Using the Outreach API to Create Opportunities in Python
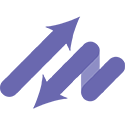
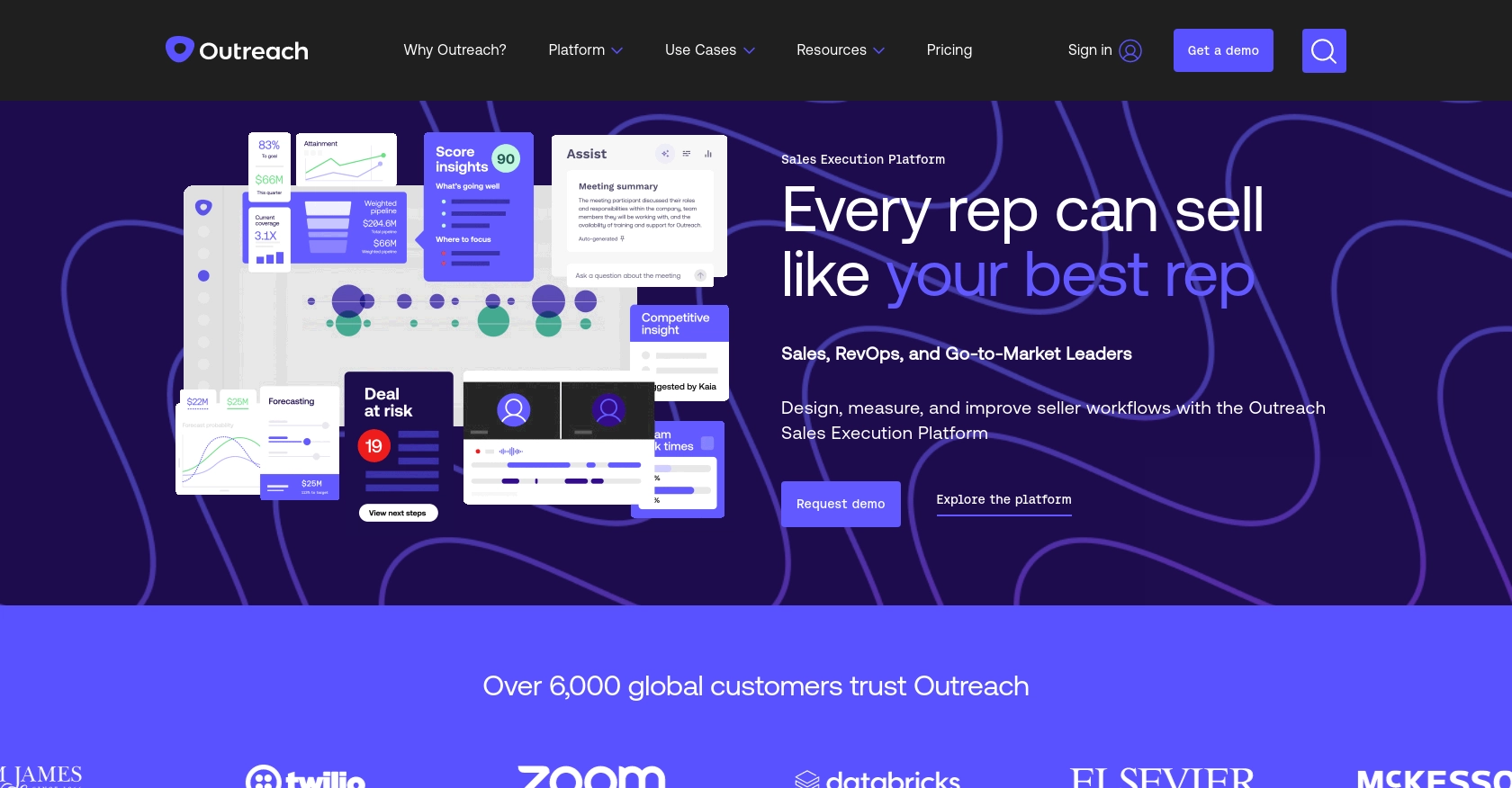
Introduction to Outreach API for Opportunity Management
Outreach is a powerful sales engagement platform that helps businesses streamline their sales processes and improve productivity. With its robust set of tools, Outreach enables sales teams to manage customer relationships, automate workflows, and enhance communication strategies.
