Using the Sap Business One API to Get Orders in Python
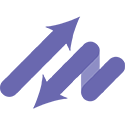
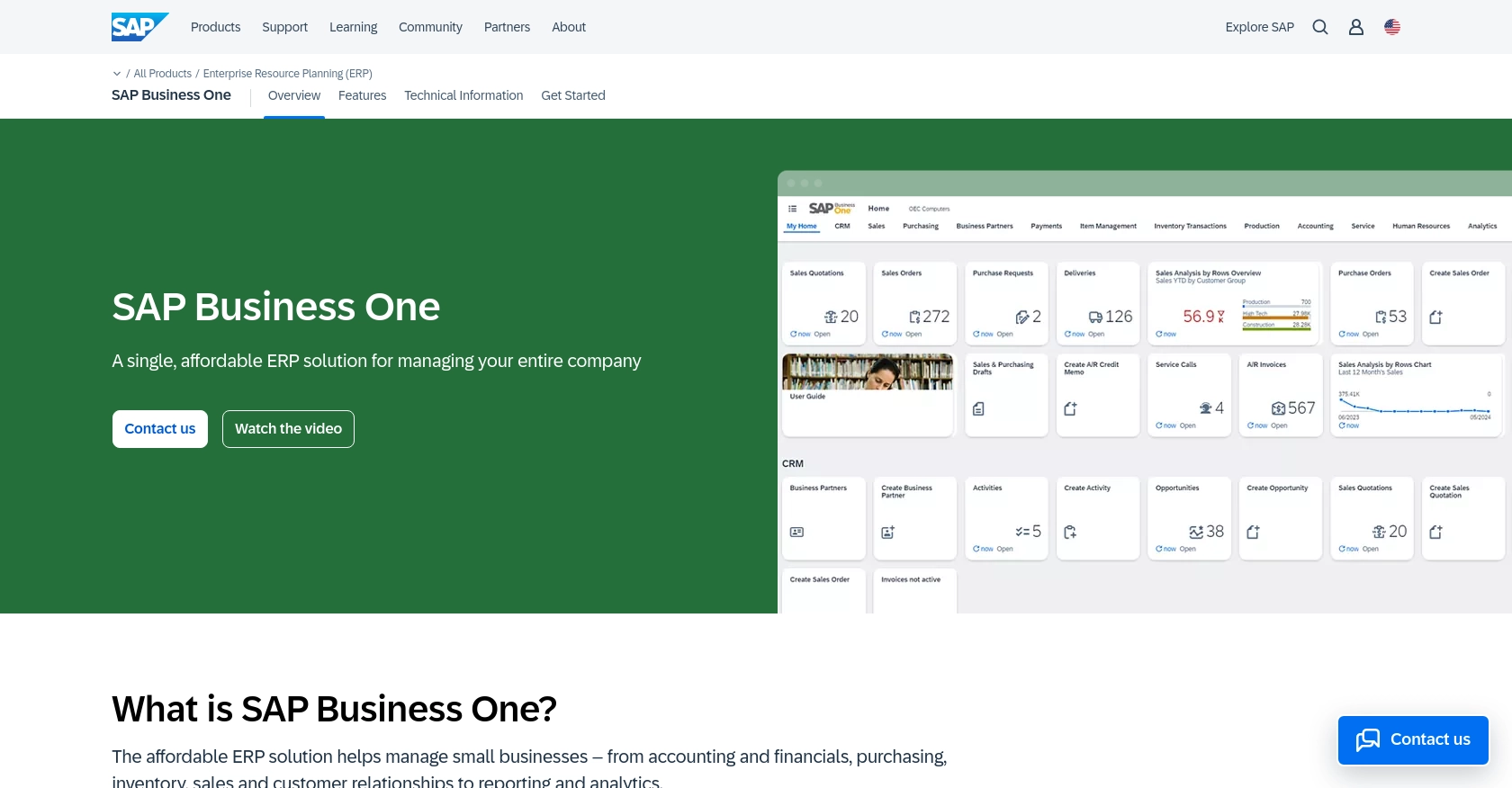
Introduction to SAP Business One
SAP Business One is a comprehensive enterprise resource planning (ERP) solution tailored for small and medium-sized businesses. It offers a wide range of functionalities, including financial management, sales, customer relationship management, and supply chain operations, all integrated into a single platform.
Developers often seek to integrate with SAP Business One's API to streamline business processes and enhance operational efficiency. For example, retrieving order data using the SAP Business One API in Python can help automate inventory management, enabling businesses to respond swiftly to customer demands and optimize their supply chain.
