Using the Capsule API to Get Users in Javascript
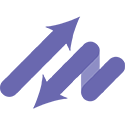
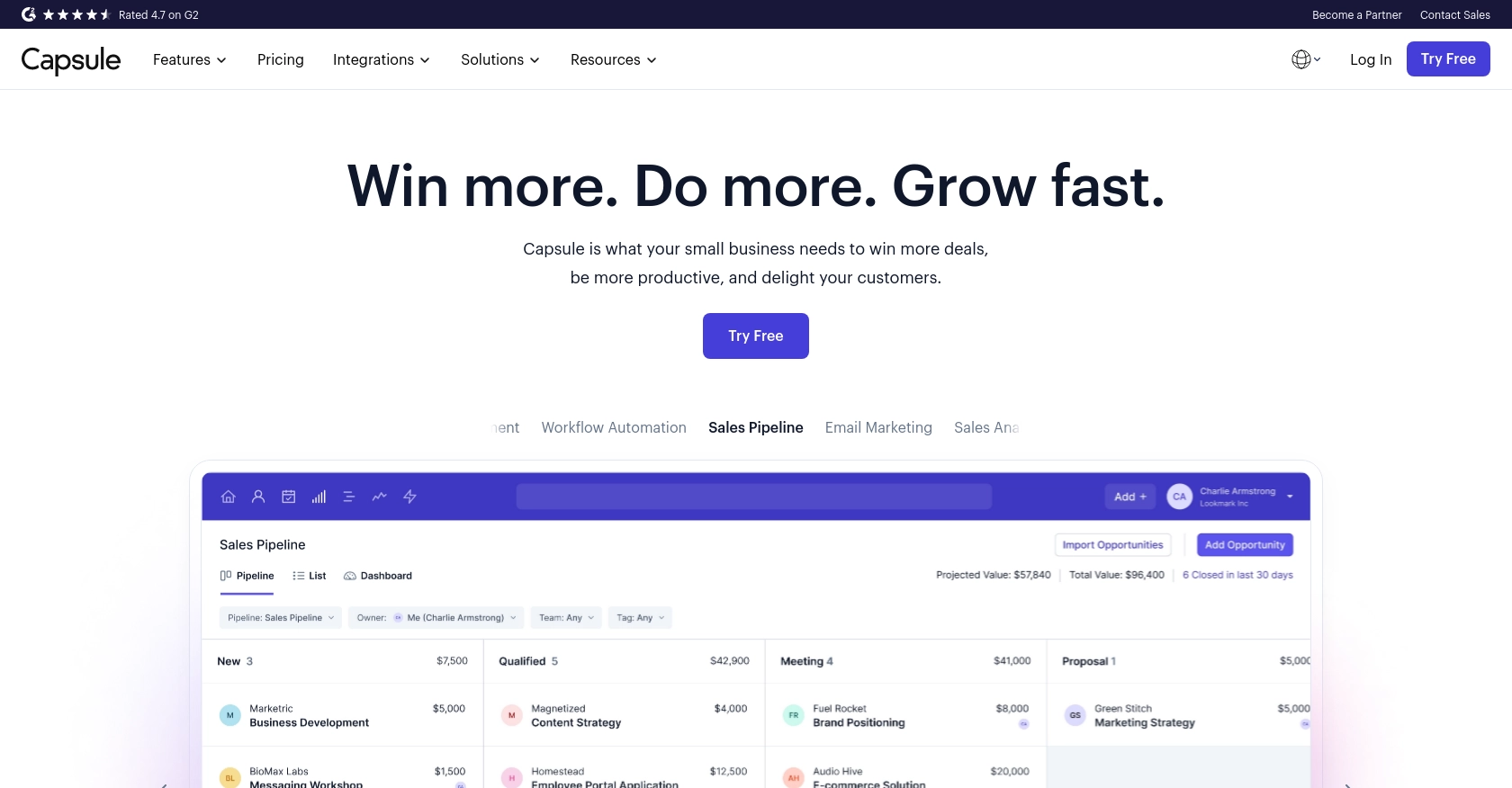
Introduction to Capsule CRM
Capsule CRM is a versatile customer relationship management platform that helps businesses manage their customer interactions, sales pipeline, and more. With its user-friendly interface and robust features, Capsule CRM is a popular choice for businesses looking to streamline their CRM processes.
Developers may want to integrate with Capsule CRM to access and manage user data, enabling seamless synchronization of customer information across various platforms. For example, using the Capsule API, a developer can retrieve user details to personalize customer interactions or automate reporting processes.
