How to Create or Update Purchase Orders with the Zoho Books API in Javascript
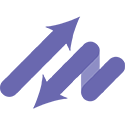
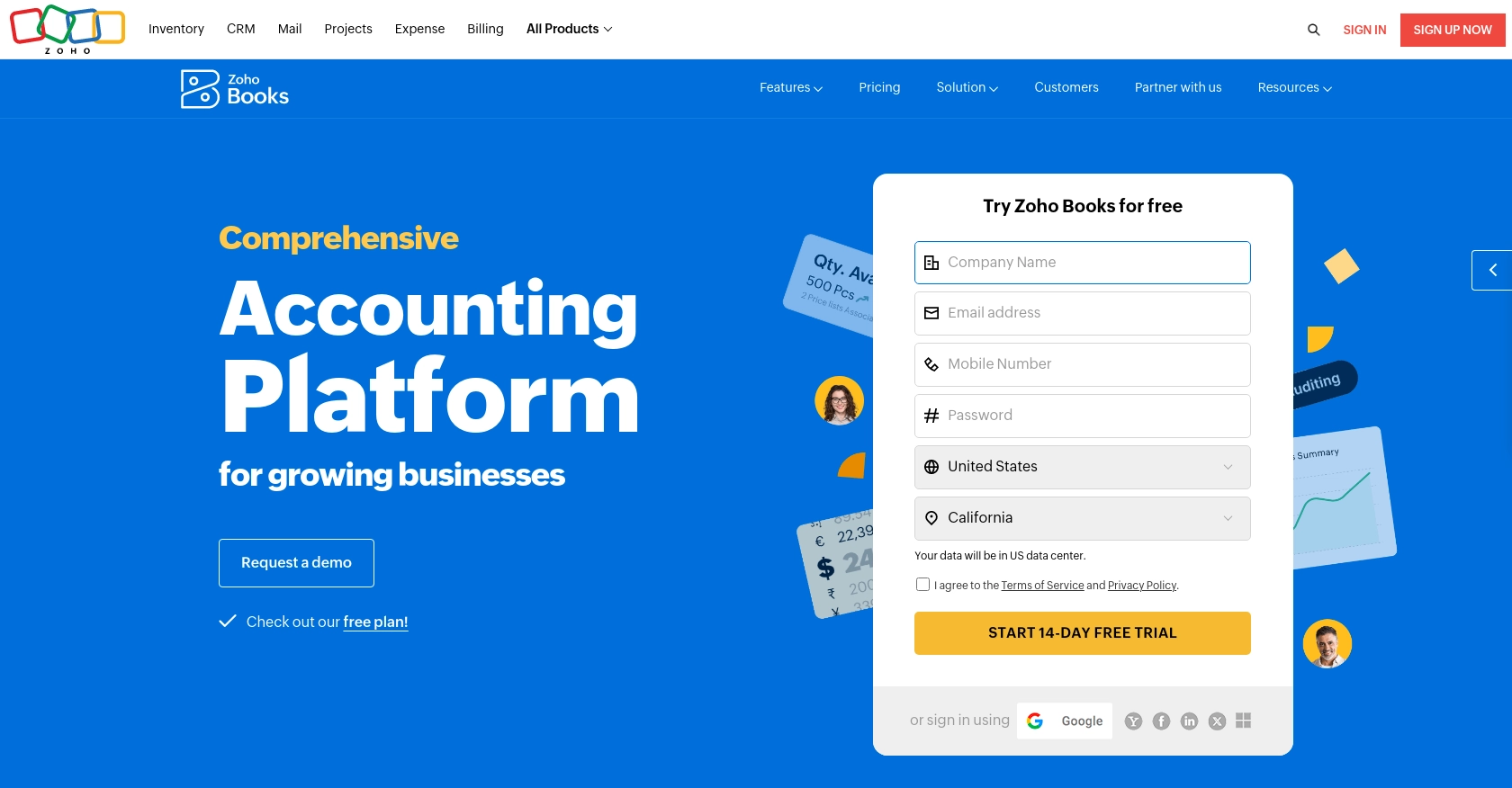
Introduction to Zoho Books API for Purchase Orders
Zoho Books is a comprehensive cloud-based accounting software designed to streamline financial operations for businesses of all sizes. It offers a wide range of features, including invoicing, expense tracking, and inventory management, making it a popular choice for companies looking to automate their accounting processes.
