How to Get Users with the Insightly API in Python
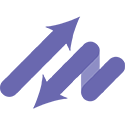
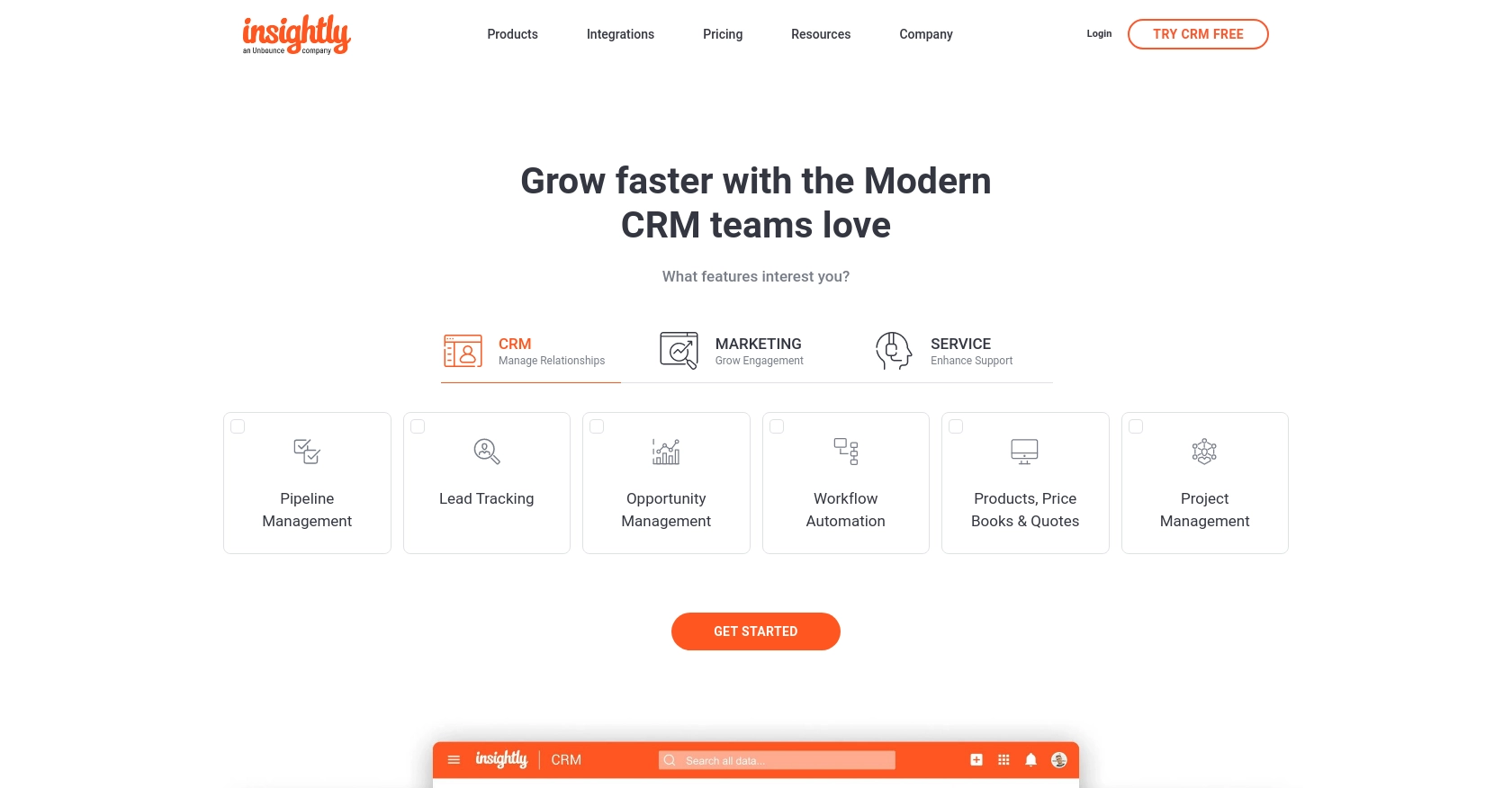
Introduction to Insightly API Integration
Insightly is a powerful CRM platform that offers a range of tools to help businesses manage their customer relationships effectively. With features like project management, contact management, and workflow automation, Insightly is a popular choice for businesses looking to streamline their operations.
