Using the Sap Business One API to Create or Update Purchase Orders in Javascript
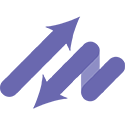
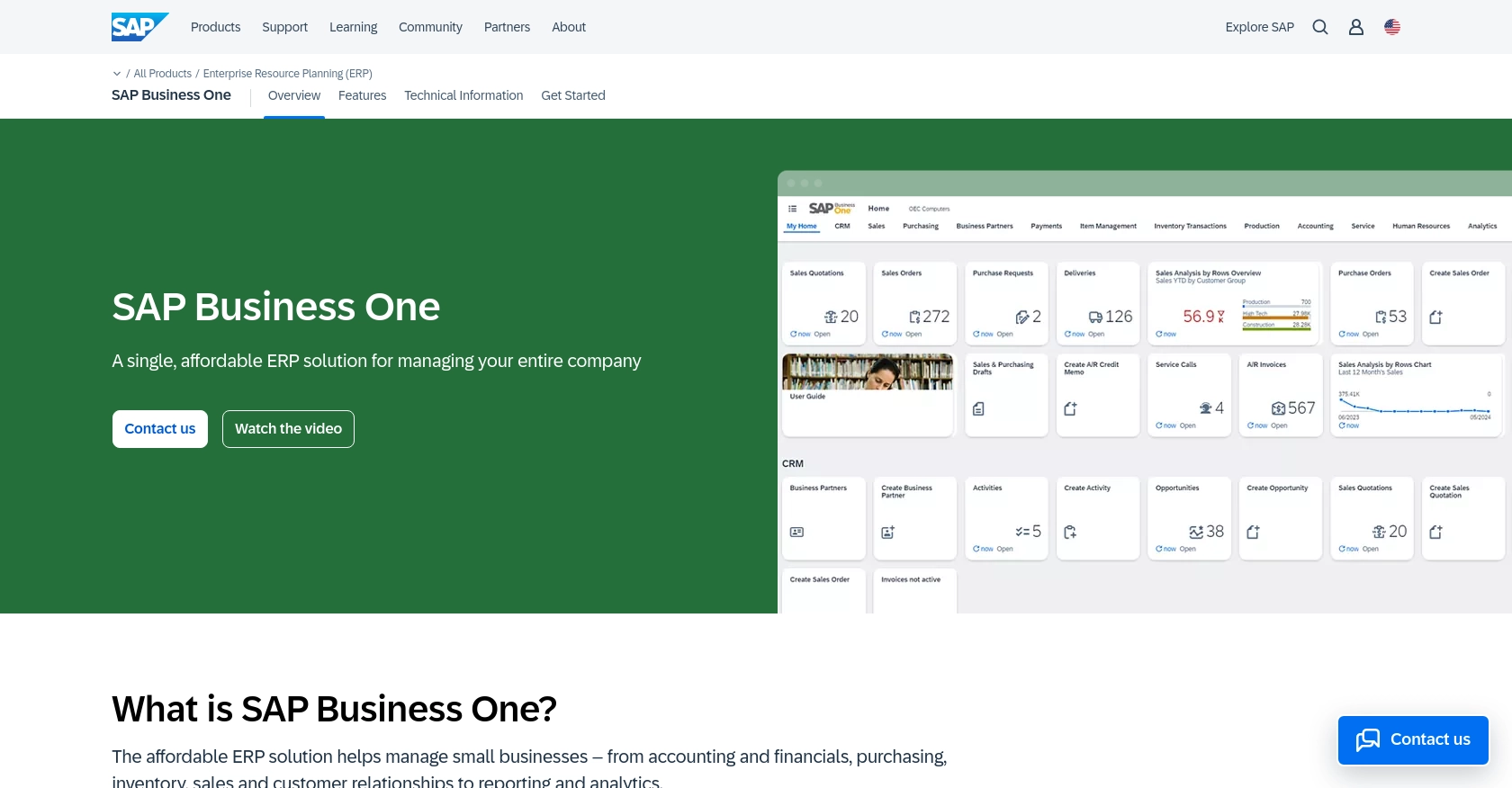
Introduction to SAP Business One
SAP Business One is a comprehensive enterprise resource planning (ERP) solution tailored for small to medium-sized businesses. It offers a wide range of functionalities, including financial management, sales, customer relationship management, and supply chain operations, all integrated into a single platform.
