Using the Younium API to Get Invoices (with Python examples)
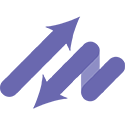
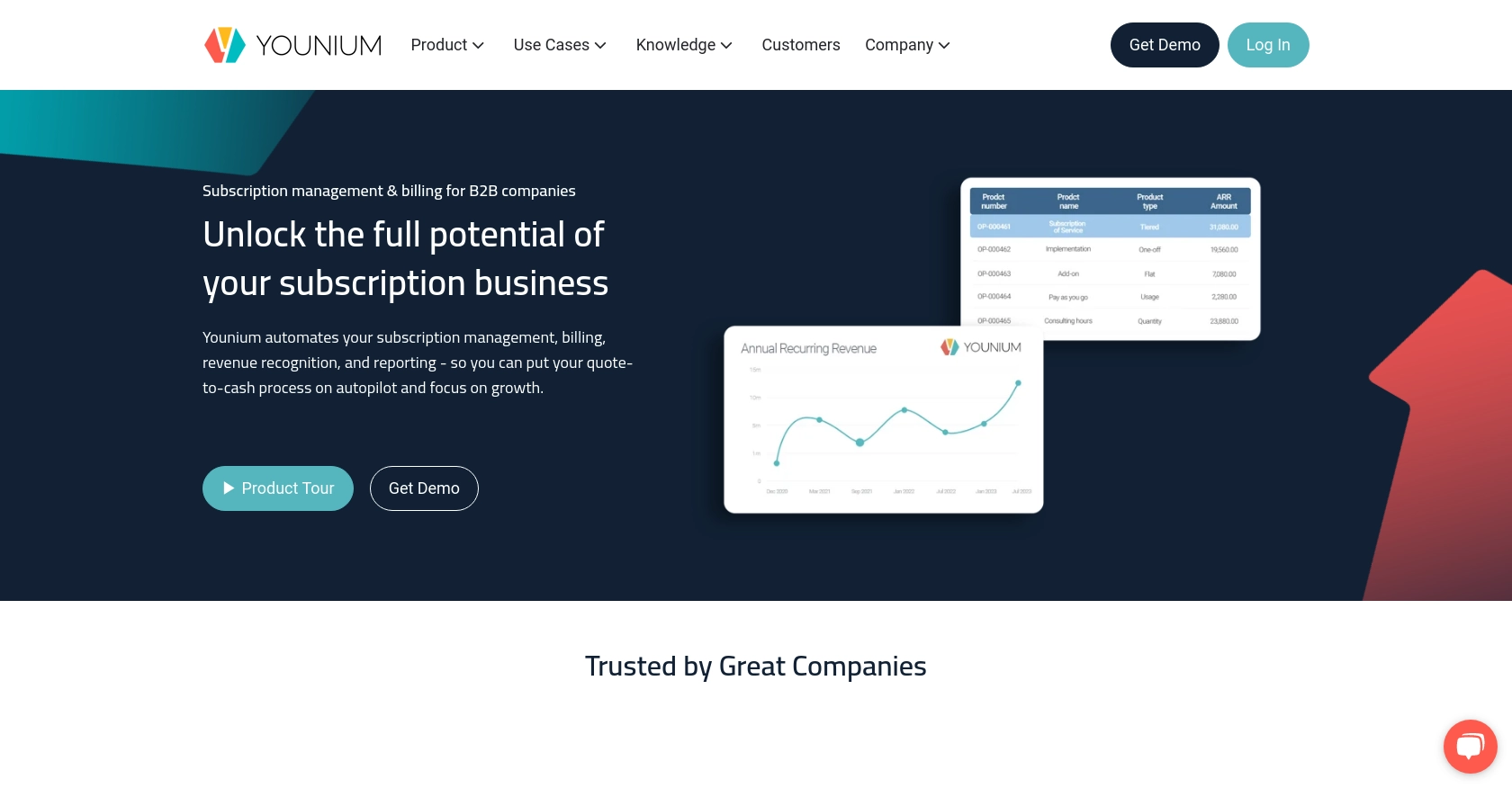
Introduction to Younium API Integration
Younium is a comprehensive subscription management platform designed to streamline billing and invoicing processes for B2B SaaS companies. It offers robust tools for managing subscriptions, invoicing, and financial reporting, making it an essential solution for businesses looking to optimize their financial operations.