Developers may want to integrate with Insightly's API to access and manage user data, enabling seamless synchronization between Insightly and other applications. For example, a developer might use the Insightly API to retrieve user information and integrate it with an internal dashboard, providing real-time insights into customer interactions.
Setting Up Your Insightly Test Account for API Integration
Before you can start using the Insightly API to retrieve user data, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting your live data.
Creating an Insightly Account
If you don't already have an Insightly account, you can sign up for a free trial on the Insightly website. Follow the instructions to create your account. Once your account is set up, you'll be able to access the Insightly dashboard.
Generating Your Insightly API Key
Insightly uses an API key for authentication. Here's how you can generate and obtain your API key:
- Log in to your Insightly account.
- Click on your user profile in the upper right corner and select User Settings.
- Navigate to the API Key section.
- Copy the API key provided. This key will be used to authenticate your API requests.
For more details, refer to the Insightly API key documentation.
Understanding Insightly API Authentication
Insightly uses HTTP Basic authentication. You will need to include your API key as the Base64-encoded username in the Authorization header, leaving the password blank. For testing purposes, you can paste your API key directly into the API key field without encoding.
Testing Your API Key in the Sandbox
To ensure your API key is working correctly, you can test it using the Insightly sandbox environment. This allows you to make API calls without affecting your live data.
Use a tool like Postman to make a simple GET request to the Insightly API. For example, to retrieve a list of users, you can use the following endpoint:
GET: https://api.na1.insightly.com/v3.1/Users
Include your API key in the Authorization header as follows:
Authorization: Basic Your_Base64_Encoded_API_Key
If successful, you should receive a response with user data from your Insightly account.
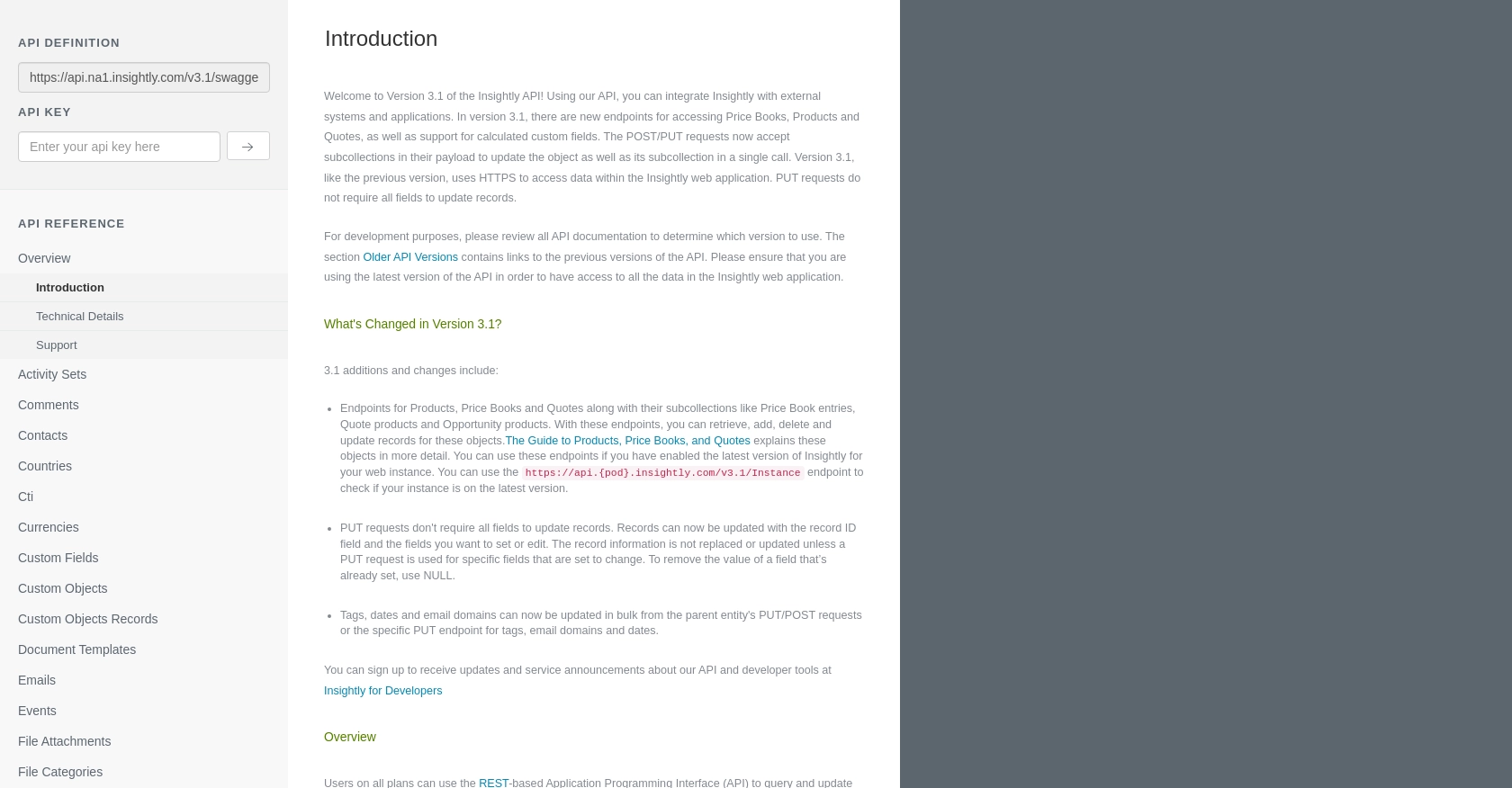
sbb-itb-96038d7
Making API Calls to Retrieve Users with Insightly API in Python
To interact with the Insightly API using Python, you'll need to ensure you have the correct version of Python installed and the necessary dependencies. This section will guide you through setting up your environment and making API calls to retrieve user data from Insightly.
Setting Up Your Python Environment for Insightly API Integration
Before making API calls, ensure you have Python 3.11.1 installed on your machine. You will also need the requests
library to handle HTTP requests. Install it using the following command:
pip install requests
Writing Python Code to Retrieve Users from Insightly API
Create a new Python file named get_insightly_users.py
and add the following code to retrieve user data:
import requests
import base64
# Set the API endpoint
url = "https://api.na1.insightly.com/v3.1/Users"
