Using the Todoist API to Create Tasks (with PHP examples)
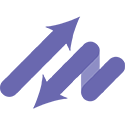
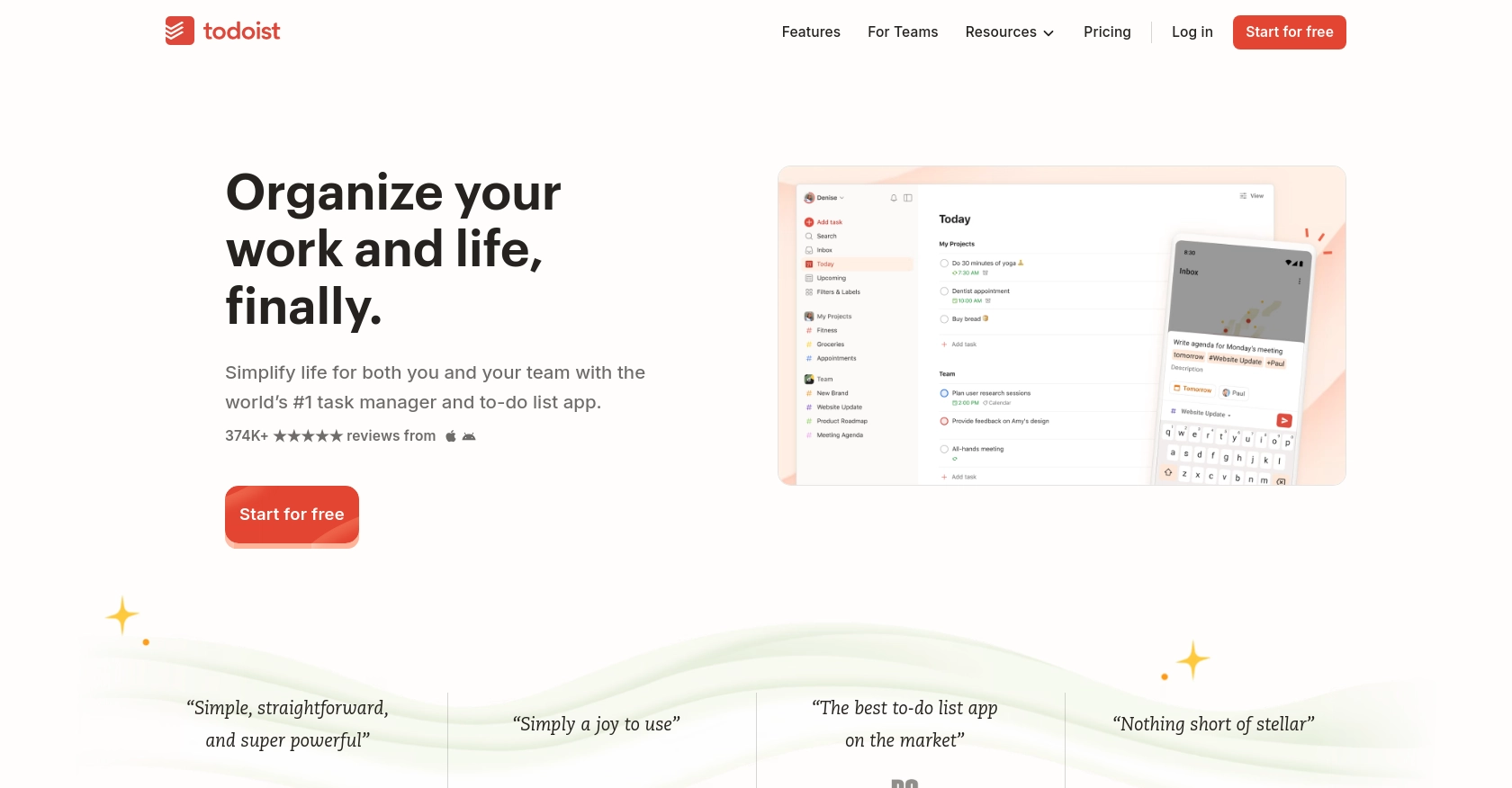
Introduction to Todoist API for Task Management
Todoist is a powerful task management platform that helps individuals and teams organize, plan, and collaborate on tasks efficiently. With its intuitive interface and robust features, Todoist is a popular choice for managing personal and professional projects.
Integrating with the Todoist API allows developers to automate and enhance task management workflows. For example, you can create tasks directly from an external application, enabling seamless task tracking and management. This integration can be particularly useful for developers looking to streamline project management processes by automatically generating tasks based on specific triggers or events.
