Using the Pipedrive API to Create or Update People in PHP
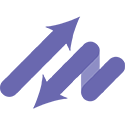
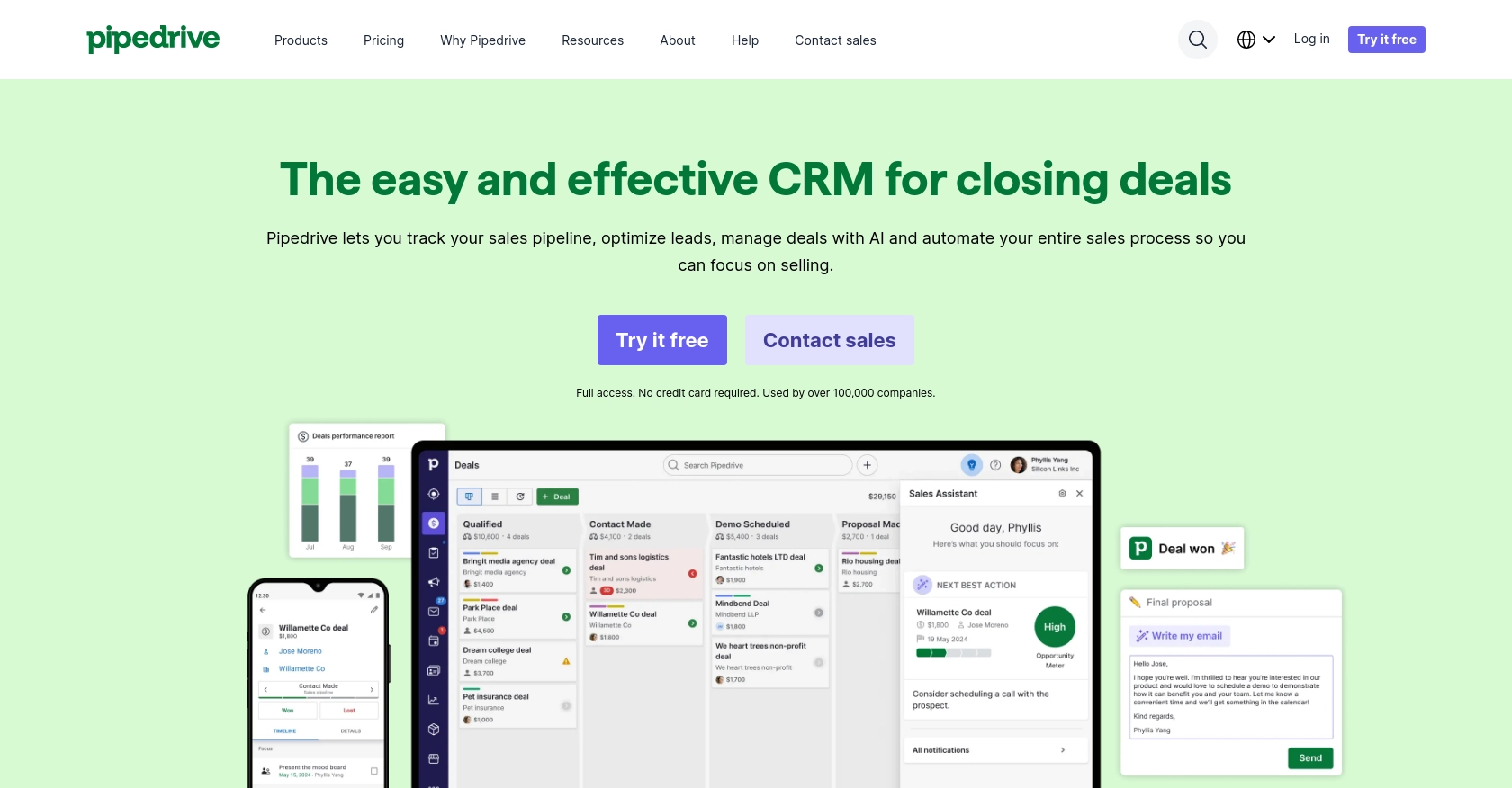
Introduction to Pipedrive CRM
Pipedrive is a powerful sales CRM platform designed to help businesses manage their sales processes more effectively. With its intuitive interface and robust features, Pipedrive enables sales teams to track leads, manage deals, and automate repetitive tasks, ensuring a streamlined workflow.
For developers, integrating with Pipedrive's API offers the opportunity to enhance CRM functionalities by automating tasks such as creating or updating contact information. For example, you might want to automatically update customer details from an external database into Pipedrive, ensuring that your sales team always has access to the most current information.
This article will guide you through using PHP to interact with the Pipedrive API, specifically focusing on creating or updating people records. By following this tutorial, you can efficiently manage your contacts within Pipedrive, leveraging its API capabilities to enhance your CRM operations.