For developers, integrating with the SAP Business One API can significantly enhance business processes by automating tasks such as creating or updating purchase orders. This integration allows businesses to streamline procurement workflows, reduce manual data entry, and ensure data consistency across systems.
In this article, we will explore how to use JavaScript to interact with the SAP Business One API, focusing on creating or updating purchase orders. This guide will provide step-by-step instructions to help you efficiently manage purchase orders within the SAP Business One environment.
Setting Up Your SAP Business One Test/Sandbox Account
Before diving into the integration process, it's crucial to set up a test or sandbox account with SAP Business One. This environment allows developers to safely experiment with API interactions without affecting live data.
Creating a SAP Business One Sandbox Account
To begin, you need access to a SAP Business One sandbox account. If you don't have one, you can request access through SAP's official channels or contact your SAP Business One provider for a trial or demo account.
Configuring OAuth-Based Authentication for SAP Business One API
SAP Business One uses a custom authentication method. Follow these steps to configure your app for API access:
- Log in to your SAP Business One sandbox account.
- Navigate to the Service Layer section.
- Create a new application by providing necessary details such as the application name and description.
- Once the application is created, note down the Client ID and Client Secret. These credentials are essential for authenticating API requests.
Generating API Keys for SAP Business One
In addition to OAuth credentials, you may need an API key for certain operations. Follow these steps to generate an API key:
- In your SAP Business One sandbox account, go to the API Management section.
- Select Create API Key and fill in the required information.
- After creation, copy the API key and store it securely. This key will be used in your API calls.
With your sandbox account and authentication credentials ready, you can now proceed to interact with the SAP Business One API using JavaScript. This setup ensures a secure and controlled environment for testing and development.
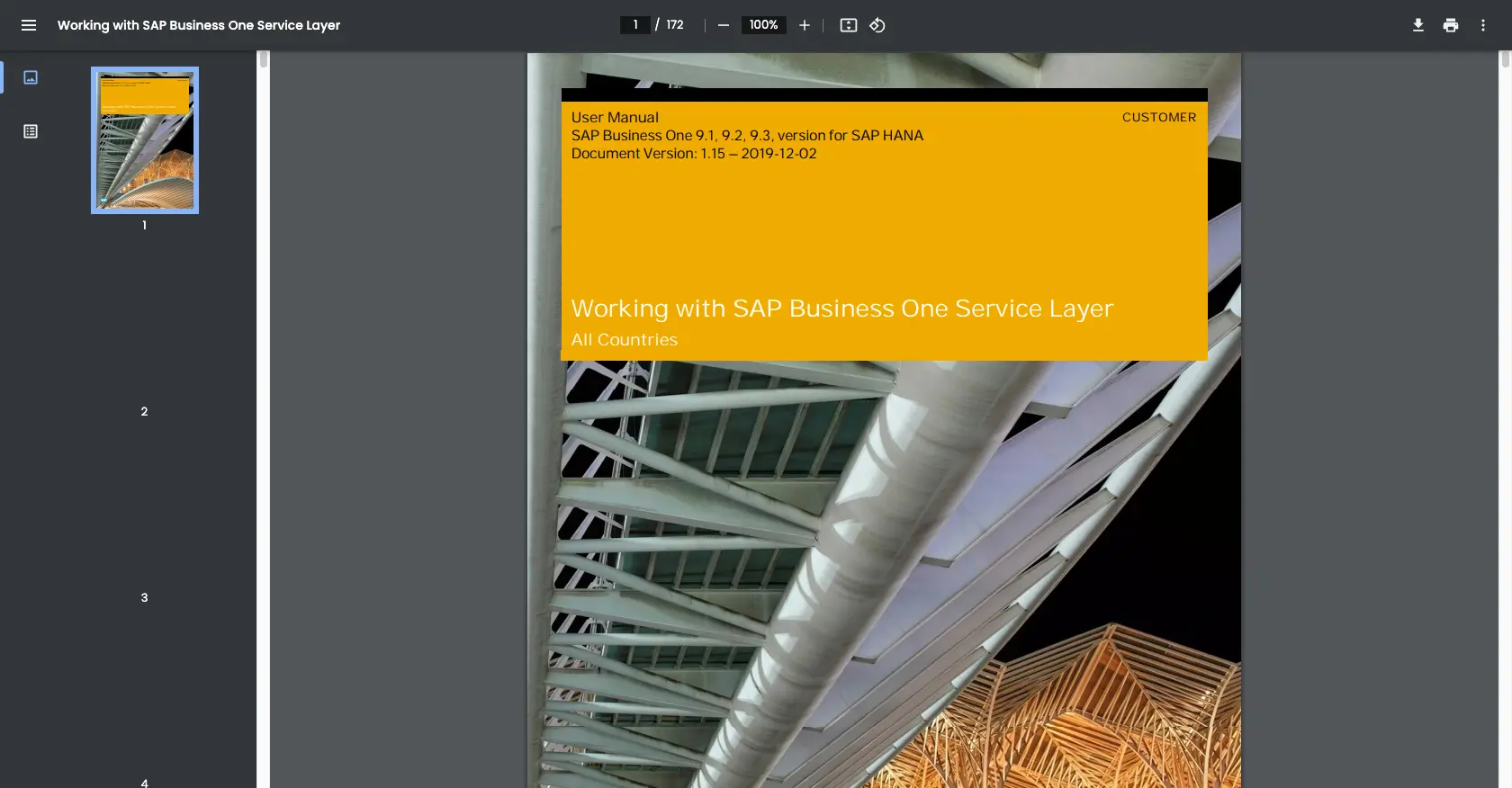
sbb-itb-96038d7
Making API Calls to SAP Business One for Purchase Orders Using JavaScript
To interact with the SAP Business One API for creating or updating purchase orders, you'll need to use JavaScript. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses and errors.
Setting Up Your JavaScript Environment for SAP Business One API Integration
Before you start coding, ensure you have the following prerequisites:
- Node.js installed on your machine. You can download it from the official Node.js website.
- A text editor or IDE, such as Visual Studio Code, to write your JavaScript code.
- The
axios
