo API offers a robust solution for developers looking to enhance their contact management capabilities. By leveraging the API, you can automate data retrieval and streamline your sales processes, ensuring that your team has access to the most current and relevant contact information.
Best Practices for Secure and Efficient API Usage
- Secure API Key Storage: Always store your API keys securely. Avoid hardcoding them in your scripts. Instead, use environment variables or secure vaults to manage sensitive information.
- Implement Rate Limiting: Be mindful of Apollo's rate limits (50 requests per minute, 100 requests per hour, 300 requests per day). Implement strategies to manage your API calls effectively, such as batching requests or using exponential backoff in case of rate limit errors.
- Error Handling: Enhance your error handling by checking for specific HTTP status codes and implementing retries or alternative actions. This ensures your application can gracefully handle API errors and maintain functionality.
- Data Standardization: When integrating contact data into your systems, ensure that data fields are standardized and consistent. This will facilitate better data management and reporting.
Streamlining Integrations with Endgrate
While integrating with the Apollo API can significantly enhance your contact management processes, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including Apollo.
By using Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing your team to focus on core product development.
- Build once for each use case, rather than multiple times for different integrations.
- Provide an intuitive and seamless integration experience for your customers.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate.
Read More
Ready to get started?