How to Get Tax Rates with the Quickbooks API in Javascript
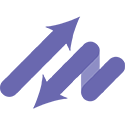
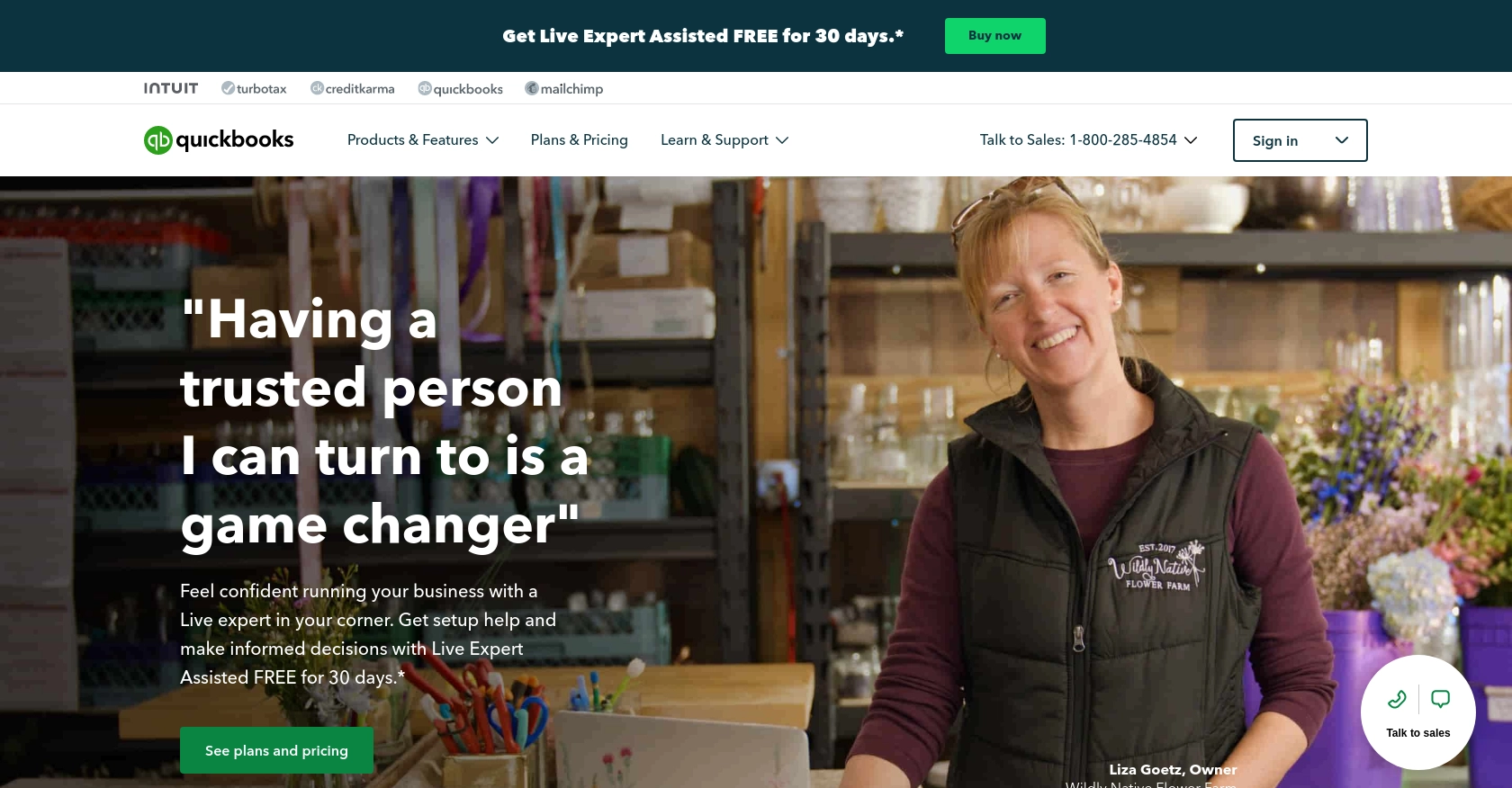
Introduction to QuickBooks API
QuickBooks is a widely-used accounting software that offers a robust platform for managing financial tasks such as invoicing, payroll, and tax calculations. Its comprehensive suite of tools makes it an essential resource for businesses looking to streamline their accounting processes.
Developers often integrate with the QuickBooks API to automate and enhance financial operations. For example, accessing tax rates through the QuickBooks API can help businesses ensure accurate tax calculations and compliance with local tax regulations. By retrieving tax rates programmatically, developers can create applications that automatically apply the correct tax rates to transactions, reducing manual errors and saving time.
