Using the LinkedIn API to Get Ad Form Leads in PHP
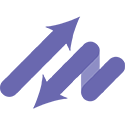
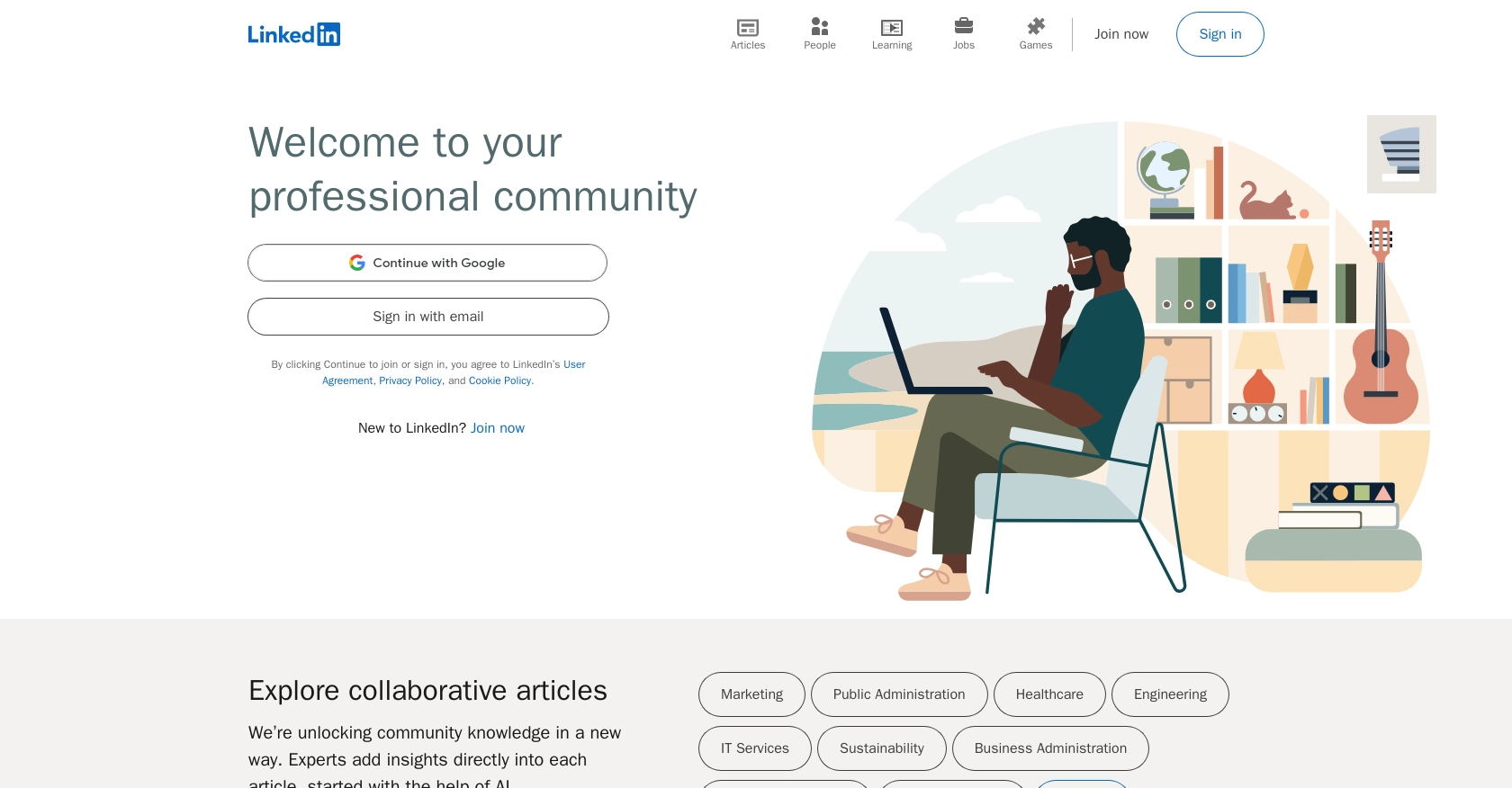
Introduction to LinkedIn API for Ad Form Leads
LinkedIn is a premier professional networking platform that offers a robust suite of tools for businesses to connect with professionals and potential clients. With its vast user base, LinkedIn provides unparalleled opportunities for B2B marketing and lead generation.
Integrating with LinkedIn's API allows developers to access and manage ad form leads, streamlining the process of capturing and utilizing potential customer information. For example, a developer might use the LinkedIn API to automatically retrieve ad form leads and sync them with a CRM system, enhancing the efficiency of marketing campaigns.
