Using the Capsule API to Get Parties (with Javascript examples)
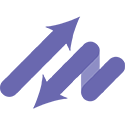
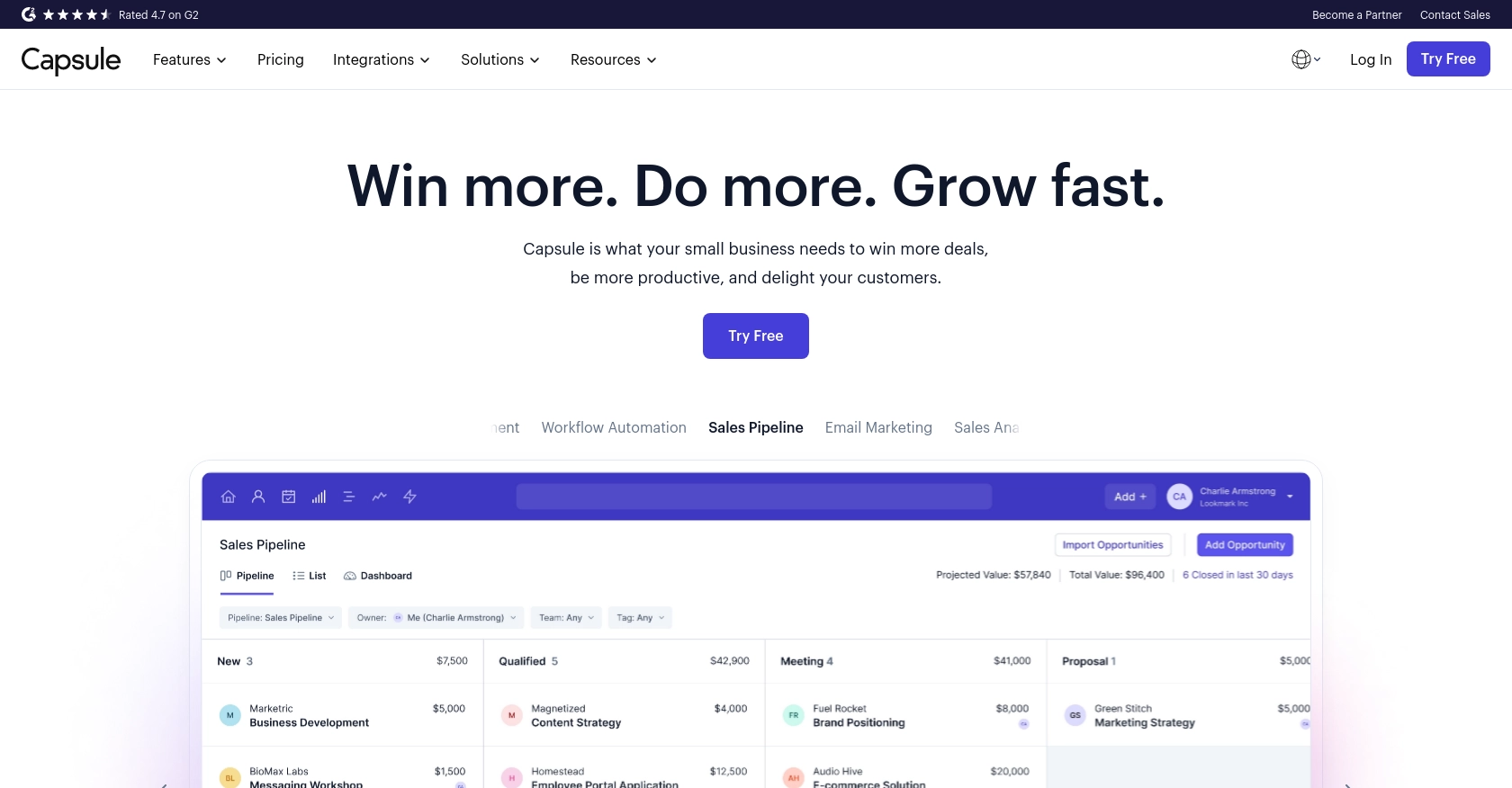
Introduction to Capsule CRM
Capsule CRM is a versatile customer relationship management platform designed to help businesses manage their contacts, sales opportunities, and customer interactions efficiently. With its user-friendly interface and robust features, Capsule CRM is a popular choice for businesses looking to enhance their customer management processes.
Developers may want to integrate with Capsule CRM to access and manage contact data, known as "parties" in Capsule terminology. This integration can streamline processes such as syncing customer information across platforms or automating communication workflows. For example, a developer might use the Capsule API to retrieve a list of contacts and update their details in a marketing automation tool, ensuring consistent and up-to-date information across systems.
