Using the Sugar Market API to Get Accounts in Javascript
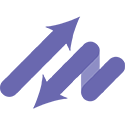
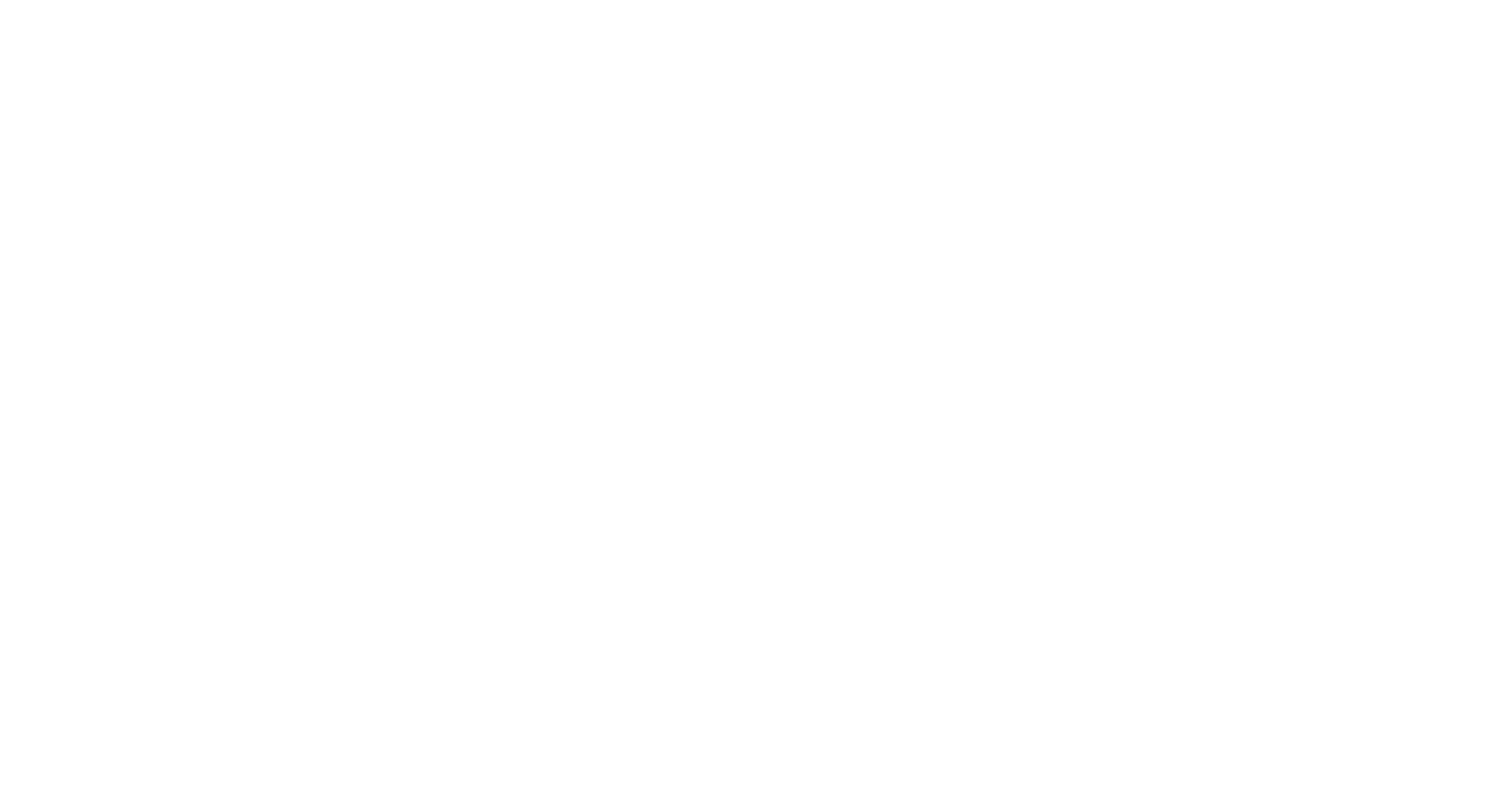
Introduction to Sugar Market API
Sugar Market, part of the SugarCRM suite, is a powerful marketing automation platform designed to enhance marketing efforts through data-driven insights and streamlined processes. It offers a comprehensive set of tools for campaign management, lead nurturing, and analytics, making it a popular choice for businesses looking to optimize their marketing strategies.
