How to Create Custom Objects with the Zoho CRM API in Javascript
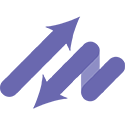
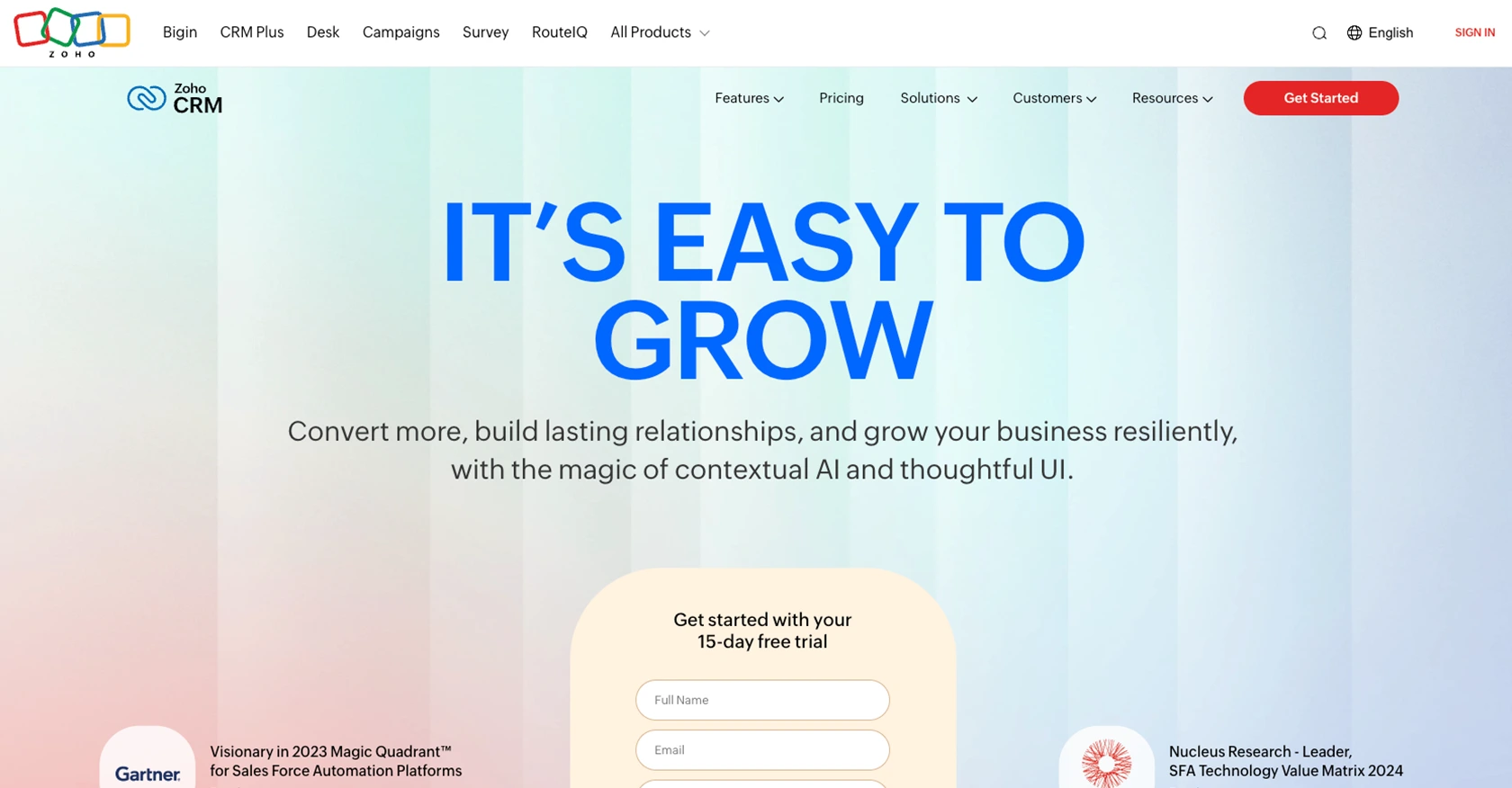
Introduction to Zoho CRM API and Custom Objects
Zoho CRM is a comprehensive customer relationship management platform that empowers businesses to manage their sales, marketing, and support in a single system. Known for its flexibility and robust features, Zoho CRM is a popular choice for organizations looking to enhance their customer interactions and streamline operations.
