Using the Copper API to Get Users in Javascript
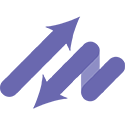
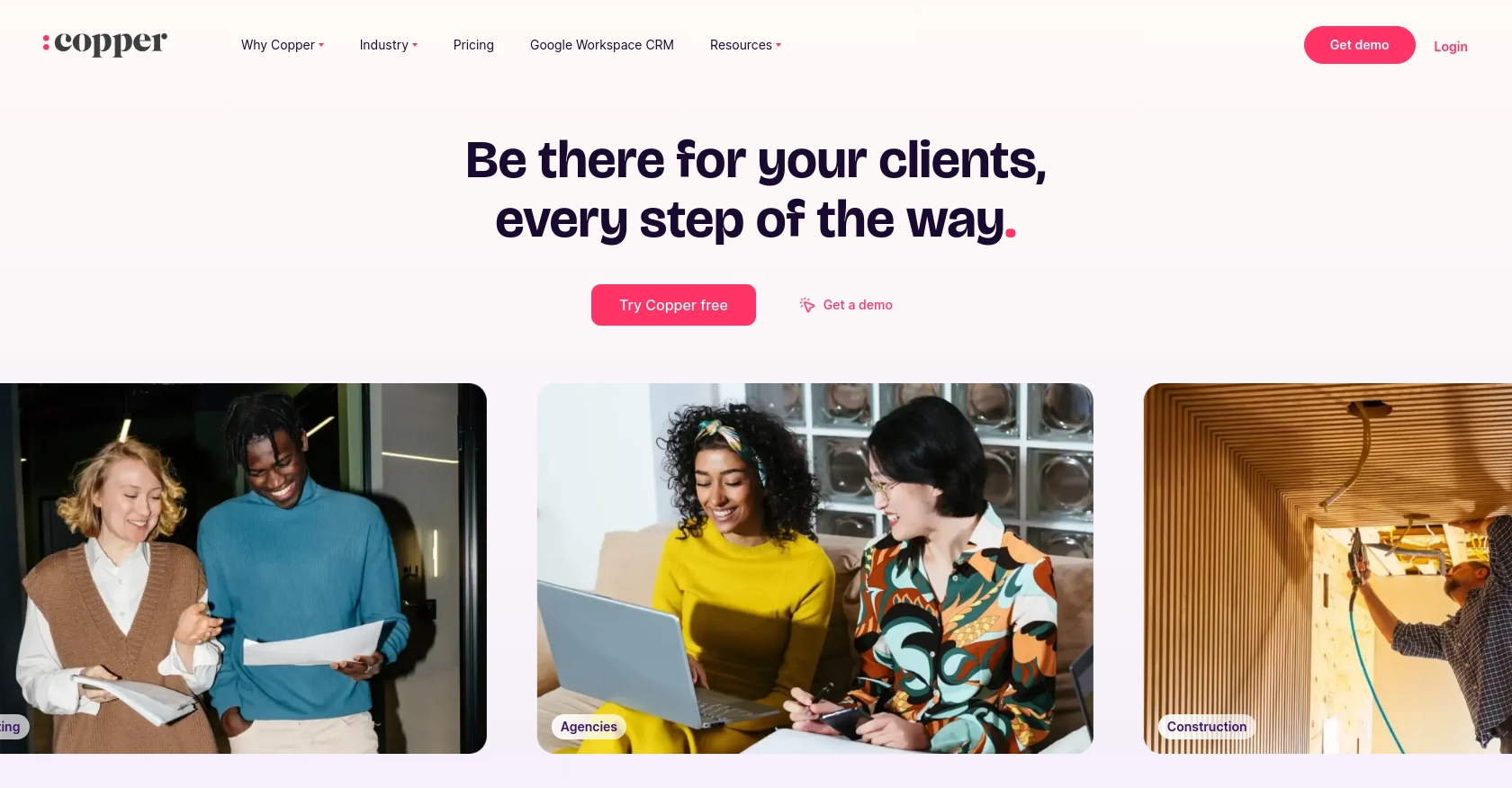
Introduction to Copper API
Copper is a robust platform offering a suite of tools for managing digital assets and financial transactions. It provides a secure and efficient way for businesses to handle cryptocurrency operations, making it a preferred choice for organizations looking to streamline their digital asset management.
Developers may want to integrate with Copper's API to automate and enhance their financial processes. For example, using the Copper API, developers can retrieve user data to manage accounts and transactions more effectively. This integration can be particularly useful for businesses that need to maintain up-to-date user information for compliance and operational efficiency.
Setting Up Your Copper Sandbox Account for API Integration
Before you can start using the Copper API to retrieve user data, you'll need to set up a sandbox account. This will allow you to test your integration without affecting live data. Copper provides a demo environment specifically for this purpose, ensuring that developers can experiment and refine their API interactions safely.