# Encode the API key
api_key = "Your_API_Key"
encoded_key = base64.b64encode(api_key.encode()).decode()
# Set the request headers
headers = {
"Authorization": f"Basic {encoded_key}",
"Accept-Encoding": "gzip"
}
# Make a GET request to the API
response = requests.get(url, headers=headers)
# Check if the request was successful
if response.status_code == 200:
users = response.json()
for user in users:
print(user)
else:
print(f"Failed to retrieve users: {response.status_code} - {response.text}")
Replace Your_API_Key
with your actual Insightly API key. This script sends a GET request to the Insightly API to retrieve a list of users and prints the user data if the request is successful.
Verifying Successful API Requests and Handling Errors
After running the script, you should see a list of users printed in the console. If the request fails, the script will output an error message with the status code and response text. Common error codes include:
- 401 Unauthorized: Check if your API key is correctly encoded and included in the headers.
- 429 Too Many Requests: You have exceeded the rate limit. Wait before making more requests.
For more information on error codes, refer to the Insightly API documentation.
Testing and Debugging Your Insightly API Integration
To ensure your API integration is working correctly, test your script in the Insightly sandbox environment. Use tools like Postman to simulate API requests and verify responses. This helps in identifying any issues with authentication or request formatting.
By following these steps, you can efficiently retrieve user data from Insightly using Python, enabling seamless integration with your applications.
Conclusion and Best Practices for Insightly API Integration
Integrating with the Insightly API using Python allows developers to efficiently manage and synchronize user data across platforms. By following the steps outlined in this guide, you can seamlessly retrieve user information and enhance your application's functionality.
Best Practices for Secure and Efficient Insightly API Usage
- Securely Store API Keys: Always store your API keys securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limits: Be mindful of Insightly's rate limits, which allow up to 10 requests per second and vary daily based on your plan. Implement retry logic to handle HTTP 429 errors gracefully.
- Data Transformation: Consider transforming and standardizing data fields to ensure consistency across different systems and applications.
Enhancing Your Integration Experience with Endgrate
For developers looking to streamline their integration processes, Endgrate offers a unified API solution. By using Endgrate, you can save time and resources by building integrations once and applying them across multiple platforms. This allows you to focus on your core product while providing an intuitive integration experience for your customers.
Explore how Endgrate can simplify your integration needs and enhance your development workflow by visiting their website and discovering the benefits of a unified API approach.
Read More
Ready to get started?