Setting Up Your Capsule CRM Test Account
Before diving into the Capsule API, you'll need to set up a test account. Capsule CRM offers a straightforward process for developers to create a sandbox environment, allowing you to experiment with API calls without affecting live data.
Creating a Capsule CRM Account
If you don't already have a Capsule CRM account, you can sign up for a free trial on the Capsule CRM website. Follow the on-screen instructions to complete the registration process.
Generating OAuth Credentials for Capsule API
Capsule CRM uses OAuth 2.0 for authentication, ensuring secure access to user data. Follow these steps to generate the necessary credentials:
- Log in to your Capsule CRM account.
- Navigate to My Preferences and select API Authentication Tokens.
- Click on Create New Token to generate a token for testing purposes.
- Copy the generated token and store it securely, as you'll need it for API requests.
Registering Your Application with Capsule
To use OAuth 2.0, you must register your application with Capsule CRM:
- Go to the Developer Portal on the Capsule website.
- Register your application by providing necessary details such as the application name and redirect URI.
- Once registered, you'll receive a Client ID and Client Secret. Keep these credentials secure.
Obtaining an Authorization Code
To obtain an authorization code, redirect users to Capsule's authorization URL:
const authorizationUrl = 'https://api.capsulecrm.com/oauth/authorise';
const clientId = 'YOUR_CLIENT_ID';
const redirectUri = 'YOUR_REDIRECT_URI';
const scope = 'read write';
const state = 'random_string';
window.location.href = `${authorizationUrl}?response_type=code&client_id=${clientId}&redirect_uri=${redirectUri}&scope=${scope}&state=${state}`;
Replace YOUR_CLIENT_ID
and YOUR_REDIRECT_URI
with your actual client ID and redirect URI.
Exchanging the Authorization Code for Tokens
After the user authorizes your application, Capsule will redirect to your specified URI with an authorization code. Exchange this code for access and refresh tokens:
const tokenUrl = 'https://api.capsulecrm.com/oauth/token';
const code = 'AUTHORIZATION_CODE';
fetch(tokenUrl, {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({
code: code,
client_id: 'YOUR_CLIENT_ID',
client_secret: 'YOUR_CLIENT_SECRET',
grant_type: 'authorization_code'
})
})
.then(response => response.json())
.then(data => {
console.log('Access Token:', data.access_token);
console.log('Refresh Token:', data.refresh_token);
})
.catch(error => console.error('Error:', error));
Ensure you replace AUTHORIZATION_CODE
, YOUR_CLIENT_ID
, and YOUR_CLIENT_SECRET
with the appropriate values.
