Using the Cloze API to Create or Update Companies in Javascript
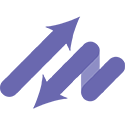
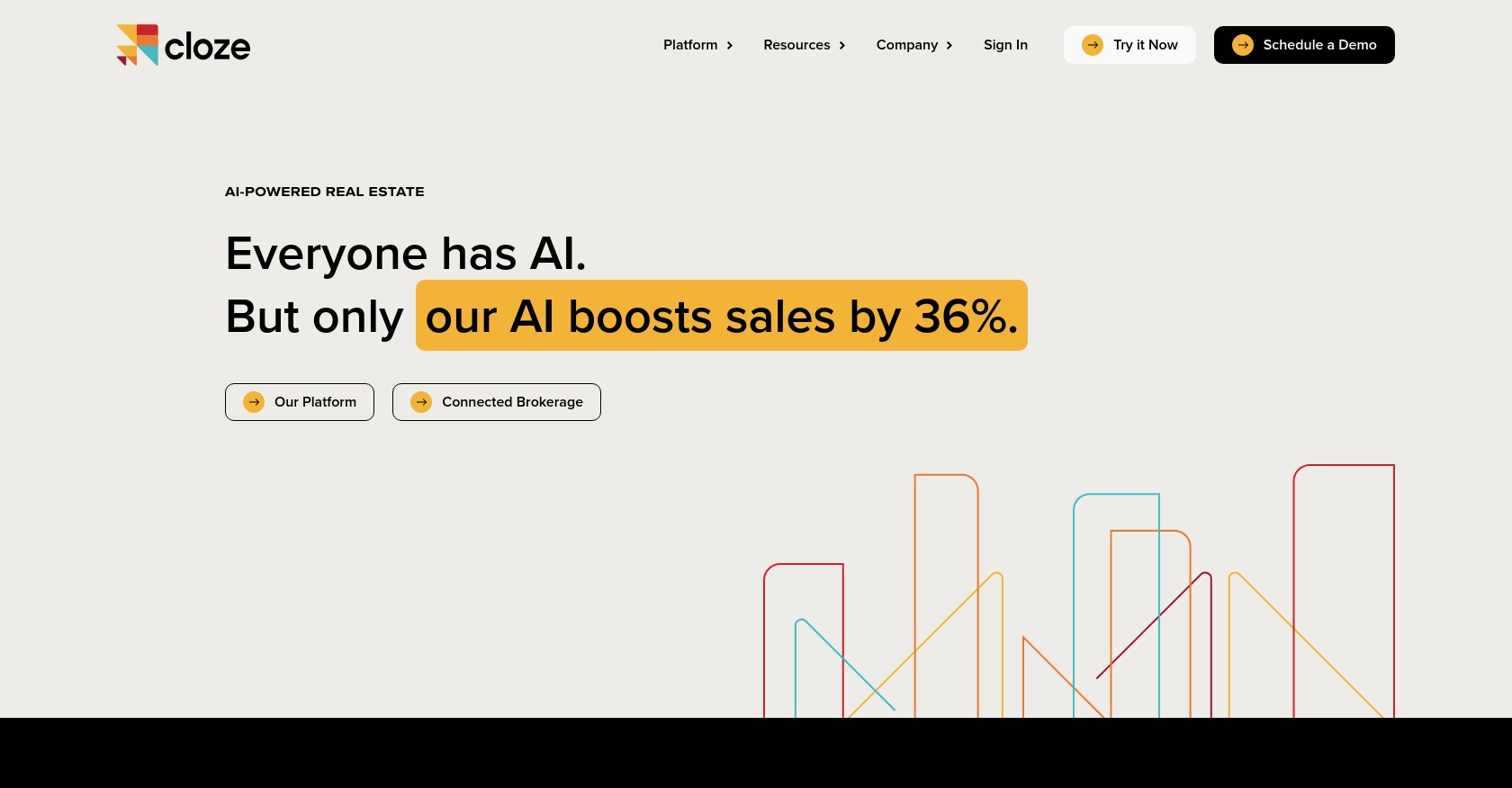
Introduction to Cloze API for Company Management
Cloze is a powerful relationship management platform that helps businesses streamline their interactions with clients, partners, and other stakeholders. By integrating with the Cloze API, developers can automate and enhance the management of company data, ensuring seamless communication and collaboration.