Setting Up Your Pipedrive Developer Sandbox Account
Before you can start integrating with the Pipedrive API, you need to set up a developer sandbox account. This account provides a risk-free environment where you can test and develop your applications without affecting live data.
Creating a Pipedrive Developer Sandbox Account
To create a sandbox account, follow these steps:
- Visit the Pipedrive Developer Sandbox page.
- Fill out the form to request access to a sandbox account. This account is similar to a regular Pipedrive account but is limited to five seats for testing purposes.
- Once your account is set up, you can import sample data to familiarize yourself with Pipedrive's features. Navigate to “...” (More) > Import data > From a spreadsheet in the Pipedrive web app to import template spreadsheets.
Creating a Pipedrive App for OAuth Authentication
Pipedrive uses OAuth 2.0 for authentication, which requires you to create an app to obtain the necessary credentials. Follow these steps to create your app:
- Log in to your Pipedrive sandbox account and navigate to the Developer Hub.
- Click on "Create an app" and fill in the required details, such as the app name and description.
- Once the app is created, you will receive a client ID and client secret. These credentials are essential for the OAuth flow.
- Define the necessary scopes and permissions for your app to ensure it can access the required data.
With your sandbox account and app set up, you are ready to start integrating with the Pipedrive API using PHP. In the next section, we will cover how to make API calls to create or update people records.
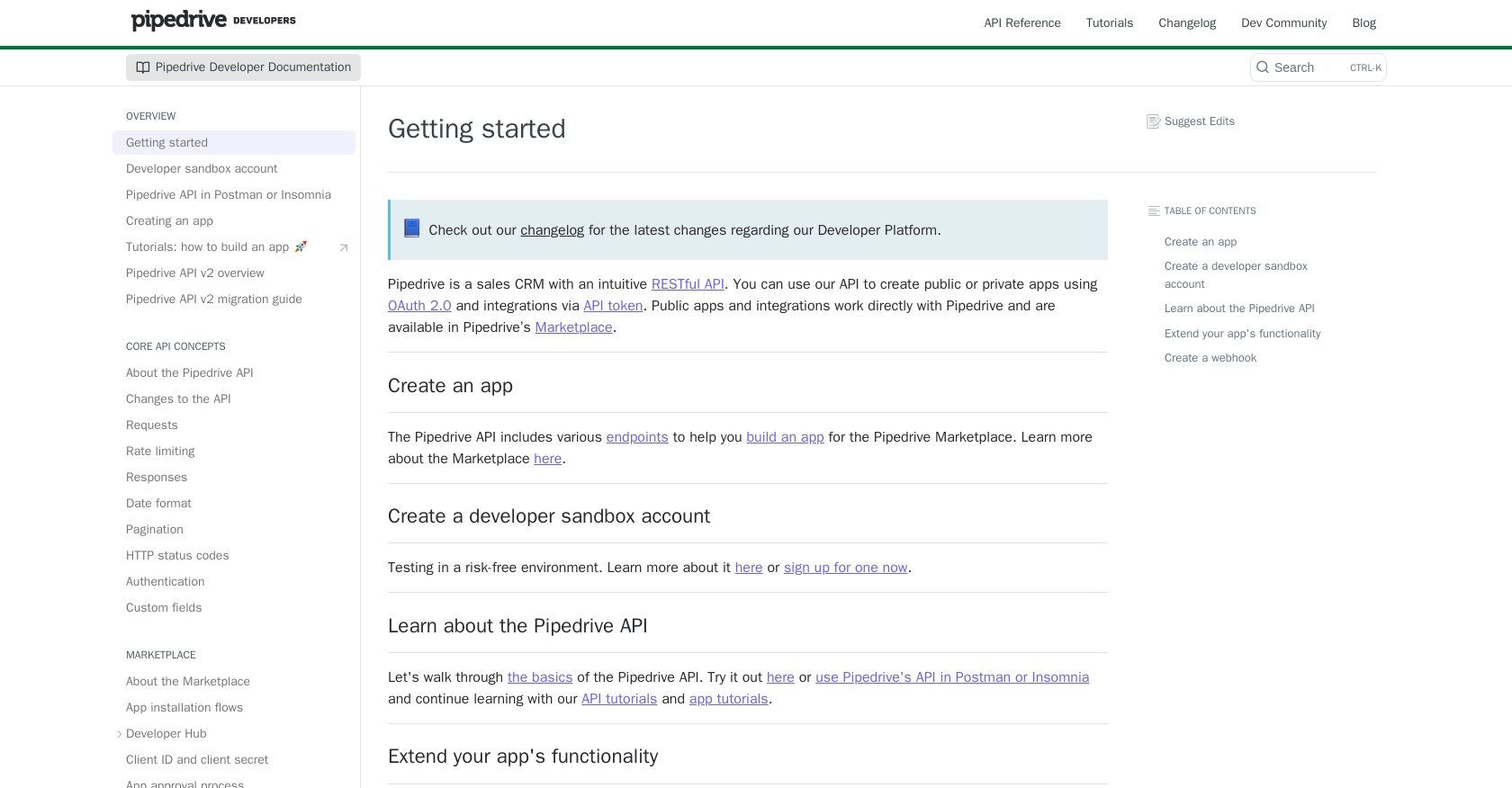
sbb-itb-96038d7
Making API Calls to Create or Update People in Pipedrive Using PHP
To interact with the Pipedrive API using PHP, you'll need to set up your environment and write code to handle the API requests. This section will guide you through the process of creating or updating people records in Pipedrive using PHP.