library for making HTTP requests. Install it using the following command:
npm install axios
Writing JavaScript Code to Create or Update Purchase Orders in SAP Business One
Now, let's write the JavaScript code to interact with the SAP Business One API:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://your-sap-business-one-instance.com/b1s/v1/PurchaseOrders';
const headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer Your_Token'
};
// Define the purchase order data
const purchaseOrderData = {
CardCode: 'C20000',
DocDate: '2023-10-01',
DocumentLines: [
{
ItemCode: 'A00001',
Quantity: 10,
Price: 100
}
]
};
// Function to create or update a purchase order
async function createOrUpdatePurchaseOrder() {
try {
const response = await axios.post(endpoint, purchaseOrderData, { headers });
console.log('Purchase Order Created/Updated Successfully:', response.data);
} catch (error) {
console.error('Error Creating/Updating Purchase Order:', error.response ? error.response.data : error.message);
}
}
// Execute the function
createOrUpdatePurchaseOrder();
Replace Your_Token
with the token obtained from your SAP Business One sandbox account.
Verifying the API Request and Handling Errors
After executing the code, verify the success of your request by checking the SAP Business One sandbox account. The newly created or updated purchase order should appear in the system.
In case of errors, the code handles them by logging the error message. Common error codes include:
- 401 Unauthorized: Check your authentication token.
- 400 Bad Request: Verify the structure of your purchase order data.
For more detailed error information, refer to the SAP Business One Service Layer documentation.
Conclusion and Best Practices for SAP Business One API Integration
Integrating with the SAP Business One API using JavaScript can significantly enhance your business operations by automating the creation and updating of purchase orders. This not only streamlines procurement workflows but also ensures data consistency across your systems.
Best Practices for Secure and Efficient SAP Business One API Usage
- Secure Storage of Credentials: Always store your API keys and tokens securely. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of any rate limits imposed by SAP Business One. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Transformation and Standardization: Ensure that data fields are consistently formatted and standardized before sending them to the API. This helps in maintaining data integrity.
Streamlining Integrations with Endgrate
While integrating with SAP Business One can be highly beneficial, managing multiple integrations can be complex and time-consuming. Endgrate offers a solution by providing a unified API endpoint that connects to various platforms, including SAP Business One.
By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers. Explore how Endgrate can simplify your integration needs by visiting Endgrate's website.
Read More
Ready to get started?