How to Get Leads with the Copper API in PHP
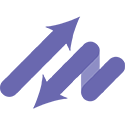
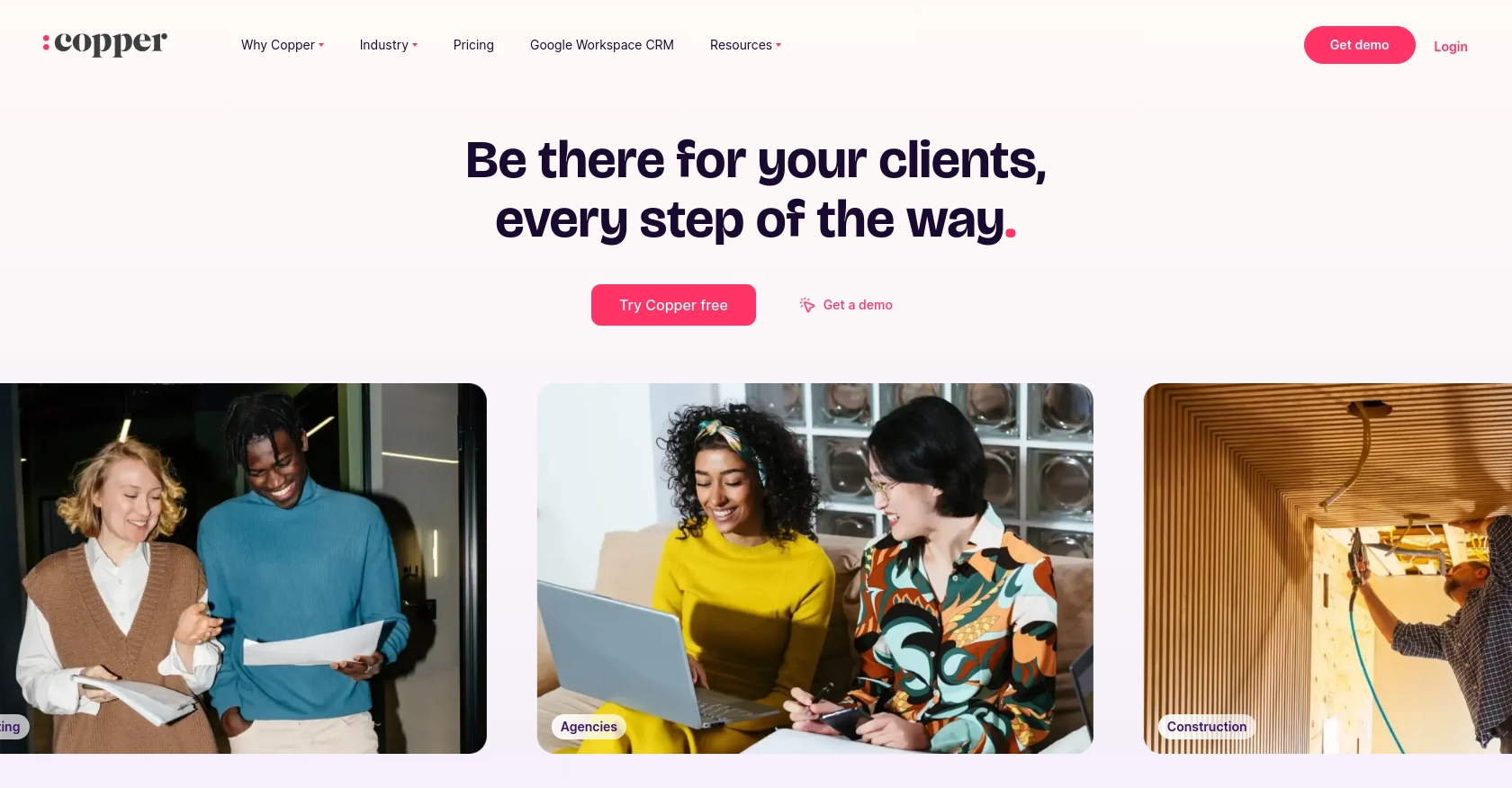
Introduction to Copper CRM
Copper CRM is a robust customer relationship management platform designed to seamlessly integrate with Google Workspace. It offers a user-friendly interface and powerful features to help businesses manage leads, track sales, and enhance customer interactions.
Developers may want to integrate with Copper's API to automate lead management processes, such as retrieving and organizing potential customer data. For example, using the Copper API in PHP, developers can efficiently gather leads from various sources and streamline them into a centralized system for better sales tracking and follow-up.
