Using the Slack API to Send Messages (User DM) in PHP
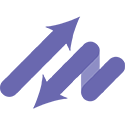
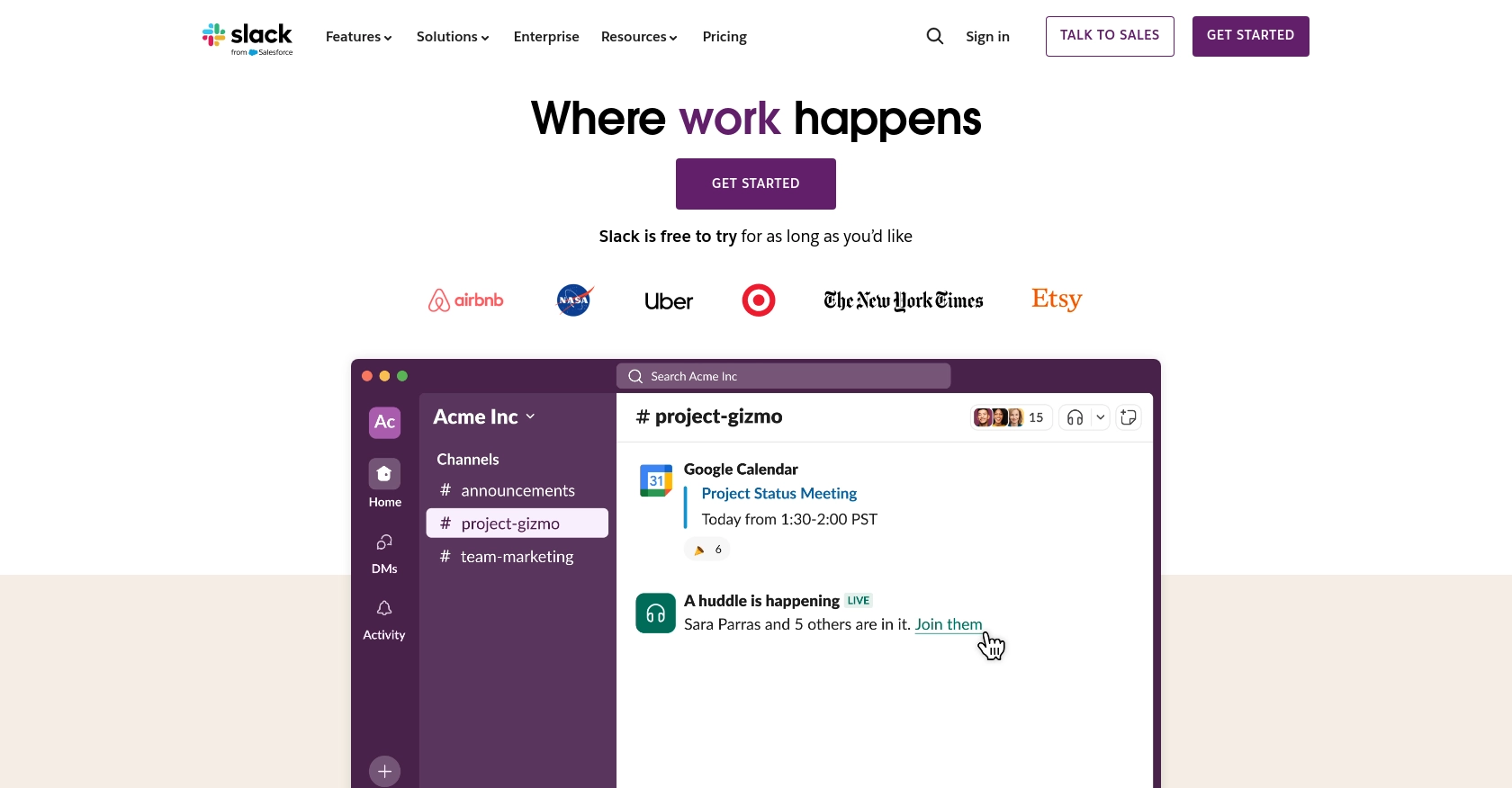
Introduction to Slack API for Direct Messaging
Slack is a powerful collaboration platform that enables teams to communicate and work together seamlessly. With its robust set of features, including channels, direct messaging, and integrations, Slack has become a staple in modern workplaces.
Developers often need to integrate with Slack's API to enhance team communication by automating message delivery. For example, using the Slack API to send direct messages (DMs) can streamline notifications, reminders, or alerts directly to users, ensuring important information is delivered promptly.
