Using the Sap Business One API to Get Purchase Orders in PHP
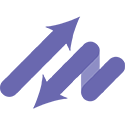
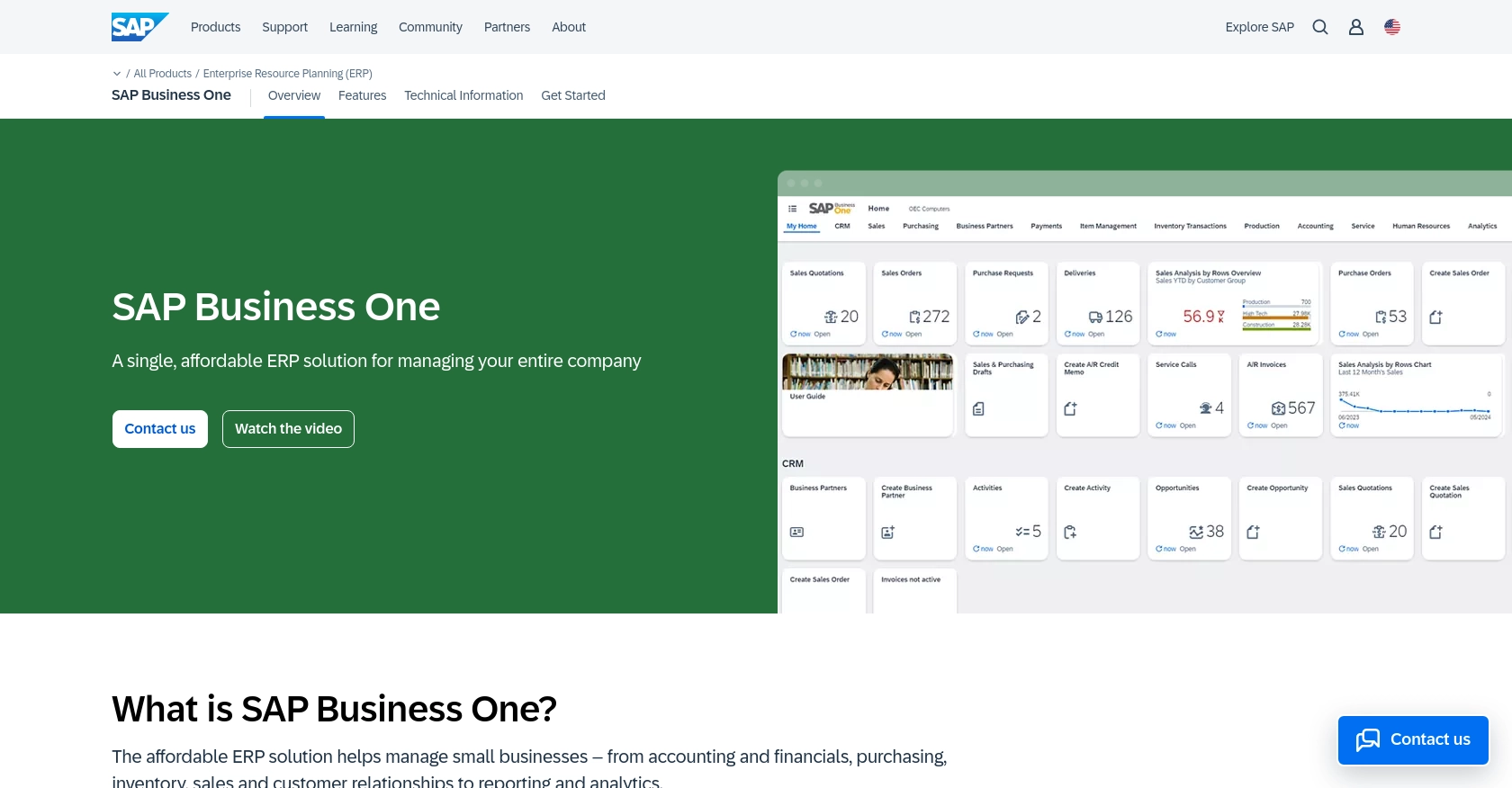
Introduction to SAP Business One API
SAP Business One is a comprehensive enterprise resource planning (ERP) solution designed for small and medium-sized businesses. It offers a wide range of functionalities, including financial management, sales, customer relationship management, and supply chain management, all integrated into a single platform.
