How to Create or Update Orders with the Sap Business One API in Javascript
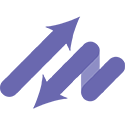
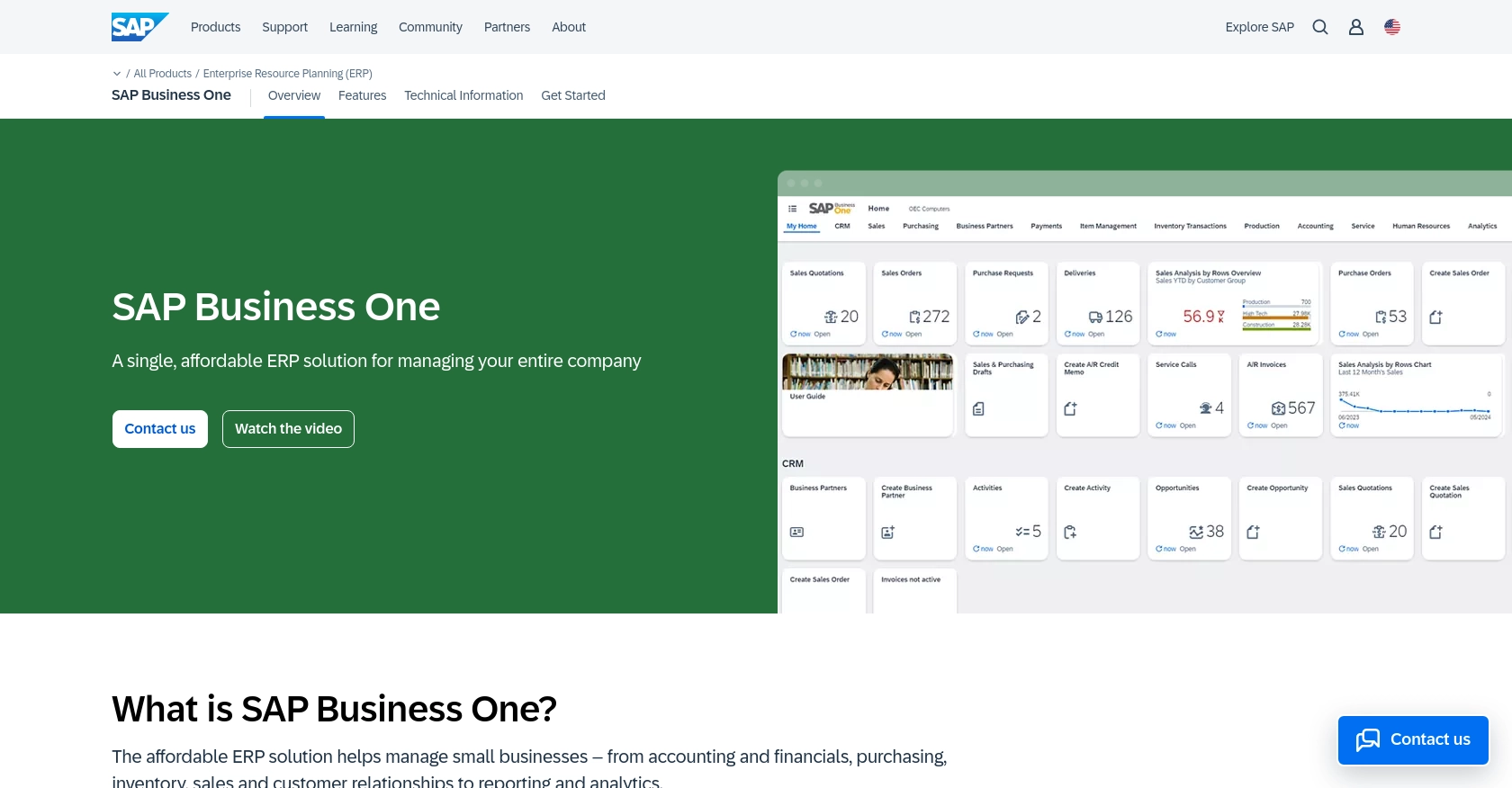
Introduction to SAP Business One
SAP Business One is a comprehensive enterprise resource planning (ERP) solution tailored for small to medium-sized businesses. It offers a wide range of functionalities, including financial management, sales, customer relationship management, and supply chain operations, all integrated into a single platform.
Developers may want to integrate with the SAP Business One API to enhance business processes by automating tasks such as order management. For example, you could use the API to create or update orders directly from an e-commerce platform, ensuring seamless synchronization between sales channels and inventory management.
