Using the Commerce7 API to Get Customers in Python
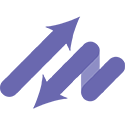
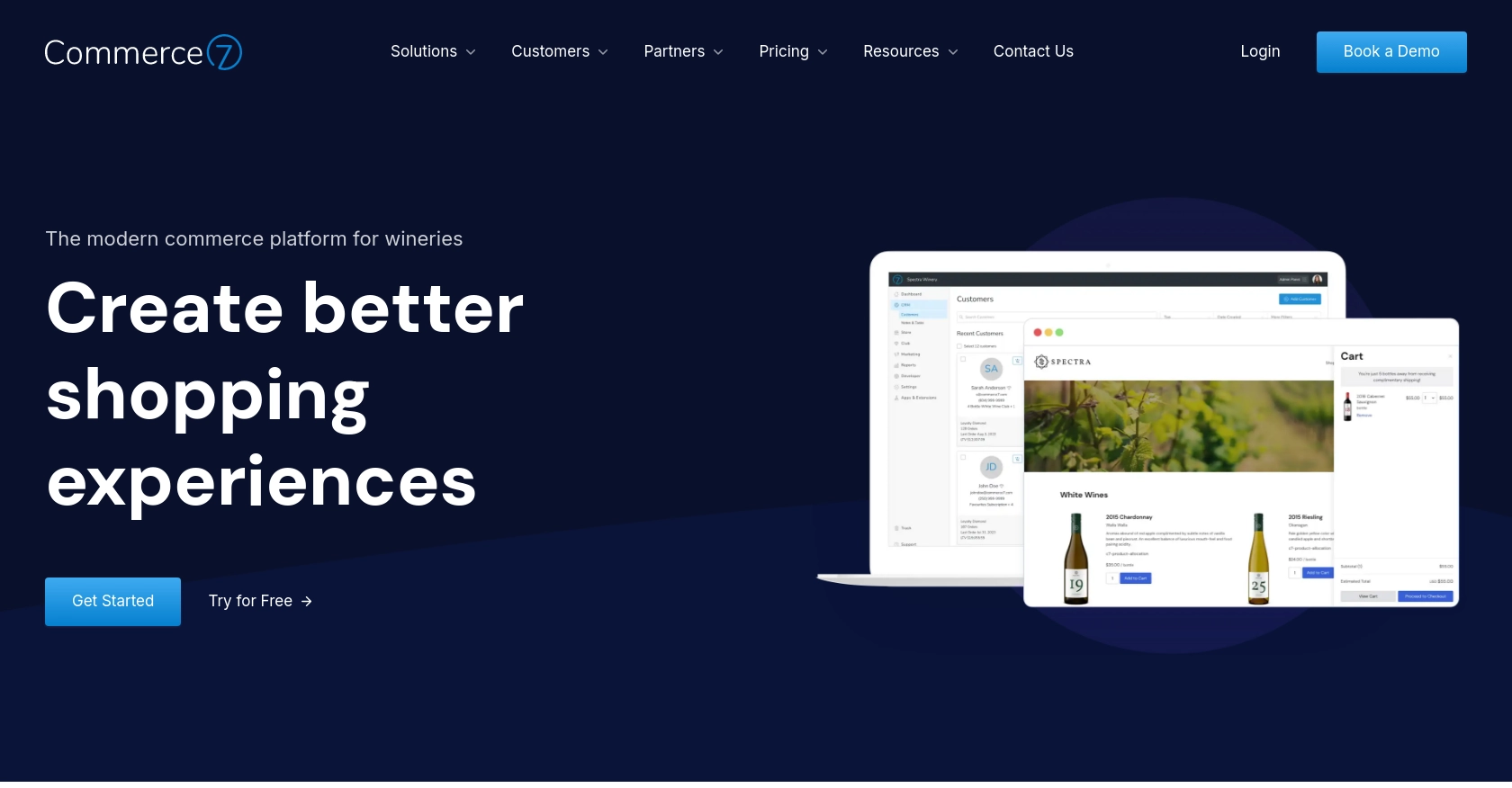
Introduction to Commerce7 API Integration
Commerce7 is a powerful e-commerce platform tailored for the wine industry, offering a comprehensive suite of tools to enhance customer engagement and streamline sales processes. With features like club memberships, reservations, and personalized marketing, Commerce7 helps wineries deliver exceptional customer experiences.
