Using the Xero API to Get Accounts (with PHP examples)
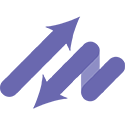
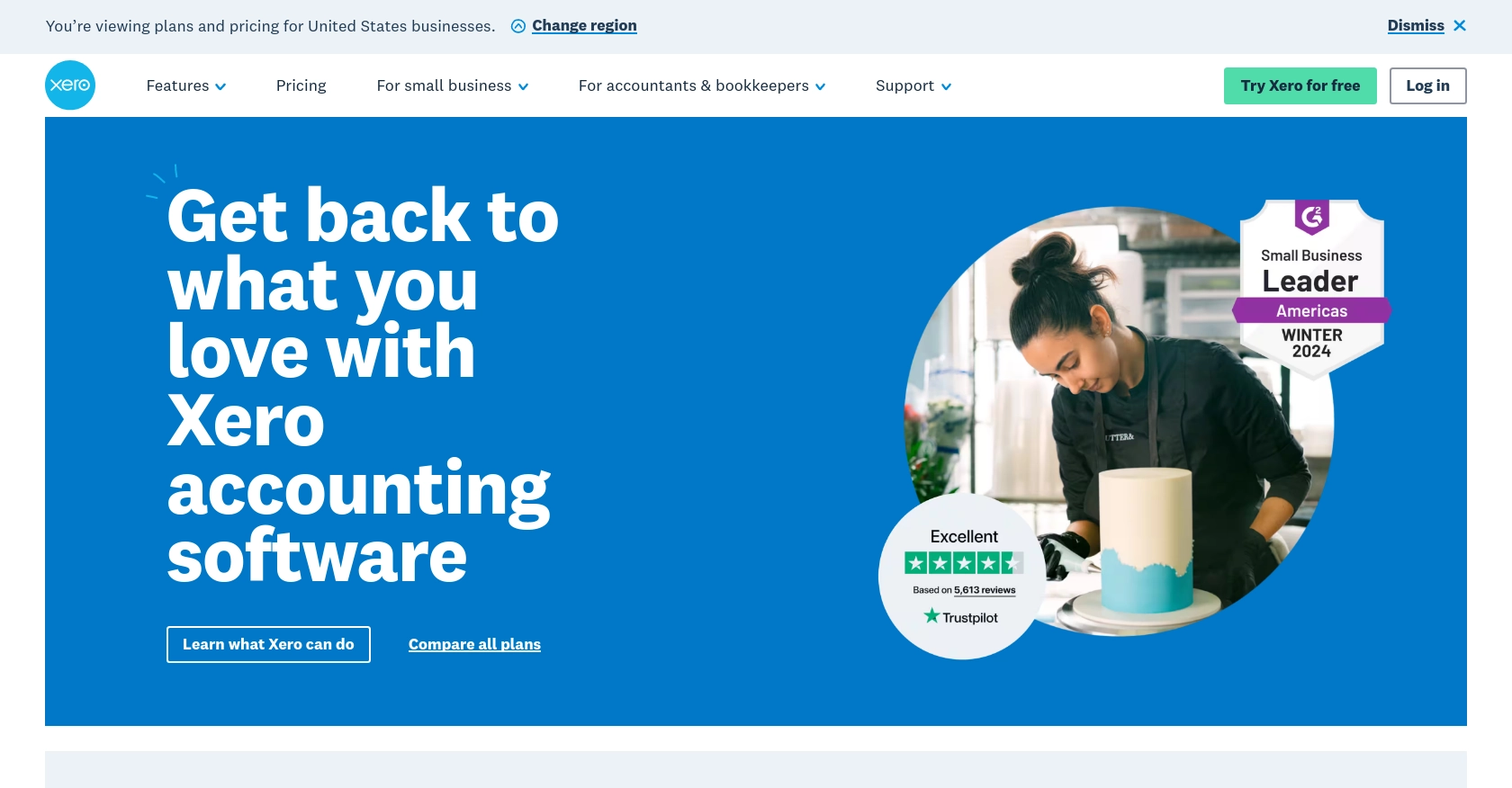
Introduction to Xero Accounting Software
Xero is a powerful cloud-based accounting software designed to simplify financial management for businesses of all sizes. It offers a wide range of features, including invoicing, bank reconciliation, expense tracking, and financial reporting, making it a popular choice among accountants and business owners.
