How to Get Devices with the ButterflyMX API in PHP
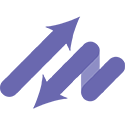
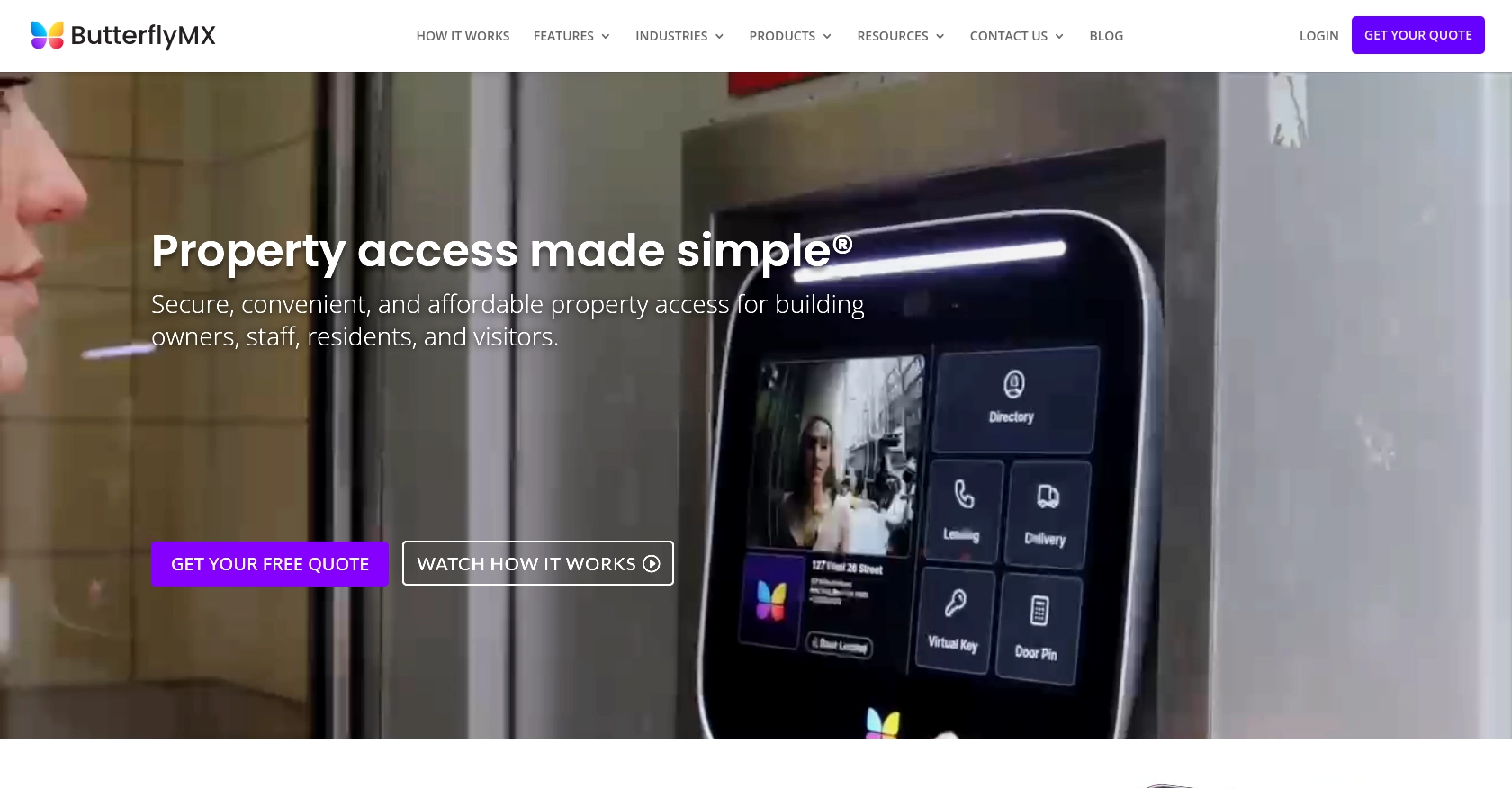
Introduction to ButterflyMX API Integration
ButterflyMX is a cutting-edge property access solution that simplifies building entry for residents, visitors, and property staff. With its innovative intercom system, ButterflyMX enhances security and convenience by allowing users to manage access through their smartphones.
Developers may want to integrate with the ButterflyMX API to streamline access management and automate device interactions within buildings. For example, using the ButterflyMX API, a developer can retrieve a list of devices in a building, enabling seamless integration with other property management systems.