Setting Up Your PHP Environment for Pipedrive API Integration
Before making API calls, ensure that your PHP environment is properly configured. You'll need:
- PHP 7.4 or higher
- cURL extension enabled
- Composer for managing dependencies
Install the necessary dependencies using Composer:
composer require guzzlehttp/guzzle
Creating a New Person in Pipedrive Using PHP
To create a new person in Pipedrive, you'll use the POST /v1/persons
endpoint. Here's a sample PHP script to add a person:
use GuzzleHttp\Client;
$client = new Client();
$response = $client->post('https://api.pipedrive.com/v1/persons', [
'headers' => [
'Authorization' => 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type' => 'application/json'
],
'json' => [
'name' => 'John Doe',
'email' => [['value' => 'john.doe@example.com', 'primary' => true]],
'phone' => [['value' => '1234567890', 'primary' => true]]
]
]);
$data = json_decode($response->getBody(), true);
echo 'Person created with ID: ' . $data['data']['id'];
Replace YOUR_ACCESS_TOKEN
with your actual OAuth access token. This script sends a POST request to create a new person with the specified name, email, and phone number.
Updating an Existing Person in Pipedrive Using PHP
To update an existing person, use the PUT /v1/persons/{id}
endpoint. Here's how to update a person's details:
$personId = 123; // Replace with the actual person ID
$response = $client->put("https://api.pipedrive.com/v1/persons/{$personId}", [
'headers' => [
'Authorization' => 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type' => 'application/json'
],
'json' => [
'name' => 'John Doe Updated',
'email' => [['value' => 'john.updated@example.com', 'primary' => true]]
]
]);
$data = json_decode($response->getBody(), true);
echo 'Person updated with ID: ' . $data['data']['id'];
This script updates the specified person's name and email. Ensure you replace $personId
with the correct ID of the person you wish to update.
Handling API Responses and Errors
After making API calls, it's crucial to handle responses and potential errors. Check the response status code to verify success:
if ($response->getStatusCode() === 200) {
echo 'Request successful!';
} else {
echo 'Error: ' . $response->getStatusCode();
}
Refer to the Pipedrive API documentation for detailed information on error codes and handling strategies: Pipedrive HTTP Status Codes.
Verifying Changes in Your Pipedrive Sandbox
To ensure your API calls are successful, verify the changes in your Pipedrive sandbox account. Check the list of people to confirm that new records are added or existing records are updated as expected.
By following these steps, you can efficiently manage people records in Pipedrive using PHP, enhancing your CRM capabilities through automation.
