Using the Moneybird API to Get External Invoices (with Python examples)
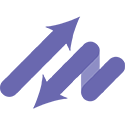
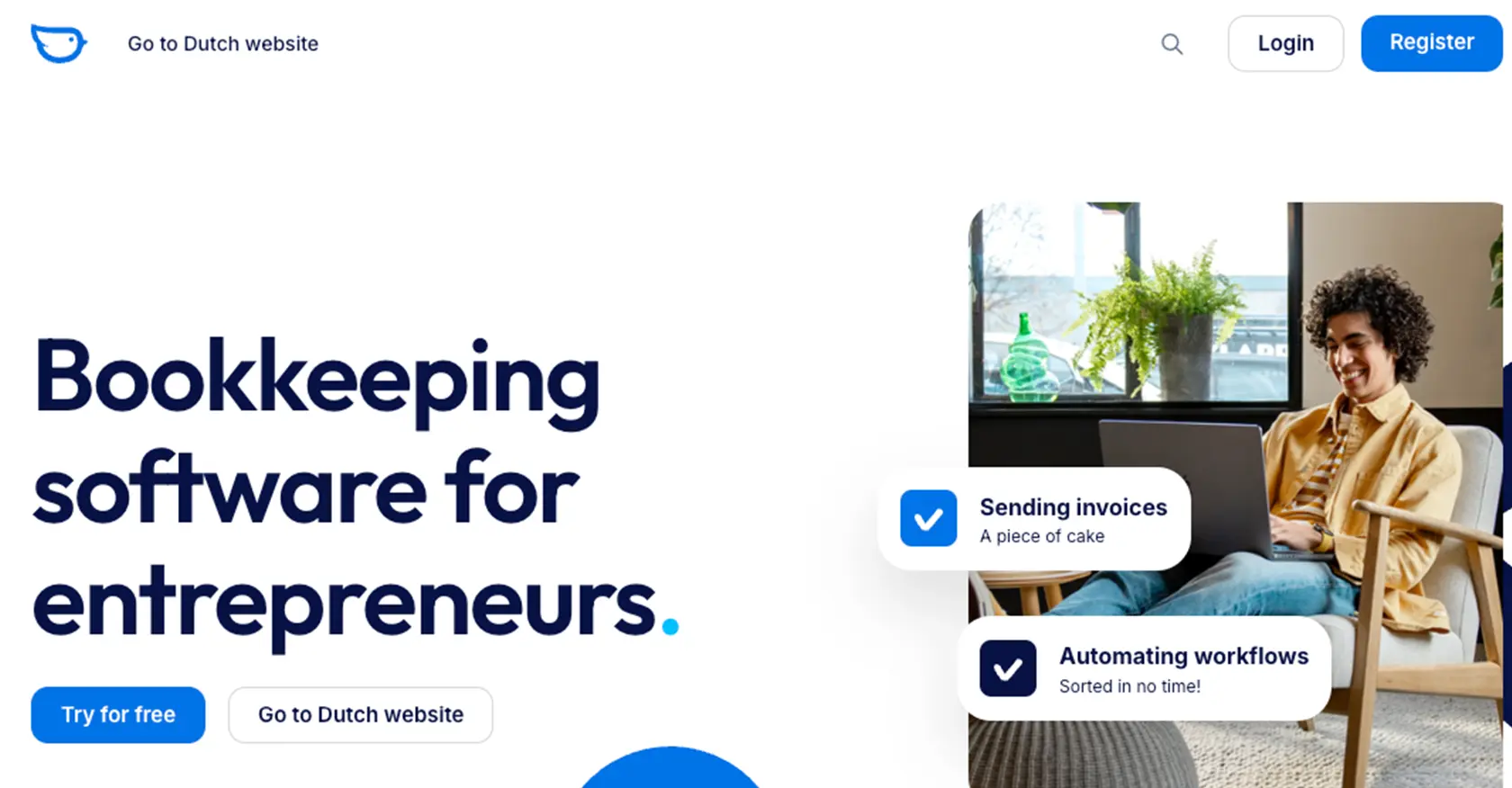
Introduction to Moneybird API for External Invoices
Moneybird is a comprehensive online accounting software designed to simplify financial management for businesses. It offers a range of features including invoicing, expense tracking, and financial reporting, making it a popular choice for small to medium-sized enterprises.
Integrating with the Moneybird API allows developers to automate and streamline financial processes, such as retrieving external invoices. For example, a developer might use the Moneybird API to automatically fetch and analyze external sales invoices, enabling more efficient financial tracking and reporting.
