Using the BigCommerce API to Get Products (with Python examples)
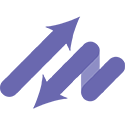
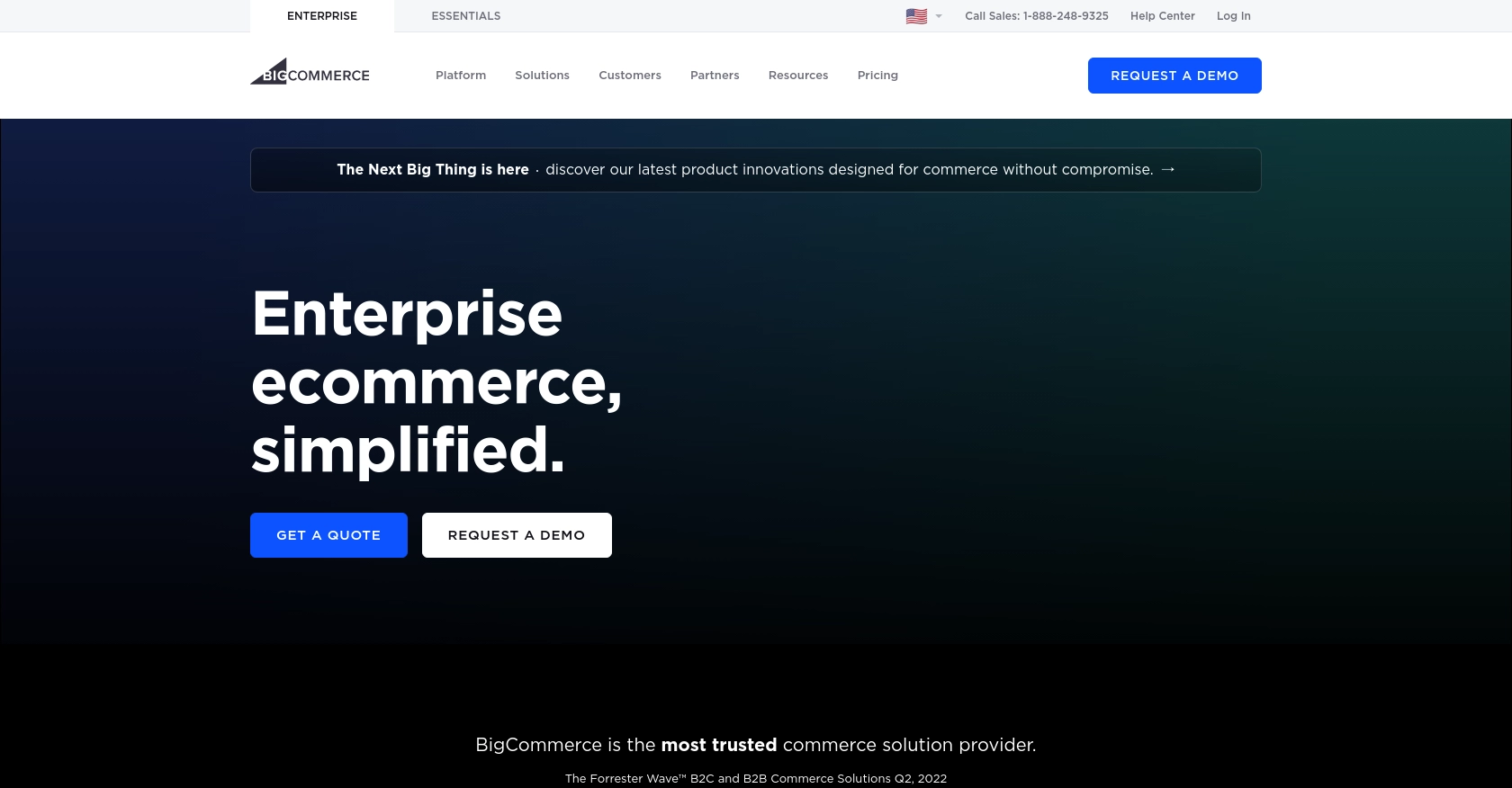
Introduction to BigCommerce API Integration
BigCommerce is a robust e-commerce platform that empowers businesses to create and manage online stores with ease. It offers a comprehensive suite of tools for managing products, orders, and customer relationships, making it a popular choice for businesses looking to scale their online presence.
Integrating with the BigCommerce API allows developers to access and manipulate store data programmatically. This can be particularly useful for automating tasks such as retrieving product information, updating inventory, or managing customer data. For example, a developer might use the BigCommerce API to fetch product details and synchronize them with an external inventory management system, ensuring that stock levels are always up-to-date.