Integrating with Xero's API allows developers to automate and enhance financial processes by accessing and managing accounting data programmatically. For example, a developer might use the Xero API to retrieve account information, enabling seamless synchronization of financial data between Xero and other business applications.
Setting Up Your Xero Sandbox Account for API Integration
Before you can start interacting with the Xero API, you'll need to set up a sandbox account. This allows you to test and develop your integration without affecting live data. Xero provides a demo company that you can use for this purpose.
Creating a Xero Developer Account
To begin, you'll need a Xero developer account. If you don't have one, follow these steps:
- Visit the Xero Developer Portal and sign up for a developer account.
- Once registered, log in to your account to access the developer dashboard.
Accessing the Xero Demo Company
After setting up your developer account, you can access the Xero demo company:
- Log in to your Xero account.
- Navigate to the "My Xero" tab and select "Try the Demo Company."
- This demo company will serve as your sandbox environment for testing API calls.
Creating a Xero App for OAuth 2.0 Authentication
Since the Xero API uses OAuth 2.0 for authentication, you'll need to create an app to obtain the necessary credentials:
- In the Xero Developer Portal, go to the "My Apps" section and click on "New App."
- Fill in the required details, such as the app name and company URL.
- Set the redirect URI, which is used during the OAuth flow.
- Once the app is created, you'll receive a client ID and client secret.
Configuring OAuth 2.0 Scopes
To interact with the Xero API, you'll need to configure the appropriate scopes for your app:
- In your app settings, navigate to the "Scopes" section.
- Select the necessary scopes for accessing account data, such as "accounting.transactions" and "accounting.reports.read."
For more details on OAuth 2.0 authentication, refer to the Xero OAuth 2.0 Overview.
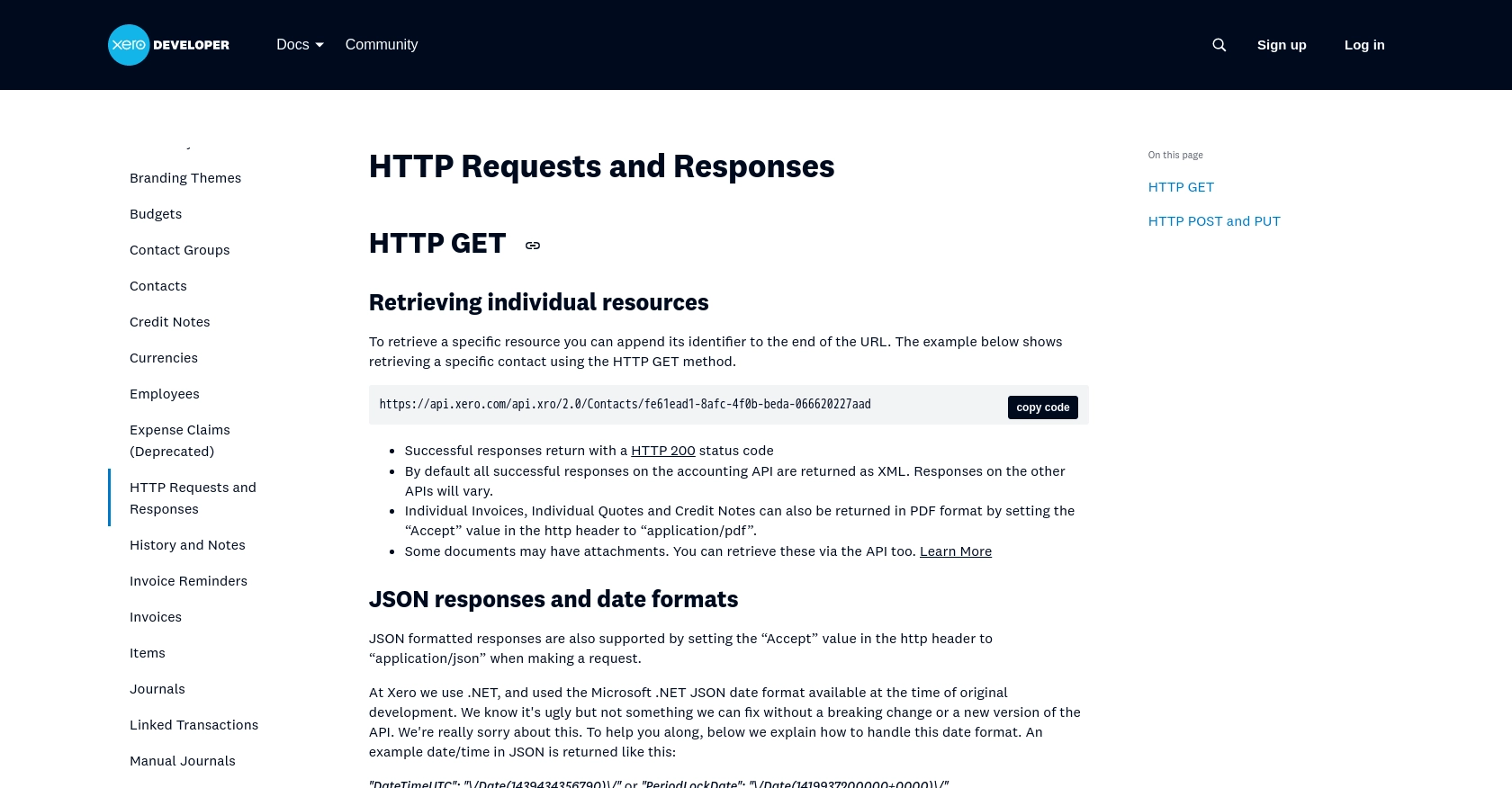
sbb-itb-96038d7
Making API Calls to Retrieve Xero Accounts Using PHP