For developers, integrating with the SAP Business One API can significantly enhance business operations by automating processes and accessing critical business data. For example, retrieving purchase orders using the SAP Business One API in PHP can streamline procurement workflows, allowing businesses to efficiently manage their supply chain and inventory levels.
Setting Up Your SAP Business One Test/Sandbox Account
Before you can begin interacting with the SAP Business One API, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting your live data.
Creating a SAP Business One Sandbox Account
To get started, you need access to a SAP Business One sandbox environment. If your organization already uses SAP Business One, you can request access to a sandbox instance from your system administrator. If not, you may need to contact an SAP partner or representative to set up a trial or demo account.
Configuring OAuth Authentication for SAP Business One API
SAP Business One uses a custom authentication method for API access. Follow these steps to configure your authentication:
- Log in to your SAP Business One sandbox environment.
- Navigate to the administration section and select the option to manage API access.
- Create a new application and note down the client ID and client secret provided.
- Set the necessary permissions for accessing purchase orders, ensuring your application has the required scopes.
For more detailed instructions, refer to the SAP Business One Service Layer documentation.
Generating API Keys for SAP Business One
In addition to OAuth, you may need to generate API keys for additional security:
- Within the API access management section, locate the API key generation option.
- Generate a new API key and store it securely, as it will be required for authenticating your API requests.
With your sandbox account and authentication details ready, you can now proceed to make API calls to retrieve purchase orders using PHP.
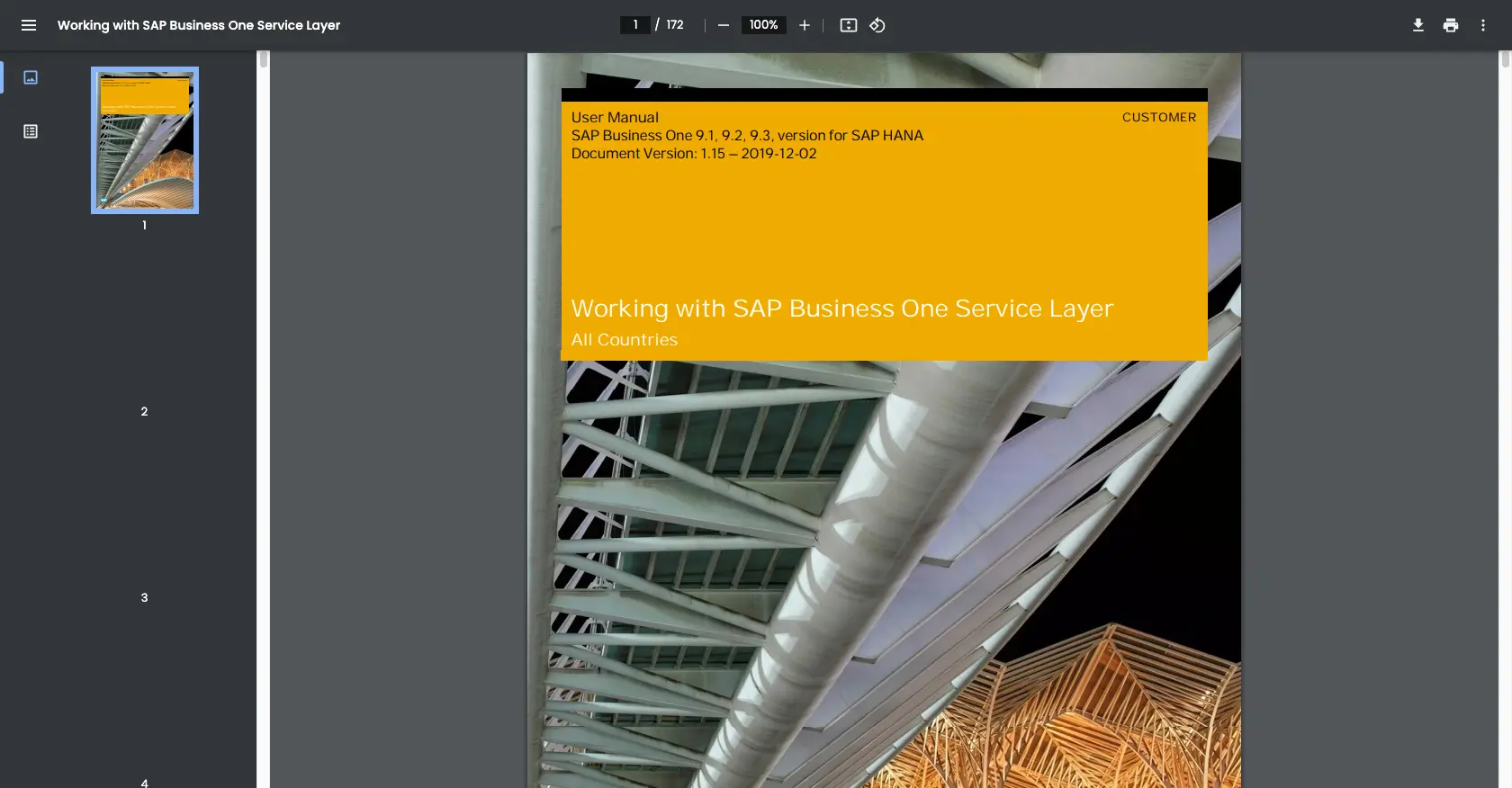
sbb-itb-96038d7
Making API Calls to Retrieve Purchase Orders from SAP Business One Using PHP
To interact with the SAP Business One API and retrieve purchase orders, you'll need to use PHP, a popular server-side scripting language. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your PHP Environment for SAP Business One API Integration
Before making API calls, ensure your development environment is properly configured:
- Install PHP 7.4 or later on your machine.