Setting Up Your Capsule CRM Test Account
Before you can start integrating with the Capsule API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with the API without affecting live data. Capsule CRM offers a straightforward process to get started with their API using OAuth 2.0 authentication.
Creating a Capsule CRM Account
If you don't already have a Capsule CRM account, you can sign up for a free trial on their website. This will give you access to all the features you need to test the API integration.
- Visit the Capsule CRM signup page.
- Follow the instructions to create your account.
- Once your account is set up, log in to access the dashboard.
Registering Your Application for OAuth 2.0
To interact with the Capsule API, you'll need to register your application to obtain the necessary credentials for OAuth 2.0 authentication.
- Navigate to the "My Preferences" section in your Capsule account.
- Select "API Authentication Tokens" to create a new application.
- Fill in the required details, such as the application name and redirect URI.
- Once registered, you'll receive a client ID and client secret. Keep these credentials secure as they are essential for authentication.
Generating an OAuth 2.0 Bearer Token
With your application registered, you can now generate a bearer token to authenticate API requests.
- Redirect users to the Capsule authorization URL to obtain an authorization code:
- After the user authorizes your application, Capsule will redirect to your specified URI with a code parameter.
- Exchange this code for an access token by making a POST request:
- Store the access token securely, as it will be used in the Authorization header for API requests.
GET https://api.capsulecrm.com/oauth/authorise?response_type=code&client_id=YOUR_CLIENT_ID&redirect_uri=YOUR_REDIRECT_URI&scope=read%20write
POST https://api.capsulecrm.com/oauth/token
{
"code": "AUTHORIZATION_CODE",
"client_id": "YOUR_CLIENT_ID",
"client_secret": "YOUR_CLIENT_SECRET",
"grant_type": "authorization_code"
}
For more detailed information on authentication, refer to the Capsule API authentication documentation.
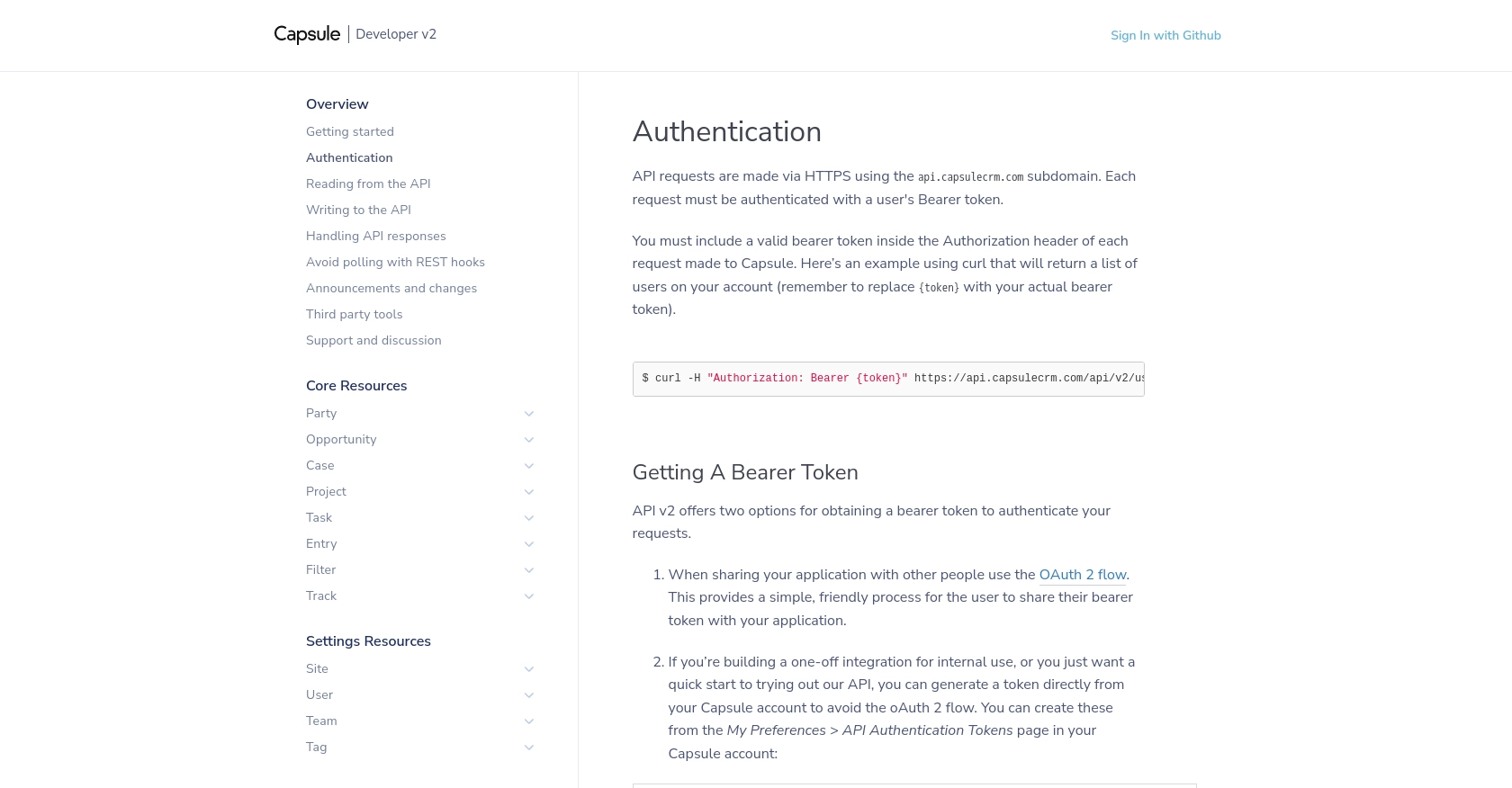
sbb-itb-96038d7
Making API Calls to Retrieve Parties from Capsule Using JavaScript