Developers may want to integrate with the Sugar Market API to access and manage account data, enabling seamless synchronization between marketing and sales platforms. For example, a developer could use the Sugar Market API to retrieve account information and use it to personalize marketing campaigns or generate detailed reports on customer interactions.
Setting Up Your Sugar Market Test Account
Before you can start interacting with the Sugar Market API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data.
Creating a Sugar Market Account
If you don't already have a Sugar Market account, you can sign up for a free trial or request access to a sandbox environment through the SugarCRM website. Follow the instructions provided to create your account.
Generating API Credentials for Sugar Market
Once your account is set up, you'll need to generate API credentials to authenticate your requests. Sugar Market uses a custom authentication method, so follow these steps to obtain your credentials:
- Log in to your Sugar Market account.
- Navigate to the API settings section, typically found under the account or developer settings.
- Follow the prompts to create a new API application. You'll need to provide details such as the application name and description.
- Once the application is created, you'll receive a client ID and client secret. Make sure to store these securely, as you'll need them to authenticate your API requests.
Configuring OAuth for Sugar Market API
Although Sugar Market uses a custom authentication method, it may involve OAuth-like steps. Here's how you can set it up:
- Use the client ID and client secret obtained earlier to request an access token from the Sugar Market authorization server.
- Include this access token in the header of your API requests to authenticate them.
Refer to the Sugar Market API documentation for detailed instructions on authentication and obtaining access tokens.
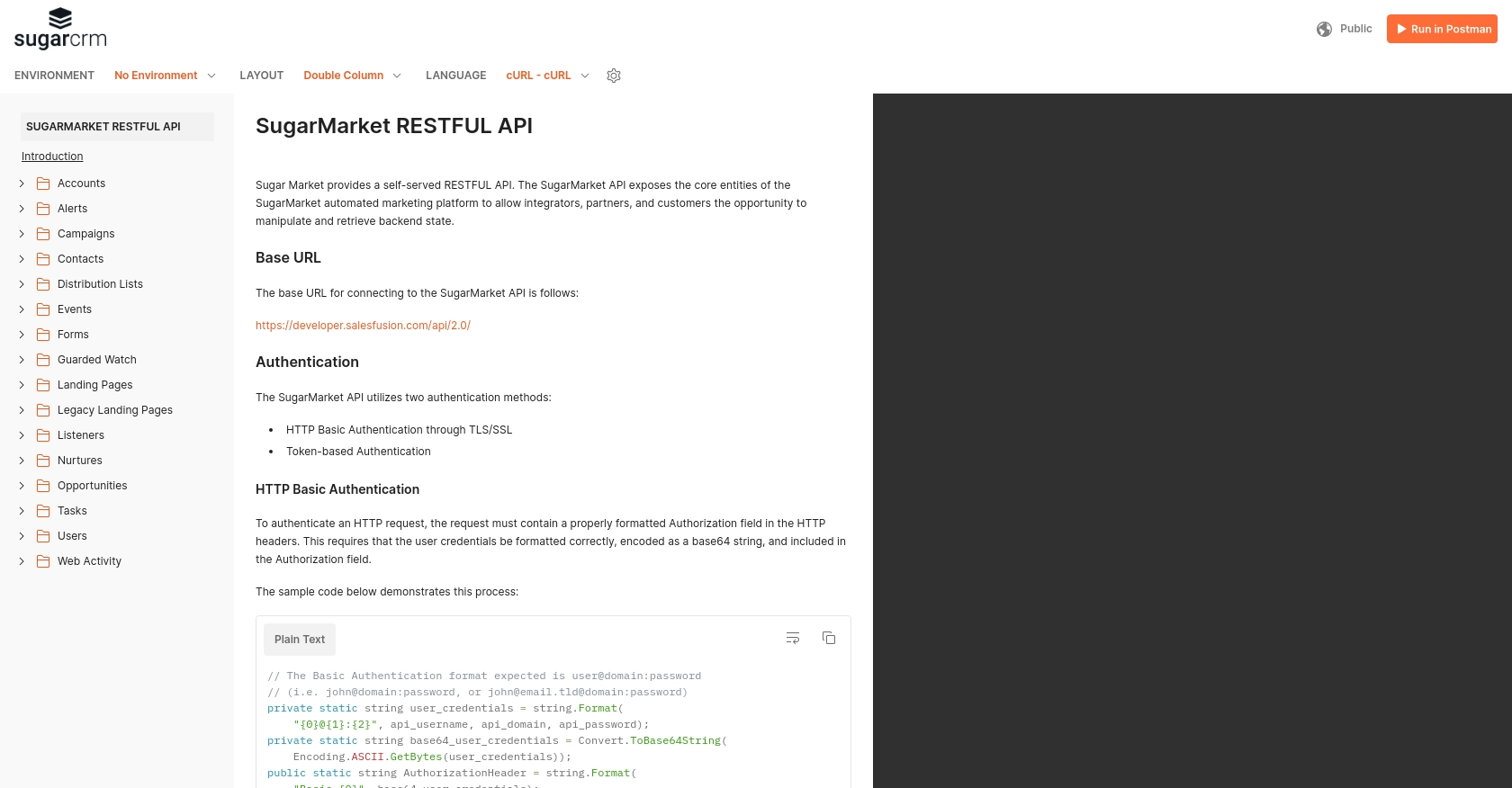
sbb-itb-96038d7
Making API Calls to Retrieve Accounts from Sugar Market Using JavaScript
To interact with the Sugar Market API and retrieve account data using JavaScript, you'll need to ensure your development environment is properly set up. This section will guide you through the necessary steps, including setting up JavaScript, installing dependencies, and executing the API call.
