How to Get Candidates with the Recruitee API in PHP
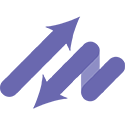
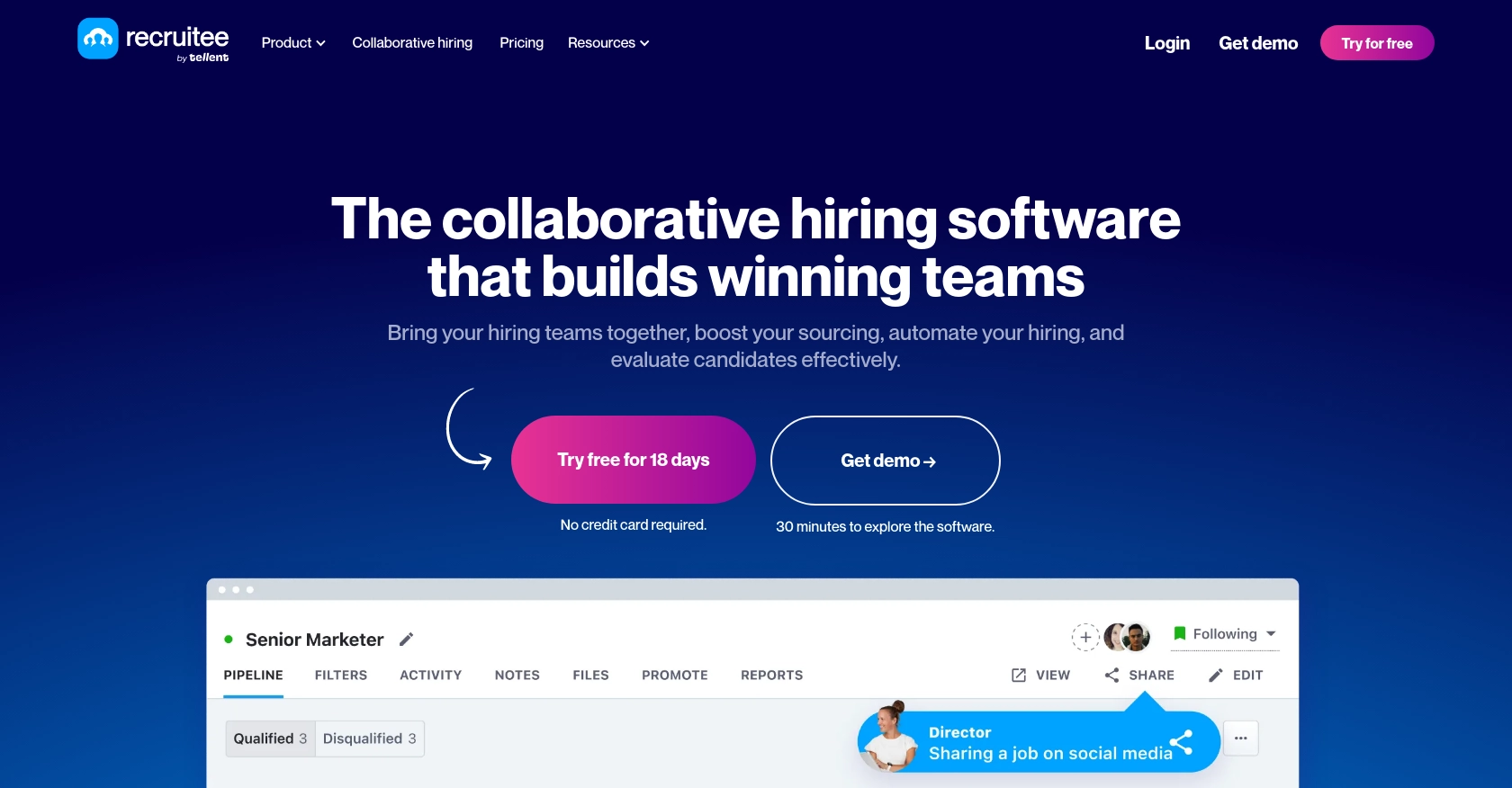
Introduction to Recruitee API
Recruitee is a powerful applicant tracking system (ATS) designed to streamline the recruitment process for businesses of all sizes. It offers a comprehensive suite of tools to manage job postings, track candidates, and collaborate with hiring teams, making it an essential platform for modern recruitment strategies.