Integrating with the Zoho Books API allows developers to automate and manage purchase orders efficiently. By leveraging this API, you can create or update purchase orders directly from your application, ensuring seamless communication between your business systems and Zoho Books. For example, a developer might want to automate the creation of purchase orders when inventory levels fall below a certain threshold, thereby optimizing the supply chain process.
Setting Up a Zoho Books Test/Sandbox Account for API Integration
Before you begin integrating with the Zoho Books API, it's essential to set up a test or sandbox account. This allows you to experiment with API calls without affecting your live data. Zoho Books offers a sandbox environment where developers can test their applications in a controlled setting.
Creating a Zoho Books Account
If you don't already have a Zoho Books account, follow these steps to create one:
- Visit the Zoho Books website and click on the "Sign Up Now" button.
- Fill in the required details, such as your email address and password, to create your account.
- Once registered, you can access the Zoho Books dashboard.
Accessing the Zoho Books Sandbox Environment
To access the sandbox environment, follow these steps:
- Log in to your Zoho Books account.
- Navigate to the "Developer Space" section in the settings menu.
- Select "Sandbox" to create a sandbox environment for testing purposes.
- Follow the prompts to set up your sandbox, which will mirror your live account settings.
Setting Up OAuth Authentication for Zoho Books API
The Zoho Books API uses OAuth 2.0 for authentication. Follow these steps to set up OAuth:
- Go to the Zoho Developer Console and click on "Add Client ID."
- Provide the necessary details, such as the client name and redirect URI, to register your application.
- Upon successful registration, you will receive a Client ID and Client Secret. Keep these credentials secure.
- Generate a grant token by redirecting users to the Zoho authorization URL with the required parameters, including your Client ID and desired scopes.
- Exchange the grant token for an access token and refresh token by making a POST request to the Zoho token endpoint.
Generating Access and Refresh Tokens
Use the following code snippet to generate access and refresh tokens:
const fetch = require('node-fetch');
const params = new URLSearchParams();
params.append('code', 'YOUR_GRANT_TOKEN');
params.append('client_id', 'YOUR_CLIENT_ID');
params.append('client_secret', 'YOUR_CLIENT_SECRET');
params.append('redirect_uri', 'YOUR_REDIRECT_URI');
params.append('grant_type', 'authorization_code');
fetch('https://accounts.zoho.com/oauth/v2/token', {
method: 'POST',
body: params
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Replace YOUR_GRANT_TOKEN
, YOUR_CLIENT_ID
, YOUR_CLIENT_SECRET
, and YOUR_REDIRECT_URI
with your actual credentials.
Once you have your access token, you can start making API calls to Zoho Books. Remember that the access token expires after a certain period, so use the refresh token to obtain a new access token when needed.