To interact with the Xero API and retrieve account information, you'll need to use PHP to make HTTP requests. This section will guide you through the process of setting up your PHP environment, making the API call, and handling the response.
Setting Up Your PHP Environment for Xero API Integration
Before making API calls, ensure your PHP environment is properly configured:
- Install PHP 7.4 or later on your machine.
- Ensure you have Composer installed for managing dependencies.
- Use Composer to install the Guzzle HTTP client, which simplifies making HTTP requests:
composer require guzzlehttp/guzzle
Making the API Call to Get Accounts from Xero
With your environment set up, you can now write the PHP code to retrieve accounts from Xero:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token'; // Replace with your actual access token
$tenantId = 'Your_Tenant_ID'; // Replace with your actual tenant ID
$response = $client->request('GET', 'https://api.xero.com/api.xro/2.0/Accounts', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Xero-tenant-id' => $tenantId,
'Accept' => 'application/json',
]
]);
if ($response->getStatusCode() == 200) {
$accounts = json_decode($response->getBody(), true);
foreach ($accounts['Accounts'] as $account) {
echo 'Account Name: ' . $account['Name'] . '<br>';
}
} else {
echo 'Failed to retrieve accounts. Status Code: ' . $response->getStatusCode();
}
Replace Your_Access_Token
and Your_Tenant_ID
with the actual values obtained during the OAuth 2.0 authentication process.
Handling API Responses and Errors
After making the API call, it's crucial to handle the response correctly:
- Check the status code to verify the request was successful (HTTP 200).
- Parse the JSON response to access account details.
- Implement error handling for unsuccessful requests, such as logging errors or retrying the request.
For more information on error codes, refer to the Xero API Requests and Responses Documentation.
Verifying API Call Success in the Xero Sandbox
To ensure your API call was successful, log in to your Xero demo company and verify the retrieved accounts match those in the sandbox environment. This step confirms that your integration is working as expected.