Integrating with Recruitee's API allows developers to automate and enhance recruitment workflows. For example, you can use the Recruitee API to retrieve candidate information and seamlessly integrate it into your company's HR systems, ensuring a smooth and efficient hiring process.
Setting Up Your Recruitee Test Account
Before you can start interacting with the Recruitee API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Recruitee provides a straightforward way to create a personal API token, which is necessary for authentication.
Creating a Recruitee Account
If you don't already have a Recruitee account, you can sign up for a free trial on their website. This will give you access to the platform's features and allow you to generate the necessary API token.
Generating a Personal API Token in Recruitee
Once you have access to your Recruitee account, follow these steps to generate a personal API token:
- Navigate to Settings in the top navigation bar.
- Go to Apps and plugins and select Personal API tokens.
- Click on + New token in the top-right corner to generate a new token.
- Save the generated token securely, as it will be used for authenticating your API requests.
According to Recruitee's documentation, a personal API token does not expire unless revoked by the user. For more details, refer to the Recruitee API documentation.
Understanding API Key Authentication
Recruitee uses API key-based authentication. This means you'll need to include your personal API token in the headers of your API requests to authenticate them. This process ensures that your requests are secure and authorized.
With your test account and API token ready, you're now set to start making API calls to retrieve candidate information using PHP.
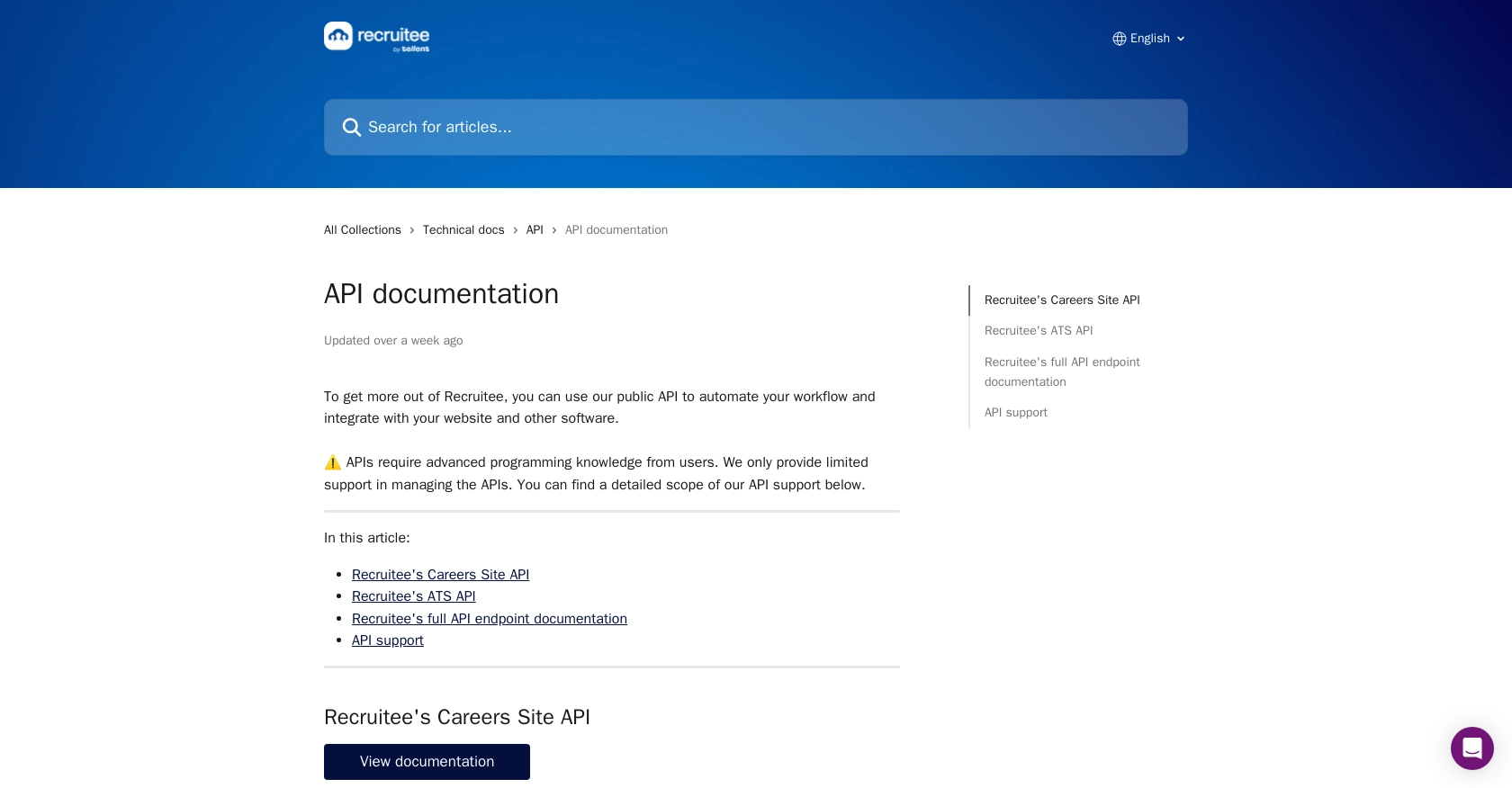
sbb-itb-96038d7
Making API Calls to Retrieve Candidates with Recruitee API in PHP
To interact with the Recruitee API and retrieve candidate information, you'll need to use PHP to make HTTP requests. This section will guide you through the process of setting up your PHP environment, making the API call, and handling the response.
Setting Up Your PHP Environment for Recruitee API
Before making API calls, ensure that you have PHP installed on your machine. You can download it from the official PHP website. Additionally, you'll need the cURL
extension enabled, which is commonly used for making HTTP requests in PHP.