Developers often seek to integrate with Zoho CRM to leverage its extensive API capabilities, allowing for the creation and management of custom objects. This integration can be particularly useful for businesses that need to tailor their CRM experience to specific workflows or data structures. For example, a developer might create custom objects to track unique business metrics or integrate third-party data sources directly into Zoho CRM.
This article will guide you through the process of creating custom objects using the Zoho CRM API with JavaScript, providing step-by-step instructions and practical examples to help you get started.
Setting Up Your Zoho CRM Sandbox Account for API Integration
Before you begin creating custom objects with the Zoho CRM API in JavaScript, you'll need to set up a sandbox account. This allows you to test your integrations without affecting live data. Zoho CRM offers a developer sandbox environment that replicates your production setup, enabling safe testing and development.
Steps to Create a Zoho CRM Sandbox Account
- Sign Up for Zoho CRM: If you don't already have a Zoho CRM account, visit the Zoho CRM sign-up page and create a free account.
- Access the Developer Console: Once logged in, navigate to the Developer Console by selecting "Setup" from the top menu, then "Developer Space" under "Developer Tools."
- Create a Sandbox: In the Developer Console, select "Sandbox" and click "Create Sandbox." Follow the prompts to set up your sandbox environment.
Configuring OAuth for Zoho CRM API Access
Zoho CRM uses OAuth 2.0 for secure API authentication. Follow these steps to configure OAuth for your application:
- Register Your Application: Go to the Zoho API Console and register your application. Choose "JavaScript" as the client type.
- Fill in Application Details: Provide the necessary details such as Client Name, Homepage URL, and Authorized Redirect URIs. Use dummy values if you don't have a domain name.
- Generate Client ID and Secret: After registration, you'll receive a Client ID and Client Secret. Keep these credentials secure as they are essential for API access.
Setting Up OAuth Scopes for Zoho CRM API
Scopes define the level of access your application has to Zoho CRM data. To create custom objects, ensure you have the necessary scopes:
- Access Scopes: Use scopes like
ZohoCRM.modules.ALL
for full access orZohoCRM.modules.custom.CREATE
for creating custom objects. - Authorization URL: Construct the authorization URL with the required scopes to obtain user consent.
Testing Your Zoho CRM Sandbox Setup
Once your sandbox and OAuth settings are configured, test your setup by making a simple API call to ensure everything is working correctly. Use the following JavaScript code snippet to test the connection:
fetch('https://www.zohoapis.com/crm/v3/MODULE_HERE', {
method: 'GET',
headers: {
'Authorization': 'Zoho-oauthtoken YOUR_ACCESS_TOKEN'
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Replace YOUR_ACCESS_TOKEN
with the access token obtained through OAuth. This call should return data from your sandbox environment, confirming that your setup is complete.