To interact with the Capsule API and retrieve party data, you'll need to make HTTP requests using JavaScript. This section will guide you through the process of setting up your environment, writing the code, and handling responses effectively.
Setting Up Your JavaScript Environment for Capsule API Integration
Before making API calls, ensure you have a modern JavaScript environment set up. You'll need Node.js and npm (Node Package Manager) installed on your machine. These tools will allow you to manage dependencies and run your JavaScript code.
- Download and install Node.js from the official website.
- Verify the installation by running
node -v
andnpm -v
in your terminal. - Create a new project directory and navigate into it:
mkdir capsule-api-integration
cd capsule-api-integration
npm init -y
npm install axios
Writing JavaScript Code to Fetch Parties from Capsule CRM
With your environment ready, you can now write the JavaScript code to make API calls to Capsule CRM and retrieve party data.
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.capsulecrm.com/api/v2/parties';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json'
};
// Function to fetch parties
async function fetchParties() {
try {
const response = await axios.get(endpoint, { headers });
const parties = response.data.parties;
console.log('Retrieved Parties:', parties);
} catch (error) {
console.error('Error fetching parties:', error.response.data);
}
}
// Call the function to fetch parties
fetchParties();
Replace YOUR_ACCESS_TOKEN
with the access token you obtained during the OAuth 2.0 authentication process. This token is crucial for authorizing your API requests.
Handling API Responses and Errors from Capsule CRM
When making API calls, it's essential to handle responses and potential errors effectively. The Capsule API will return a status code of 200 for successful requests. If an error occurs, you'll receive a different status code, such as 401 for unauthorized access or 429 for rate limit exceeded.
- Check the response status code to determine if the request was successful.
- Log any errors to understand what went wrong and how to address it.
- Refer to the Capsule API response handling documentation for more details on error codes and their meanings.
By following these steps, you can efficiently retrieve party data from Capsule CRM using JavaScript, enabling seamless integration with your applications.