Creating a Copper Sandbox Account
- Visit the Copper website and sign up for a demo account. This will give you access to the sandbox environment where you can test API calls.
- Follow the on-screen instructions to complete the registration process. Ensure you verify your email address to activate the account.
Generating API Keys for Copper Sandbox
Once your sandbox account is set up, you'll need to generate API keys to authenticate your requests:
- Log in to your Copper sandbox account.
- Navigate to the API settings section, typically found under the 'Developer' or 'Integrations' tab.
- Click on 'Create API Key' and fill in the necessary details, such as the name and permissions for the key.
- After creating the key, make sure to copy the API key and secret. Store them securely, as you'll need them for authentication in your API requests.
Understanding Copper API Authentication
Copper uses a custom authentication method that requires an API key and a secret. Here's how you can set up your authentication:
// Example of setting up authentication headers in JavaScript
const apiKey = 'your_api_key';
const apiSecret = 'your_api_secret';
const timestamp = Date.now().toString();
const method = 'GET';
const path = '/platform/users';
const body = '';
const signature = CryptoJS.HmacSHA256(timestamp + method + path + body, apiSecret).toString();
const headers = {
'Authorization': `ApiKey ${apiKey}`,
'X-Signature': signature,
'X-Timestamp': timestamp,
'Content-Type': 'application/json'
};
Ensure you replace your_api_key
and your_api_secret
with the actual values from your Copper sandbox account.
Testing Your Copper API Setup
With your sandbox account and API keys ready, you can now test your setup by making a simple API call to retrieve user data:
// Example API call to get users
fetch('https://api.stage.copper.co/platform/users', {
method: 'GET',
headers: headers
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
This code snippet demonstrates how to make a GET request to the Copper API to fetch user information. If everything is set up correctly, you should receive a JSON response with user data from your sandbox environment.
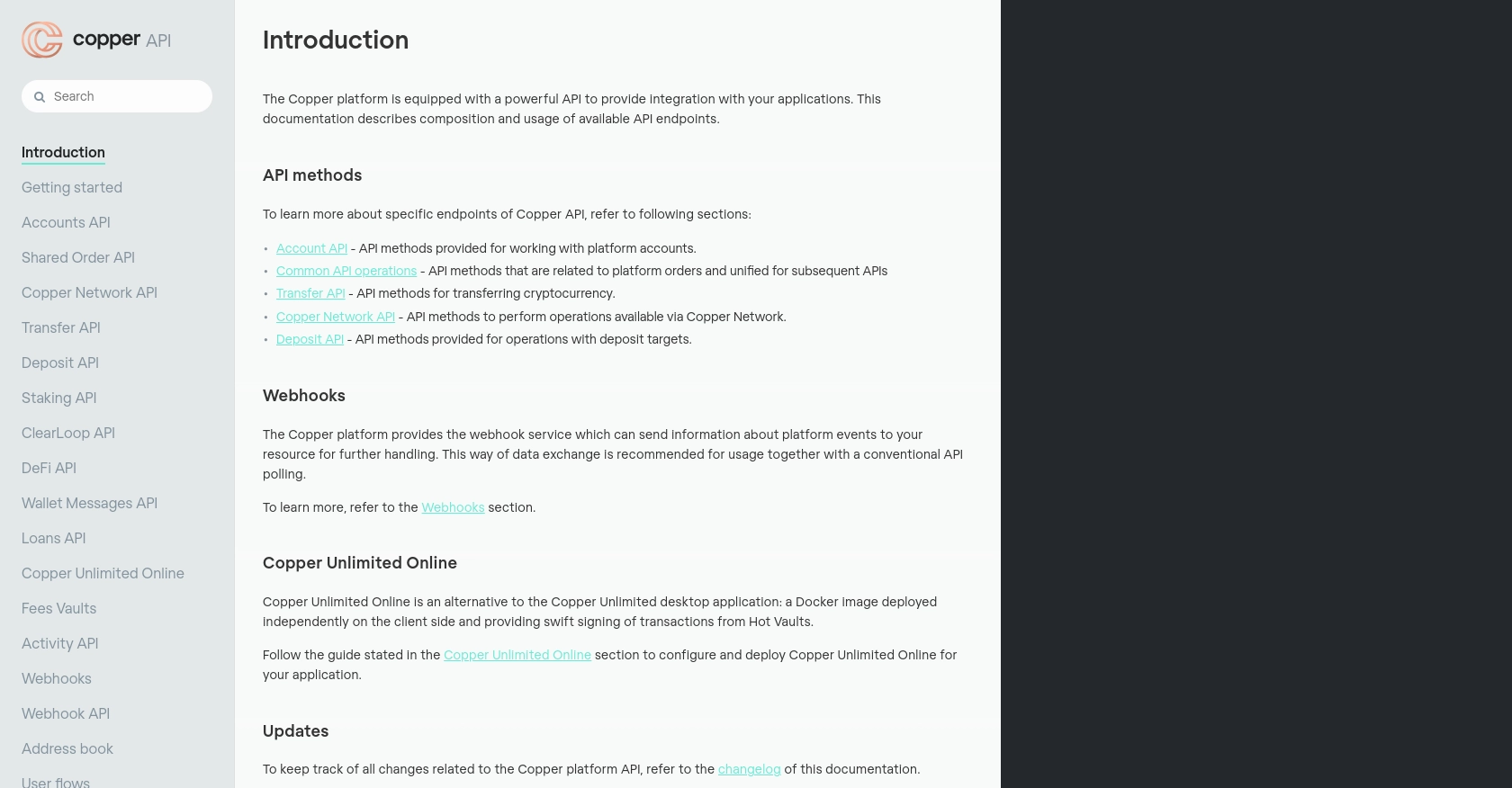
sbb-itb-96038d7
Executing Copper API Calls in JavaScript
To effectively interact with the Copper API using JavaScript, it's essential to ensure your development environment is properly configured. This section will guide you through the necessary steps to make successful API calls, including setting up your JavaScript environment and handling potential errors.