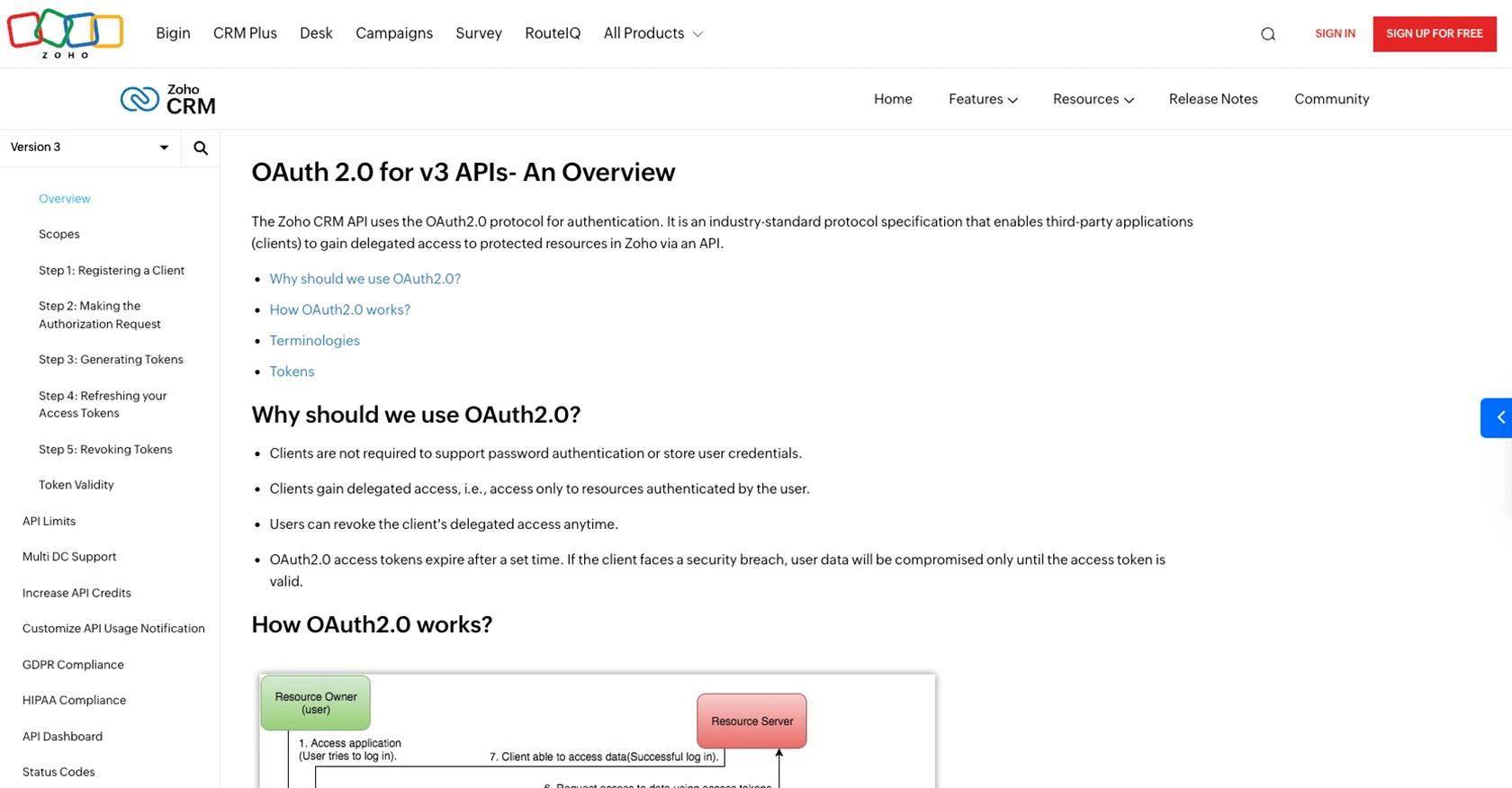
sbb-itb-96038d7
Making API Calls to Create Custom Objects in Zoho CRM Using JavaScript
To create custom objects in Zoho CRM using JavaScript, you'll need to make API calls to the Zoho CRM API. This section will guide you through the process, including setting up your JavaScript environment, writing the code to make API requests, and handling responses.
Setting Up Your JavaScript Environment for Zoho CRM API Integration
Before making API calls, ensure your development environment is ready. You'll need:
- Node.js: Install Node.js from the official website to run JavaScript outside the browser.
- Node Package Manager (npm): Use npm to manage dependencies. It comes bundled with Node.js.