To verify that cURL
is enabled, create a PHP file with the following content and run it:
<?php
phpinfo();
?>
Look for the cURL
section in the output. If it's not enabled, you may need to uncomment the extension=curl
line in your php.ini
file and restart your server.
Writing PHP Code to Call Recruitee API
With your environment ready, you can now write the PHP code to make an API call to Recruitee and retrieve candidate data. Create a new PHP file named get_candidates.php
and add the following code:
<?php
// Set your Recruitee company ID and API token
$company_id = 'your_company_id';
$api_token = 'your_api_token';
// Set the API endpoint URL
$url = "https://api.recruitee.com/c/$company_id/candidates";
// Initialize cURL session
$ch = curl_init($url);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
"Authorization: Bearer $api_token"
]);
// Execute the cURL request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'cURL error: ' . curl_error($ch);
} else {
// Parse and display the response
$candidates = json_decode($response, true);
foreach ($candidates as $candidate) {
echo "Name: " . $candidate['name'] . "<br>";
echo "Email: " . implode(', ', $candidate['emails']) . "<br>";
echo "Phone: " . implode(', ', $candidate['phones']) . "<br><br>";
}
}
// Close the cURL session
curl_close($ch);
?>
Replace your_company_id
and your_api_token
with your actual Recruitee company ID and API token.
Executing the PHP Script and Handling Responses
Run the get_candidates.php
script from the command line or your web server. The script will make a GET request to the Recruitee API and display the list of candidates with their names, emails, and phone numbers.
If the request is successful, you should see the candidate information printed on the screen. If there's an error, the script will output the cURL error message.
Handling Errors and Verifying API Call Success
It's important to handle potential errors when making API calls. The Recruitee API may return various HTTP status codes to indicate success or failure. Common error codes include:
- 400 Bad Request: The request was invalid. Check your parameters.
- 401 Unauthorized: Authentication failed. Verify your API token.
- 404 Not Found: The requested resource could not be found.
- 500 Internal Server Error: An error occurred on the server. Try again later.
For more detailed information on error handling, refer to the Recruitee API documentation.