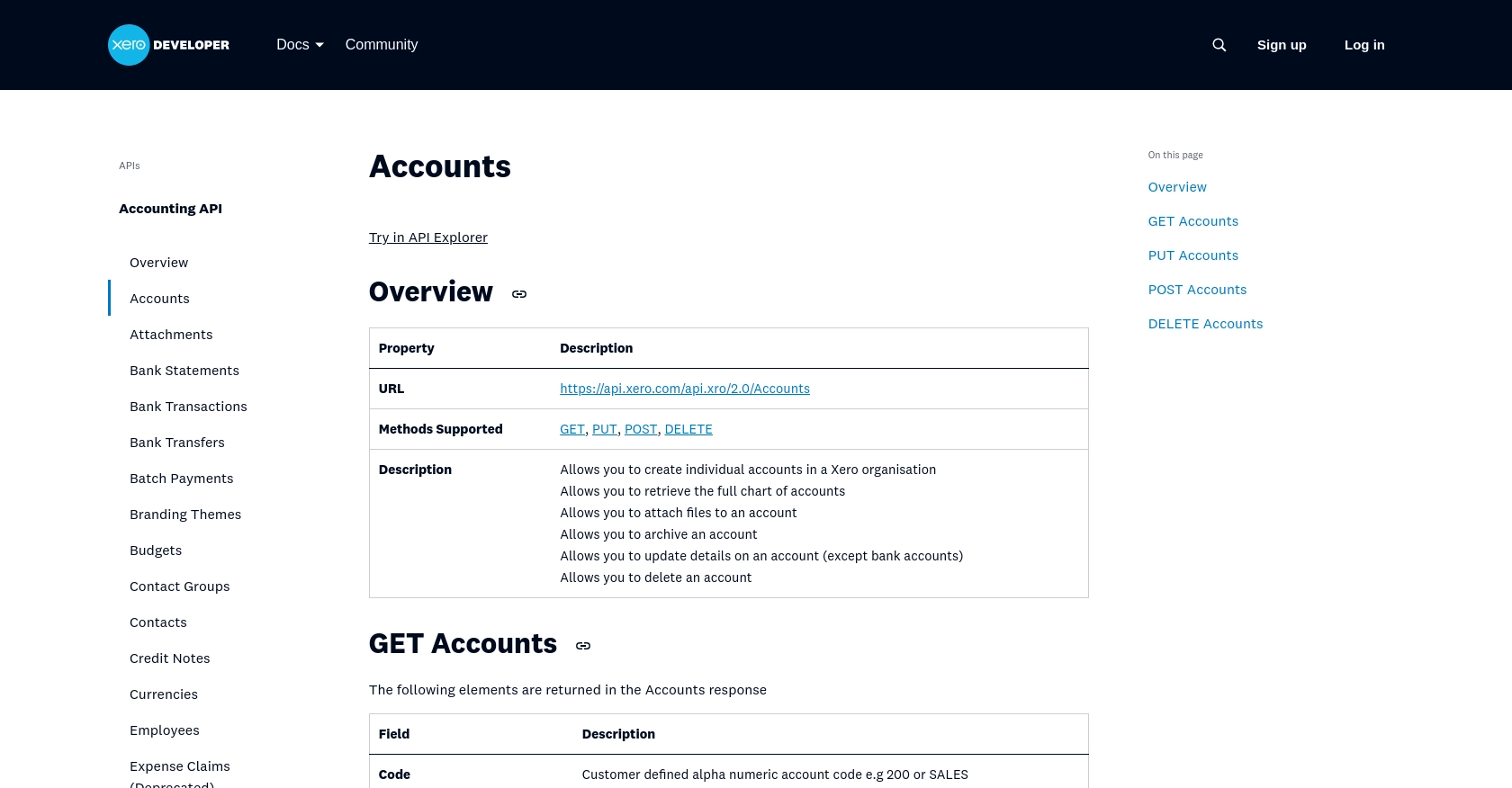
Best Practices for Secure and Efficient Xero API Integration
When integrating with the Xero API, it's essential to follow best practices to ensure security and efficiency. Here are some recommendations:
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Use environment variables or a secure vault to prevent unauthorized access.
- Handle Rate Limiting: Xero imposes rate limits on API requests. Be sure to implement logic to handle HTTP 429 status codes by retrying requests after a delay. For more information, refer to the Xero API Rate Limits Documentation.
- Data Transformation: Standardize and transform data fields as needed to ensure consistency across different systems and applications.
Streamline Your Integration Process with Endgrate
Building and maintaining integrations can be time-consuming and complex. With Endgrate, you can simplify this process by leveraging a unified API endpoint that connects to multiple platforms, including Xero.
Endgrate allows you to focus on your core product by outsourcing integrations, saving time and resources. With a single API call, you can interact with various platforms, providing an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration capabilities by visiting Endgrate today.
Read More
- https://endgrate.com/provider/xero
- https://developer.xero.com/documentation/api/accounting/requests-and-responses
- https://developer.xero.com/documentation/guides/oauth2/limits/
- https://developer.xero.com/documentation/guides/oauth2/overview
- https://developer.xero.com/documentation/guides/oauth2/scopes
- https://developer.xero.com/documentation/guides/oauth2/auth-flow
- https://developer.xero.com/documentation/guides/oauth2/tenants
- https://developer.xero.com/documentation/api/accounting/accounts
Ready to get started?