Connecting with the Cloze API allows developers to create or update company records efficiently. For example, you might want to automatically update company profiles with the latest contact information from an external CRM, ensuring that your team always has access to the most current data.
Setting Up Your Cloze API Test or Sandbox Account
Before you begin integrating with the Cloze API, it's essential to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data. Follow these steps to get started:
Create a Cloze Account
- Visit the Cloze website and sign up for a free account if you don't already have one.
- Once registered, log in to your Cloze dashboard.
Generate an API Key for Cloze Integration
To authenticate your API requests, you'll need an API key. Here's how to generate one:
- Navigate to the settings section in your Cloze dashboard.
- Find the API settings or developer section.
- Follow the instructions to create a new API key. Make sure to note down the key as you'll need it for authentication.
For more detailed instructions, refer to the Cloze API documentation.
Configure OAuth for Public Integrations
If you're developing a public integration, OAuth is required for user authentication:
- Contact Cloze support at support@cloze.com to initiate the OAuth setup process.
- During development, you can use the API key for testing purposes.
With your API key or OAuth setup, you're ready to start making API calls to create or update company records in Cloze.
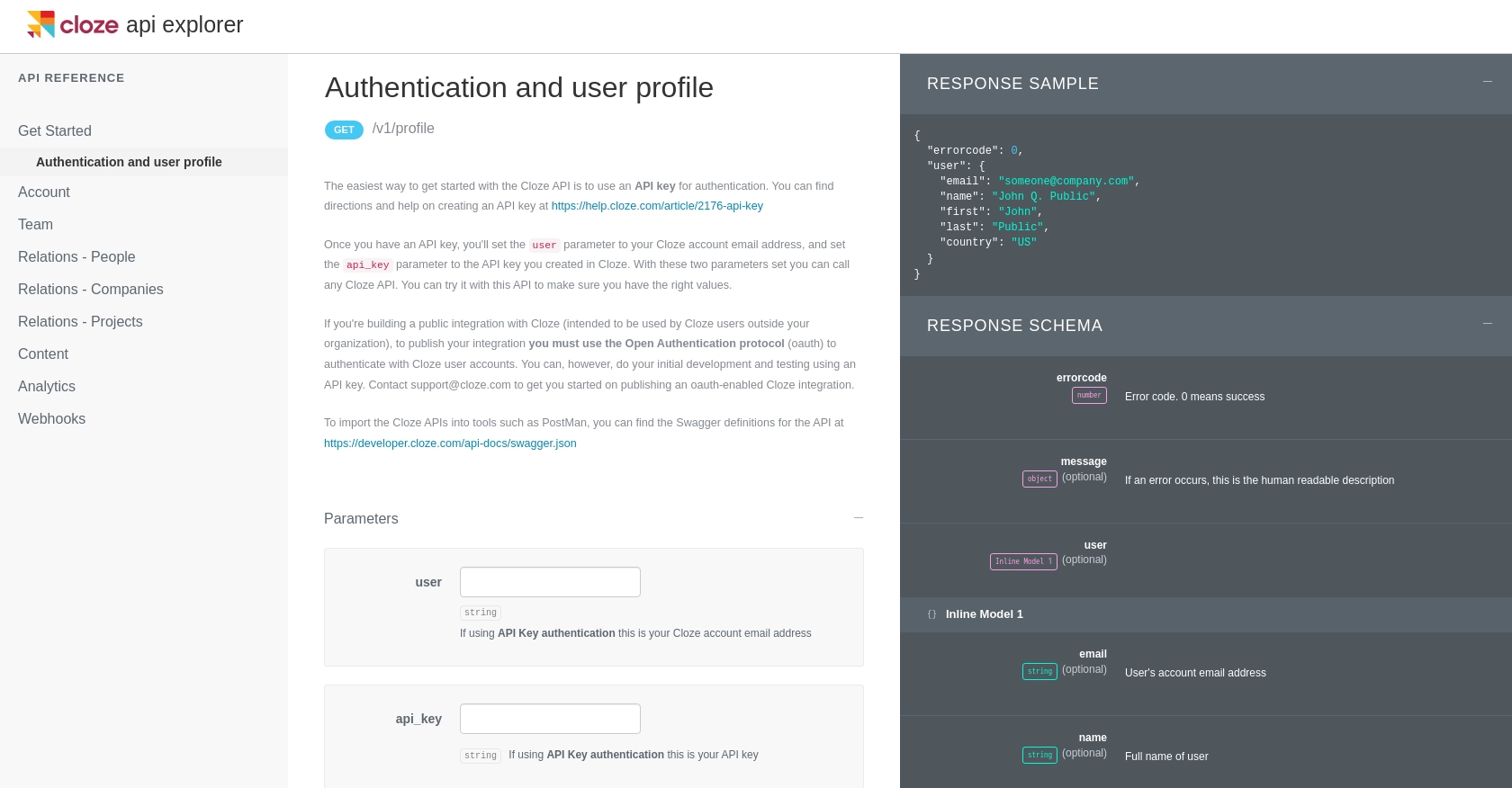
sbb-itb-96038d7
Making API Calls to Create or Update Companies in Cloze Using JavaScript
To interact with the Cloze API for creating or updating company records, you need to use JavaScript to make HTTP requests. This section will guide you through the process, including setting up your environment and writing the code to perform these actions.
Setting Up Your JavaScript Environment for Cloze API Integration
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine. You can download it from the official Node.js website.