Setting Up Your QuickBooks Sandbox Account for API Integration
Before you can start retrieving tax rates using the QuickBooks API, you need to set up a QuickBooks sandbox account. This sandbox environment allows developers to test API interactions without affecting live data, ensuring a safe space for development and experimentation.
Creating a QuickBooks Developer Account
To begin, you'll need to create a QuickBooks Developer account. Follow these steps:
- Visit the QuickBooks Developer Portal.
- Click on "Sign Up" to create a new account or "Sign In" if you already have one.
- Complete the registration process by providing the necessary information.
Setting Up a QuickBooks Sandbox Company
Once your developer account is ready, you can set up a sandbox company:
- Log in to your QuickBooks Developer account.
- Navigate to the "Sandbox" section in the dashboard.
- Click on "Add Sandbox" to create a new sandbox company.
- Follow the prompts to configure your sandbox environment.
Creating an App for OAuth Authentication
QuickBooks API uses OAuth 2.0 for authentication. You need to create an app to obtain the client ID and client secret:
- In your QuickBooks Developer account, go to the "My Apps" section.
- Click on "Create an App" and select "QuickBooks Online and Payments."
- Fill in the required details about your app, such as name and description.
- Once the app is created, navigate to the "Keys & OAuth" tab.
- Here, you'll find your client ID and client secret. Keep these credentials secure as they will be used for API authentication.
For more detailed instructions, refer to the QuickBooks OAuth Setup Guide.
Configuring OAuth Scopes for Tax Rate Access
To access tax rates, ensure your app has the necessary OAuth scopes:
- In the "Keys & OAuth" tab of your app, click on "Select Scopes."
- Choose the scopes related to accounting data, specifically those required for tax rate access.
- Save your changes to update the app's permissions.
With your sandbox account and app set up, you're now ready to start making API calls to retrieve tax rates using JavaScript. This setup ensures you have a secure and isolated environment to test your integration with QuickBooks.
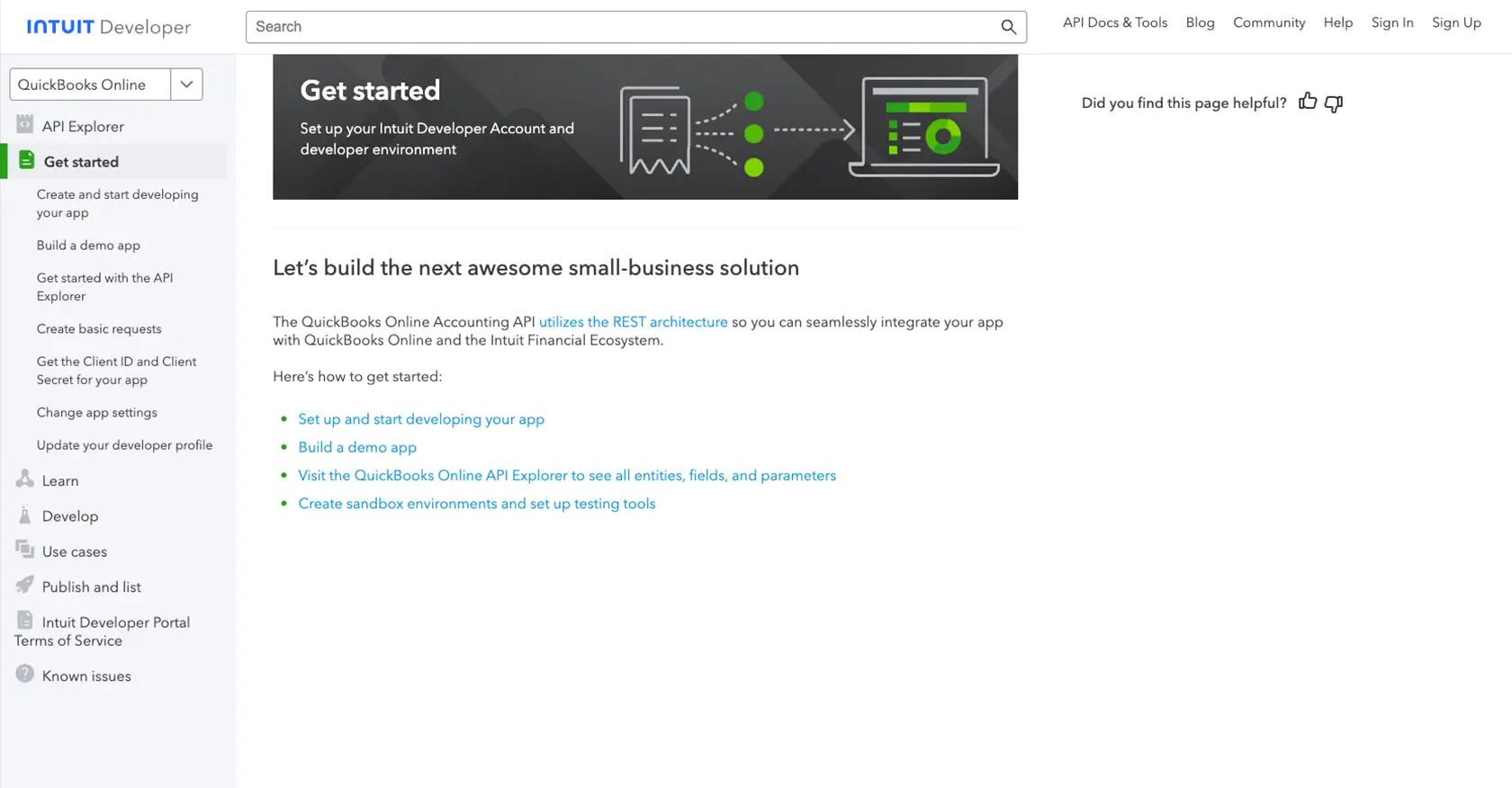
sbb-itb-96038d7
Making API Calls to Retrieve Tax Rates with QuickBooks API in JavaScript