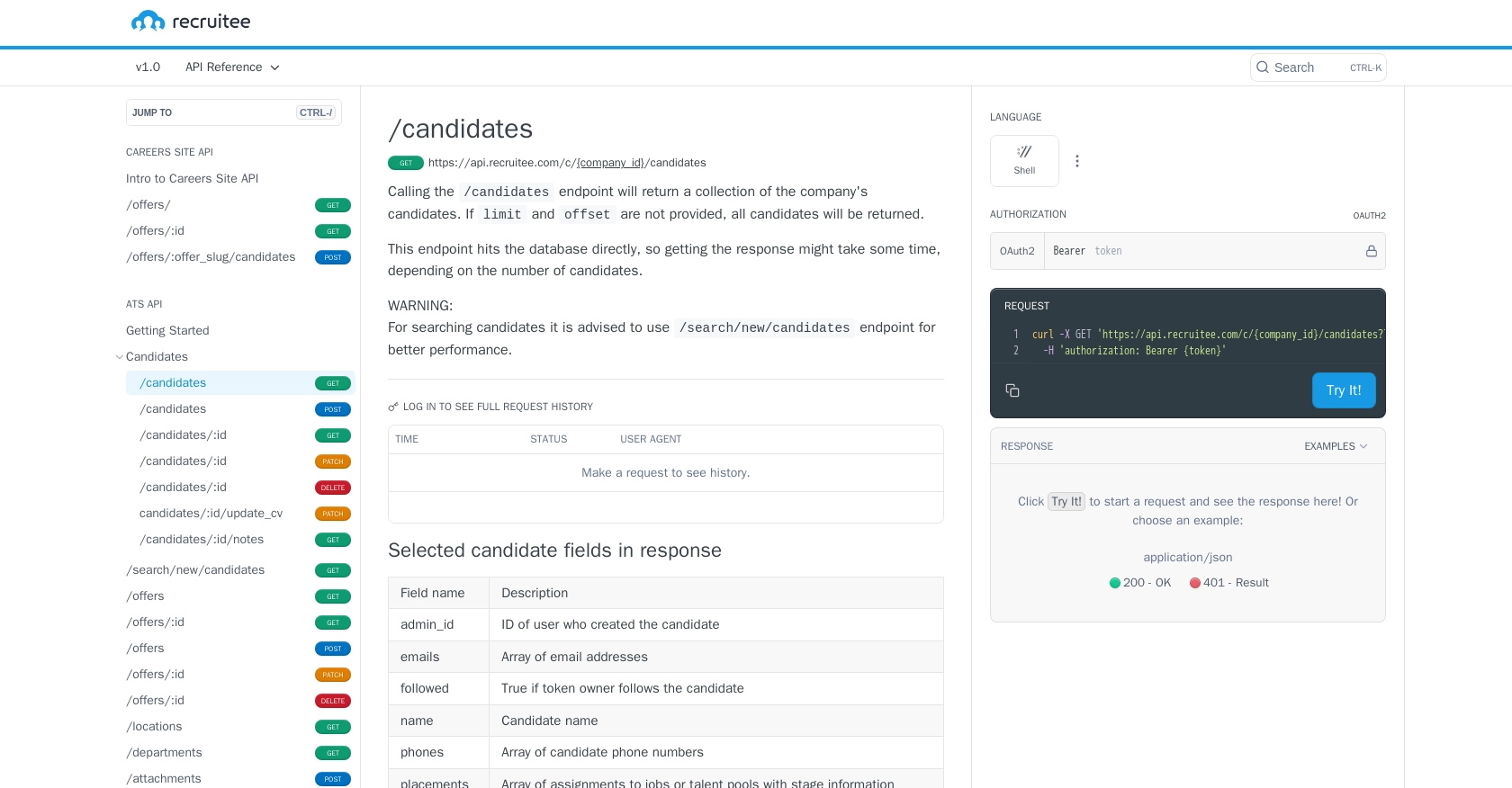
Conclusion and Best Practices for Using Recruitee API in PHP
Integrating with the Recruitee API using PHP can significantly enhance your recruitment processes by automating candidate data retrieval and management. By following the steps outlined in this guide, you can efficiently connect to Recruitee's ATS API and access valuable candidate information.
Best Practices for Secure and Efficient API Integration
- Secure Storage of API Tokens: Always store your API tokens securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of Recruitee's rate limits to avoid throttling. Implement exponential backoff strategies to manage retries effectively.
- Data Standardization: Ensure that candidate data retrieved from the API is standardized to match your internal data structures for seamless integration.
- Error Handling: Implement robust error handling to manage API response codes and ensure smooth operation even when issues arise.
Streamline Your Integrations with Endgrate
While integrating with Recruitee's API can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Recruitee.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. Build once for each use case and enjoy an intuitive integration experience for your customers. Explore how Endgrate can transform your integration strategy by visiting Endgrate.
Read More
Ready to get started?