- A code editor, such as Visual Studio Code, to write and execute your JavaScript code.
Once you have Node.js installed, you can use npm (Node Package Manager) to install the necessary dependencies.
Installing Required Dependencies for Cloze API Calls
To make HTTP requests in JavaScript, you'll need the axios
library. Install it using the following command:
npm install axios
Writing JavaScript Code to Create or Update Companies in Cloze
Now, let's write the JavaScript code to create or update a company record in Cloze. Here's a sample script:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.cloze.com/v1/companies/create';
const headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer YOUR_API_KEY'
};
// Define the company data
const companyData = {
name: 'Example Company',
description: 'A sample company for demonstration purposes',
domains: ['example.com'],
stage: 'lead',
segment: 'customer'
};
// Make a POST request to create or update the company
axios.post(endpoint, companyData, { headers })
.then(response => {
console.log('Company created/updated successfully:', response.data);
})
.catch(error => {
console.error('Error creating/updating company:', error.response ? error.response.data : error.message);
});
Replace YOUR_API_KEY
with the API key you generated earlier. This script uses the axios
library to send a POST request to the Cloze API, creating or updating a company record with the specified data.
Verifying Successful API Requests in Cloze
After running the script, you can verify the success of your API call by checking the response in the console. Additionally, log in to your Cloze dashboard to ensure the company record has been created or updated as expected.
Handling Errors and Understanding Cloze API Error Codes
When making API calls, it's crucial to handle potential errors. The sample code above includes a catch
block to log any errors that occur during the request. For more detailed error information, refer to the Cloze API documentation.