This article will guide you through using PHP to interact with the LinkedIn API, focusing on retrieving ad form leads. You'll learn how to set up your environment, authenticate with LinkedIn, and make API calls to access valuable lead data.
Setting Up Your LinkedIn Developer Account for API Access
Before you can start retrieving ad form leads using the LinkedIn API, you'll need to set up a LinkedIn Developer account. This account will allow you to create applications and access LinkedIn's API services.
Follow these steps to get started:
-
Create a LinkedIn Developer Account:
If you don't already have a LinkedIn account, sign up on the LinkedIn website. Once you have an account, visit the LinkedIn Developer Portal and log in with your LinkedIn credentials.
-
Create a New Application:
Navigate to the "My Apps" section and click on "Create App." Fill in the required details such as the app name, company, and description. This app will be used to access the LinkedIn API.
-
Configure OAuth 2.0 Authentication:
LinkedIn uses OAuth 2.0 for authentication. To set this up, go to the "Auth" tab in your app settings and note down the Client ID and Client Secret. You'll need these to authenticate your API requests.
-
Set Redirect URLs:
Under the "Auth" tab, specify the redirect URLs that LinkedIn will use to send authentication responses. Ensure these URLs are correctly configured to handle OAuth callbacks in your application.
-
Request API Permissions:
In the "Products" section, add the "Ads Lead Sync API" to your application. Complete the access form and submit it for review. LinkedIn will evaluate your application and grant access if it meets their criteria.
Once your application is approved, you'll be ready to authenticate and make API calls to retrieve ad form leads from LinkedIn.
For more detailed information, refer to the official LinkedIn documentation: LinkedIn Lead Generation and LinkedIn Lead Sync API Access Guide.
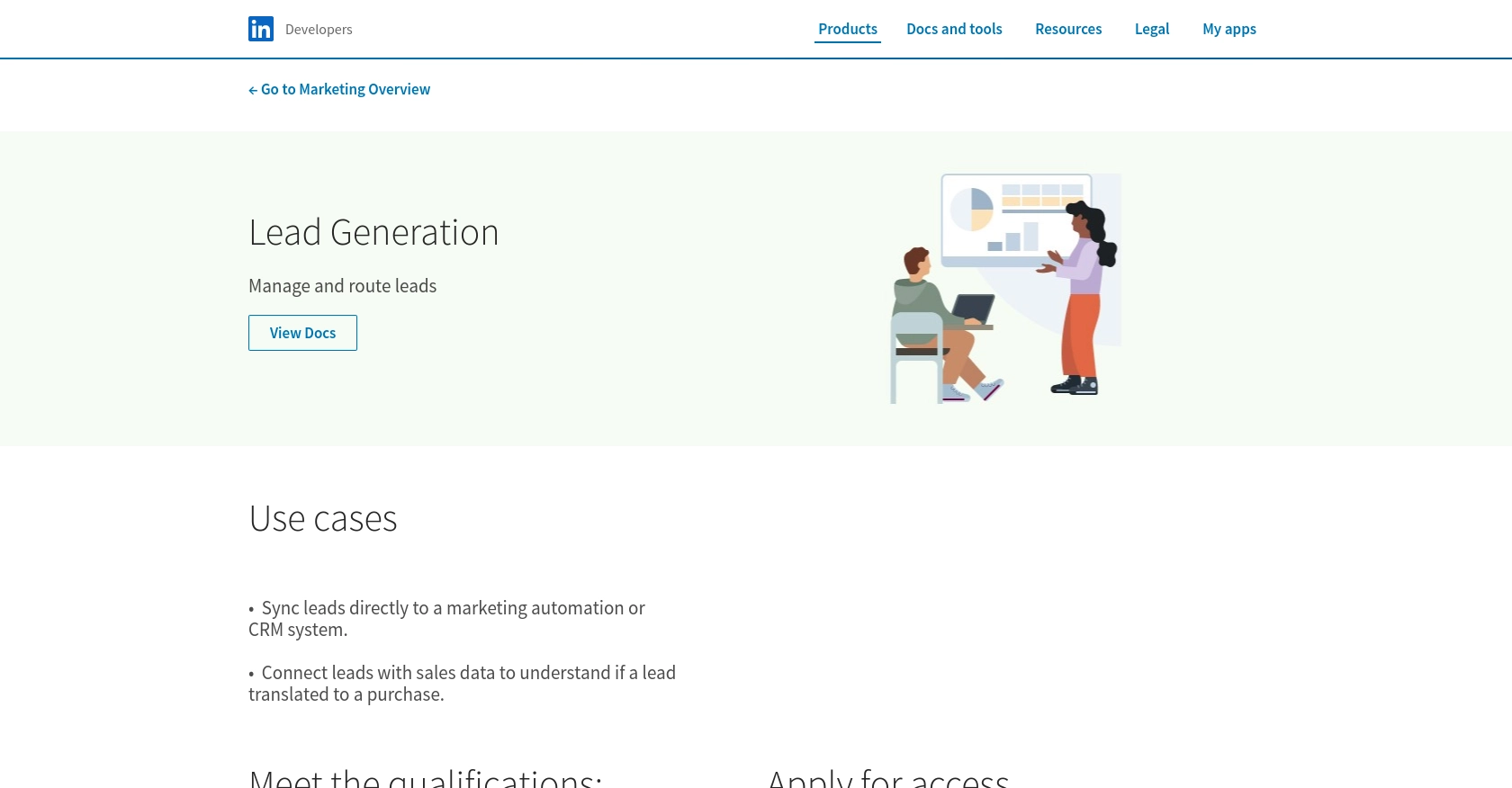
sbb-itb-96038d7
Making API Calls to Retrieve LinkedIn Ad Form Leads Using PHP
To interact with the LinkedIn API and retrieve ad form leads using PHP, you'll need to set up your environment and write code that handles authentication and API requests. This section will guide you through the necessary steps to achieve this.