With your QuickBooks sandbox and app configured, you're ready to make API calls to retrieve tax rates using JavaScript. This section will guide you through the process of setting up your environment, writing the code, and handling potential errors.
Setting Up Your JavaScript Environment for QuickBooks API
Before you start coding, ensure you have the necessary tools and libraries installed:
- Node.js: Make sure you have Node.js installed on your machine. You can download it from the official Node.js website.
- Axios: A promise-based HTTP client for the browser and Node.js. Install it using npm:
npm install axios
Writing JavaScript Code to Retrieve Tax Rates from QuickBooks API
Create a new JavaScript file named getTaxRates.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://sandbox-quickbooks.api.intuit.com/v3/company/YOUR_COMPANY_ID/query';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type': 'application/text'
};
// Define the query to retrieve tax rates
const query = 'SELECT * FROM TaxRate';
// Make a POST request to the API
axios.post(endpoint, query, { headers })
.then(response => {
// Parse and display the tax rates
const taxRates = response.data.QueryResponse.TaxRate;
taxRates.forEach(rate => {
console.log(`Tax Rate Name: ${rate.Name}, Rate: ${rate.RateValue}`);
});
})
.catch(error => {
console.error('Error fetching tax rates:', error.response ? error.response.data : error.message);
});
Replace YOUR_COMPANY_ID
and YOUR_ACCESS_TOKEN
with your actual QuickBooks company ID and access token.
Running the JavaScript Code and Verifying Results
Execute the script using Node.js by running the following command in your terminal:
node getTaxRates.js
If successful, you should see a list of tax rates printed in the console. Verify the results by checking the tax rates in your QuickBooks sandbox account.
Handling Errors and Understanding QuickBooks API Error Codes
When making API calls, it's crucial to handle potential errors gracefully. The QuickBooks API may return various error codes, such as:
- 401 Unauthorized: Check if your access token is valid and has the correct scopes.
- 403 Forbidden: Ensure your app has the necessary permissions to access tax rates.
- 500 Internal Server Error: Retry the request or contact QuickBooks support if the issue persists.
For more detailed error information, refer to the QuickBooks API documentation.