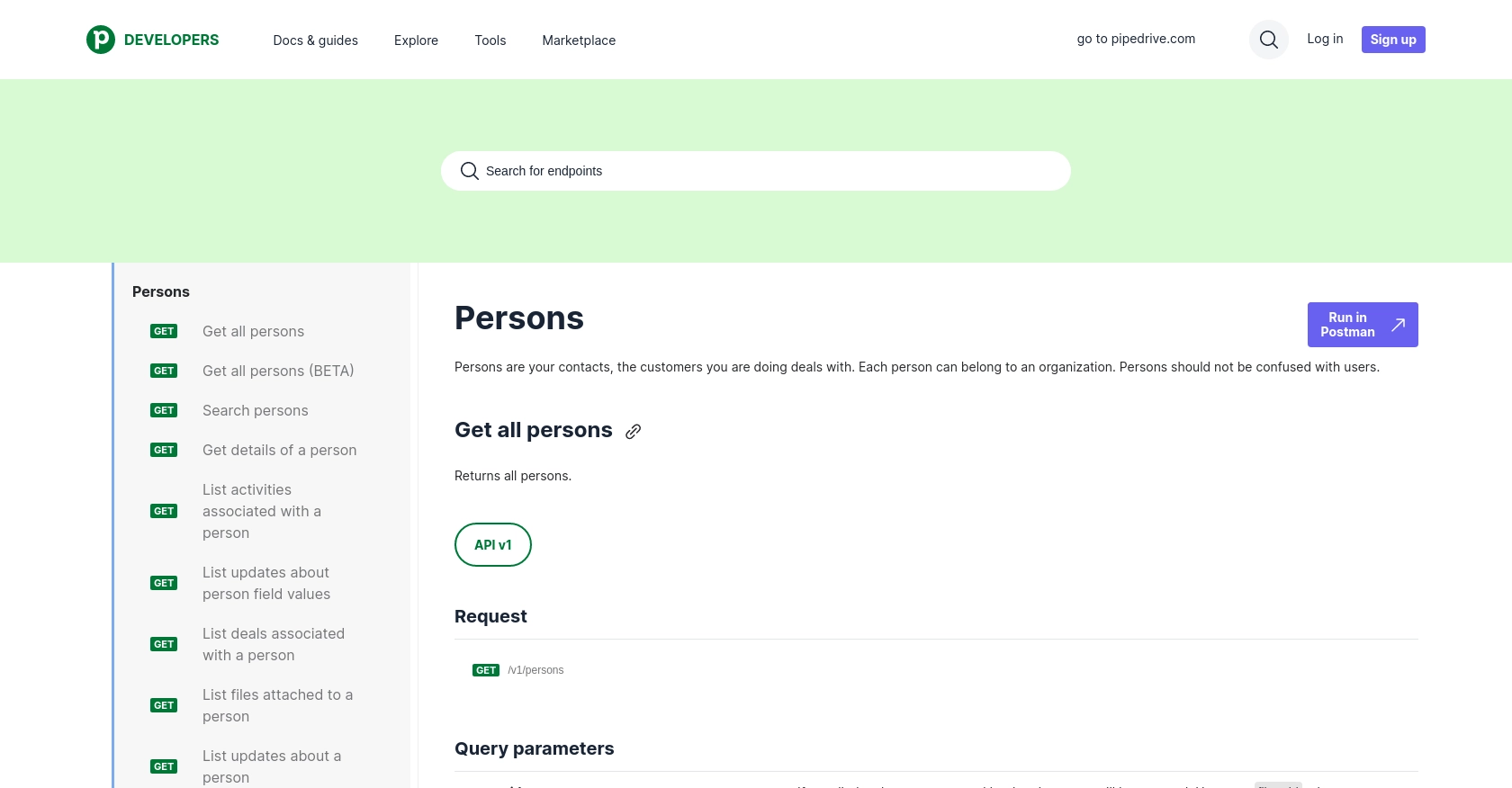
Conclusion and Best Practices for Pipedrive API Integration Using PHP
Integrating with the Pipedrive API using PHP allows developers to automate and enhance CRM functionalities, ensuring that sales teams have access to up-to-date contact information. By following the steps outlined in this guide, you can efficiently create or update people records in Pipedrive, streamlining your CRM operations.
Best Practices for Storing User Credentials Securely
When handling OAuth credentials, ensure they are stored securely. Use environment variables or secure vaults to keep your client ID
, client secret
, and access tokens safe from unauthorized access.
Handling Pipedrive API Rate Limiting
Be mindful of Pipedrive's rate limits to avoid disruptions. For OAuth apps, the rate limit is 80 requests per 2 seconds per access token. Implement error handling to manage HTTP 429 "Too Many Requests" responses and consider using webhooks to reduce API calls. For more details, refer to the Pipedrive Rate Limiting Documentation.
Data Transformation and Standardization
Ensure that data fields are standardized before sending them to Pipedrive. This includes formatting phone numbers, emails, and other fields consistently to avoid errors and maintain data integrity.
Call to Action: Simplify Integrations with Endgrate
While integrating with Pipedrive's API can enhance your CRM capabilities, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies integration processes, allowing you to focus on your core product. Save time and resources by outsourcing integrations to Endgrate, ensuring a seamless experience for your customers. Visit Endgrate to learn more about how we can help streamline your integration needs.
Read More
- https://endgrate.com/provider/pipedrive
- https://pipedrive.readme.io/docs/getting-started
- https://pipedrive.readme.io/docs/developer-sandbox-account
- https://pipedrive.readme.io/docs/marketplace-creating-a-proper-app
- https://pipedrive.readme.io/docs/core-api-concepts-rate-limiting
- https://pipedrive.readme.io/docs/core-api-concepts-pagination
- https://pipedrive.readme.io/docs/core-api-concepts-http-status-codes
- https://pipedrive.readme.io/docs/core-api-concepts-custom-fields
- https://developers.pipedrive.com/docs/api/v1/Persons
- https://developers.pipedrive.com/docs/api/v1/PersonFields
Ready to get started?