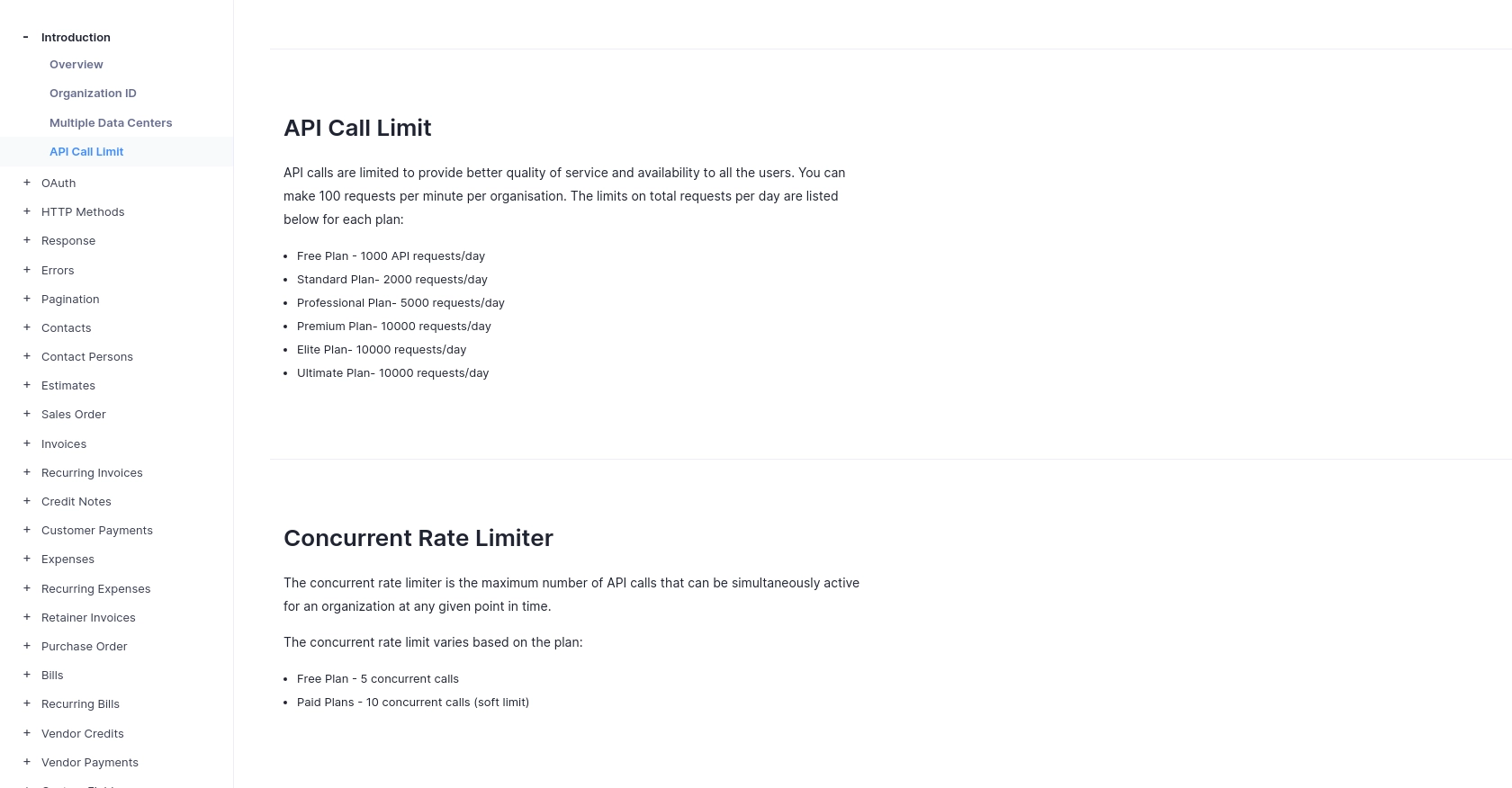
sbb-itb-96038d7
Making API Calls to Create or Update Purchase Orders in Zoho Books Using JavaScript
With your Zoho Books account and OAuth authentication set up, you can now proceed to make API calls to create or update purchase orders. This section will guide you through the process of making these API calls using JavaScript, ensuring seamless integration with Zoho Books.
Prerequisites for Zoho Books API Integration with JavaScript
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine.
- The
node-fetch
library for making HTTP requests. Install it using the command:
npm install node-fetch
Creating a Purchase Order with Zoho Books API
To create a purchase order, you'll need to make a POST request to the Zoho Books API endpoint. Here's a sample code snippet to create a purchase order:
const fetch = require('node-fetch');
const createPurchaseOrder = async () => {
const url = 'https://www.zohoapis.com/books/v3/purchaseorders?organization_id=YOUR_ORG_ID';
const token = 'YOUR_ACCESS_TOKEN';
const purchaseOrderData = {
vendor_id: '460000000026049',
line_items: [
{
item_id: '460000000027009',
quantity: 1,
rate: 112
}
],
purchaseorder_number: 'PO-00001'
};
const response = await fetch(url, {
method: 'POST',
headers: {
'Authorization': `Zoho-oauthtoken ${token}`,
'Content-Type': 'application/json'
},
body: JSON.stringify(purchaseOrderData)
});
const data = await response.json();
console.log(data);
};
createPurchaseOrder().catch(console.error);
Replace YOUR_ORG_ID
and YOUR_ACCESS_TOKEN
with your actual organization ID and access token. The purchaseOrderData
object contains the necessary details for the purchase order, such as the vendor ID and line items.