Setting Up a Test/Sandbox Account for SAP Business One API
Before you can start interacting with the SAP Business One API, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data. Follow these steps to get started:
Create a SAP Business One Sandbox Account
To access the SAP Business One API, you first need to create a sandbox account. This can typically be done through SAP's official website or by contacting their support team for access to a developer environment.
- Visit the SAP Business One website and navigate to the developer section.
- Sign up for a developer account if you haven't already. This may involve providing some basic information about your business and development needs.
- Once your account is set up, log in to access the sandbox environment.
Generate API Credentials for SAP Business One
With your sandbox account ready, the next step is to generate the necessary API credentials. SAP Business One uses a custom authentication method, so you'll need to follow these steps:
- Log in to your SAP Business One sandbox account.
- Navigate to the API management section, where you can create a new application.
- Fill in the required details for your application, such as the application name and description.
- Once the application is created, you will receive a client ID and client secret. Keep these credentials secure, as they are essential for authenticating your API requests.
Configure OAuth for SAP Business One API Access
Although SAP Business One uses custom authentication, you may need to configure OAuth settings for certain integrations:
- In the API management section, locate the OAuth settings for your application.
- Set up the necessary redirect URIs and scopes required for your integration.
- Save the configuration to ensure your application can authenticate API requests properly.
With your sandbox account and API credentials ready, you can now proceed to make API calls to SAP Business One using Python. This setup ensures you have a secure and isolated environment to test your integrations.
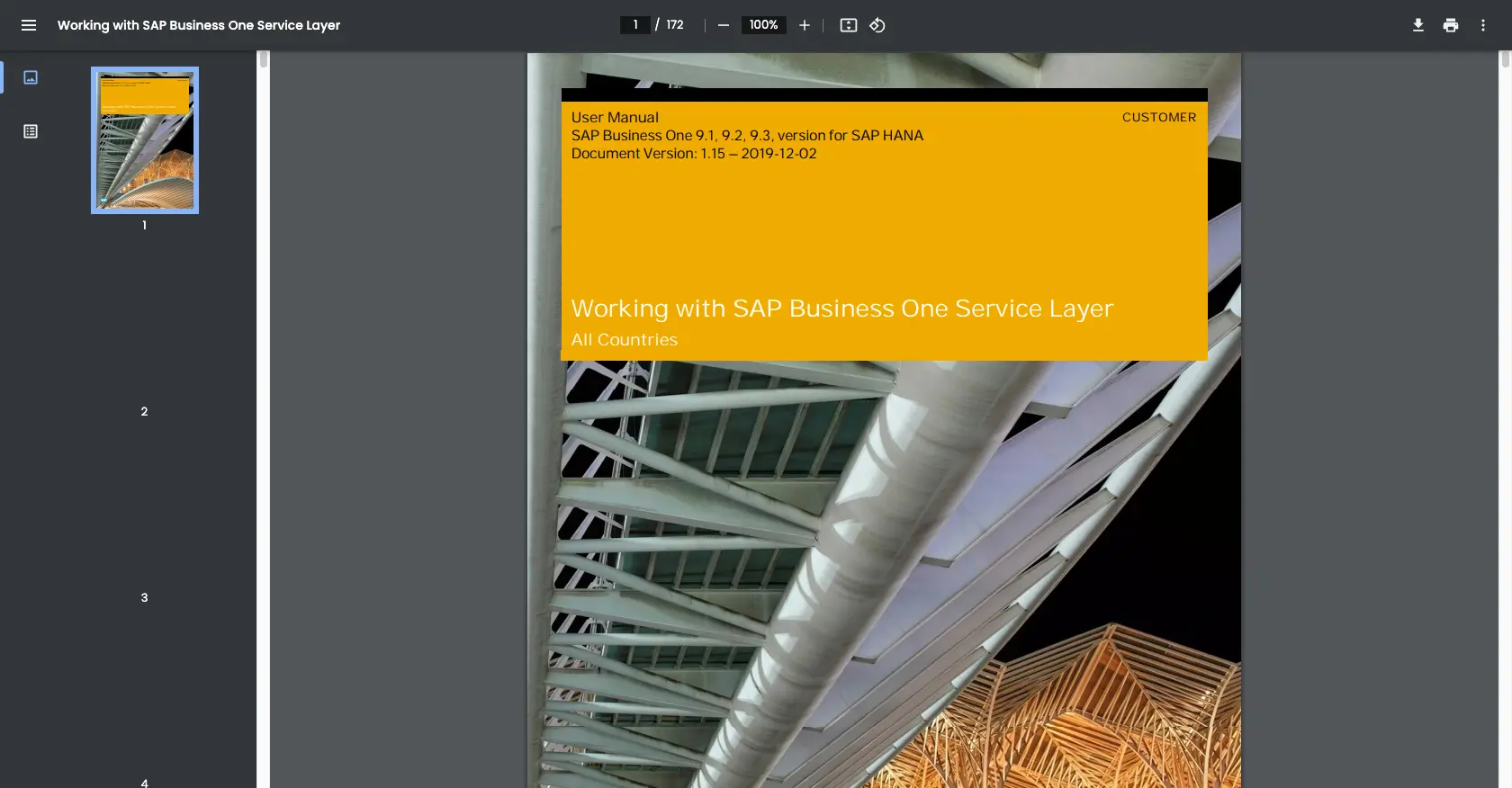
sbb-itb-96038d7
Making API Calls to Retrieve Orders from SAP Business One Using Python
To effectively interact with the SAP Business One API and retrieve order data, you'll need to set up your Python environment and write the necessary code. This section will guide you through the process, ensuring you have all the tools and knowledge required to make successful API calls.