With your test account and OAuth credentials set up, you're ready to start making API calls to Capsule CRM.
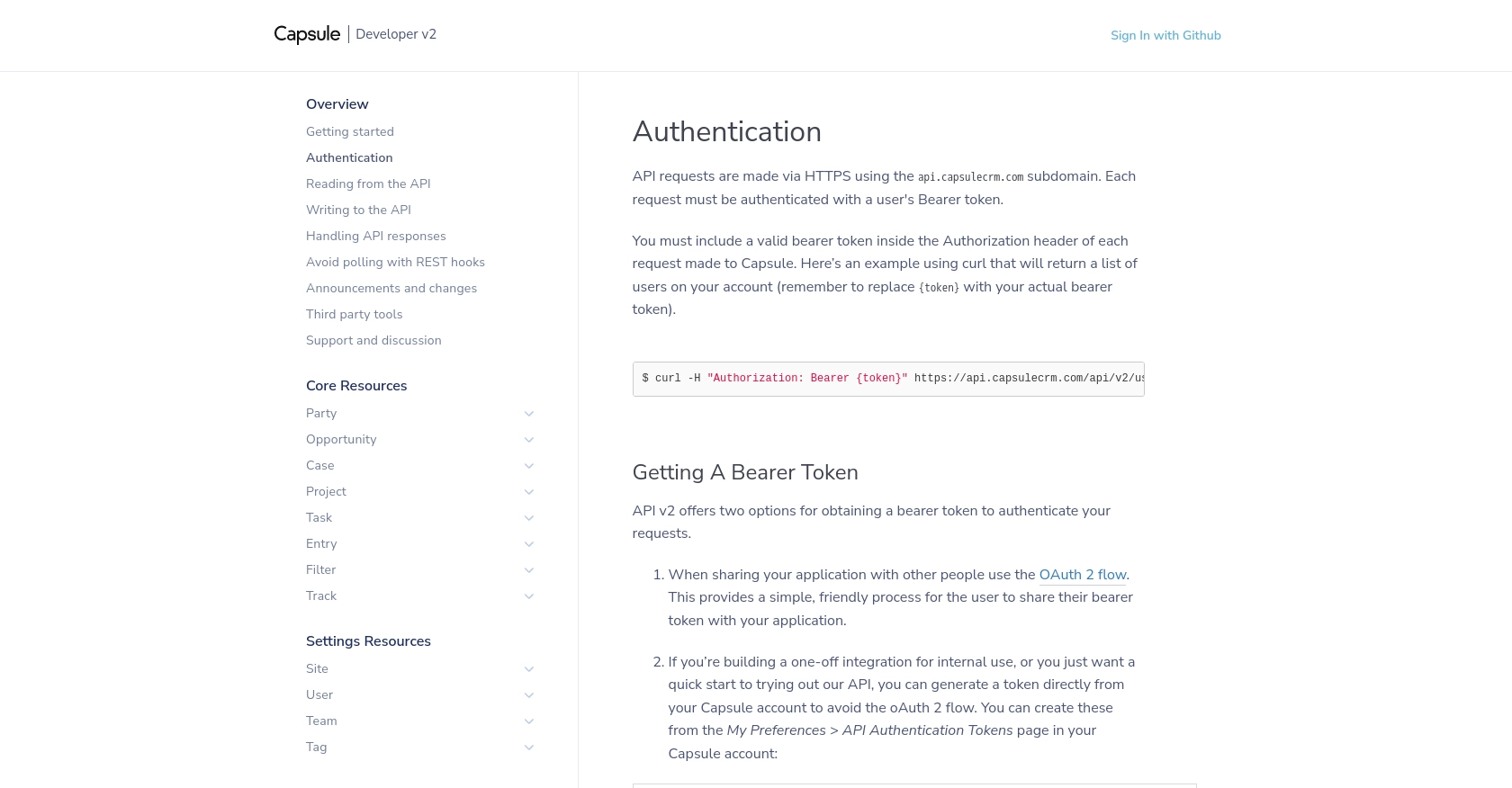
sbb-itb-96038d7
Making API Calls to Retrieve Users from Capsule CRM Using JavaScript
Now that you have set up your Capsule CRM account and obtained the necessary OAuth credentials, it's time to make API calls to retrieve user information. This section will guide you through the process of using JavaScript to interact with the Capsule API and fetch user data.
Prerequisites for Capsule API Integration with JavaScript
Before proceeding, ensure you have the following:
- Node.js installed on your machine.
- A text editor or IDE for writing JavaScript code.
- Access and refresh tokens obtained from the OAuth process.
Installing Required Dependencies for Capsule API Calls
To make HTTP requests in JavaScript, you'll need to use a library like axios
. Install it using npm:
npm install axios
Fetching Users from Capsule CRM
With the setup complete, you can now write the code to fetch users from Capsule CRM. Create a new JavaScript file named getCapsuleUsers.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.capsulecrm.com/api/v2/users';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json'
};
// Function to get users
async function getUsers() {
try {
const response = await axios.get(endpoint, { headers });
const users = response.data.users;
console.log('Users:', users);
} catch (error) {
console.error('Error fetching users:', error.response ? error.response.data : error.message);
}
}
// Call the function
getUsers();
Replace YOUR_ACCESS_TOKEN
with the access token you obtained earlier.
Running the JavaScript Code to Retrieve Capsule Users
To execute the code, run the following command in your terminal:
node getCapsuleUsers.js
If successful, you should see a list of users from your Capsule CRM account displayed in the console.
Handling Errors and Verifying API Call Success
When making API calls, it's crucial to handle potential errors. The code above includes error handling to log any issues that arise during the request. Common errors include:
- 401 Unauthorized: Invalid or expired access token. Ensure your token is correct and hasn't expired.
- 403 Forbidden: Insufficient permissions. Check if your token has the necessary scope.
- 429 Too Many Requests: Rate limit exceeded. Capsule allows up to 4,000 requests per hour. Implement rate limiting strategies if needed.
For more information on error handling, refer to the Capsule API documentation.
By following these steps, you can efficiently retrieve user data from Capsule CRM using JavaScript, enabling seamless integration and data synchronization across your applications.