Updating a Purchase Order with Zoho Books API
To update an existing purchase order, use the PUT method. Here's how you can update a purchase order:
const updatePurchaseOrder = async (purchaseOrderId) => {
const url = `https://www.zohoapis.com/books/v3/purchaseorders/${purchaseOrderId}?organization_id=YOUR_ORG_ID`;
const token = 'YOUR_ACCESS_TOKEN';
const updateData = {
line_items: [
{
line_item_id: '460000000074009',
quantity: 2
}
]
};
const response = await fetch(url, {
method: 'PUT',
headers: {
'Authorization': `Zoho-oauthtoken ${token}`,
'Content-Type': 'application/json'
},
body: JSON.stringify(updateData)
});
const data = await response.json();
console.log(data);
};
updatePurchaseOrder('460000000062001').catch(console.error);
In this example, replace YOUR_ORG_ID
, YOUR_ACCESS_TOKEN
, and purchaseOrderId
with your organization ID, access token, and the ID of the purchase order you wish to update. The updateData
object specifies the changes to be made, such as updating the quantity of a line item.
Handling API Responses and Errors
After making an API call, it's crucial to handle the response and any potential errors. Zoho Books API uses HTTP status codes to indicate the result of an API call. Here are some common status codes:
- 200 OK: The request was successful.
- 201 Created: The resource was successfully created.
- 400 Bad Request: The request was invalid or cannot be served.
- 401 Unauthorized: Authentication failed or user does not have permissions.
- 429 Rate Limit Exceeded: Too many requests in a given amount of time.
For more detailed error handling, refer to the Zoho Books API Error Documentation.
Verifying API Call Success in Zoho Books
To verify that your API calls have succeeded, you can check the Zoho Books dashboard. Newly created or updated purchase orders should appear in the purchase orders section. Additionally, review the API response for confirmation messages.
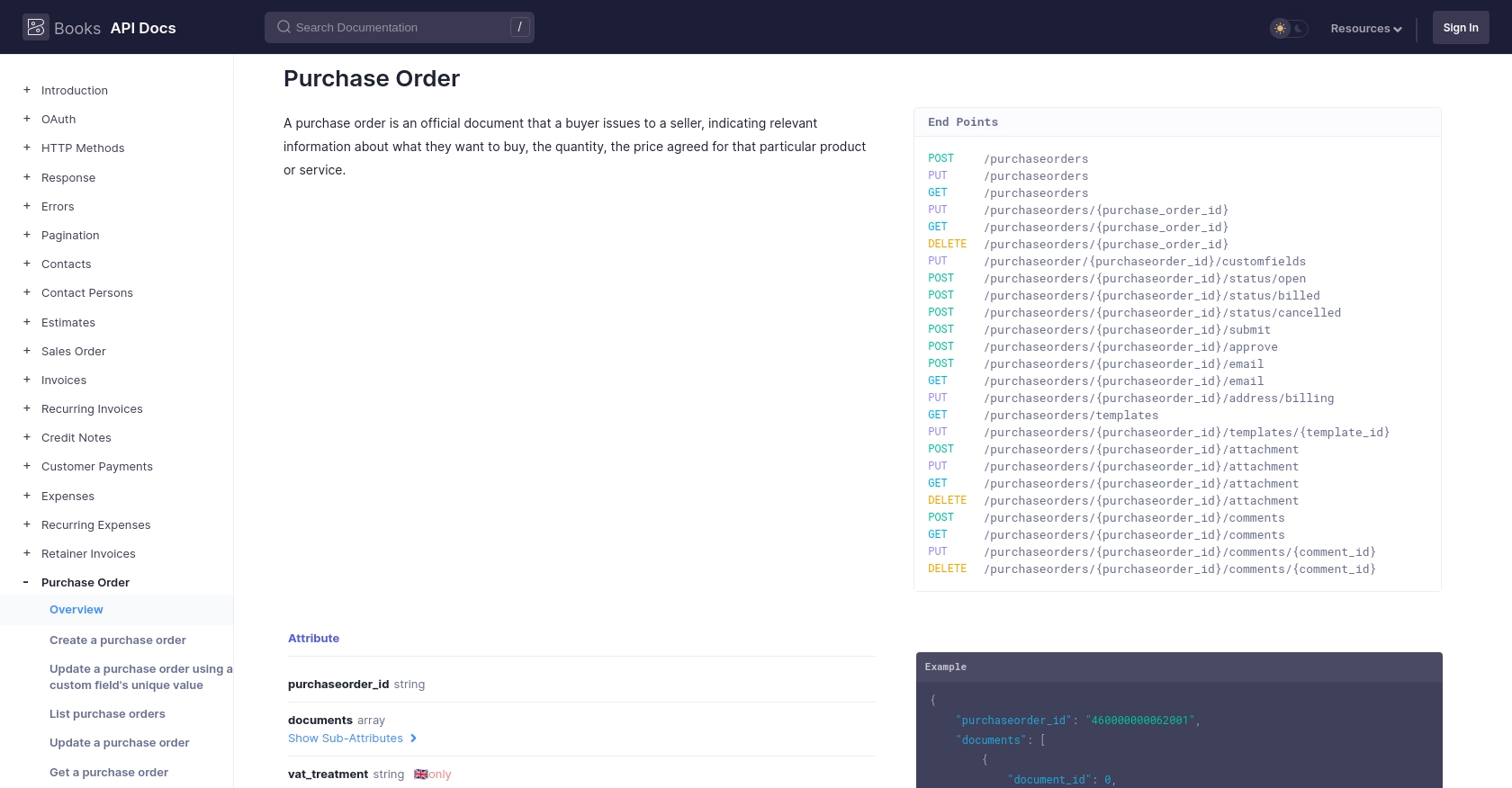
Conclusion and Best Practices for Zoho Books API Integration