Setting Up Your BigCommerce Test or Sandbox Account for API Integration
Before you can start interacting with the BigCommerce API, it's essential to set up a test or sandbox account. This environment allows you to experiment with API calls without affecting your live store data, providing a safe space to develop and test your integrations.
Creating a BigCommerce Account
If you don't already have a BigCommerce account, you can sign up for a free trial on the BigCommerce website. This trial account will give you access to all the features you need to test the API.
- Visit the BigCommerce website and click on "Start Your Free Trial."
- Fill in the required information to create your account.
- Once your account is set up, you'll have access to the BigCommerce dashboard.
Generating API Credentials
To interact with the BigCommerce API, you'll need to generate API credentials. These credentials include an access token, which you'll use to authenticate your API requests.
- Log in to your BigCommerce account and navigate to the "Advanced Settings" section in the dashboard.
- Select "API Accounts" and click on "Create API Account."
- Choose the type of API account that suits your needs. For most integrations, a "Store API Account" is appropriate.
- Fill in the required details, such as the name of the API account and the OAuth scopes you need. Ensure you select the scopes that allow you to access product data.
- After creating the account, you'll receive an access token. Make sure to store this token securely, as it will be used in the "X-Auth-Token" header for your API requests.
Configuring OAuth Scopes
When setting up your API account, it's crucial to configure the OAuth scopes correctly. These scopes determine the level of access your API account has to the store's data.
- Ensure you have selected the necessary scopes for accessing product information, such as "Products" and "Catalog."
- Review the BigCommerce authentication documentation for detailed information on available scopes and their purposes.
Testing API Calls in the Sandbox Environment
With your API credentials ready, you can begin testing API calls in the sandbox environment. This allows you to verify your integration without impacting your live store data.
Use the access token in the "X-Auth-Token" header of your API requests to authenticate. You can start by making simple GET requests to retrieve product data and ensure your setup is correct.
By following these steps, you'll be well-prepared to start integrating with the BigCommerce API and leveraging its capabilities to enhance your e-commerce operations.
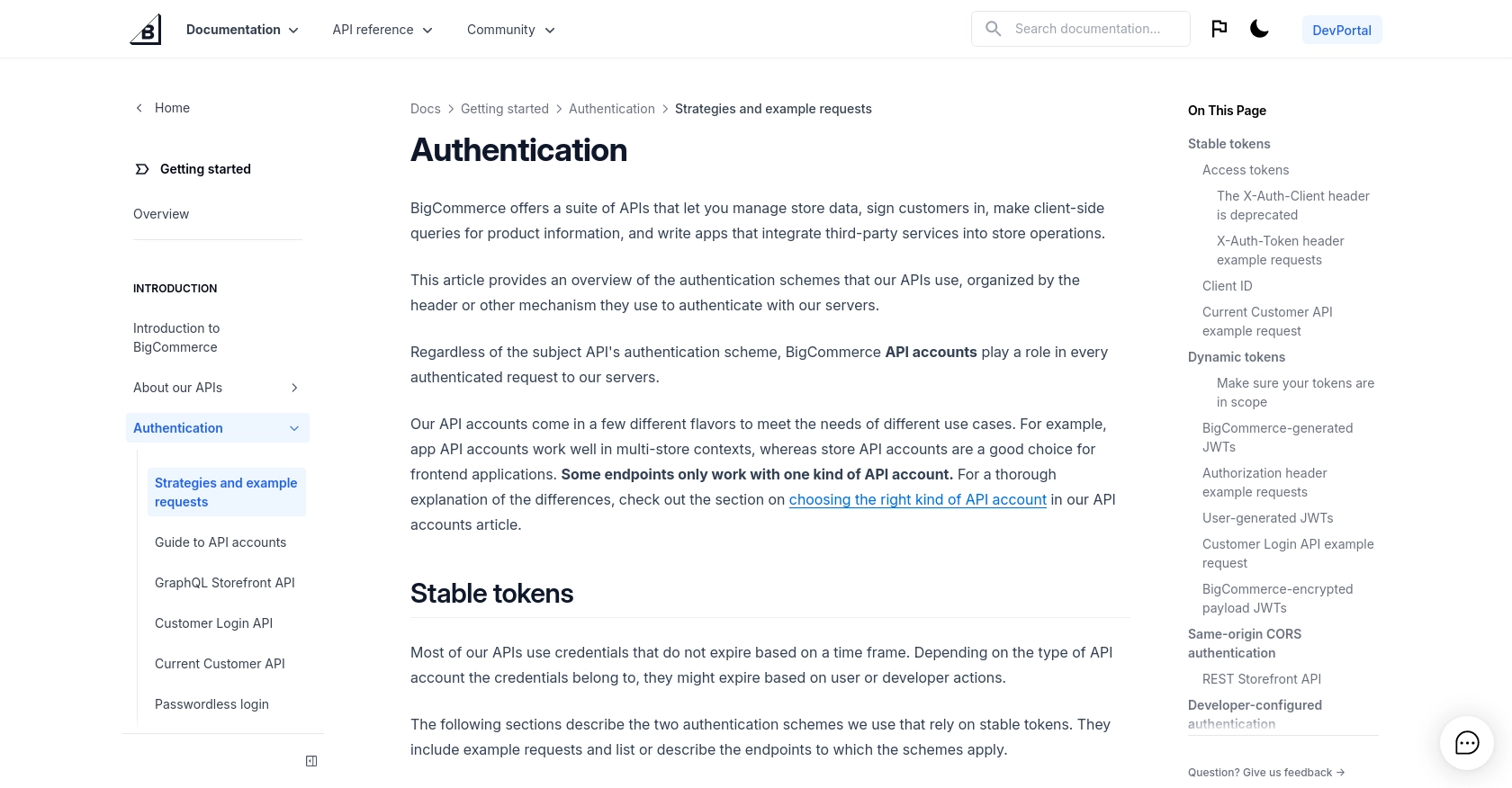
sbb-itb-96038d7
Making API Calls to Retrieve Products from BigCommerce Using Python