This article will guide you through using Python to interact with the Moneybird API, specifically focusing on retrieving external invoices. By following this tutorial, you'll learn how to set up your environment, authenticate with Moneybird, and execute API calls to manage your financial data effectively.
Setting Up Your Moneybird Sandbox Account for API Integration
Before diving into the Moneybird API, it's essential to set up a sandbox account. This allows you to test your integration without affecting real data. Moneybird offers a sandbox environment that provides full access to its features, ensuring a safe space for development and testing.
Create a Moneybird Account
If you don't already have a Moneybird account, start by registering on the Moneybird website. This will give you access to both the live and sandbox environments.
- Visit the Moneybird website and click on "Sign Up."
- Follow the registration process to create your account.
Access the Moneybird Sandbox Environment
Once your account is set up, you can create a sandbox administration to test your API integration.
- Log in to your Moneybird account.
- Navigate to the sandbox creation page by visiting this link.
- Follow the instructions to create a sandbox administration, which will be used for testing purposes.
Register Your Application for OAuth Authentication
To interact with the Moneybird API, you'll need to register your application and set up OAuth authentication. This process involves obtaining a Client ID and Client Secret.
- Log in to your Moneybird account and visit the application registration page.
- Fill in the necessary details to register your application.
- Upon registration, you'll receive a Client ID and Client Secret. Keep these credentials secure as they are essential for authenticating API requests.
Authorize Your Application with Moneybird
With your application registered, you can now authorize it to access Moneybird's resources using OAuth.
import requests
# Define the authorization URL
auth_url = "https://moneybird.com/oauth/authorize"
# Set your client ID and redirect URI
params = {
"client_id": "your_client_id",
"redirect_uri": "urn:ietf:wg:oauth:2.0:oob",
"response_type": "code"
}
# Redirect the user to the authorization URL
response = requests.get(auth_url, params=params)
print("Visit this URL to authorize the application:", response.url)
After visiting the URL, the user will be prompted to authorize the application. Once authorized, a code will be displayed, which can be exchanged for an access token.
Exchange Authorization Code for Access Token
Use the authorization code to obtain an access token, which will allow you to make API requests on behalf of the user.
import requests
# Define the token URL
token_url = "https://moneybird.com/oauth/token"
# Set the parameters for the token request
data = {
"client_id": "your_client_id",
"client_secret": "your_client_secret",