Setting Up a Test or Sandbox Account for SAP Business One API
To begin integrating with the SAP Business One API, you'll need to set up a test or sandbox environment. This allows you to safely experiment with API calls without affecting live data. Follow these steps to get started:
Registering for a SAP Business One Sandbox Account
If you don't already have access to a SAP Business One sandbox, you can request one through SAP's official channels. This typically involves contacting your SAP representative or accessing the SAP Partner Portal if you are a registered partner.
Configuring OAuth Authentication for SAP Business One
SAP Business One uses a custom authentication method. To authenticate API requests, you need to create an application within your sandbox account. Follow these steps:
- Log in to your SAP Business One sandbox account.
- Navigate to the Administration section and select Setup.
- Under General, choose OAuth Applications and click on Create New Application.
- Fill in the necessary details, such as the application name and redirect URI.
- Once created, note down the Client ID and Client Secret. These will be used to authenticate your API requests.
Generating API Keys for SAP Business One
In addition to OAuth credentials, you may need an API key for certain operations. Here's how to generate one:
- Go to the API Management section in your SAP Business One sandbox.
- Select API Keys and click on Generate New Key.
- Provide a name for the key and select the appropriate permissions.
- Save the generated API key securely, as it will be required for API calls.
With your sandbox account set up and authentication details ready, you can now proceed to make API calls to create or update orders using JavaScript.
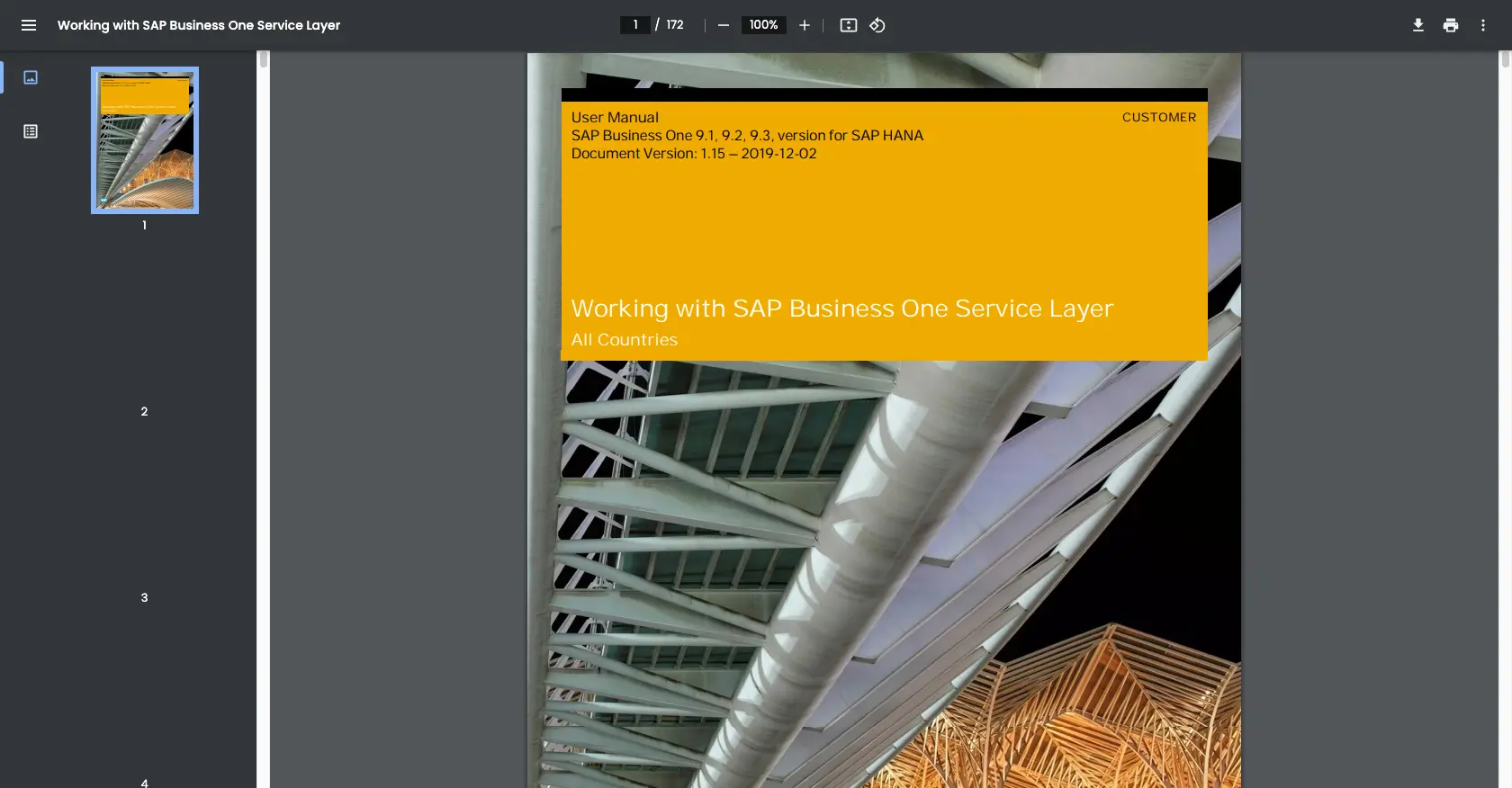
sbb-itb-96038d7
Making API Calls to Create or Update Orders with SAP Business One in JavaScript
To interact with the SAP Business One API using JavaScript, you'll need to ensure you have the correct environment and dependencies set up. This section will guide you through the process of making API calls to create or update orders.
