Using the Sage 100 API to Get Customers in Javascript
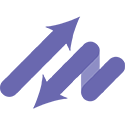
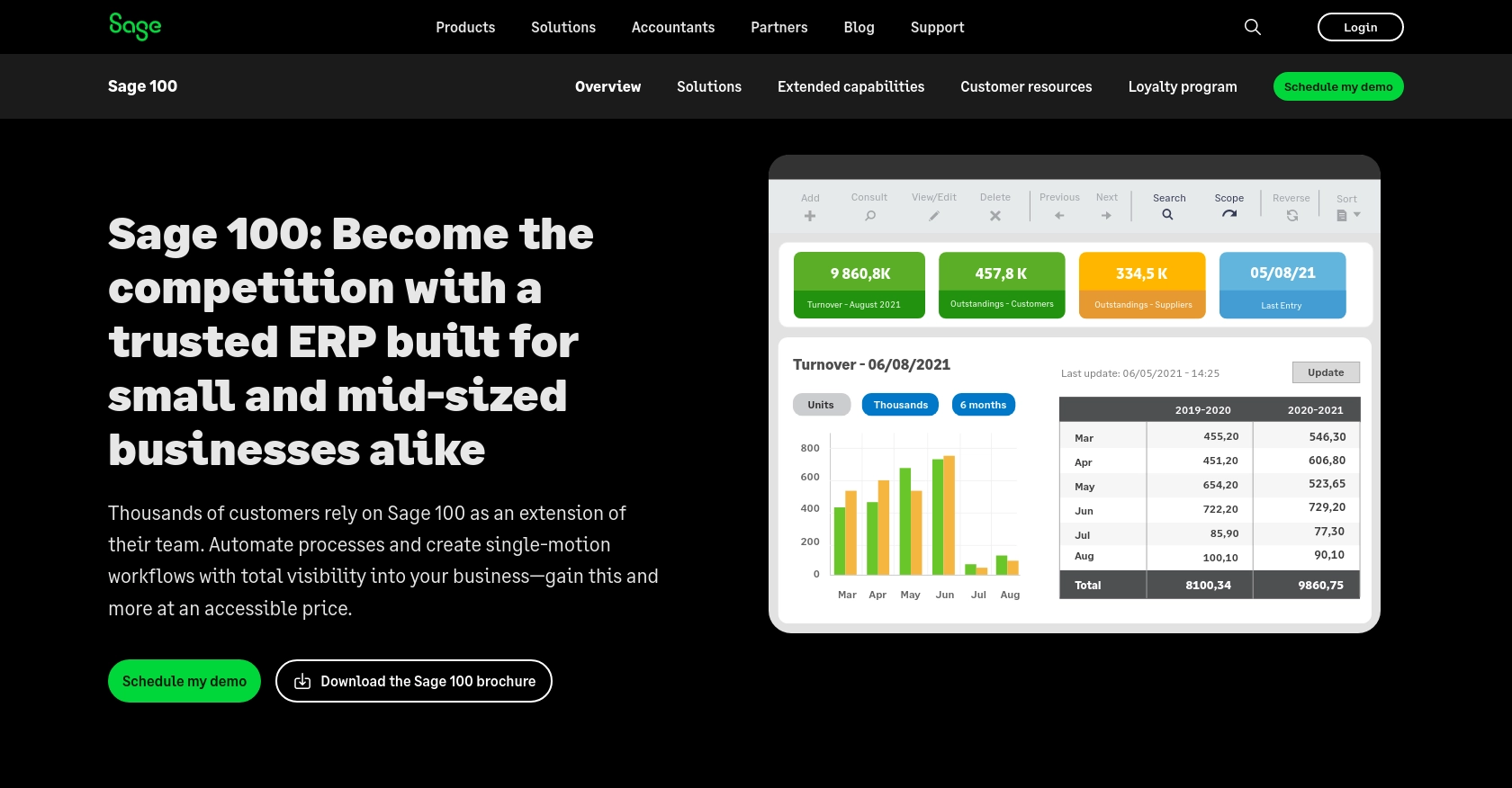
Introduction to Sage 100 API
Sage 100 is a comprehensive ERP solution designed to manage accounting, inventory, and business operations for small to medium-sized enterprises. Its robust features and flexibility make it a popular choice for businesses looking to streamline their processes and improve efficiency.