Setting Up Your Copper API Test Account
Before you can start integrating with the Copper API, you'll need to set up a test account. This will allow you to experiment with API calls without affecting your live data. Follow these steps to create a sandbox environment for your Copper API integration.
Step 1: Sign Up for a Copper Account
If you don't already have a Copper account, visit the Copper website and sign up for a free trial. This will give you access to the necessary tools and features to begin testing the API.
Step 2: Access the Copper Developer Portal
Once your account is set up, navigate to the Copper Developer Portal. Here, you can find documentation, API keys, and other resources needed for integration.
Step 3: Generate Your API Key
To authenticate your API requests, you'll need an API key. Follow these steps to generate one:
- Log in to your Copper account.
- Go to the 'Settings' section.
- Navigate to 'Integrations' and select 'API Keys'.
- Click 'Create API Key' and provide a name for your key.
- Copy the generated API key and keep it secure, as you'll need it for authentication.
Step 4: Set Up OAuth Authentication
Copper uses OAuth 2.0 for secure authentication. To set up OAuth, you'll need to create an application within your Copper account:
- In the Developer Portal, go to 'Applications'.
- Click 'Create Application' and fill in the required details, such as the application name and redirect URI.
- Once created, note the client ID and client secret, which are essential for OAuth authentication.
Step 5: Configure Your PHP Environment
Ensure your PHP environment is ready for API integration:
- Install PHP version 7.4 or higher.
- Use Composer to install necessary dependencies, such as Guzzle for HTTP requests:
composer require guzzlehttp/guzzle
With your test account and environment set up, you're ready to start making API calls to Copper and begin integrating lead management features into your application.
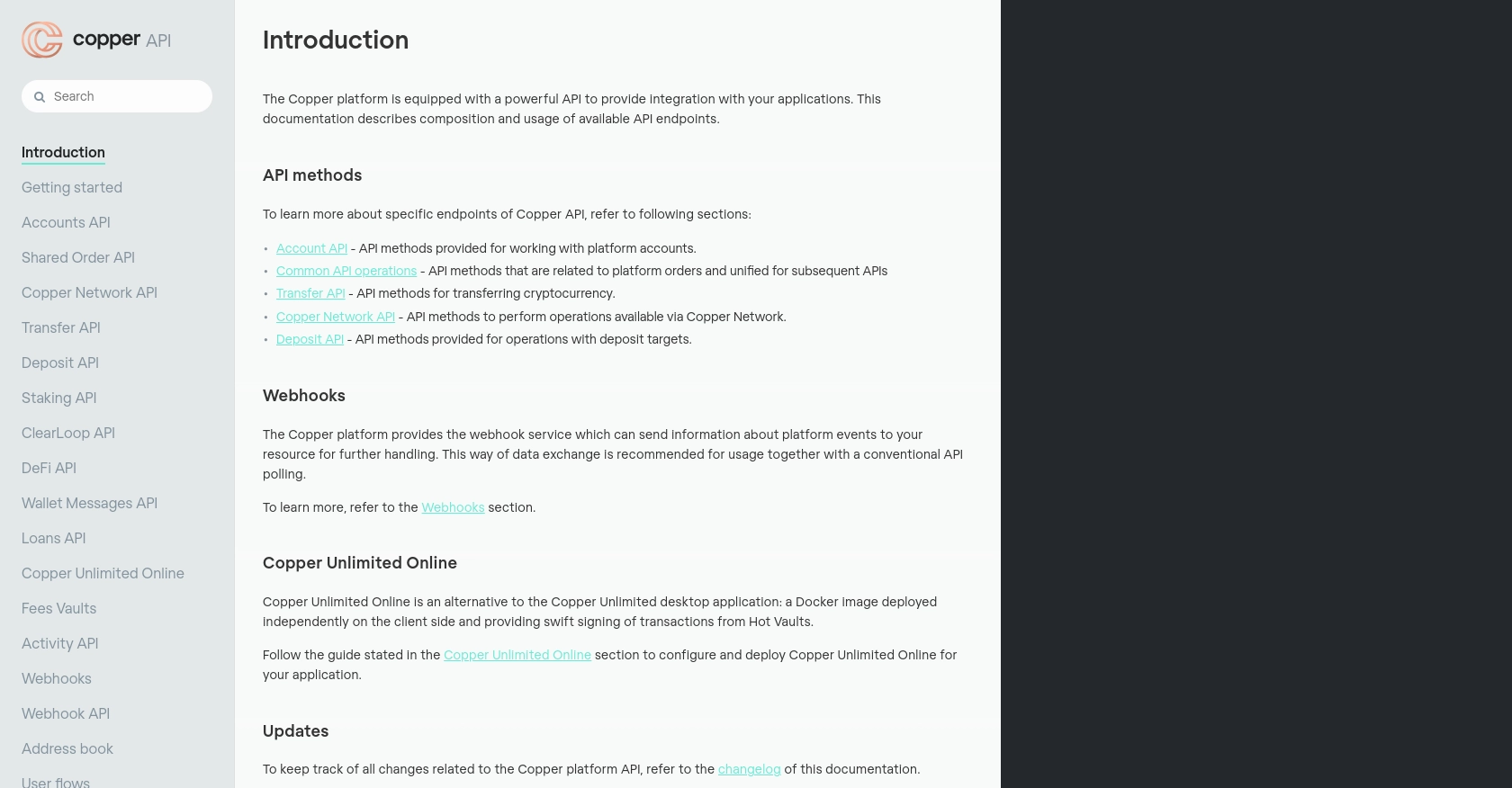
sbb-itb-96038d7
Making API Calls to Copper Using PHP
To interact with the Copper API using PHP, you'll need to ensure your environment is properly configured and understand how to make HTTP requests. This section will guide you through the process of making API calls to retrieve leads from Copper.