Integrating with the Younium API allows developers to automate and enhance their invoicing processes. For example, a developer might use the Younium API to retrieve invoice data and integrate it into a custom financial dashboard, providing real-time insights into billing activities and revenue streams.
Setting Up Your Younium Test/Sandbox Account for API Integration
Before you can start using the Younium API to retrieve invoices, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data. Younium provides a sandbox environment that mimics the production environment, making it ideal for development and testing purposes.
Creating a Younium Sandbox Account
To get started, you'll need to create a Younium sandbox account. Follow these steps:
- Visit the Younium Developer Portal.
- Sign up for a sandbox account by following the registration process. If you already have an account, simply log in.
- Once logged in, navigate to the sandbox environment to begin setting up your API credentials.
Generating API Tokens and Client Credentials
Younium uses JWT access tokens for API authentication. Here's how to generate the necessary tokens:
- Open your user profile menu by clicking your name in the top right corner and select "Privacy & Security".
- In the left panel, click on "Personal Tokens" and then "Generate Token".
- Provide a relevant description for your token and click "Create".
- Copy the generated Client ID and Secret Key. These credentials will be used to generate your JWT access token. Note that these values will not be visible again, so ensure they are stored securely.
Acquiring a JWT Access Token
With your client credentials ready, you can now generate a JWT access token:
import requests
# Define the endpoint and headers
url = "https://api.sandbox.younium.com/auth/token"
headers = {"Content-Type": "application/json"}
# Define the request body with your client credentials
body = {
"clientId": "Your_Client_ID",
"secret": "Your_Secret_Key"
}
# Make a POST request to acquire the JWT token
response = requests.post(url, json=body, headers=headers)
# Extract the access token from the response
token_data = response.json()
access_token = token_data.get("accessToken")
print("Access Token:", access_token)
Replace Your_Client_ID
and Your_Secret_Key
with the credentials you generated earlier. This script will return a JWT access token, valid for 24 hours, which you will use to authenticate your API requests.