Integrating with the Commerce7 API allows developers to access and manage customer data efficiently. For example, you can retrieve customer information to personalize marketing campaigns or analyze purchasing trends. This integration can significantly enhance your ability to tailor services and improve customer satisfaction.
Setting Up Your Commerce7 Developer Account
Before you can interact with the Commerce7 API, you'll need to set up a developer account. This will allow you to create an app and obtain the necessary credentials for API access.
Creating a Commerce7 Developer Account
To get started, visit the Commerce7 Developer Center and sign up for an account. If you already have an account, simply log in.
Creating a Commerce7 App for API Access
Once logged in, navigate to the Developers tab and select the App Dev Center. Here, you can create a new app that will allow you to interact with the Commerce7 API.
- Click the Add App button to start creating your app.
- Fill in the necessary details for your app, such as the app name and description.
- Select the API endpoints you need access to, ensuring you include the customer-related endpoints.
Generating API Credentials
After creating your app, you'll receive an App ID and an App Secret Key. These credentials are essential for authenticating your API requests.
- Keep your App Secret Key secure and do not expose it in client-side code.
- You can regenerate the App Secret Key if needed from the app dashboard.
Understanding Commerce7 Authentication
Commerce7 uses Basic Auth for API requests. You'll authenticate using your App ID as the username and your App Secret Key as the password. Additionally, you'll need the tenant ID of the client account you're accessing.
For more detailed information, refer to the Commerce7 App Creation Guide and the Commerce7 API Documentation.
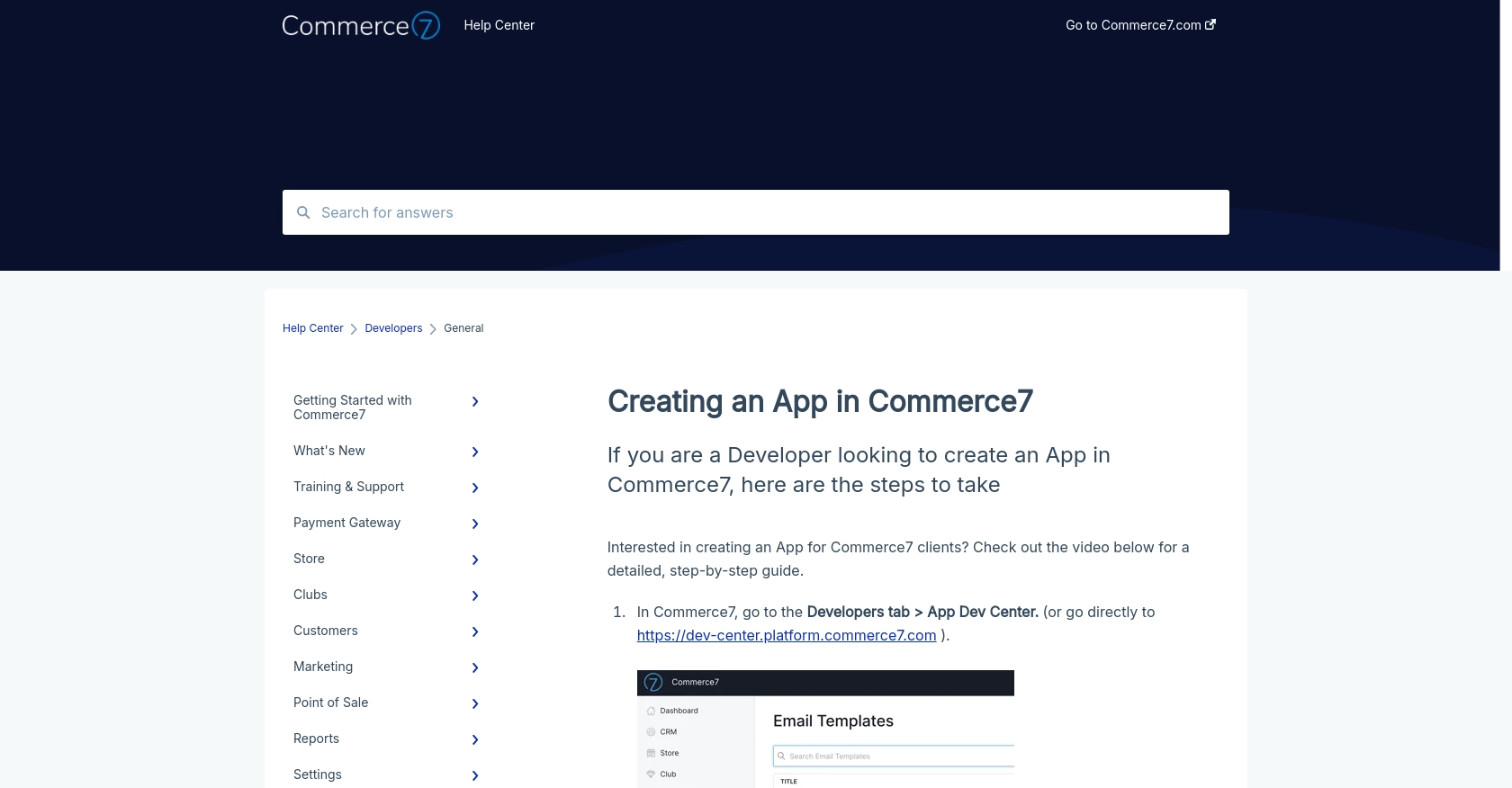
sbb-itb-96038d7
Making API Calls to Retrieve Customers from Commerce7 Using Python
To interact with the Commerce7 API and retrieve customer data, you'll need to use Python, a versatile and widely-used programming language. This section will guide you through setting up your environment, writing the code, and executing API calls to fetch customer information.