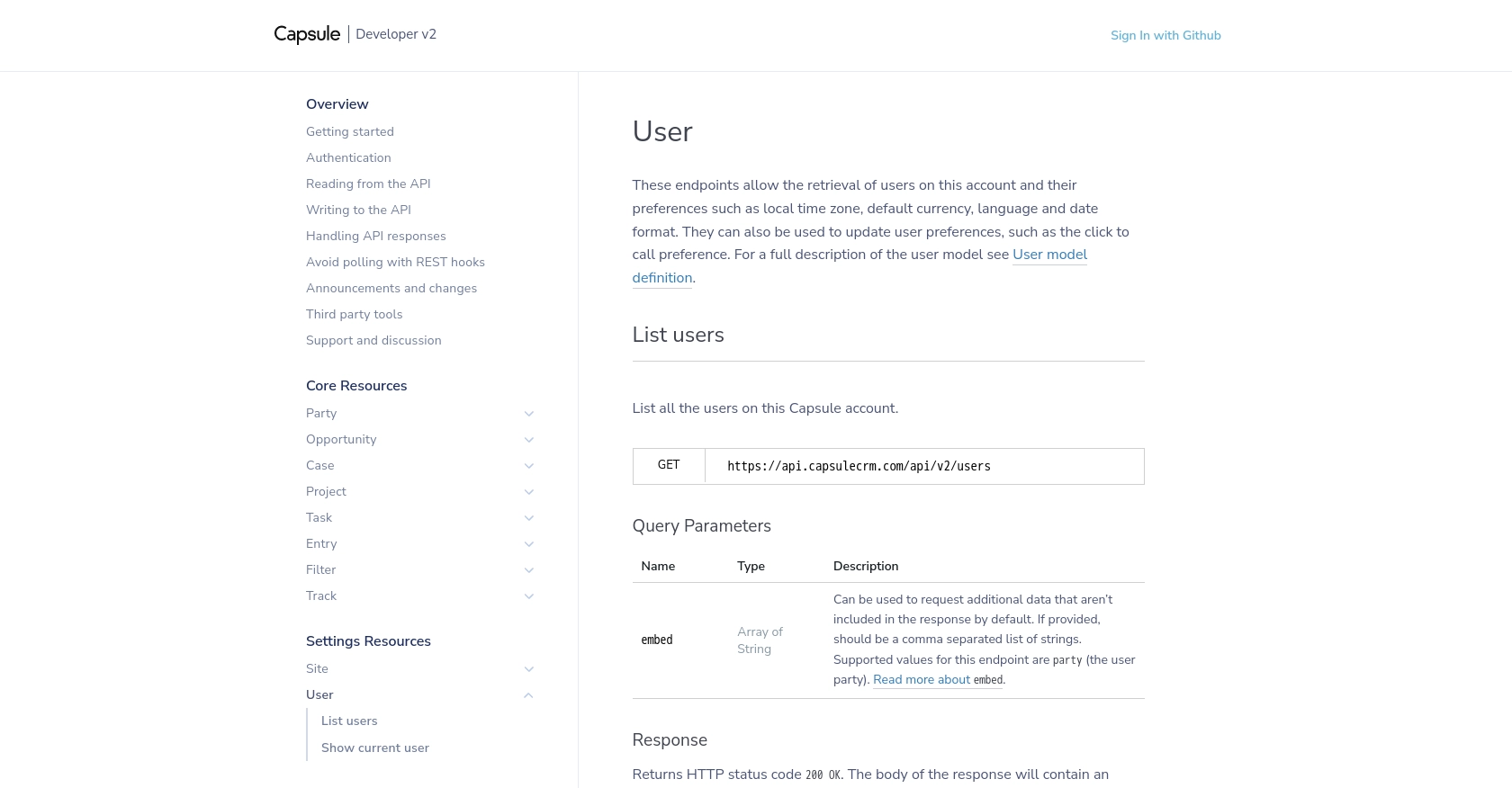
Best Practices for Capsule API Integration and User Data Management
Successfully integrating with the Capsule API requires attention to best practices to ensure secure and efficient data handling. Here are some key recommendations:
Securely Storing OAuth Credentials
Always store your OAuth credentials, such as client ID, client secret, and tokens, securely. Avoid hardcoding them in your source code. Instead, use environment variables or secure vaults to manage sensitive information.
Handling Capsule API Rate Limits
Capsule API enforces a rate limit of 4,000 requests per hour. To avoid exceeding this limit, implement strategies such as:
- Throttling requests by adding delays between API calls.
- Caching responses to minimize redundant requests.
- Monitoring the
X-RateLimit-Remaining
header to adjust request frequency dynamically.
For more details, refer to the Capsule API documentation.
Transforming and Standardizing User Data
When retrieving user data from Capsule, consider transforming and standardizing fields to match your application's data model. This ensures consistency and simplifies data processing across different systems.
Enhance Your Integration Experience with Endgrate
Building and maintaining integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to multiple platforms, including Capsule. With Endgrate, you can:
- Save time and resources by outsourcing integration tasks.
- Focus on your core product while ensuring seamless integration experiences for your users.
- Build once for each use case, reducing the need for multiple integrations.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate.
Read More
Ready to get started?