Setting Up a ButterflyMX Test/Sandbox Account for API Integration
To begin integrating with the ButterflyMX API, you'll need to set up a test or sandbox account. This environment allows developers to safely experiment with API calls without affecting live data. Unfortunately, ButterflyMX does not offer a self-service option for creating sandbox accounts directly on their website. Instead, you must contact the company directly to request access to a sandbox account.
Contacting ButterflyMX for Sandbox Account Access
Follow these steps to request a sandbox account:
- Visit the ButterflyMX API documentation page to find contact information.
- Reach out to ButterflyMX support or sales team via email or phone, explaining your need for a sandbox account for development purposes.
- Once your request is approved, ButterflyMX will provide you with the necessary credentials to access the sandbox environment.
Creating a ButterflyMX App for OAuth2 Authentication
ButterflyMX uses OAuth2 for authentication, which requires you to create an app to obtain the necessary credentials. Follow these steps once you have sandbox access:
- Log in to your ButterflyMX sandbox account.
- Navigate to the app creation section within the developer portal.
- Create a new app by providing the required details such as app name and description.
- Note down the Client ID and Client Secret provided upon app creation. These will be used for OAuth2 authentication.
Obtaining OAuth2 Tokens for API Access
With your app created, you can now obtain the OAuth2 tokens needed for API access:
curl --location --request POST 'https://accountssandbox.butterflymx.com/oauth/token' \
--form 'grant_type=authorization_code' \
--form 'code=$code' \
--form 'client_id=$CLIENT_ID' \
--form 'client_secret=$CLIENT_SECRET' \
--form 'redirect_uri=https://usersandbox.butterflymx.com/oauth/callbacks/butterflymx'
Replace $code
, $CLIENT_ID
, and $CLIENT_SECRET
with the appropriate values obtained during the app creation process. Upon successful request, you will receive an access_token
and a refresh_token
to authenticate your API calls.
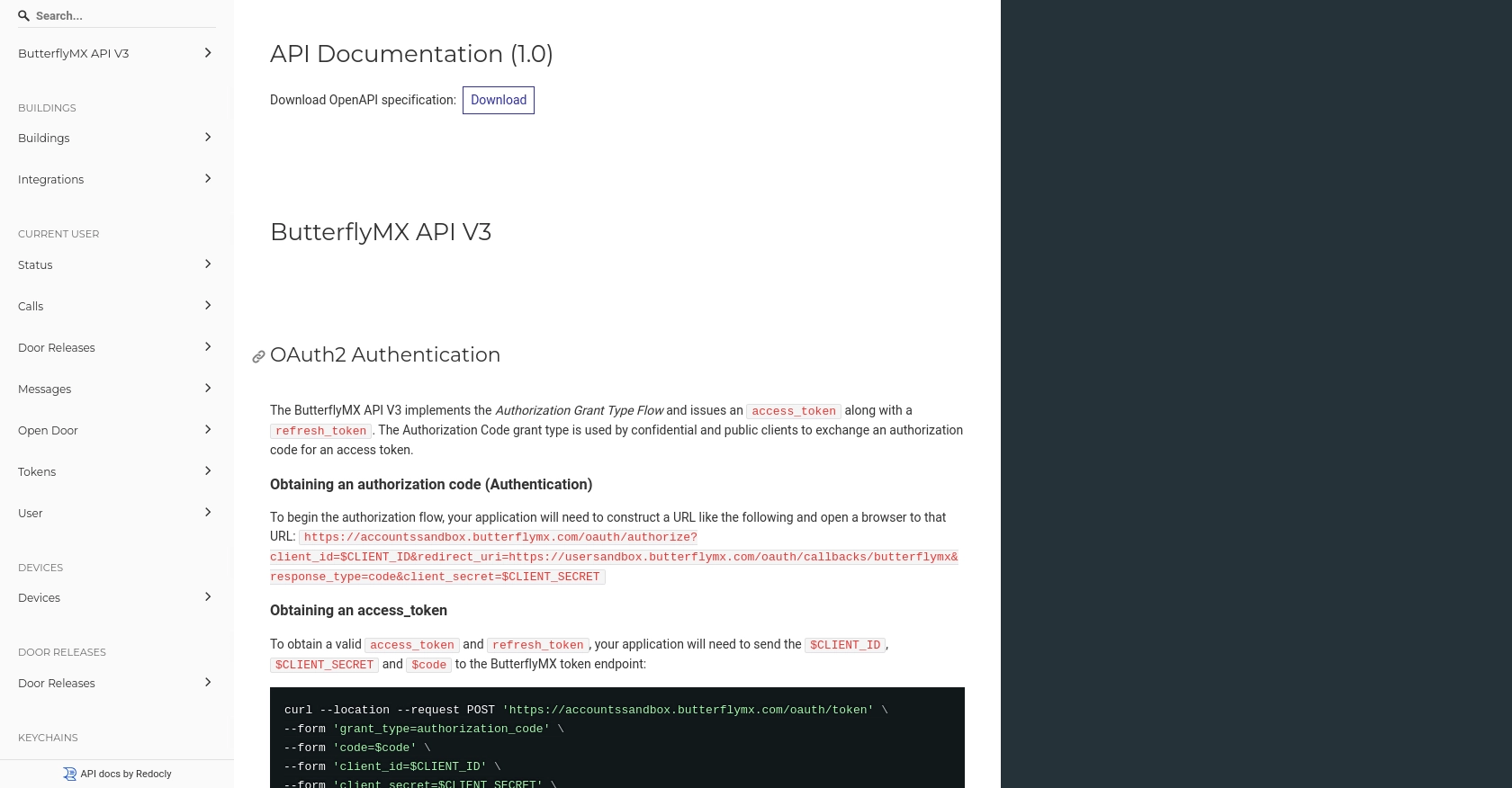
sbb-itb-96038d7
Making API Calls to Retrieve Devices with ButterflyMX API in PHP
To interact with the ButterflyMX API and retrieve a list of devices, you'll need to use PHP. This section will guide you through setting up your PHP environment, making the API call, and handling the response effectively.
Setting Up Your PHP Environment for ButterflyMX API Integration
Before making API calls, ensure your PHP environment is properly configured. You'll need:
- PHP 7.4 or higher
- Composer for dependency management
Install the necessary dependencies using Composer:
composer require guzzlehttp/guzzle
This command installs Guzzle, a PHP HTTP client that simplifies making HTTP requests.
Writing PHP Code to Call ButterflyMX API and Retrieve Devices