Integrating with the Outreach API allows developers to automate and manage sales opportunities efficiently. By leveraging the API, developers can create, update, and track opportunities directly from their applications, enhancing the sales pipeline and improving decision-making processes.
For example, a developer might use the Outreach API to automatically create new sales opportunities based on leads generated from a marketing campaign. This integration ensures that sales teams can quickly act on potential deals, reducing the time from lead generation to conversion.
Setting Up Your Outreach Test/Sandbox Account for API Integration
Before diving into the Outreach API, you'll need to set up a test or sandbox account. This environment allows you to experiment with API calls without affecting live data, ensuring a safe space for development and testing.
Creating an Outreach Sandbox Account
If you don't already have an Outreach account, you can sign up for a free trial or demo account on the Outreach website. This will provide you with access to the necessary tools and features to begin your integration process.
- Visit the Outreach website and navigate to the sign-up page.
- Follow the instructions to create your account, providing the required information.
- Once your account is set up, log in to access the Outreach dashboard.
Configuring OAuth for Outreach API Access
Outreach uses OAuth 2.0 for authentication, which requires setting up an application within your Outreach account. This process will provide you with the client ID and client secret needed for API authorization.
Follow these steps to configure OAuth:
- Log in to your Outreach account and navigate to the "Settings" section.
- Under "Integrations," select "API Access" to begin creating a new application.
- Click on "Create New App" and fill in the necessary details, such as the app name and description.
- Specify the redirect URI(s) that your application will use. Ensure these are properly URL-encoded.
- Select the OAuth scopes that your application requires. Make sure to include scopes relevant to opportunity management.
- Save the application to generate your client ID and client secret. Note that the client secret will only be displayed once, so store it securely.
For more detailed information on setting up OAuth, refer to the Outreach OAuth documentation.
Obtaining and Refreshing Access Tokens
Once your application is set up, you'll need to obtain an access token to authenticate API requests. Here's how to do it:
import requests
# Define the token endpoint and payload
token_url = "https://api.outreach.io/oauth/token"
payload = {
"client_id": "Your_Client_ID",
"client_secret": "Your_Client_Secret",
"redirect_uri": "Your_Redirect_URI",
"grant_type": "authorization_code",
"code": "Authorization_Grant_Code"
}
# Request the access token
response = requests.post(token_url, data=payload)