"code": "authorization_code",
"redirect_uri": "urn:ietf:wg:oauth:2.0:oob",
"grant_type": "authorization_code"
}
# Request the access token
response = requests.post(token_url, data=data)
access_token = response.json().get("access_token")
print("Access Token:", access_token)
Store the access token securely, as it will be used to authenticate your API requests.
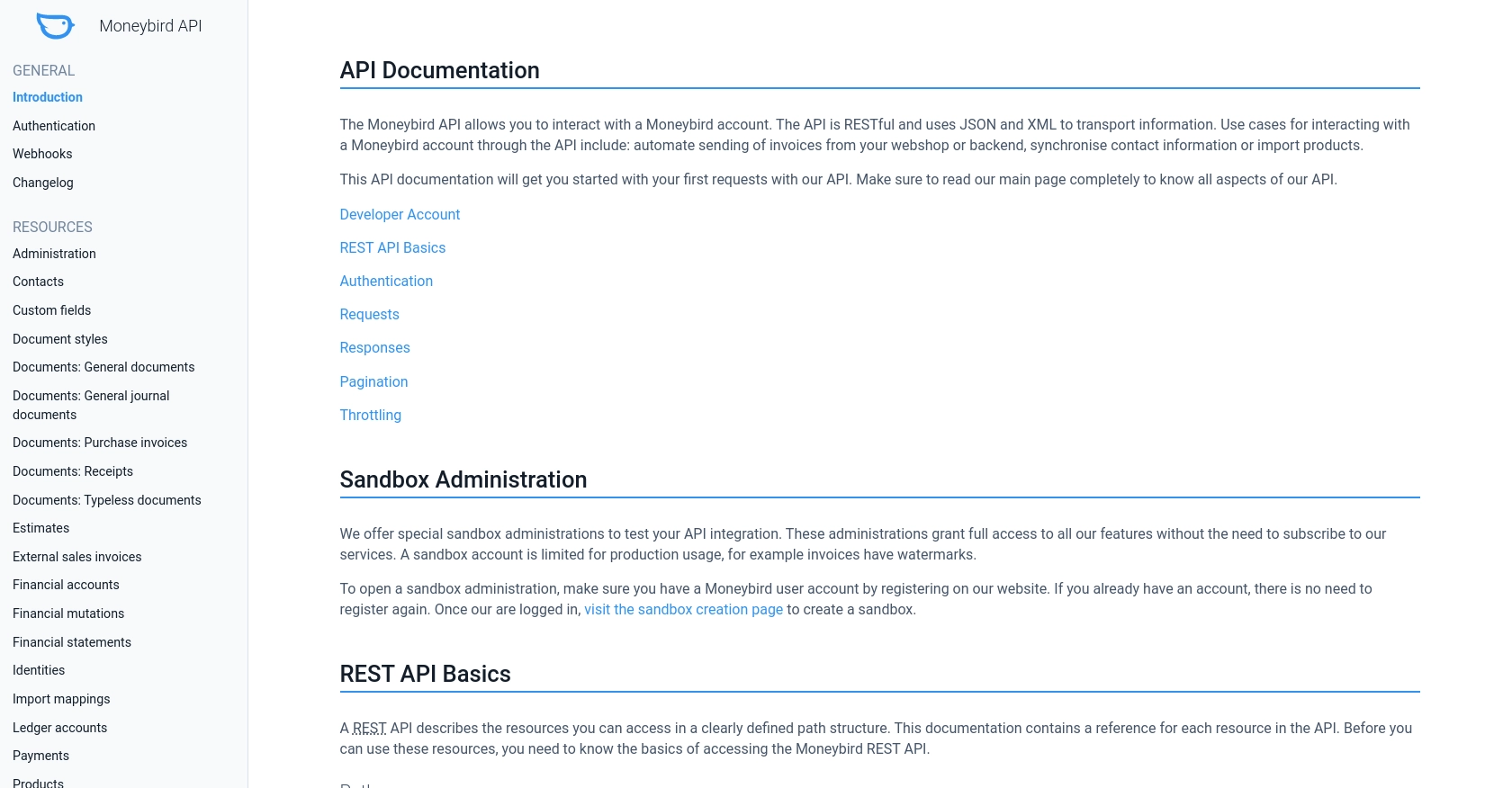
sbb-itb-96038d7
Making API Calls to Retrieve External Invoices from Moneybird Using Python
Now that you have set up your Moneybird sandbox account and obtained your access token, you can proceed to make API calls to retrieve external invoices. This section will guide you through the process of using Python to interact with the Moneybird API, ensuring you can efficiently manage your financial data.
Prerequisites for Python Integration with Moneybird API
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1
- The Python package installer
pip
Install the requests
library, which will be used to make HTTP requests:
pip install requests
Retrieving External Invoices from Moneybird API
To retrieve external invoices, you'll need to make a GET request to the Moneybird API endpoint. Here's how you can do it using Python:
import requests
# Set the API endpoint and headers
administration_id = "your_administration_id"
endpoint = f"https://moneybird.com/api/v2/{administration_id}/external_sales_invoices.json"
headers = {
"Authorization": "Bearer your_access_token",
"Content-Type": "application/json"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
invoices = response.json()
for invoice in invoices:
print(f"Invoice ID: {invoice['id']}, Reference: {invoice['reference']}")
else:
print(f"Failed to retrieve invoices: {response.status_code} - {response.text}")
Replace your_administration_id
and your_access_token
with your actual administration ID and access token. The code above will fetch all external invoices and print their IDs and references.
Handling API Response and Errors
It's crucial to handle API responses and potential errors effectively. The Moneybird API provides various HTTP status codes to indicate the outcome of your requests:
- 200 OK: Request was successful.
- 400 Bad Request: Parameters are missing or malformed.
- 401 Unauthorized: Invalid authorization information.
- 404 Not Found: Entity not found.
- 429 Too Many Requests: Rate limit exceeded. Retry after the specified time.
For more details on error codes, refer to the Moneybird API documentation.
Verifying API Call Success in Moneybird Sandbox
After making the API call, verify the success by checking the returned data against the resources in your Moneybird sandbox account. Ensure the invoices retrieved match those in your sandbox environment.
By following these steps, you can efficiently interact with the Moneybird API to manage external invoices, enhancing your financial processes through automation and integration.