This article will guide you through using PHP to interact with the Slack API, specifically focusing on sending direct messages to users. By the end of this tutorial, you'll be equipped to automate messaging within Slack, enhancing your team's productivity and communication.
Setting Up Your Slack Developer Account and App for Direct Messaging
Before you can start sending direct messages using the Slack API, you need to set up a Slack developer account and create an app. This process involves obtaining the necessary credentials and permissions to interact with Slack's API.
Creating a Slack Developer Account
If you don't already have a Slack developer account, you'll need to create one. Follow these steps to get started:
- Visit the Slack API Quickstart page.
- Click on "Your Apps" and select "Create New App."
- Choose "From scratch" and enter your app's name, such as "DM Bot."
- Select the workspace where you want to develop your app and click "Create App."
Requesting OAuth Scopes for Slack API Access
To send direct messages, your app needs specific permissions. You'll need to request OAuth scopes to grant these permissions:
- Navigate to "OAuth & Permissions" in your app's settings.
- Under "Scopes," add the following Bot Token Scopes:
chat:write
- Allows your app to send messages.im:write
- Allows your app to send direct messages.
- Click "Save Changes" to update your app's permissions.
Installing and Authorizing Your Slack App
After setting up your app and requesting the necessary scopes, you need to install and authorize it within your Slack workspace:
- Go back to the "Basic Information" section of your app's settings.
- Click "Install to Workspace" to initiate the OAuth flow.
- Authorize the app by clicking "Allow" on the permissions screen.
- Once authorized, you'll receive an OAuth access token. Store this token securely as it will be used to authenticate API requests.
With your Slack developer account and app set up, you're now ready to start sending direct messages using the Slack API in PHP. In the next section, we'll cover how to make the actual API call to send a message.
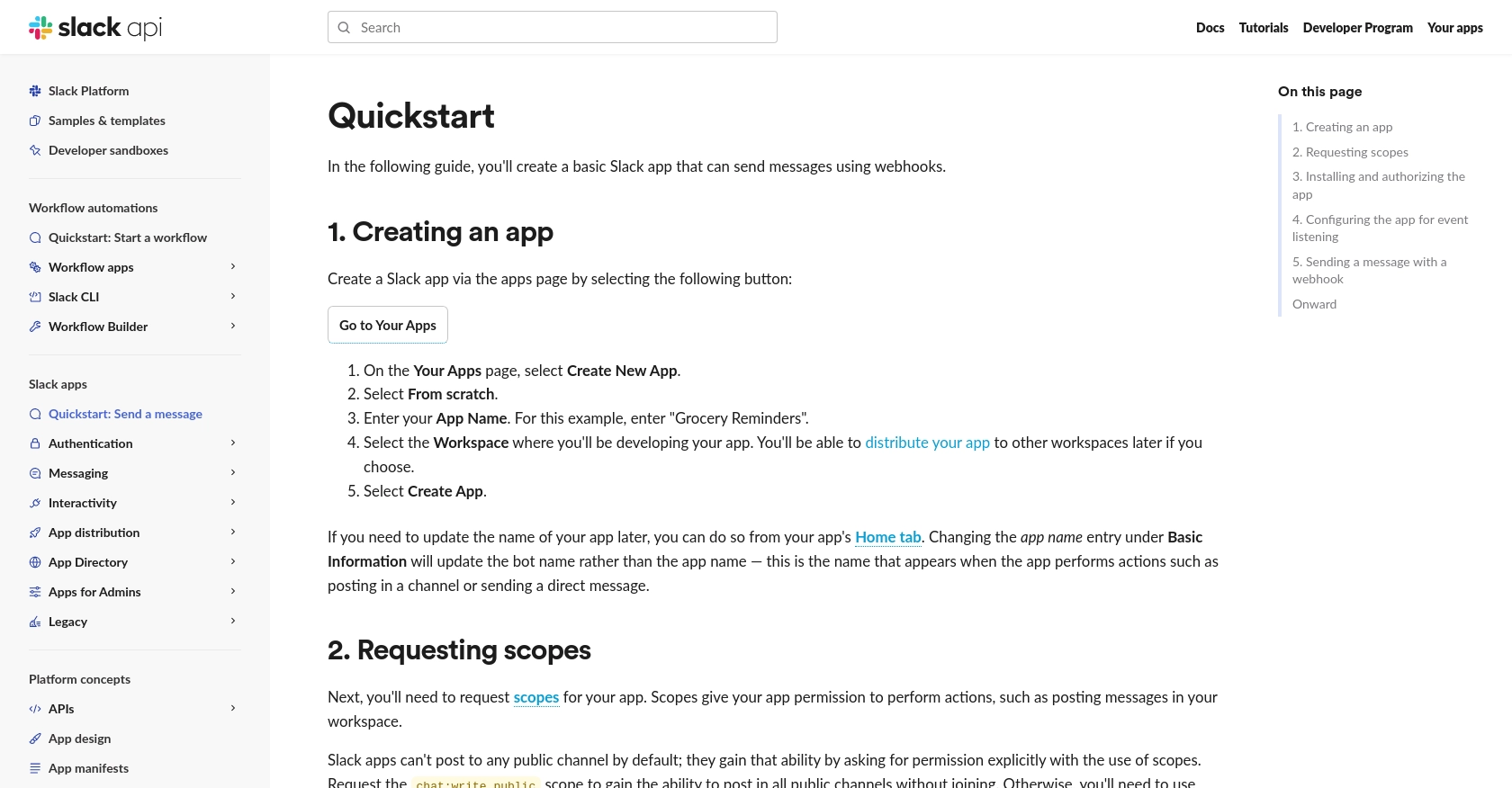
sbb-itb-96038d7
Making the Slack API Call to Send Direct Messages Using PHP
With your Slack app set up and authorized, it's time to dive into the code and make the actual API call to send direct messages. This section will guide you through the necessary steps to interact with the Slack API using PHP, ensuring you can automate messaging effectively.