Setting Up Your Python Environment for Commerce7 API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Verify your Python installation by running
python --version
in your terminal. - Install the
requests
library using pip:
pip install requests
Writing Python Code to Fetch Customers from Commerce7
Create a new Python file named get_commerce7_customers.py
and add the following code:
import requests
# Set the API endpoint and authentication details
endpoint = "https://api.commerce7.com/v1/customer"
app_id = "Your_App_ID"
app_secret = "Your_App_Secret_Key"
tenant_id = "Your_Tenant_ID"
# Set up Basic Auth
auth = (app_id, app_secret)
# Make a GET request to the API
response = requests.get(endpoint, auth=auth, headers={"tenant": tenant_id})
# Check if the request was successful
if response.status_code == 200:
customers = response.json().get("customers", [])
for customer in customers:
print(f"Customer Name: {customer['firstName']} {customer['lastName']}")
else:
print(f"Failed to retrieve customers: {response.status_code} - {response.text}")
Replace Your_App_ID
, Your_App_Secret_Key
, and Your_Tenant_ID
with your actual Commerce7 credentials.
Executing the Python Script to Retrieve Commerce7 Customers
Run the script from your terminal using the following command:
python get_commerce7_customers.py
If successful, the script will output a list of customer names retrieved from your Commerce7 account.
Handling Errors and Verifying API Call Success
It's crucial to handle potential errors when making API calls. The script checks the HTTP status code to determine if the request was successful. A status code of 200 indicates success, while other codes may indicate issues such as authentication errors or rate limits.
For more detailed error handling, refer to the Commerce7 API Documentation.
Understanding Commerce7 API Rate Limits
Commerce7 imposes rate limits of 100 requests per minute per tenant. If you exceed this limit, you'll need to implement logic to handle rate limiting, such as pausing requests or using cursor-based pagination for efficient data retrieval. For more details, see the Commerce7 API Rate Limiting Guide.