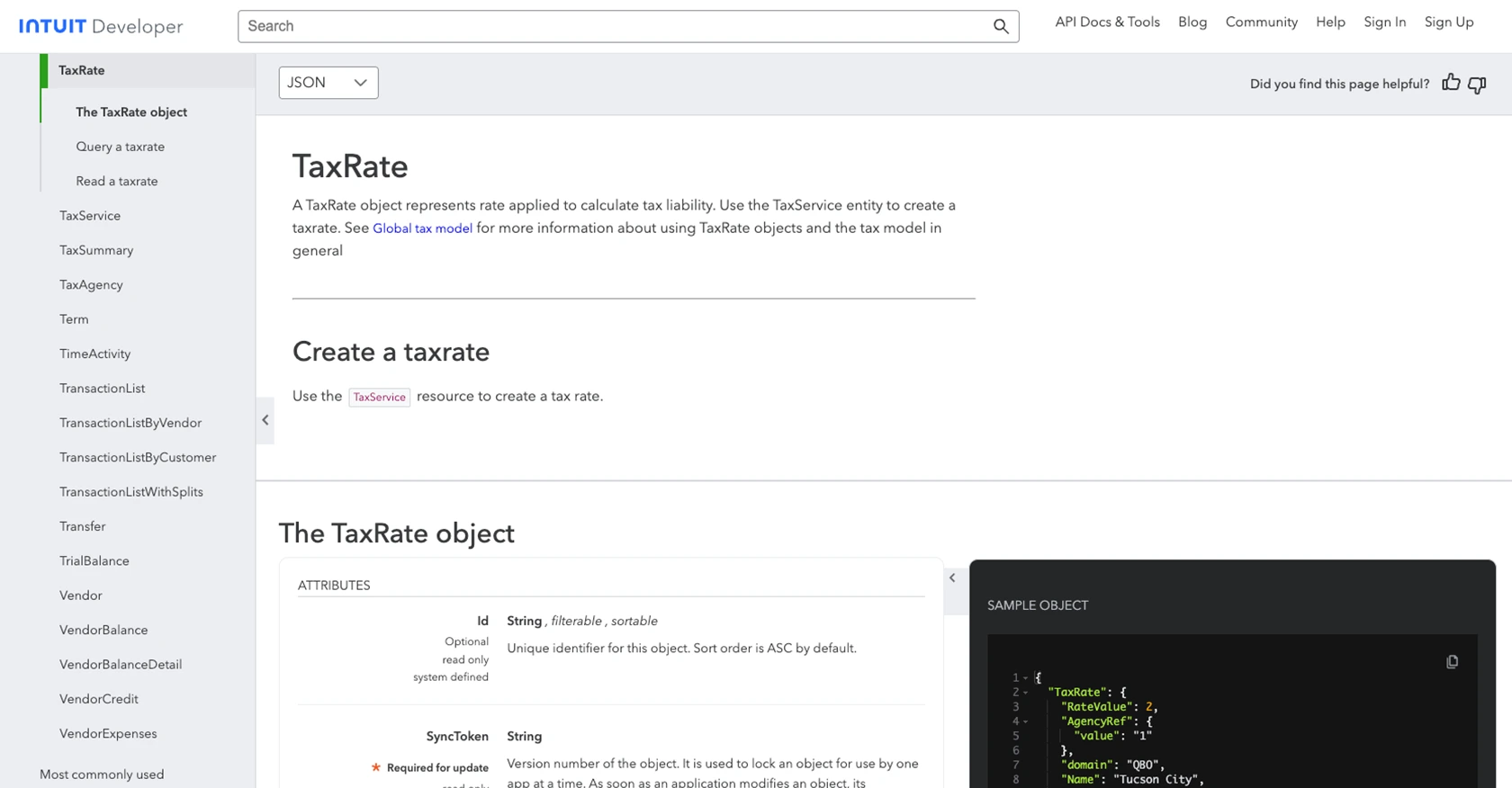
Conclusion and Best Practices for Using QuickBooks API in JavaScript
Integrating with the QuickBooks API to retrieve tax rates using JavaScript can significantly enhance your application's financial accuracy and efficiency. By automating tax calculations, you reduce manual errors and ensure compliance with local regulations, ultimately saving time and resources.
Best Practices for Secure and Efficient QuickBooks API Integration
- Secure Storage of Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limiting: QuickBooks API may impose rate limits. Implement logic to handle HTTP 429 responses by retrying requests after a delay. Refer to the QuickBooks API documentation for specific rate limit details.
- Data Standardization: Ensure that tax rate data retrieved from QuickBooks is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement robust error handling to manage API errors gracefully. Log errors for troubleshooting and provide user-friendly messages when issues occur.
Streamlining Integration with Endgrate
While integrating with QuickBooks API can be powerful, managing multiple integrations can become complex. Endgrate simplifies this process by providing a unified API endpoint for various platforms, including QuickBooks. This allows you to focus on your core product while outsourcing integration complexities.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Explore how Endgrate can save you time and capital by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/quickbooks
- https://developer.intuit.com/app/developer/qbo/docs/get-started/start-developing-your-app
- https://developer.intuit.com/app/developer/qbo/docs/get-started/get-client-id-and-client-secret
- https://developer.intuit.com/app/developer/qbo/docs/get-started/app-settings
- https://developer.intuit.com/app/developer/qbo/docs/api/accounting/all-entities/taxrate
Ready to get started?