- Ensure you have Composer, the PHP dependency manager, installed.
- Use Composer to install the Guzzle HTTP client, which simplifies making HTTP requests:
composer require guzzlehttp/guzzle
Writing PHP Code to Retrieve Purchase Orders from SAP Business One
With your environment set up, you can now write the PHP code to interact with the SAP Business One API:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$apiUrl = 'https://your-sap-business-one-instance.com/b1s/v1/PurchaseOrders';
$apiKey = 'Your_API_Key';
$clientId = 'Your_Client_ID';
$clientSecret = 'Your_Client_Secret';
$response = $client->request('GET', $apiUrl, [
'headers' => [
'Authorization' => 'Bearer ' . $apiKey,
'Content-Type' => 'application/json'
]
]);
if ($response->getStatusCode() === 200) {
$purchaseOrders = json_decode($response->getBody(), true);
foreach ($purchaseOrders['value'] as $order) {
echo 'Order ID: ' . $order['DocEntry'] . "\n";
echo 'Supplier: ' . $order['CardName'] . "\n";
echo 'Total: ' . $order['DocTotal'] . "\n\n";
}
} else {
echo 'Failed to retrieve purchase orders. Status code: ' . $response->getStatusCode();
}
Replace Your_API_Key
, Your_Client_ID
, and Your_Client_Secret
with your actual credentials. This script uses Guzzle to send a GET request to the SAP Business One API endpoint for purchase orders. If successful, it will print out details of each purchase order.
Verifying API Call Success and Handling Errors
After running the script, verify the output by checking your SAP Business One sandbox account to ensure the purchase orders match the returned data. If the request fails, the script will output the HTTP status code, which can help diagnose issues.
Common error codes include:
- 401 Unauthorized: Check your API key and authentication credentials.
- 403 Forbidden: Ensure your application has the necessary permissions.
- 500 Internal Server Error: This may indicate a server issue; try again later.
Refer to the SAP Business One Service Layer documentation for more detailed error handling guidance.
Conclusion and Best Practices for SAP Business One API Integration
Integrating with the SAP Business One API using PHP can greatly enhance your business operations by automating procurement processes and providing real-time access to purchase order data. By following the steps outlined in this guide, you can efficiently retrieve purchase orders and handle potential errors effectively.
Best Practices for Secure and Efficient SAP Business One API Usage
- Secure Storage of Credentials: Always store your API keys, client ID, and client secret securely. Consider using environment variables or a secure vault to protect sensitive information.
- Handling Rate Limits: Be mindful of any rate limits imposed by the SAP Business One API. Implement logic to handle rate limiting gracefully, such as retry mechanisms with exponential backoff.
- Data Transformation and Standardization: Ensure that the data retrieved from the API is transformed and standardized to fit your application's requirements. This can help maintain consistency across different systems.
Streamlining Integrations with Endgrate
While building integrations with SAP Business One can be rewarding, it can also be time-consuming and complex. Endgrate offers a solution by providing a unified API endpoint that connects to multiple platforms, including SAP Business One. By using Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations, simplifying your development process.
- Provide an intuitive integration experience for your customers, enhancing user satisfaction and engagement.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate's website and discover the benefits of a unified integration approach.
Read More
Ready to get started?