With your environment set up, create a PHP script to make the API call:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token'; // Replace with your access token
try {
$response = $client->request('GET', 'https://apisandbox.butterflymx.com/v3/devices', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Accept' => 'application/vnd.api+json'
]
]);
$data = json_decode($response->getBody(), true);
foreach ($data['data'] as $device) {
echo 'Device ID: ' . $device['id'] . '<br>';
echo 'Device Type: ' . $device['type'] . '<br>';
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
?>
Replace Your_Access_Token
with the access token obtained during the OAuth2 authentication process. This script uses Guzzle to send a GET request to the ButterflyMX API endpoint for devices. It then parses and displays the device information.
Verifying API Call Success and Handling Errors
After running the script, verify the success of your API call by checking the output. The device information should match the data in your ButterflyMX sandbox account.
Handle potential errors by catching exceptions and displaying error messages. Common errors include:
- 401 Unauthorized: Check your access token and ensure it's valid.
- 404 Not Found: Verify the API endpoint URL.
For more detailed error handling, refer to the ButterflyMX API documentation.
Conclusion and Best Practices for ButterflyMX API Integration in PHP
Integrating with the ButterflyMX API using PHP can significantly enhance property management systems by automating access control and device management. By following the steps outlined in this guide, developers can efficiently retrieve device information and integrate it with other systems.
Best Practices for Secure and Efficient API Integration with ButterflyMX
- Secure Storage of Credentials: Always store your
Client ID
,Client Secret
, andaccess_token
securely. Consider using environment variables or a secure vault to prevent unauthorized access. - Handle Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that data retrieved from the API is transformed and standardized to fit your application's data models, facilitating seamless integration.
- Error Handling: Implement comprehensive error handling to manage different HTTP status codes and provide meaningful feedback to users or logs for debugging purposes.
Enhance Your Integration Strategy with Endgrate
While integrating with ButterflyMX is a powerful way to streamline property management, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies integration management across various platforms, including ButterflyMX.
By leveraging Endgrate, developers can:
- Save time and resources by outsourcing integration management and focusing on core product development.
- Build once for each use case and apply it across multiple integrations, reducing redundancy.
- Provide an intuitive integration experience for customers, enhancing user satisfaction and engagement.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?