How to Create or Update Contacts with the Microsoft Dynamics 365 Business Central API in Javascript
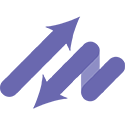
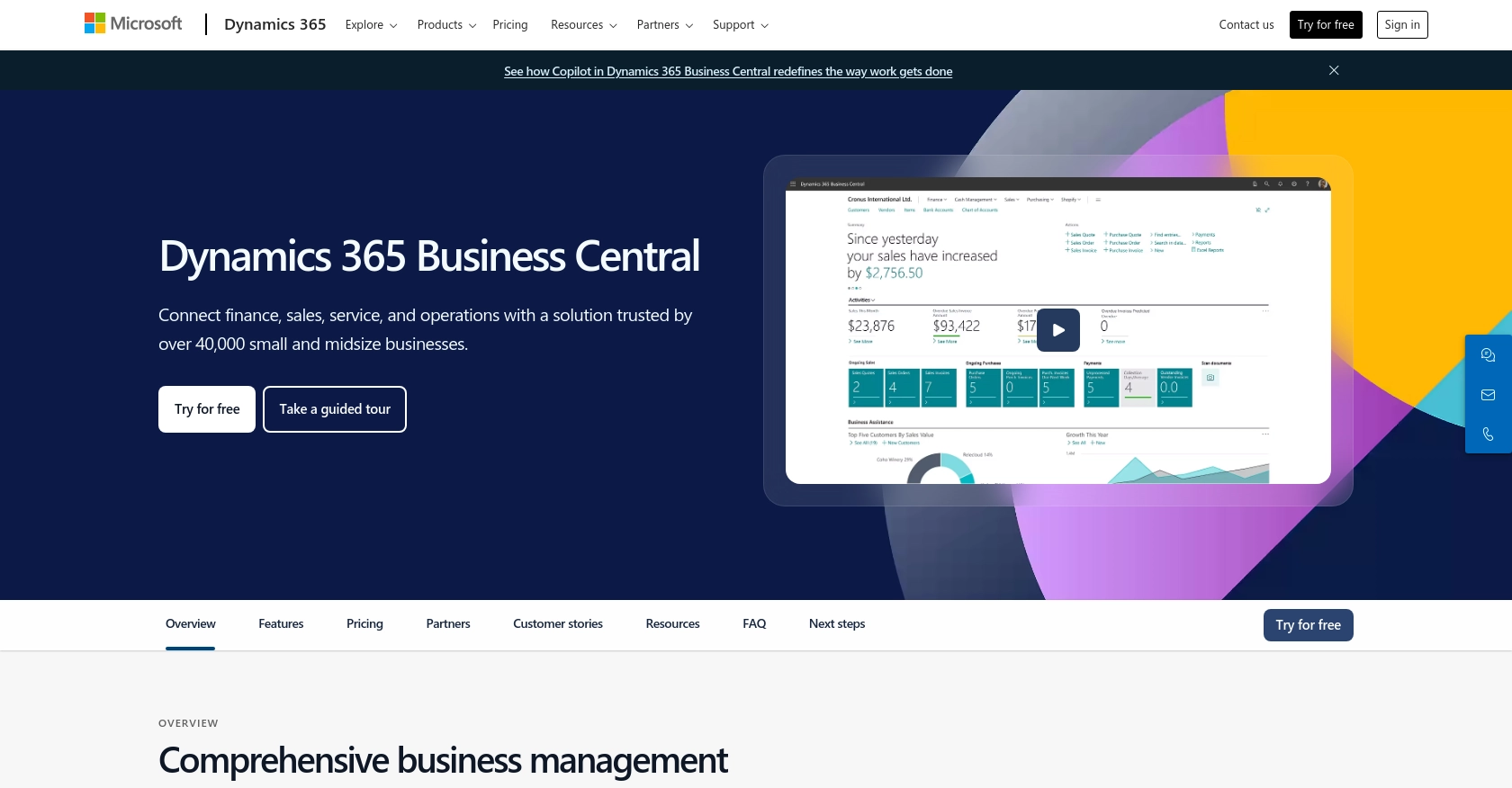
Introduction to Microsoft Dynamics 365 Business Central
Microsoft Dynamics 365 Business Central is a comprehensive business management solution designed for small to medium-sized businesses. It integrates various business processes, including finance, sales, service, and operations, into a single platform, enabling organizations to streamline their operations and make informed decisions.
Developers often seek to integrate with Microsoft Dynamics 365 Business Central to enhance business workflows and data management. By connecting with its API, developers can automate tasks such as creating or updating contacts, which is crucial for maintaining accurate customer data and improving customer relationship management.