If you encounter any issues, such as a 400 or 401 error, double-check your credentials and ensure they are correct. For more details, refer to the Younium Authentication Documentation.
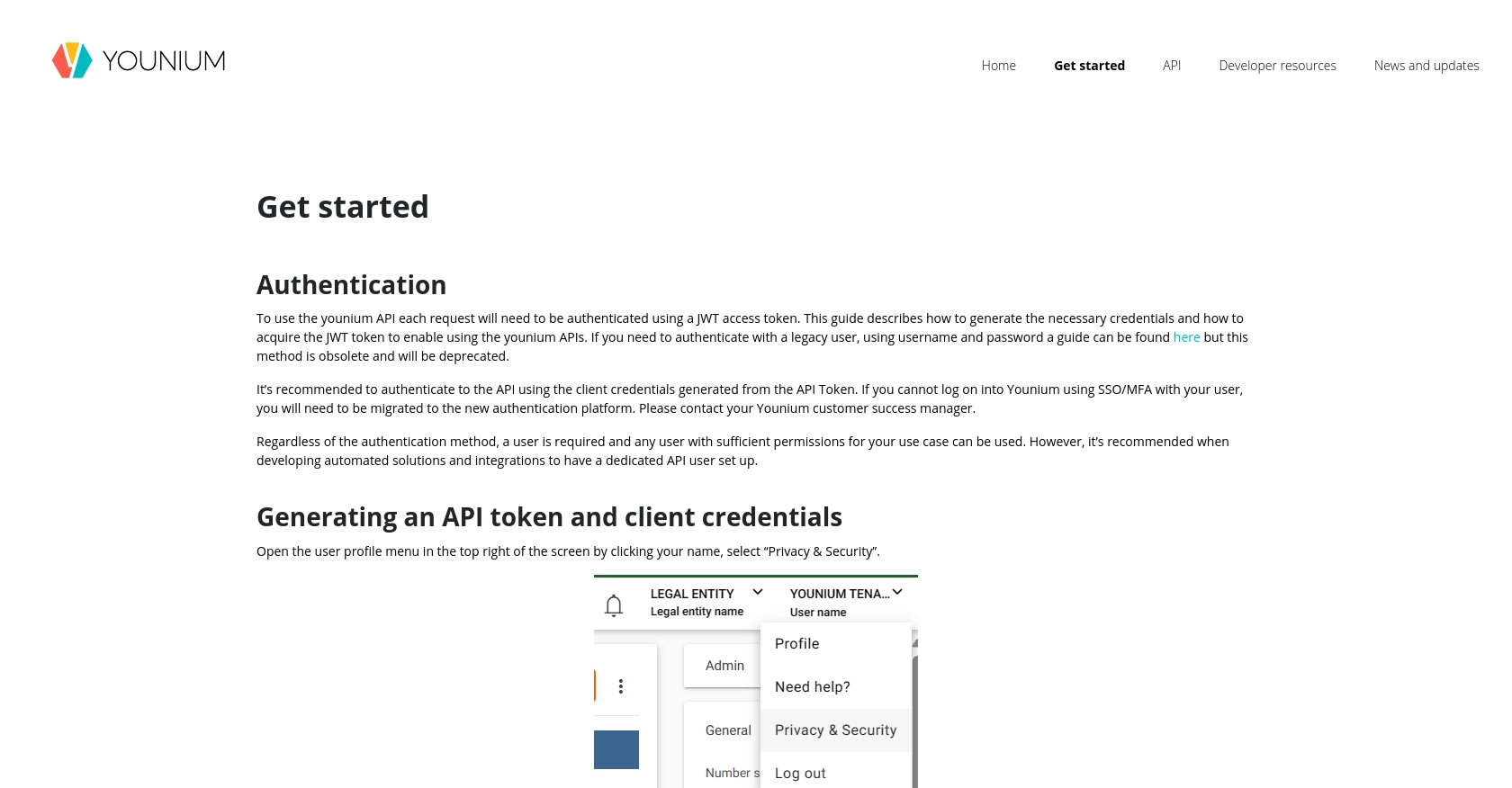
sbb-itb-96038d7
Making API Calls to Retrieve Invoices Using Younium API with Python
To effectively interact with the Younium API and retrieve invoice data, you'll need to make authenticated API calls using Python. This section will guide you through the process of setting up your Python environment, making the necessary API requests, and handling responses.
Setting Up Your Python Environment for Younium API Integration
Before making API calls, ensure your Python environment is properly configured. You'll need Python 3.x and the requests
library to handle HTTP requests. If you haven't installed the requests
library, you can do so using pip:
pip install requests
Retrieving Invoices from Younium API
Once your environment is set up, you can proceed to retrieve invoices from the Younium API. The following Python script demonstrates how to make a GET request to the Younium API to fetch invoice data:
import requests
# Define the API endpoint and headers
endpoint = "https://api.sandbox.younium.com/invoices"
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/json",
"api-version": "2.1"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
invoices = response.json()
print("Invoices retrieved successfully:", invoices)
else:
print("Failed to retrieve invoices. Status code:", response.status_code)
print("Error message:", response.json().get("error"))
Replace Your_Access_Token
with the JWT access token you obtained earlier. This script sends a GET request to the Younium API to retrieve invoices. If successful, it prints the retrieved invoice data.
Verifying API Call Success and Handling Errors
After executing the script, verify the success of your API call by checking the response status code. A status code of 200 indicates a successful request. If the request fails, the script will output the status code and error message, helping you diagnose the issue.
Common error codes include:
- 401 Unauthorized: Indicates an expired or incorrect access token. Ensure your token is valid and correctly included in the request headers.
- 403 Forbidden: May occur if the specified legal entity is incorrect or if the user lacks necessary permissions. Verify the legal entity and user permissions.
For more details on error handling, refer to the Younium API Documentation.