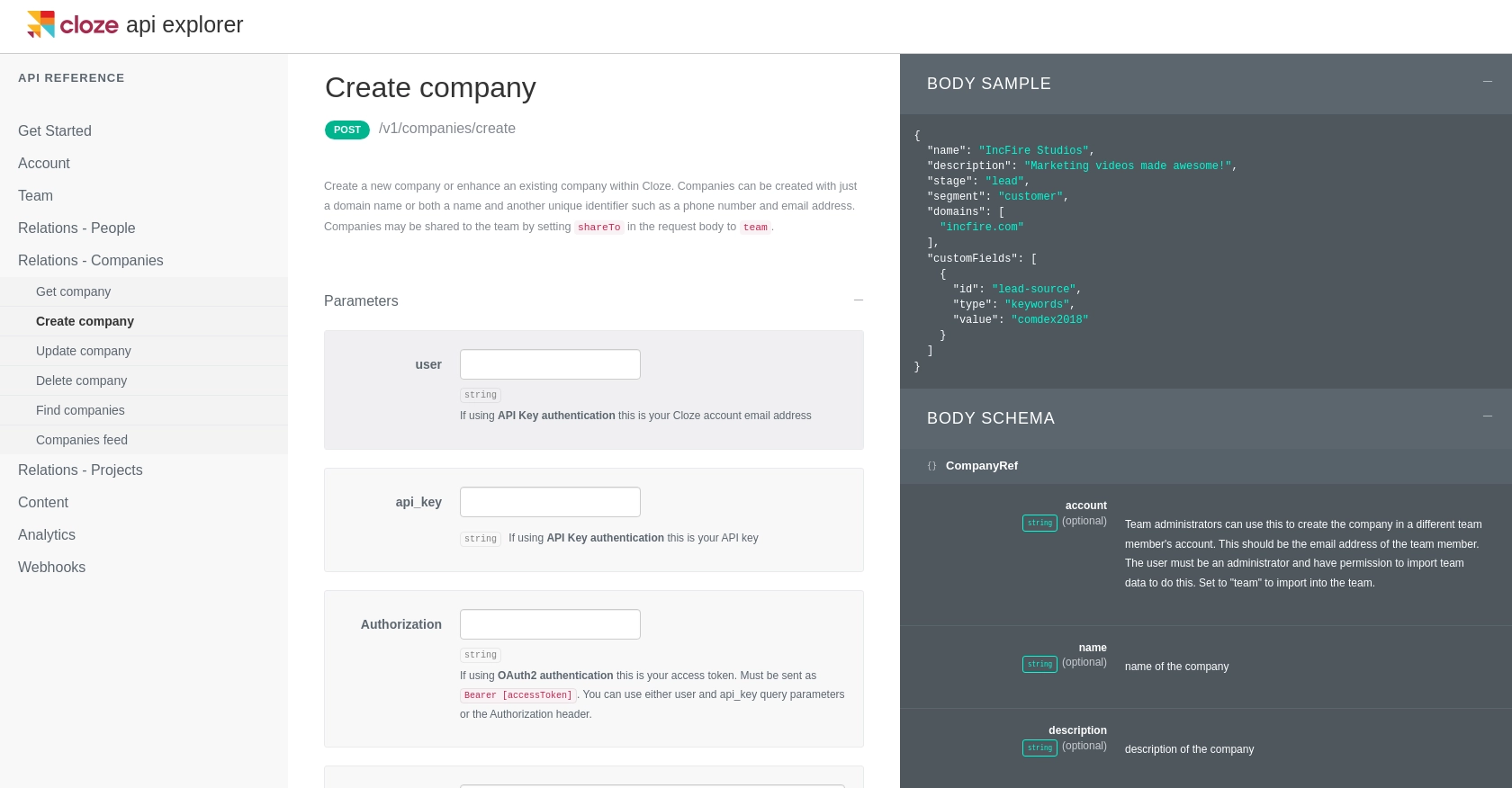
Conclusion and Best Practices for Using Cloze API in JavaScript
Integrating with the Cloze API using JavaScript provides a robust solution for managing company data efficiently. By following the steps outlined in this guide, you can create or update company records seamlessly, ensuring your team has access to the most up-to-date information.
Best Practices for Secure and Efficient API Integration with Cloze
- Secure Storage of API Keys: Always store your API keys securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of the API rate limits and implement retry logic to handle rate limit errors gracefully. Refer to the Cloze API documentation for specific rate limit details.
- Data Standardization: Ensure that data fields are standardized and consistent with your existing systems to facilitate smooth integration and data management.
Leverage Endgrate for Streamlined Integration Solutions
If managing multiple integrations becomes overwhelming, consider using Endgrate to simplify the process. Endgrate allows you to build once for each use case, providing a unified API endpoint that connects to various platforms, including Cloze. This approach saves time and resources, allowing you to focus on your core product.
Explore how Endgrate can enhance your integration experience by visiting Endgrate's website.
Read More
Ready to get started?