Setting Up Your Todoist Developer Account for API Integration
Before you can start integrating with the Todoist API, you'll need to set up a developer account and obtain the necessary credentials. This process involves creating a Todoist app and generating an OAuth token for authentication.
Creating a Todoist Developer Account
If you don't already have a Todoist account, you can sign up for a free account on the Todoist website. Once your account is created, log in to access the developer portal.
Registering Your Application in Todoist
To interact with the Todoist API, you need to register your application:
- Navigate to the Todoist Developer Console.
- Click on "Create New App" and fill in the required details such as the app name and description.
- Set the redirect URI, which is the URL to which users will be redirected after they authorize your app.
- Once your app is created, you will receive a client ID and client secret. Keep these credentials secure as they are essential for OAuth authentication.
Generating an OAuth Token for Todoist API Access
Todoist uses OAuth 2.0 for authentication. Follow these steps to generate an OAuth token:
- Direct users to the Todoist authorization URL with your client ID and redirect URI.
- After the user authorizes your app, they will be redirected to your specified redirect URI with an authorization code.
- Exchange this authorization code for an access token by making a POST request to the Todoist token endpoint. Include your client ID, client secret, and the authorization code in the request.
// Example PHP code to exchange authorization code for access token
$client_id = 'your_client_id';
$client_secret = 'your_client_secret';
$code = 'authorization_code_received';
$redirect_uri = 'your_redirect_uri';
$response = file_get_contents('https://todoist.com/oauth/access_token', false, stream_context_create([
'http' => [
'method' => 'POST',
'header' => 'Content-Type: application/x-www-form-urlencoded',
'content' => http_build_query([
'client_id' => $client_id,
'client_secret' => $client_secret,
'code' => $code,
'redirect_uri' => $redirect_uri
])
]
]));
$access_token = json_decode($response)->access_token;
Store the access token securely as it will be used to authenticate API requests on behalf of the user.
For more detailed information on OAuth authentication, refer to the Todoist API documentation.
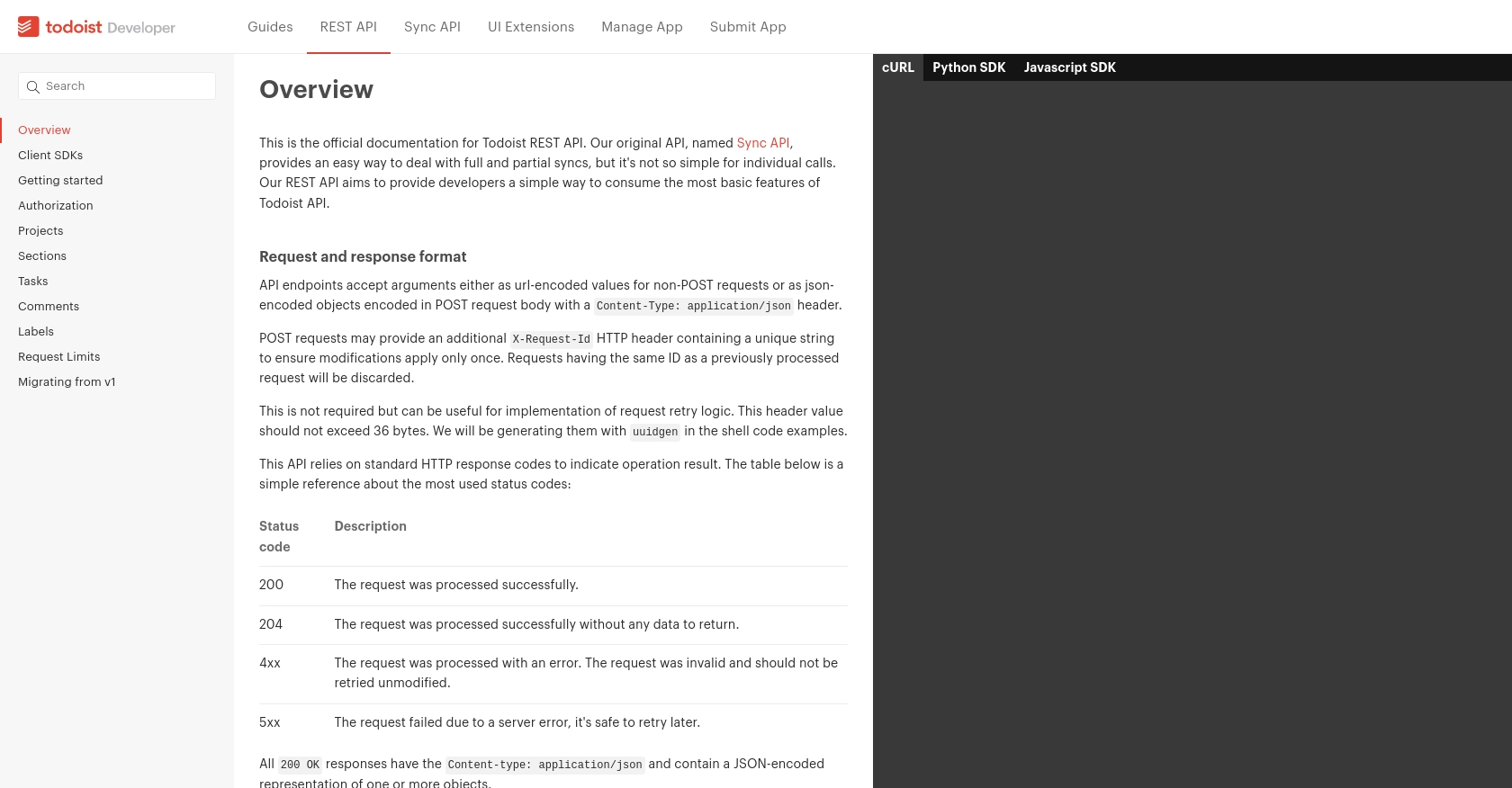
sbb-itb-96038d7
Making API Calls to Todoist for Task Creation Using PHP
Once you have your OAuth token, you can start making API calls to Todoist to create tasks. This section will guide you through the process of setting up your PHP environment and executing the API call to create a new task in Todoist.
Setting Up PHP Environment for Todoist API Integration
Before making API calls, ensure you have PHP installed on your system. You will also need the cURL
extension enabled to handle HTTP requests. You can check your PHP version and installed extensions using the following command:
php -v
php -m | grep curl
If cURL
is not enabled, you can enable it by modifying your php.ini
file or installing it via your package manager.
Creating a Task in Todoist Using PHP
With your environment set up, you can now create a task in Todoist using the API. The following PHP code demonstrates how to make a POST request to the Todoist API to create a new task:
// Set the API endpoint and access token