To interact with the BigCommerce API and retrieve product information, you'll need to use Python, a versatile programming language known for its simplicity and readability. This section will guide you through the process of setting up your environment, making API calls, and handling responses effectively.
Setting Up Your Python Environment for BigCommerce API Integration
Before making API calls, ensure you have Python installed on your machine. We recommend using Python 3.6 or later. Additionally, you'll need the requests
library to handle HTTP requests.
- Install Python from the official website if you haven't already.
- Use the following command to install the
requests
library:
pip install requests
Example Code to Fetch Products from BigCommerce API
Once your environment is set up, you can start writing code to interact with the BigCommerce API. Below is a sample Python script to retrieve a list of products from your BigCommerce store.
import requests
# Define the API endpoint and headers
store_hash = 'your_store_hash'
access_token = 'your_access_token'
endpoint = f'https://api.bigcommerce.com/stores/{store_hash}/v3/catalog/products'
headers = {
'X-Auth-Token': access_token,
'Accept': 'application/json'
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
products = response.json()
for product in products['data']:
print(f"Product Name: {product['name']}, Price: {product['price']}")
else:
print(f"Failed to retrieve products: {response.status_code}")
Replace your_store_hash
and your_access_token
with your actual store hash and access token. This script sends a GET request to the BigCommerce API to fetch product data and prints the name and price of each product.
Verifying API Call Success and Handling Errors
After running the script, you should see a list of product names and prices printed in the console. If the request fails, the script will output an error message with the status code. Common error codes include:
- 401 Unauthorized: Check your access token and ensure it has the correct permissions.
- 404 Not Found: Verify the endpoint URL and store hash.
- 500 Internal Server Error: This may indicate an issue with the BigCommerce server. Try again later.
For more detailed information on error codes, refer to the BigCommerce API documentation.
Best Practices for BigCommerce API Integration
When working with the BigCommerce API, consider the following best practices:
- Store your access token securely and avoid hardcoding it in your scripts.
- Implement error handling to manage API call failures gracefully.
- Be mindful of API rate limits to avoid exceeding the allowed number of requests.
By following these guidelines, you can ensure a smooth and efficient integration with the BigCommerce API.