Integrating with the Zoho Books API to manage purchase orders can significantly enhance your business operations by automating and streamlining processes. By following the steps outlined in this guide, you can efficiently create and update purchase orders using JavaScript, ensuring seamless communication between your application and Zoho Books.
Best Practices for Secure and Efficient API Integration with Zoho Books
- Securely Store Credentials: Always store your Client ID, Client Secret, and access tokens securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limits: Zoho Books API has a rate limit of 100 requests per minute per organization. Implement logic to handle rate limiting gracefully to avoid disruptions in service. For more details, refer to the API Call Limit Documentation.
- Refresh Tokens Regularly: Access tokens expire after a certain period. Use the refresh token to obtain new access tokens without user intervention, ensuring uninterrupted API access.
- Standardize Data Fields: Ensure that data fields are standardized and validated before making API calls to avoid errors and maintain data integrity.
Leverage Endgrate for Streamlined Integration Solutions
While integrating with Zoho Books API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies integration processes across various platforms, including Zoho Books. By using Endgrate, you can:
- Save time and resources by outsourcing integration tasks and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations, reducing redundancy.
- Provide an intuitive integration experience for your customers, enhancing user satisfaction.
Explore how Endgrate can help you streamline your integration processes by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/zohobooks
- https://www.zoho.com/books/api/v3/introduction/#api-call-limit
- https://www.zoho.com/books/api/v3/oauth/#overview
- https://www.zoho.com/books/api/v3/errors/#overview
- https://www.zoho.com/books/api/v3/pagination/#overview
- https://www.zoho.com/books/api/v3/purchase-order/#overview
Ready to get started?