$api_url = 'https://api.todoist.com/rest/v2/tasks';
$access_token = 'your_access_token';
// Define the task data
$task_data = [
'content' => 'Complete the project documentation',
'due_string' => 'tomorrow at 5pm',
'priority' => 4
];
// Initialize cURL session
$ch = curl_init($api_url);
// Set cURL options
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Content-Type: application/json',
'Authorization: Bearer ' . $access_token
]);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($task_data));
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the cURL request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Request Error:' . curl_error($ch);
} else {
$response_data = json_decode($response, true);
echo 'Task Created Successfully: ' . $response_data['id'];
}
// Close the cURL session
curl_close($ch);
Replace your_access_token
with the OAuth token you obtained earlier. The $task_data
array contains the task details, such as content, due date, and priority.
Verifying Task Creation in Todoist
After executing the API call, you can verify the task creation by checking your Todoist account. The new task should appear in your task list with the specified details.
Handling Errors and API Response Codes
Todoist API uses standard HTTP response codes to indicate the result of an API call. Here are some common response codes you might encounter:
- 200 OK: The request was successful, and the task was created.
- 204 No Content: The request was successful, but there is no content to return.
- 4xx Client Error: There was an error with the request. Check the request parameters and try again.
- 5xx Server Error: There was a server error. You can retry the request later.
For more information on handling errors, refer to the Todoist API documentation.
Conclusion and Best Practices for Todoist API Integration
Integrating with the Todoist API using PHP provides a powerful way to automate and enhance task management workflows. By following the steps outlined in this guide, you can create tasks programmatically, allowing for seamless integration with other applications and systems.
Best Practices for Secure and Efficient Todoist API Usage
- Secure Storage of OAuth Tokens: Ensure that OAuth tokens are stored securely, using encryption and access controls to prevent unauthorized access.
- Handle Rate Limiting: The Todoist API allows a maximum of 1000 requests per user within a 15-minute period. Implement logic to handle rate limiting gracefully, such as retrying requests after a delay.
- Data Standardization: Consider standardizing task data fields to ensure consistency across different integrations and applications.
- Error Handling: Implement robust error handling to manage API response codes effectively, ensuring that your application can recover from errors smoothly.
Streamlining Integrations with Endgrate
For developers looking to simplify the integration process across multiple platforms, consider using Endgrate. Endgrate offers a unified API endpoint that connects to various platforms, including Todoist, allowing you to build integrations once and deploy them across different services. This approach saves time and resources, enabling you to focus on your core product development.
Explore how Endgrate can enhance your integration experience by visiting Endgrate's website and discover the benefits of a streamlined, efficient integration process.
Read More
Ready to get started?