- Axios Library: Install Axios, a promise-based HTTP client, to simplify API requests. Run the following command in your terminal:
npm install axios
Writing JavaScript Code to Create Custom Objects in Zoho CRM
With your environment set up, you can write JavaScript code to create custom objects in Zoho CRM. Here's a sample script:
const axios = require('axios');
// Define the API endpoint and headers
const endpoint = 'https://www.zohoapis.com/crm/v3/MODULE_HERE';
const headers = {
'Authorization': 'Zoho-oauthtoken YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json'
};
// Define the custom object data
const customObjectData = {
data: [
{
Custom_Field_1: 'Value1',
Custom_Field_2: 'Value2'
}
]
};
// Make a POST request to create the custom object
axios.post(endpoint, customObjectData, { headers })
.then(response => {
console.log('Custom Object Created:', response.data);
})
.catch(error => {
console.error('Error creating custom object:', error.response.data);
});
Replace YOUR_ACCESS_TOKEN
with your actual access token and MODULE_HERE
with the API name of your custom module. Customize Custom_Field_1
and Custom_Field_2
with your specific field names and values.
Handling API Responses and Errors in Zoho CRM Integration
After making the API call, it's crucial to handle the response and potential errors effectively:
- Success Response: If the API call is successful, you'll receive a response containing the details of the created custom object. Log this information for verification.
- Error Handling: If an error occurs, the catch block will capture it. Log the error details to understand the issue. Common errors include invalid tokens or incorrect module names.
Verifying Custom Object Creation in Zoho CRM Sandbox
To ensure that your custom object was created successfully, verify it in your Zoho CRM sandbox environment:
- Log into Zoho CRM: Access your sandbox account.
- Navigate to Custom Modules: Check the module where the custom object was supposed to be created.
- Confirm Object Details: Verify that the object appears with the correct data.
By following these steps, you can efficiently create custom objects in Zoho CRM using JavaScript, enhancing your CRM's functionality to meet specific business needs.
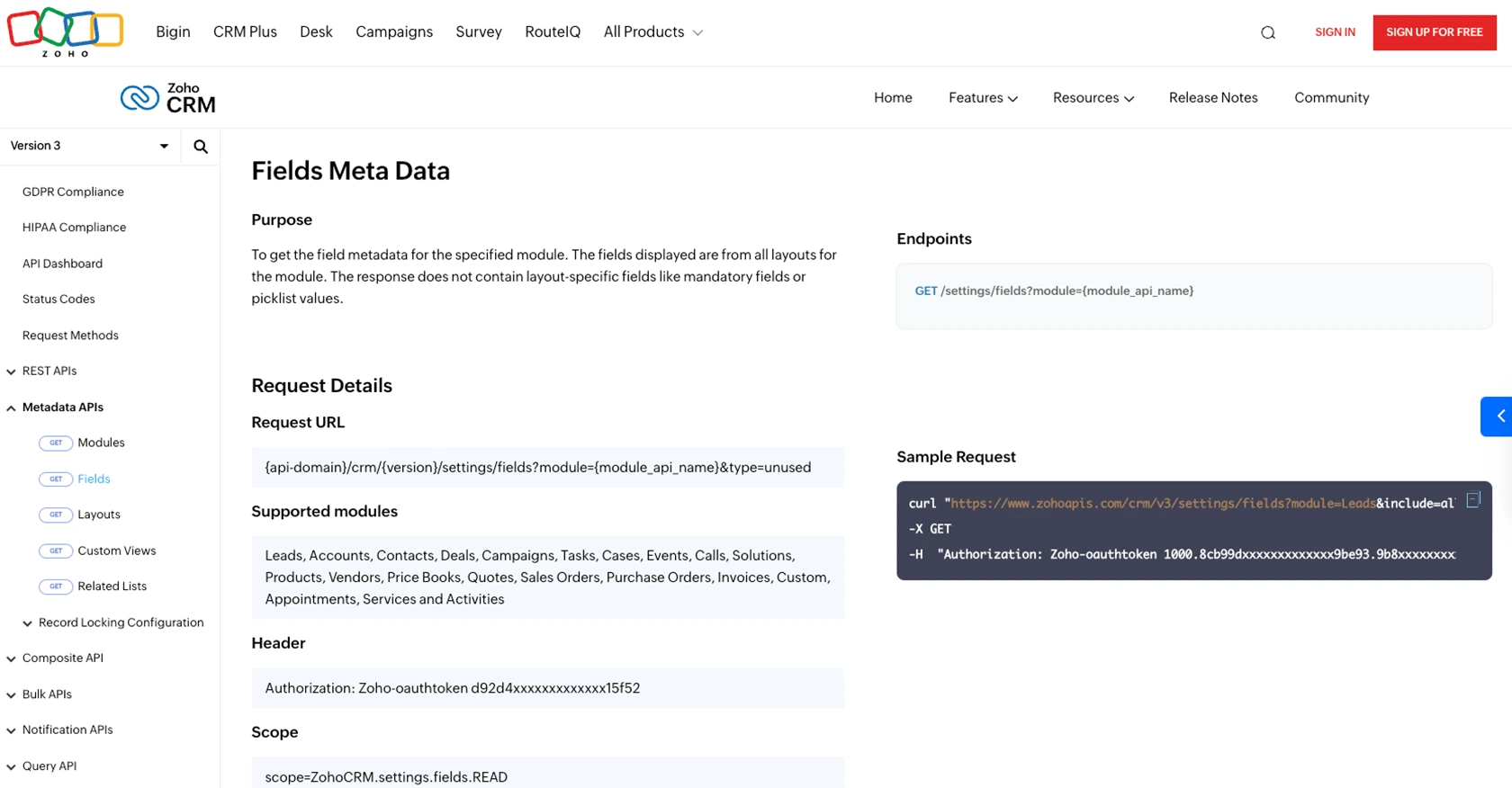
Conclusion and Best Practices for Zoho CRM API Integration