Setting Up Your PHP Environment for Slack API Integration
Before making API calls, ensure your PHP environment is ready. You'll need PHP 7.4 or higher and the curl
extension enabled to handle HTTP requests. Follow these steps to set up your environment:
- Verify your PHP version by running
php -v
in your terminal. - Ensure the
curl
extension is enabled in yourphp.ini
file. - Restart your web server to apply any changes.
Installing Required PHP Dependencies for Slack API
To interact with the Slack API, you'll need the guzzlehttp/guzzle
library, a popular HTTP client for PHP. Install it using Composer:
composer require guzzlehttp/guzzle
Writing PHP Code to Send Direct Messages via Slack API
Now that your environment is set up, you can write the PHP code to send direct messages. Follow the example below to create a script that sends a message to a user:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$token = 'YOUR_SLACK_OAUTH_ACCESS_TOKEN';
$userId = 'USER_ID_TO_MESSAGE';
$message = 'Hello, this is a test message from your Slack app!';
$response = $client->post('https://slack.com/api/chat.postMessage', [
'headers' => [
'Authorization' => 'Bearer ' . $token,
'Content-Type' => 'application/json',
],
'json' => [
'channel' => $userId,
'text' => $message,
],
]);
$body = json_decode($response->getBody(), true);
if ($body['ok']) {
echo "Message sent successfully!";
} else {
echo "Error: " . $body['error'];
}
Replace YOUR_SLACK_OAUTH_ACCESS_TOKEN
with your actual OAuth access token and USER_ID_TO_MESSAGE
with the Slack user ID you wish to message.
Verifying Successful Slack API Requests and Handling Errors
After running your script, check the response to verify the message was sent successfully. The response will include an ok
field indicating success or failure. If the request fails, the error
field will provide details.
Common error codes include:
invalid_auth
: The access token is invalid.channel_not_found
: The specified channel or user ID is incorrect.rate_limited
: The app has exceeded the rate limit for API calls.
For more details on error handling, refer to the Slack API documentation.
.webp)
Best Practices for Using Slack API in PHP
Integrating with the Slack API to send direct messages in PHP can significantly enhance your team's communication and productivity. However, following best practices ensures your integration is secure, efficient, and scalable.
Securely Storing Slack API Credentials
Always store your Slack OAuth access token securely. Avoid hardcoding it in your scripts or sharing it publicly. Use environment variables or secure vaults to manage sensitive information.
Handling Slack API Rate Limits
Slack imposes rate limits on API calls to prevent abuse. Monitor your API usage and implement retry logic with exponential backoff to handle rate_limited
errors gracefully. For more details, refer to the Slack API documentation.
Standardizing and Transforming Data
Ensure that the data you send via the Slack API is standardized and formatted correctly. This includes validating user IDs and message content to prevent errors and ensure smooth communication.
Enhancing Your Integration Experience with Endgrate
While building Slack integrations can be rewarding, it can also be time-consuming and complex. Endgrate offers a streamlined solution to manage integrations efficiently.
- Save time and resources by outsourcing integrations, allowing you to focus on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Provide an intuitive integration experience for your customers with Endgrate's unified API.
Explore how Endgrate can simplify your integration process by visiting Endgrate.
Read More
Ready to get started?