Setting Up Your JavaScript Environment for SAP Business One API
Before you begin, make sure you have Node.js installed on your machine. You can download it from the official Node.js website. Additionally, you'll need the axios
library to handle HTTP requests. Install it using npm:
npm install axios
Creating an Order with SAP Business One API
To create an order, you'll need to make a POST request to the SAP Business One API endpoint. Here's a sample code snippet to help you get started:
const axios = require('axios');
const createOrder = async () => {
const endpoint = 'https://your-sap-business-one-instance.com/b1s/v1/Orders';
const headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer Your_Access_Token'
};
const orderData = {
CardCode: 'C20000',
DocDueDate: '2023-10-15',
DocumentLines: [
{
ItemCode: 'A00001',
Quantity: 10,
Price: 15.00
}
]
};
try {
const response = await axios.post(endpoint, orderData, { headers });
console.log('Order Created:', response.data);
} catch (error) {
console.error('Error creating order:', error.response ? error.response.data : error.message);
}
};
createOrder();
Replace Your_Access_Token
with the token obtained from your SAP Business One sandbox account. The orderData
object contains the necessary details for the order, such as customer code, due date, and line items.
Updating an Order with SAP Business One API
To update an existing order, you'll need to make a PATCH request. Here's how you can do it:
const updateOrder = async (orderId) => {
const endpoint = `https://your-sap-business-one-instance.com/b1s/v1/Orders(${orderId})`;
const headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer Your_Access_Token'
};
const updatedData = {
DocumentLines: [
{
LineNum: 0,
Quantity: 20
}
]
};
try {
const response = await axios.patch(endpoint, updatedData, { headers });
console.log('Order Updated:', response.data);
} catch (error) {
console.error('Error updating order:', error.response ? error.response.data : error.message);
}
};
updateOrder(12345);
In this example, replace orderId
with the ID of the order you wish to update. The updatedData
object specifies the changes, such as modifying the quantity of a line item.
Verifying API Call Success in SAP Business One
After making API calls, verify the success of your operations by checking the SAP Business One sandbox environment. For order creation, ensure the new order appears in the orders list. For updates, confirm that the changes reflect in the order details.
Handling Errors and Error Codes
When making API calls, handle potential errors gracefully. The SAP Business One API returns error codes and messages that can help diagnose issues. Common error codes include:
- 400 Bad Request: The request was malformed or contains invalid data.
- 401 Unauthorized: Authentication failed due to invalid credentials.
- 404 Not Found: The specified resource does not exist.
Refer to the SAP Business One Service Layer documentation for more detailed information on error handling.
Conclusion and Best Practices for Using SAP Business One API in JavaScript
Integrating with the SAP Business One API using JavaScript can significantly enhance your business operations by automating order management processes. By following the steps outlined in this guide, you can efficiently create and update orders, ensuring seamless synchronization between your e-commerce platform and SAP Business One.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your API keys and OAuth credentials securely. Consider using environment variables or a secure vault to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of the API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that data fields are standardized and validated before making API calls to prevent errors and maintain data integrity.
Streamlining Integrations with Endgrate
While integrating with SAP Business One can be highly beneficial, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies this process by providing a single endpoint for various platforms, including SAP Business One.
By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development. Whether you need to build once for each use case or provide an intuitive integration experience for your customers, Endgrate can help streamline your integration efforts.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?