For example, a developer might use the Microsoft Dynamics 365 Business Central API to automatically update contact information from an external CRM system, ensuring that all customer interactions are based on the most current data available.
Setting Up a Microsoft Dynamics 365 Business Central Test/Sandbox Account
Before you can start integrating with the Microsoft Dynamics 365 Business Central API, you need to set up a test or sandbox account. This environment allows you to safely experiment and test your API interactions without affecting live data.
Creating a Microsoft Dynamics 365 Business Central Sandbox Account
To begin, you need to create a sandbox account. Microsoft offers a free trial for Dynamics 365 Business Central, which you can use to access the sandbox environment:
- Visit the Microsoft Dynamics 365 Business Central website and sign up for a free trial.
- Follow the on-screen instructions to complete the registration process.
- Once registered, navigate to the Dynamics 365 Business Central dashboard.
- In the dashboard, select Sandbox Environments to create a new sandbox.
- Configure your sandbox environment as needed and launch it.
Registering an Application for OAuth Authentication
Microsoft Dynamics 365 Business Central uses OAuth 2.0 for authentication. To interact with the API, you need to register an application in Microsoft Entra ID:
- Go to the Azure Portal and sign in with your Microsoft account.
- Navigate to Azure Active Directory > App registrations.
- Click on New registration and fill in the required details, such as the application name and redirect URI.
- Once registered, note down the Application (client) ID and Directory (tenant) ID.
- Under Certificates & secrets, create a new client secret and save it securely.
Configuring API Permissions
To allow your application to access the Dynamics 365 Business Central API, you need to configure the necessary permissions:
- In the Azure Portal, go to your registered application.
- Select API permissions and click on Add a permission.
- Choose Dynamics 365 Business Central and select the permissions required for your integration, such as user_impersonation.
- Grant admin consent for the selected permissions.
With your sandbox account and application registration set up, you are now ready to start making API calls to Microsoft Dynamics 365 Business Central using OAuth authentication. For more detailed information on OAuth setup, refer to the Microsoft OAuth documentation.
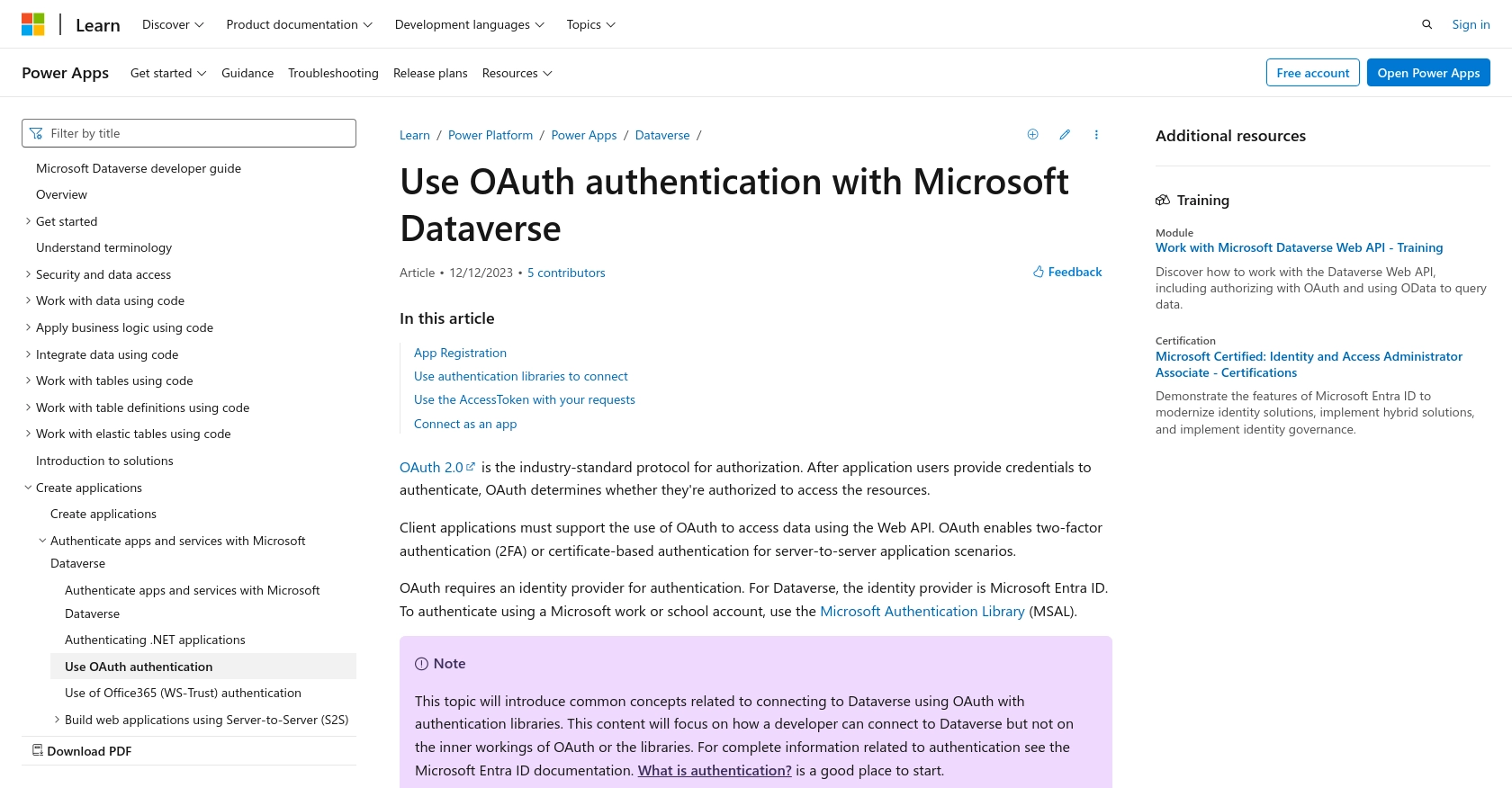
sbb-itb-96038d7