Setting Up Your PHP Environment
Before making API calls, ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or higher
- Composer for managing dependencies
Next, install the Guzzle HTTP client, which will be used to make HTTP requests to the LinkedIn API:
composer require guzzlehttp/guzzle
Authenticating with LinkedIn API Using OAuth 2.0
LinkedIn uses OAuth 2.0 for authentication. You'll need to obtain an access token to make API requests. Here's a basic example of how to authenticate:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$response = $client->post('https://www.linkedin.com/oauth/v2/accessToken', [
'form_params' => [
'grant_type' => 'authorization_code',
'code' => 'YOUR_AUTHORIZATION_CODE',
'redirect_uri' => 'YOUR_REDIRECT_URI',
'client_id' => 'YOUR_CLIENT_ID',
'client_secret' => 'YOUR_CLIENT_SECRET',
],
]);
$accessToken = json_decode($response->getBody(), true)['access_token'];
Replace YOUR_AUTHORIZATION_CODE
, YOUR_REDIRECT_URI
, YOUR_CLIENT_ID
, and YOUR_CLIENT_SECRET
with your actual LinkedIn app credentials.
Retrieving LinkedIn Ad Form Leads
Once authenticated, you can make API calls to retrieve ad form leads. Here's how to fetch lead data:
$response = $client->get('https://api.linkedin.com/rest/leadFormResponses', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'LinkedIn-Version' => '202307',
'Content-Type' => 'application/json',
],
'query' => [
'owner' => 'urn:li:organization:YOUR_ORGANIZATION_URN',
'leadType' => 'SPONSORED',
'q' => 'owner',
],
]);
$leads = json_decode($response->getBody(), true);
print_r($leads);
Replace YOUR_ORGANIZATION_URN
with your organization's URN. This code retrieves leads associated with your LinkedIn ad forms.
Handling API Responses and Errors
After making an API call, it's crucial to handle responses and potential errors. Check the response status code to ensure the request was successful:
if ($response->getStatusCode() === 200) {
echo "Leads retrieved successfully!";
} else {
echo "Failed to retrieve leads. Status code: " . $response->getStatusCode();
}
For more detailed error handling, refer to the LinkedIn API documentation: LinkedIn Lead Sync API Documentation.