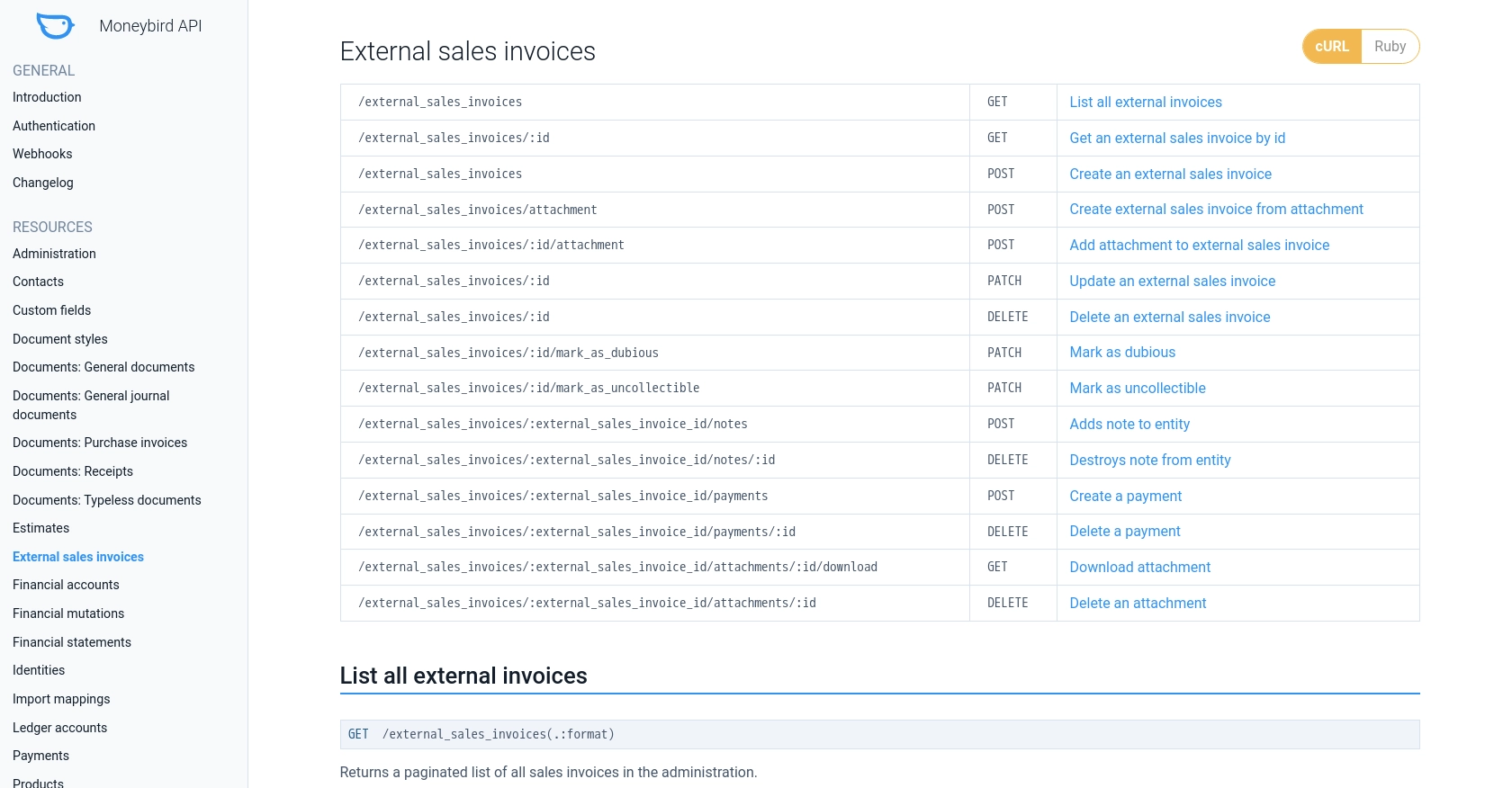
Best Practices for Using the Moneybird API
When integrating with the Moneybird API, it's essential to follow best practices to ensure security, efficiency, and reliability. Here are some key recommendations:
- Securely Store Credentials: Always store your API credentials, such as the Client ID, Client Secret, and access tokens, securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: The Moneybird API allows up to 150 requests every 5 minutes. Implement logic to handle the
429 Too Many Requests
status code by retrying after the specified time. For more details, refer to the Moneybird API documentation. - Data Standardization: Ensure that data fields retrieved from the API are standardized and transformed as needed for your application. This helps maintain consistency and accuracy in your financial records.
- Monitor API Usage: Regularly monitor your API usage and logs to detect any anomalies or unauthorized access attempts. This proactive approach helps maintain the integrity of your integration.
Enhancing Your Integration with Endgrate
While integrating with the Moneybird API can streamline your financial processes, managing multiple integrations can be complex and time-consuming. Endgrate offers a solution by providing a unified API endpoint that connects to various platforms, including Moneybird.
With Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the complexities of multiple integrations.
- Build Once, Use Everywhere: Develop a single integration for each use case and apply it across different platforms effortlessly.
- Enhance Customer Experience: Deliver a seamless and intuitive integration experience to your customers, boosting satisfaction and engagement.
Explore how Endgrate can simplify your integration needs by visiting Endgrate's website and discover the benefits of a unified integration approach.
Read More
Ready to get started?