Making API Calls to Microsoft Dynamics 365 Business Central Using JavaScript
Setting Up Your JavaScript Environment for Microsoft Dynamics 365 Business Central API
Before making API calls to Microsoft Dynamics 365 Business Central, ensure that your JavaScript environment is properly set up. You'll need Node.js installed on your machine, as well as the axios
library to handle HTTP requests.
- Install Node.js from the official website.
- Open your terminal and run the following command to install
axios
:
npm install axios
Creating or Updating Contacts with Microsoft Dynamics 365 Business Central API
To create or update contacts, you'll use the POST method provided by the Microsoft Dynamics 365 Business Central API. This requires an access token obtained through OAuth authentication.
Sample JavaScript Code for Creating a Contact
Below is a sample code snippet demonstrating how to create a contact using the API:
const axios = require('axios');
async function createContact() {
const token = 'Your_Access_Token'; // Replace with your OAuth access token
const url = 'https://{businesscentralPrefix}/api/v2.0/companies({id})/contacts';
const contactData = {
id: '5d115c9c-44e3-ea11-bb43-000d3a2feca1',
number: '108001',
type: 'Company',
displayName: 'CRONUS USA, Inc.',
companyNumber: '17806',
companyName: 'CRONUS US',
businessRelation: 'Vendor',
addressLine1: '7122 South Ashford Street',
city: 'Atlanta',
state: 'GA',
country: 'US',
postalCode: '31772',
phoneNumber: '+1 425 555 0100',
email: 'ah@contoso.com'
};
try {
const response = await axios.post(url, contactData, {
headers: {
'Authorization': `Bearer ${token}`,
'Content-Type': 'application/json'
}
});
console.log('Contact Created:', response.data);
} catch (error) {
console.error('Error creating contact:', error.response ? error.response.data : error.message);
}
}
createContact();
Understanding the API Response
If the request is successful, the API will return a 201 Created status code along with the contact object in the response body. You can verify the creation by checking the contact details in your sandbox environment.
Handling Errors and Common Error Codes
When interacting with the API, you may encounter errors. Common HTTP status codes include:
- 400 Bad Request: The request was malformed or contains invalid data.
- 401 Unauthorized: The access token is missing or invalid.
- 404 Not Found: The specified resource could not be found.
- 500 Internal Server Error: An error occurred on the server side.
For more detailed error information, refer to the API documentation.