Integrating with the Sage 100 API allows developers to access and manipulate various data points within the system, such as customer information. For example, a developer might use the Sage 100 API to retrieve customer data and integrate it with a CRM system, enabling seamless data synchronization and enhanced customer relationship management.
Setting Up a Sage 100 Test or Sandbox Account for API Integration
Before you can start interacting with the Sage 100 API, you'll need to set up a test or sandbox account. This environment allows developers to safely experiment with API calls without affecting live data. Follow these steps to get started:
Install and Configure the Sage 100 ODBC Driver
To connect with the Sage 100 API, ensure that the Sage 100 ODBC driver is installed and properly configured on your system. This involves setting up a Data Source Name (DSN) that connects to the Sage 100 ERP system.
- Access the ODBC Data Source Administrator on your system.
- Create a new DSN using the Sage 100 ODBC driver.
- Enter the correct server, database, and authentication settings to establish the connection.
For detailed instructions, refer to the Sage 100 ODBC Driver Configuration Guide.
Create a Sage 100 Sandbox Environment
If you don't already have a Sage 100 sandbox environment, you may need to contact Sage support or your system administrator to set one up. This environment will mimic your production setup, allowing you to test API interactions safely.
Configure Authentication for Sage 100 API Access
Sage 100 uses a custom authentication method. Ensure that your credentials are correctly configured in the DSN setup. This typically involves:
- Setting up the client/server ODBC driver to run as an application or service.
- Configuring server-side settings to allow remote access.
- Testing the connection to ensure successful setup.
Refer to the official documentation for more details on configuring authentication.
Verify Your Setup
Once your sandbox environment and ODBC driver are configured, verify the setup by testing the connection:
- Open the ODBC Data Source Administrator.
- Select your DSN and click "Test Connection."
- Ensure the connection is successful before proceeding with API calls.
With your Sage 100 test environment ready, you can now proceed to make API calls using JavaScript to retrieve customer data.
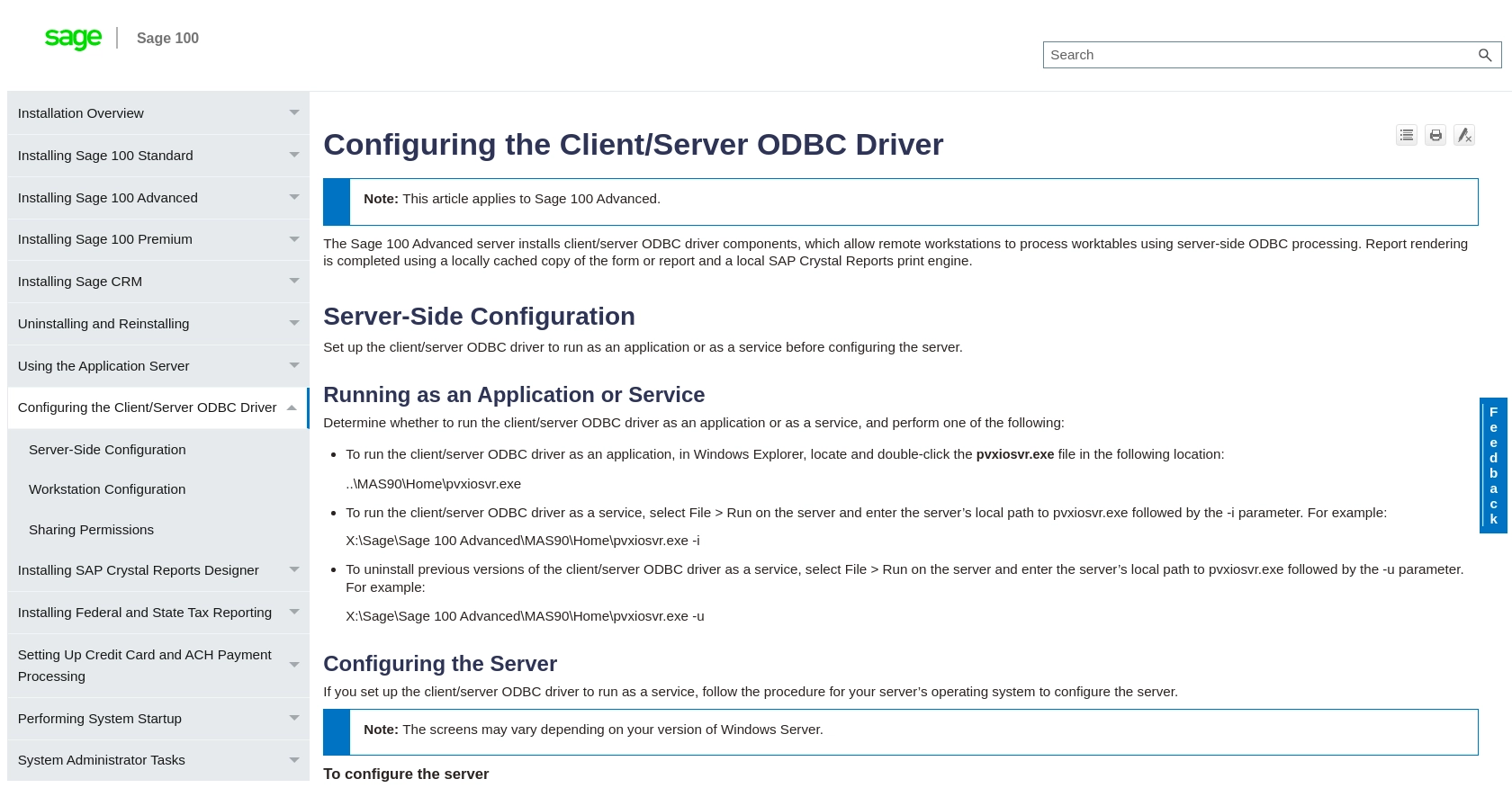