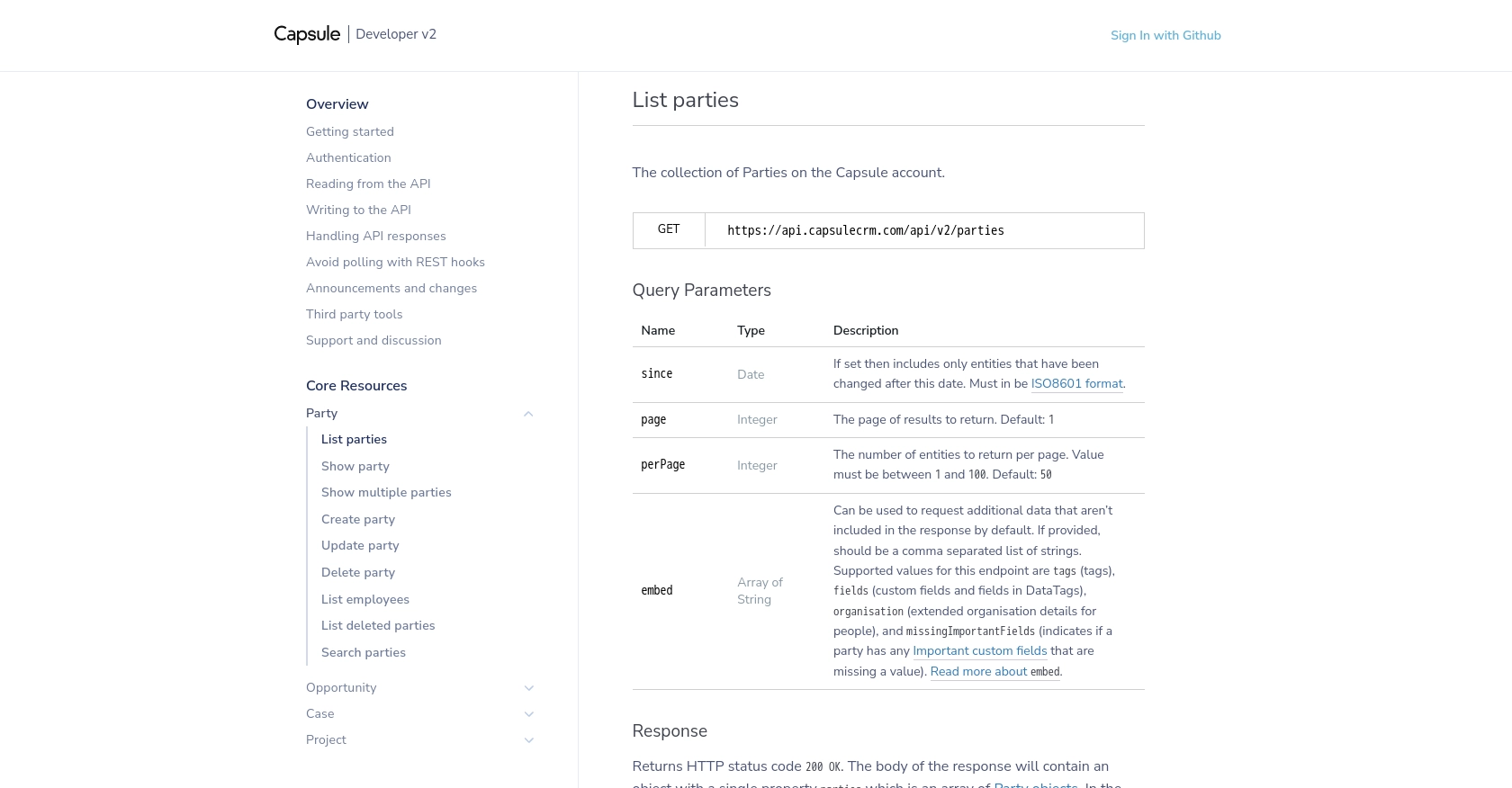
Conclusion and Best Practices for Capsule API Integration
Integrating with the Capsule API using JavaScript allows developers to efficiently manage and synchronize contact data across various platforms. By following the steps outlined in this guide, you can retrieve and handle party data seamlessly, enhancing your application's functionality and user experience.
Best Practices for Secure and Efficient Capsule API Usage
- Securely Store Credentials: Always store your OAuth 2.0 credentials, such as client ID, client secret, and access tokens, securely. Avoid hardcoding them in your source code.
- Handle Rate Limiting: Capsule API enforces a rate limit of 4,000 requests per hour. Monitor the
X-RateLimit-Remaining
header and implement backoff strategies to avoid exceeding this limit. For more details, refer to the Capsule API rate limit documentation. - Implement Error Handling: Ensure robust error handling by checking response status codes and logging errors for troubleshooting. This will help you quickly identify and resolve issues.
- Optimize Data Requests: Use query parameters like
since
andembed
to optimize data retrieval and reduce unnecessary API calls.
Enhancing Your Integration with Endgrate
For developers looking to streamline multiple integrations, Endgrate offers a unified API solution that simplifies the process. By using Endgrate, you can focus on your core product while outsourcing complex integrations, saving time and resources. Explore how Endgrate can enhance your integration experience by visiting Endgrate's website.
By adhering to these best practices and leveraging tools like Endgrate, you can ensure a secure, efficient, and scalable integration with Capsule CRM, providing a seamless experience for your users.
Read More
- https://endgrate.com/provider/capsulecrm
- https://developer.capsulecrm.com/v2/overview/authentication
- https://developer.capsulecrm.com/v2/overview/handling-api-responses
- https://developer.capsulecrm.com/v2/overview/avoid-polling-with-rest-hooks
- https://developer.capsulecrm.com/v2/operations/Party#listParties
Ready to get started?