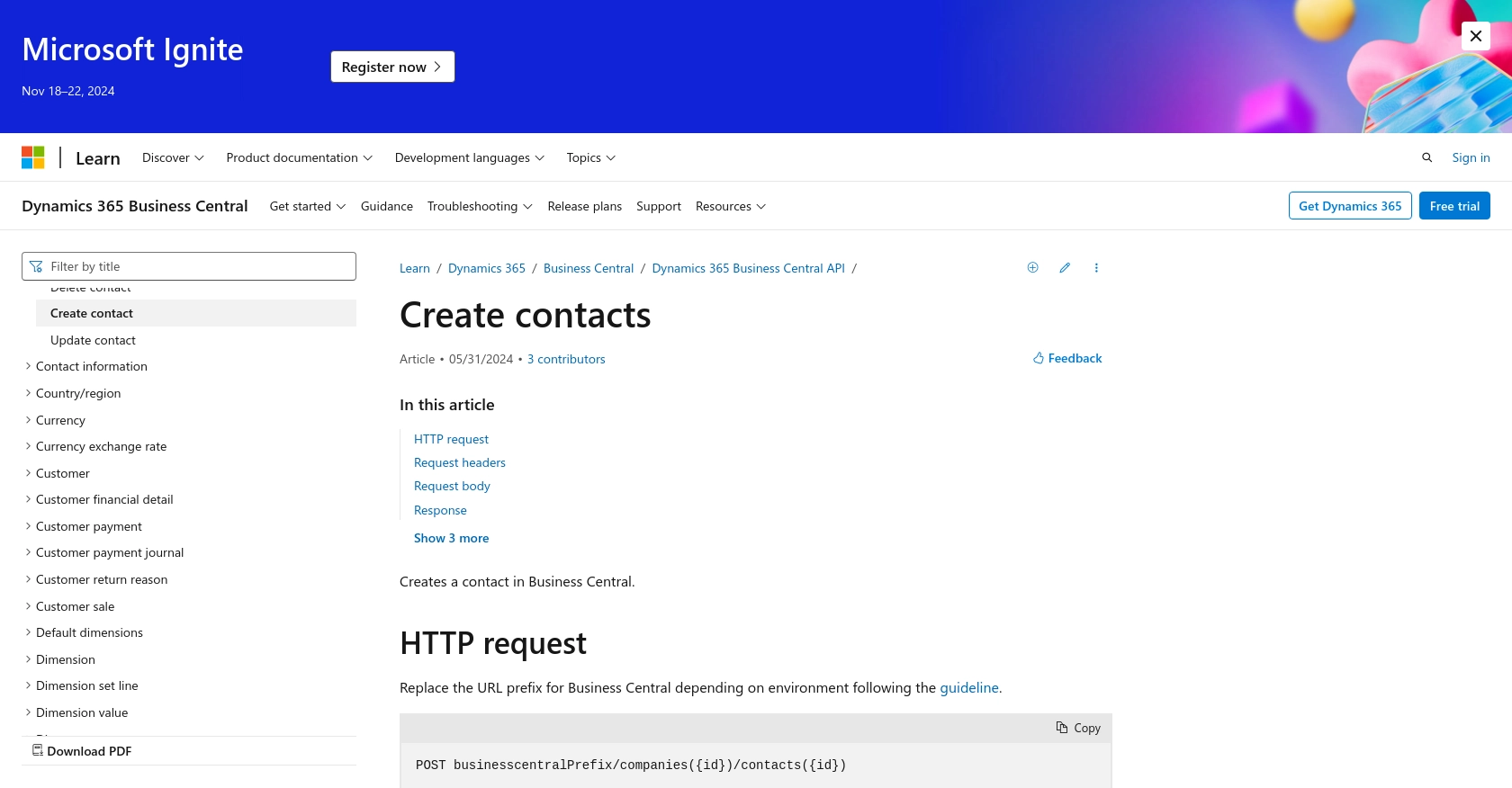
Conclusion and Best Practices for Integrating with Microsoft Dynamics 365 Business Central API
Integrating with the Microsoft Dynamics 365 Business Central API using JavaScript can significantly enhance your business processes by automating contact management and ensuring data accuracy across platforms. By following the steps outlined in this guide, you can efficiently create or update contacts, leveraging the robust capabilities of Dynamics 365 Business Central.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your OAuth tokens and client secrets securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limiting: Be aware of API rate limits and implement retry logic or exponential backoff strategies to handle throttling gracefully.
- Data Standardization: Ensure that data fields are standardized and validated before making API calls to maintain data integrity and prevent errors.
- Error Handling: Implement comprehensive error handling to manage different HTTP status codes and provide meaningful feedback to users or logs.
Streamlining Integrations with Endgrate
For developers looking to simplify their integration processes, Endgrate offers a unified API solution that connects to multiple platforms, including Microsoft Dynamics 365 Business Central. By using Endgrate, you can save time and resources, allowing you to focus on your core product development while providing an intuitive integration experience for your customers.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
- https://endgrate.com/provider/microsoftdynamics365businesscentral
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/authenticate-oauth
- https://learn.microsoft.com/en-us/graph/use-the-api
- https://learn.microsoft.com/en-us/dynamics365/business-central/dev-itpro/api-reference/v2.0/api/dynamics_contact_create
Ready to get started?