Setting Up Your JavaScript Environment for Copper API
Before making API calls, ensure you have Node.js installed on your machine. This will allow you to run JavaScript code outside of a browser environment. Additionally, you'll need to install the node-fetch
package to make HTTP requests.
npm install node-fetch
Making a GET Request to Retrieve Copper Users
Once your environment is set up, you can proceed to make a GET request to the Copper API to retrieve user data. Here's a step-by-step guide:
- Import the
node-fetch
package in your JavaScript file. - Set up the authentication headers using your API key and secret.
- Make the API call and handle the response.
const fetch = require('node-fetch');
const CryptoJS = require('crypto-js');
const apiKey = 'your_api_key';
const apiSecret = 'your_api_secret';
const timestamp = Date.now().toString();
const method = 'GET';
const path = '/platform/users';
const body = '';
const signature = CryptoJS.HmacSHA256(timestamp + method + path + body, apiSecret).toString();
const headers = {
'Authorization': `ApiKey ${apiKey}`,
'X-Signature': signature,
'X-Timestamp': timestamp,
'Content-Type': 'application/json'
};
fetch('https://api.stage.copper.co/platform/users', {
method: 'GET',
headers: headers
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Replace your_api_key
and your_api_secret
with your actual Copper API credentials. This code snippet demonstrates how to authenticate and make a GET request to fetch user data from Copper.
Handling Errors and Verifying API Call Success
It's crucial to handle errors gracefully and verify the success of your API requests. Check the HTTP status code in the response to determine if the request was successful. Copper API typically returns a 2xx status code for successful requests.
fetch('https://api.stage.copper.co/platform/users', {
method: 'GET',
headers: headers
})
.then(response => {
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
return response.json();
})
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
This enhanced code snippet includes error handling to catch and log any issues that arise during the API call, ensuring you can debug effectively.
Verifying Data in Copper Sandbox Environment
After making the API call, verify the returned data by checking your Copper sandbox account. Ensure that the user data retrieved matches the expected results in your sandbox environment. This step confirms that your API integration is functioning as intended.
Conclusion and Best Practices for Using Copper API in JavaScript
Integrating with the Copper API using JavaScript offers a powerful way to automate and enhance your digital asset management processes. By following the steps outlined in this guide, you can efficiently retrieve user data and ensure your integration is both secure and effective.
Best Practices for Secure Copper API Integration
- Secure Storage of API Credentials: Always store your API key and secret securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of Copper's API rate limits to avoid disruptions. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Validation and Sanitization: Ensure that any data retrieved from the API is validated and sanitized before use to prevent security vulnerabilities.
Optimizing Copper API Calls for Performance
- Efficient Data Handling: Use pagination when retrieving large datasets to minimize load times and reduce the risk of timeouts.
- Asynchronous Processing: Leverage JavaScript's asynchronous capabilities to handle API responses efficiently without blocking the main execution thread.
Enhancing User Experience with Copper API
By integrating Copper API into your applications, you can provide users with real-time access to their digital asset data, improving transparency and operational efficiency. Ensure that your application interface is intuitive and responsive to enhance user satisfaction.
Call to Action: Streamline Your Integrations with Endgrate
For developers looking to simplify and scale their integration efforts, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Take advantage of a unified API experience that connects seamlessly with multiple platforms, including Copper. Visit Endgrate to learn more and start optimizing your integration processes today.
Read More
Ready to get started?