Integrating with the Zoho CRM API using JavaScript offers a powerful way to customize and enhance your CRM experience. By creating custom objects, you can tailor Zoho CRM to meet specific business requirements, ensuring that your CRM system aligns perfectly with your operational needs.
Best Practices for Secure and Efficient Zoho CRM API Integration
- Secure Storage of Credentials: Always store your OAuth credentials, such as Client ID and Client Secret, securely. Avoid hardcoding them in your source code.
- Handle Rate Limiting: Be aware of Zoho CRM's API rate limits to prevent disruptions. Implement retry logic and exponential backoff strategies to handle rate limit errors gracefully. For more details, refer to the API limits documentation.
- Data Standardization: Ensure consistent data formats when creating custom objects. This helps maintain data integrity and simplifies data processing.
Enhancing Your Integration Strategy with Endgrate
For developers looking to streamline their integration processes, Endgrate offers a unified API solution that simplifies connecting with multiple platforms, including Zoho CRM. By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development while ensuring a seamless integration experience for your users.
Explore how Endgrate can transform your integration strategy by visiting Endgrate and discover the benefits of a unified API approach.
By following these best practices and utilizing tools like Endgrate, you can maximize the potential of your Zoho CRM integration, driving efficiency and innovation in your business processes.
Read More
- https://endgrate.com/provider/zohocrm
- https://www.zoho.com/crm/developer/docs/api/v3/oauth-overview.html
- https://www.zoho.com/crm/developer/docs/api/v3/scopes.html
- https://www.zoho.com/crm/developer/docs/api/v3/register-client.html
- https://www.zoho.com/crm/developer/docs/api/v3/api-limits.html
- https://www.zoho.com/crm/developer/docs/api/v3/status-codes.html
- https://www.zoho.com/crm/developer/docs/api/v3/module-meta.html
- https://www.zoho.com/crm/developer/docs/api/v3/field-meta.html
- https://www.zoho.com/crm/developer/docs/api/v3/search-records.html
- https://www.zoho.com/crm/developer/docs/api/v3/insert-records.html
- https://www.zoho.com/crm/developer/docs/api/v3/update-records.html
Ready to get started?