Setting Up Your JavaScript Environment for Sugar Market API
Before making API calls, ensure you have Node.js installed on your machine. Node.js allows you to run JavaScript code outside of a browser, which is essential for server-side operations like API requests.
- Download and install Node.js from the official website.
- Verify the installation by running the following command in your terminal:
node -v
This command should return the version of Node.js installed on your system.
Installing Required Libraries for Sugar Market API Integration
To make HTTP requests in JavaScript, you'll need the Axios library. Install it using npm (Node Package Manager) with the following command:
npm install axios
Axios simplifies the process of making HTTP requests and handling responses, making it ideal for API interactions.
Executing the Sugar Market API Call to Retrieve Accounts
With your environment set up, you can now write the JavaScript code to call the Sugar Market API and retrieve account data. Create a new JavaScript file, get_sugar_market_accounts.js
, and add the following code:
const axios = require('axios');
// Define the API endpoint and headers
const endpoint = 'https://api.sugarmarket.com/v1/accounts';
const headers = {
'Authorization': 'Bearer Your_Access_Token',
'Content-Type': 'application/json'
};
// Function to get accounts from Sugar Market
async function getAccounts() {
try {
const response = await axios.get(endpoint, { headers });
const accounts = response.data;
console.log('Accounts retrieved successfully:', accounts);
} catch (error) {
console.error('Error retrieving accounts:', error.response ? error.response.data : error.message);
}
}
// Execute the function
getAccounts();
Replace Your_Access_Token
with the access token obtained during the authentication setup.
Running the JavaScript Code and Verifying the API Response
To execute the code and retrieve account data, run the following command in your terminal:
node get_sugar_market_accounts.js
If successful, the console will display the retrieved account data. Verify the response by checking the accounts listed in your Sugar Market sandbox account.
Handling Errors and Troubleshooting API Requests
When making API calls, it's crucial to handle potential errors gracefully. The code above includes a try-catch
block to catch and log errors. Common issues may include invalid tokens, incorrect endpoints, or network errors. Refer to the Sugar Market API documentation for detailed error codes and troubleshooting tips.
Best Practices for Using Sugar Market API in JavaScript
When working with the Sugar Market API, it's essential to follow best practices to ensure efficient and secure integration. Here are some key recommendations:
- Securely Store API Credentials: Always store your client ID, client secret, and access tokens securely. Avoid hardcoding them in your source code. Consider using environment variables or a secure vault.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Sugar Market API. Implement logic to handle rate limit responses gracefully, such as retrying requests after a delay.
- Standardize Data Fields: Ensure that data retrieved from the API is transformed and standardized to match your application's data structures. This will facilitate seamless integration and data consistency.
- Implement Error Handling: Use comprehensive error handling to manage potential issues during API calls. Log errors for troubleshooting and provide meaningful feedback to users.
Streamline Integrations with Endgrate
Building and maintaining integrations with multiple platforms can be time-consuming and complex. Endgrate simplifies this process by offering a unified API endpoint that connects to various platforms, including Sugar Market.
With Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the integration complexities.
- Build Once, Use Everywhere: Develop a single integration for multiple platforms, reducing development efforts.
- Enhance User Experience: Provide an intuitive and seamless integration experience for your customers.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?