sbb-itb-96038d7
Making API Calls to Retrieve Customer Data from Sage 100 Using JavaScript
To interact with the Sage 100 API and retrieve customer data using JavaScript, you'll need to ensure you have the necessary setup and dependencies. This section will guide you through the process of making API calls, handling responses, and managing potential errors.
Setting Up Your JavaScript Environment for Sage 100 API Integration
Before making API calls, ensure you have a working JavaScript environment. You can use Node.js for server-side JavaScript execution. Install Node.js from the official website if you haven't already.
Next, you'll need to install the odbc
package to interact with the Sage 100 ODBC driver:
npm install odbc
Writing JavaScript Code to Connect and Retrieve Customers from Sage 100
Create a new JavaScript file, get_customers.js
, and add the following code to connect to Sage 100 and retrieve customer data:
const odbc = require('odbc');
async function getCustomers() {
try {
// Establish a connection using the DSN configured for Sage 100
const connection = await odbc.connect('DSN=Your_Sage100_DSN');
// Execute a query to retrieve customer data
const result = await connection.query('SELECT * FROM AR_Customer');
// Log the customer data
console.log(result);
// Close the connection
await connection.close();
} catch (error) {
console.error('Error retrieving customers:', error);
}
}
getCustomers();
Replace Your_Sage100_DSN
with the name of your configured Data Source Name (DSN) for Sage 100.
Running the JavaScript Code and Verifying Output
Run the JavaScript file using Node.js to execute the API call:
node get_customers.js
If the connection is successful, you should see the customer data output in your console. This data will include all the customer records retrieved from the Sage 100 system.
Handling Errors and Debugging Sage 100 API Calls
When making API calls, it's crucial to handle potential errors gracefully. The code above uses a try-catch
block to catch and log any errors that occur during the connection or query execution.
Common issues might include incorrect DSN configuration, network problems, or SQL query errors. Ensure your DSN is correctly set up and that your SQL queries are valid according to the Sage 100 database schema.
For more detailed information on the Sage 100 API and its capabilities, refer to the official Sage 100 API documentation.