Setting Up Your Python Environment
Before diving into the code, ensure that your Python environment is correctly configured. You'll need Python 3.x and the requests
library to make HTTP requests to the SAP Business One API.
- Ensure Python 3.x is installed on your machine. You can download it from the official Python website.
- Install the
requests
library using pip:
pip install requests
Writing Python Code to Retrieve Orders from SAP Business One
With your environment set up, you can now write the Python code to retrieve orders from SAP Business One. The following example demonstrates how to make a GET request to the API to fetch order data.
import requests
# Define the API endpoint and headers
endpoint = "https://your-sap-business-one-instance.com/b1s/v1/Orders"
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Access_Token"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
orders = response.json()
for order in orders['value']:
print(f"Order ID: {order['DocEntry']}, Customer: {order['CardName']}")
else:
print(f"Failed to retrieve orders. Status code: {response.status_code}")
Replace Your_Access_Token
with the token you obtained during the authentication setup. This code snippet sends a GET request to the SAP Business One API endpoint for orders and prints out the order ID and customer name for each order.
Verifying API Call Success and Handling Errors
After executing the code, verify the success of your API call by checking the response status code. A status code of 200 indicates a successful request. If the request fails, the code will print an error message with the status code.
To handle potential errors, consider implementing additional error handling logic. For example, you can check for specific status codes and handle them accordingly, such as retrying the request or logging the error for further investigation.
Testing in the SAP Business One Sandbox Environment
Once you've successfully retrieved order data, you can verify the results in your SAP Business One sandbox environment. Ensure that the data returned by the API matches the orders present in your sandbox, confirming that your integration is working as expected.
By following these steps, you can efficiently interact with the SAP Business One API using Python, enabling you to automate order retrieval and enhance your business processes.
Conclusion and Best Practices for Integrating with SAP Business One API
Integrating with the SAP Business One API using Python provides a powerful way to automate and streamline business processes. By retrieving order data programmatically, businesses can enhance their operational efficiency and respond more swiftly to customer demands.
Best Practices for Secure and Efficient SAP Business One API Integration
- Secure Storage of Credentials: Always store your API credentials, such as client ID and client secret, securely. Consider using environment variables or a secure vault to protect sensitive information.
- Handling Rate Limits: Be mindful of any rate limits imposed by the SAP Business One API. Implement logic to handle rate limiting gracefully, such as retrying requests after a delay.
- Error Handling: Implement robust error handling to manage different status codes and potential API errors. This ensures that your application can recover gracefully from unexpected issues.
- Data Transformation: Standardize and transform data fields as needed to ensure compatibility with your existing systems and processes.
Streamlining Integrations with Endgrate
While integrating with SAP Business One can be highly beneficial, managing multiple integrations can become complex and time-consuming. Endgrate offers a solution by providing a unified API endpoint that connects to various platforms, including SAP Business One.
By leveraging Endgrate, developers can save time and resources by building once for each use case instead of multiple times for different integrations. This allows you to focus on your core product while providing an intuitive integration experience for your customers.
Explore how Endgrate can simplify your integration processes by visiting Endgrate's website and discover how you can enhance your business operations with seamless integrations.
Read More
Ready to get started?