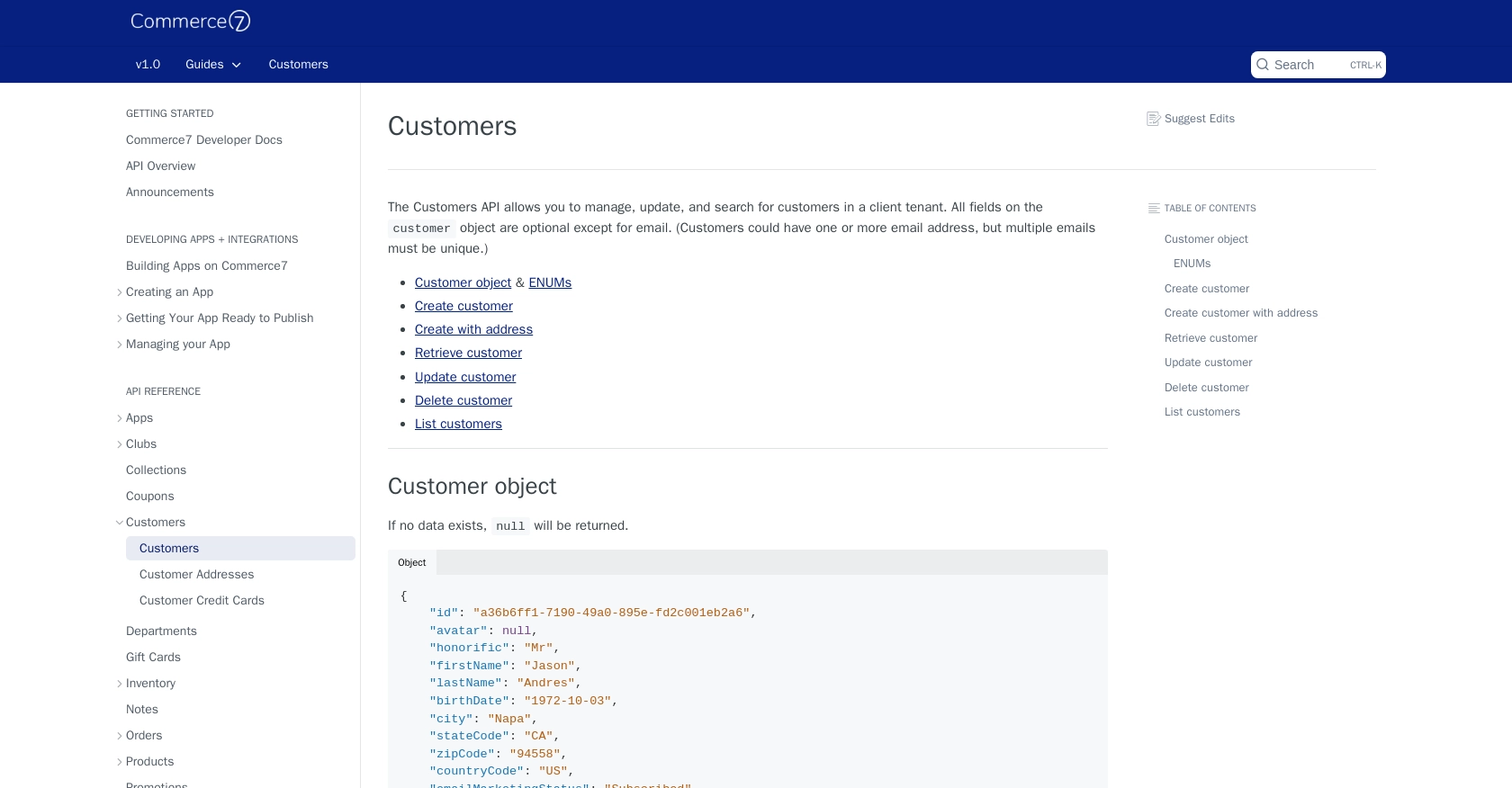
Best Practices for Commerce7 API Integration
When integrating with the Commerce7 API, it's essential to follow best practices to ensure a secure and efficient implementation. Here are some recommendations:
- Securely Store Credentials: Always store your App Secret Key securely and avoid exposing it in client-side code. Use environment variables or secure vaults to manage sensitive information.
- Implement Rate Limiting: Commerce7 enforces a rate limit of 100 requests per minute per tenant. To prevent exceeding this limit, implement logic to pause requests or use cursor-based pagination for efficient data retrieval.
- Handle Errors Gracefully: Ensure your application can handle various HTTP status codes and error messages. Implement retry logic for transient errors and provide meaningful feedback to users.
- Standardize Data Fields: When processing customer data, ensure consistency by standardizing fields such as dates and currency amounts according to Commerce7's specifications.
Enhance Your Integration Experience with Endgrate
Integrating multiple platforms can be complex and time-consuming. Endgrate simplifies this process by offering a unified API endpoint that connects to various platforms, including Commerce7. By using Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Provide an easy, intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate.
Read More
Ready to get started?