Setting Up Your PHP Environment for Copper API Integration
Before making API calls, ensure your PHP environment is ready:
- Ensure PHP version 7.4 or higher is installed.
- Install Composer, a dependency manager for PHP, if you haven't already.
- Use Composer to install Guzzle, a PHP HTTP client:
composer require guzzlehttp/guzzle
Creating a PHP Script to Retrieve Leads from Copper
With your environment set up, you can now create a PHP script to interact with the Copper API. Follow these steps to retrieve leads:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
// Copper API credentials
$apiKey = 'YOUR_API_KEY';
$clientId = 'YOUR_CLIENT_ID';
$clientSecret = 'YOUR_CLIENT_SECRET';
// Create a new Guzzle client
$client = new Client([
'base_uri' => 'https://api.copper.com',
'headers' => [
'Authorization' => 'Bearer ' . $apiKey,
'Content-Type' => 'application/json'
]
]);
try {
// Make a GET request to retrieve leads
$response = $client->request('GET', '/v1/leads');
$leads = json_decode($response->getBody(), true);
// Display the leads
foreach ($leads as $lead) {
echo 'Lead Name: ' . $lead['name'] . '<br>';
echo 'Lead Email: ' . $lead['email'] . '<br>';
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
?>
Understanding the Copper API Response
After executing the script, you should see a list of leads retrieved from Copper. The response includes various fields such as lead name and email. You can customize the script to handle additional data as needed.
Handling Errors and Verifying API Requests
It's important to handle potential errors when making API calls. The example script includes a try-catch block to catch exceptions and display error messages. Additionally, verify the success of your requests by checking the response status code and data.
By following these steps, you can efficiently integrate Copper's lead management features into your PHP application, streamlining your sales processes and enhancing customer relationship management.
Best Practices for Copper API Integration in PHP
When integrating with the Copper API using PHP, it's essential to follow best practices to ensure a secure and efficient implementation. Here are some key recommendations:
- Securely Store API Credentials: Always keep your API key, client ID, and client secret secure. Avoid hardcoding them directly into your scripts. Instead, use environment variables or secure vaults.
- Handle Rate Limiting: Copper API has rate limits to prevent abuse. Implement logic to handle HTTP 429 errors by retrying requests after a delay. This ensures your application remains compliant with Copper's usage policies.
- Optimize Data Handling: When retrieving large datasets, consider using pagination to manage data efficiently. This helps in reducing memory usage and improves performance.
- Implement Error Handling: Use try-catch blocks to gracefully handle exceptions and errors. Log errors for debugging and provide meaningful messages to users.
- Regularly Update Dependencies: Keep your PHP environment and dependencies, such as Guzzle, up to date to benefit from security patches and performance improvements.
Enhance Your Integration with Endgrate
Integrating Copper API with PHP can significantly streamline your lead management processes. However, managing multiple integrations can be complex and time-consuming. This is where Endgrate can help.
Endgrate offers a unified API solution that simplifies the integration process across multiple platforms, including Copper. By using Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the complexities of integration.
- Build Once, Use Everywhere: Create a single integration that works across various platforms, reducing the need for multiple implementations.
- Provide a Seamless Experience: Offer your customers an intuitive and consistent integration experience.
Visit Endgrate to learn more about how you can enhance your integration capabilities and streamline your development process.
Read More
Ready to get started?