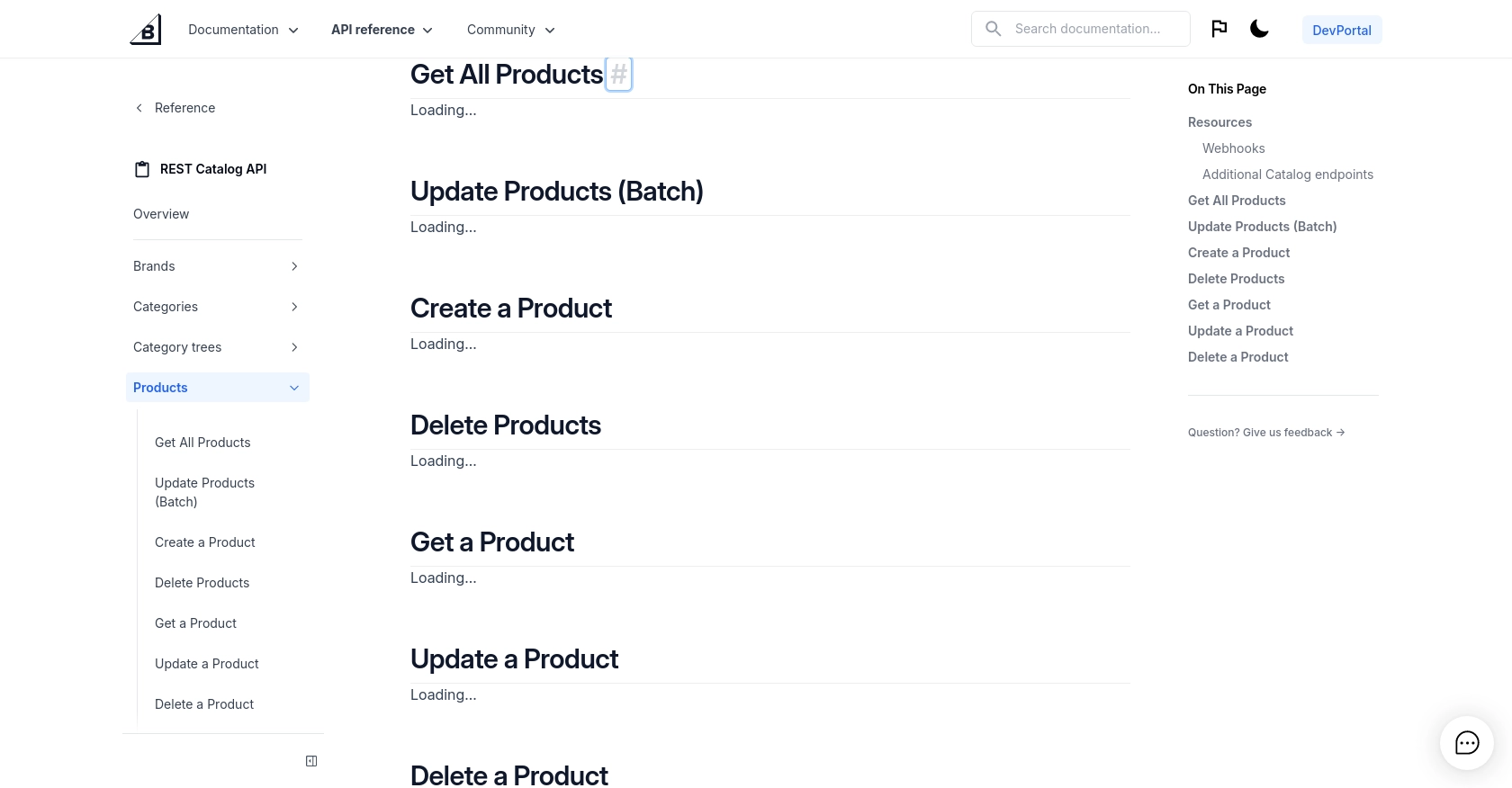
Conclusion and Best Practices for BigCommerce API Integration
Integrating with the BigCommerce API using Python offers a powerful way to automate and streamline your e-commerce operations. By following the steps outlined in this guide, you can efficiently retrieve product data and ensure your inventory management systems are always up-to-date.
To maximize the benefits of your integration, consider implementing the following best practices:
- Secure API Credentials: Always store your access tokens securely and avoid exposing them in your codebase. Consider using environment variables or secure vaults to manage sensitive information.
- Handle API Rate Limits: Be aware of BigCommerce's API rate limits to prevent disruptions in service. Implement retry logic and exponential backoff strategies to handle rate limit errors gracefully.
- Standardize Data Formats: Ensure that the data retrieved from BigCommerce is transformed and standardized to match your internal systems, facilitating seamless integration and data consistency.
- Monitor API Performance: Regularly monitor your API calls for performance and error rates. Use logging and alerting mechanisms to quickly identify and resolve issues.
By adhering to these best practices, you can create a robust and efficient integration with BigCommerce, enhancing your store's capabilities and improving overall operational efficiency.
Streamline Your Integrations with Endgrate
If managing multiple integrations becomes overwhelming, consider leveraging Endgrate to simplify the process. With Endgrate, you can build once for each use case and connect to multiple platforms through a single API endpoint. This approach not only saves time and resources but also provides an intuitive integration experience for your customers.
Visit Endgrate to learn more about how you can optimize your integration strategy and focus on your core product development.
Read More
Ready to get started?