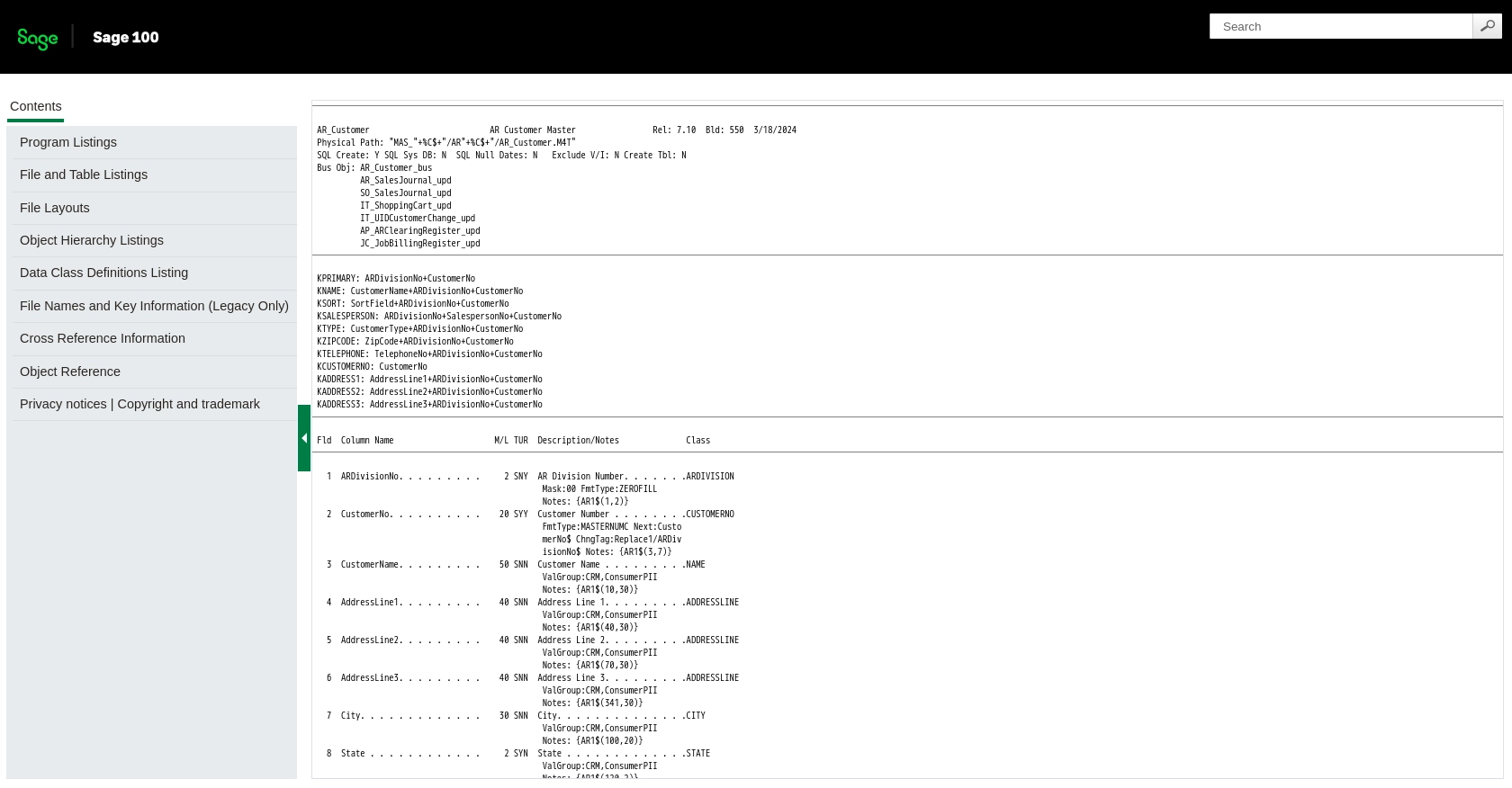
Conclusion and Best Practices for Sage 100 API Integration Using JavaScript
Integrating with the Sage 100 API using JavaScript provides a powerful way to access and manage customer data, enhancing your business operations and customer relationship management. By following the steps outlined in this guide, you can efficiently set up your environment, make API calls, and handle any potential errors.
Best Practices for Secure and Efficient Sage 100 API Usage
- Secure Credentials: Always store your DSN and authentication credentials securely. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the Sage 100 API. Implement retry logic and exponential backoff strategies to manage requests efficiently.
- Data Standardization: Ensure that the data retrieved from Sage 100 is standardized and transformed as needed to integrate seamlessly with other systems.
- Error Handling: Implement robust error handling to manage connection issues, query errors, and other potential problems gracefully.
Streamlining Integrations with Endgrate
While integrating with Sage 100 directly can be effective, managing multiple integrations across different platforms can be complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product development.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Explore how Endgrate can help you save time and resources by visiting Endgrate today.
Read More
Ready to get started?