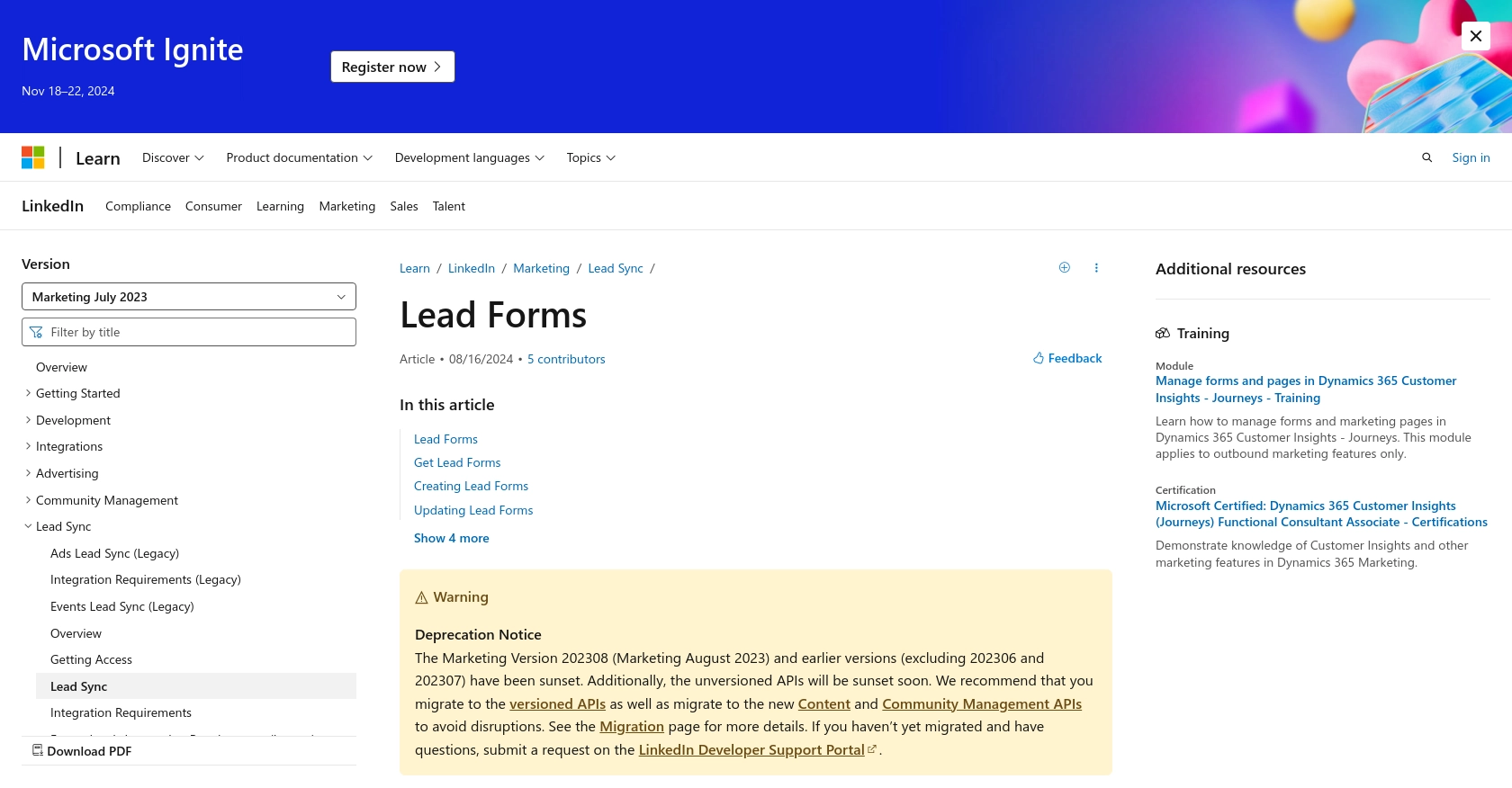
Conclusion and Best Practices for Using LinkedIn API with PHP
Integrating with LinkedIn's API to retrieve ad form leads using PHP can significantly enhance your marketing and lead management processes. By automating the retrieval and synchronization of leads, businesses can streamline their operations and focus on converting leads into customers.
Best Practices for Secure and Efficient LinkedIn API Integration
- Securely Store Credentials: Always store your LinkedIn API credentials, such as the client ID and client secret, securely. Use environment variables or a secure vault to prevent unauthorized access.
- Handle Rate Limiting: LinkedIn's API may have rate limits. Implement logic to handle rate limit errors gracefully and retry requests after the appropriate wait time.
- Standardize Data Fields: Ensure that the data retrieved from LinkedIn is transformed and standardized before integrating it into your CRM or marketing systems. This helps maintain data consistency across platforms.
- Monitor API Changes: Keep an eye on LinkedIn's API documentation for any updates or changes. This ensures your integration remains functional and up-to-date.
By following these best practices, you can create a robust and secure integration with LinkedIn's API, maximizing the value of your lead generation efforts.
Streamline Your Integrations with Endgrate
If managing multiple integrations is becoming a challenge, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers. Visit Endgrate to learn more about how it can simplify your integration needs.
Read More
- https://endgrate.com/provider/linkedin
- https://developer.linkedin.com/product-catalog/marketing/lead-generation
- https://business.linkedin.com/content/dam/me/business/en-us/marketing-solutions/cx/2022/pdf/linkedin-lead-sync-api-access-guide.pdf
- https://learn.microsoft.com/en-us/linkedin/marketing/lead-sync/leadsync?view=li-lms-2023-07&tabs=http
Ready to get started?