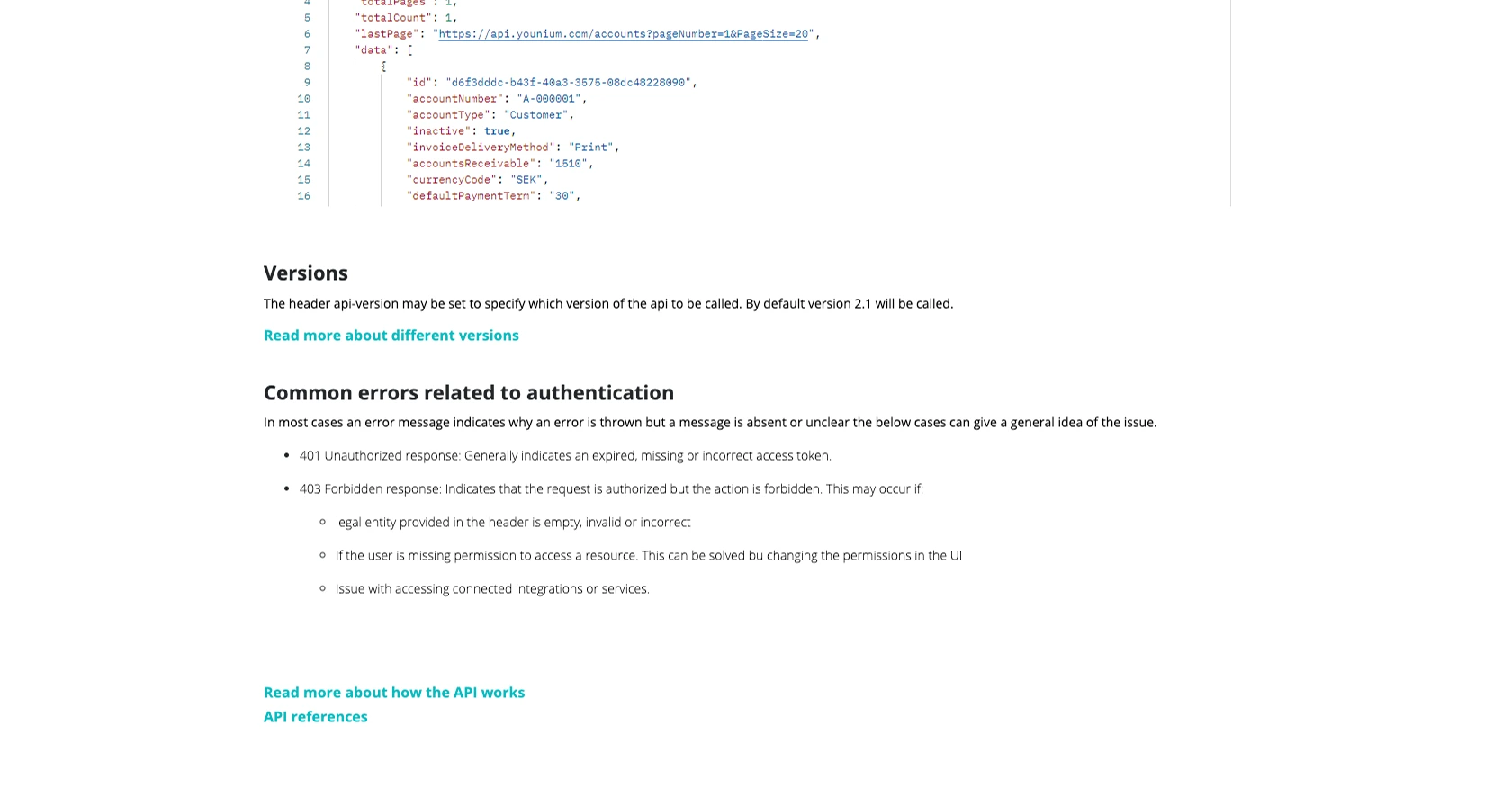
Conclusion and Best Practices for Using Younium API with Python
Integrating with the Younium API can significantly enhance your invoicing and subscription management processes, providing real-time insights and automation capabilities. By following the steps outlined in this guide, you can efficiently retrieve invoice data using Python, ensuring seamless integration with your existing systems.
Best Practices for Secure and Efficient Younium API Integration
- Secure Storage of Credentials: Always store your Client ID, Secret Key, and JWT access token securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handling Rate Limits: Be mindful of the API's rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Data Standardization: Ensure that the data retrieved from the Younium API is standardized and transformed as needed to fit your application's requirements.
- Regular Token Renewal: Remember that the JWT access token is valid for 24 hours. Implement a mechanism to refresh the token automatically to maintain uninterrupted access to the API.
Leverage Endgrate for Simplified Integration Solutions
While integrating with the Younium API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies integration processes across various platforms, including Younium.
By using Endgrate, you can save time and resources, allowing your team to focus on core product development. With a single API endpoint, you can manage multiple integrations efficiently, providing an intuitive experience for your customers.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate and discover the benefits of a unified integration platform.
Read More
Ready to get started?