tokens = response.json()
# Extract the access token
access_token = tokens['access_token']
print("Access Token:", access_token)
Remember to replace placeholders with your actual client ID, client secret, redirect URI, and authorization grant code. The access token will expire after a set period, so use the refresh token to obtain a new one as needed.
For more details on token management, see the Outreach API Getting Started Guide.
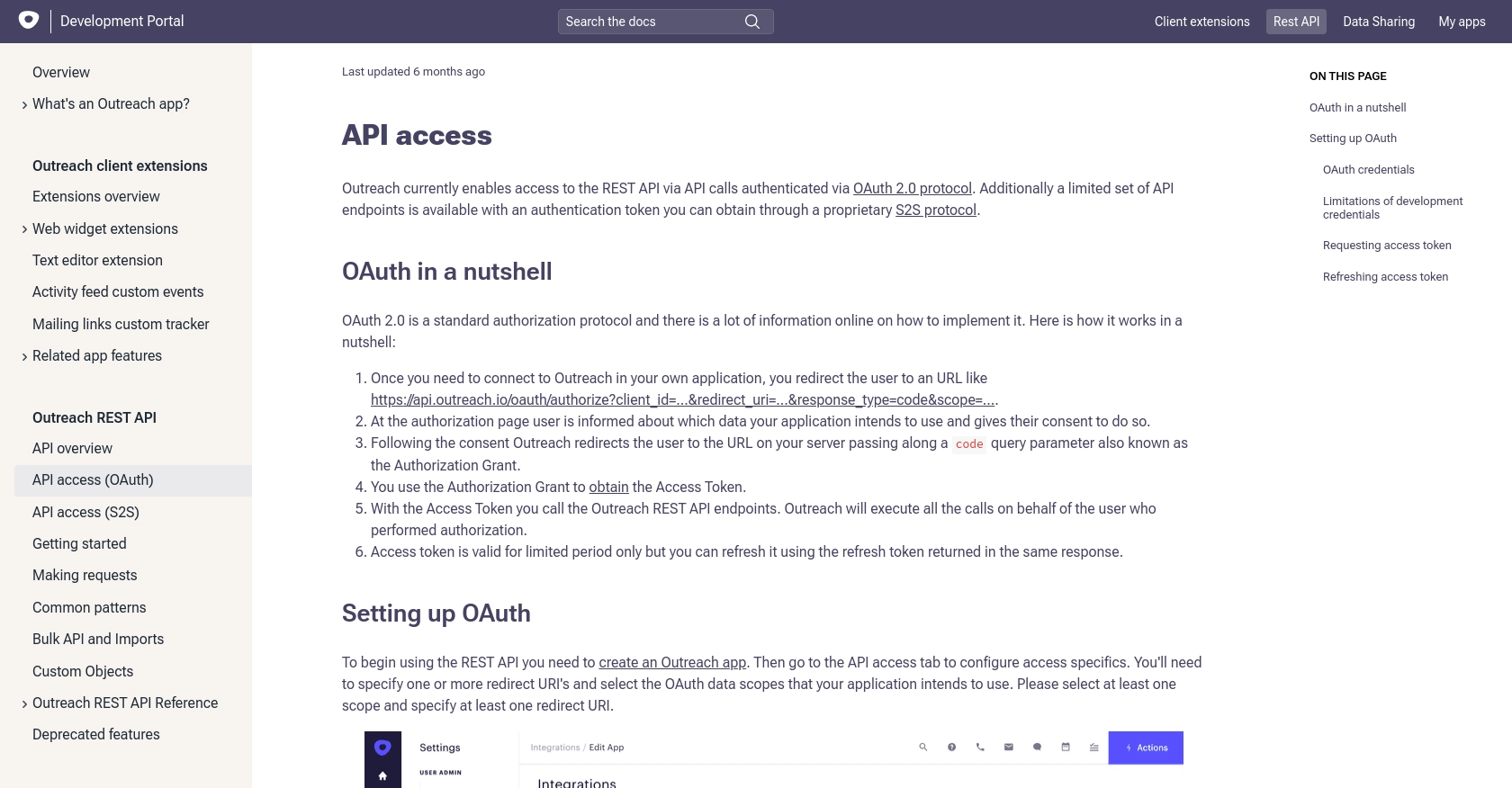
sbb-itb-96038d7
Making API Calls to Create Opportunities with Outreach in Python
To interact with the Outreach API and create opportunities, you'll need to use Python, a versatile and widely-used programming language. This section will guide you through setting up your Python environment and making API calls to manage opportunities in Outreach.
Setting Up Your Python Environment for Outreach API Integration
Before making API calls, ensure you have the necessary tools and libraries installed. You'll need Python 3.x and the requests
library to handle HTTP requests.
- Ensure Python 3.x is installed on your machine. You can download it from the official Python website.
- Install the
requests
library using pip:
pip install requests
Creating an Opportunity in Outreach Using Python
With your environment set up, you can now create an opportunity in Outreach using the API. Follow these steps to make the API call:
import requests
# Define the API endpoint and headers
url = "https://api.outreach.io/api/v2/opportunities"
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/vnd.api+json"
}
# Define the opportunity data
data = {
"data": {
"attributes": {
"name": "New Opportunity",
"amount": 10000,
"currencyType": "USD",
"closeDate": "2023-12-31"
},
"relationships": {
"account": {
"data": {
"id": "12345",
"type": "account"
}
}
},
"type": "opportunity"
}
}
# Make the POST request to create the opportunity
response = requests.post(url, json=data, headers=headers)
# Check if the request was successful
if response.status_code == 201:
print("Opportunity created successfully:", response.json())
else:
print("Failed to create opportunity:", response.status_code, response.json())
Replace Your_Access_Token
with the access token obtained earlier. The example above creates a new opportunity with specified attributes such as name, amount, and close date. Adjust these fields as needed for your use case.
Verifying the API Call and Handling Errors
After making the API call, verify that the opportunity was created successfully by checking the response. A successful creation will return a status code of 201. If the request fails, the response will include error details.
Common error codes include:
- 401 Unauthorized: Check your access token and ensure it is valid.
- 422 Unprocessable Entity: Verify that all required fields are correctly formatted and included.
For more detailed error handling, refer to the Outreach API documentation.
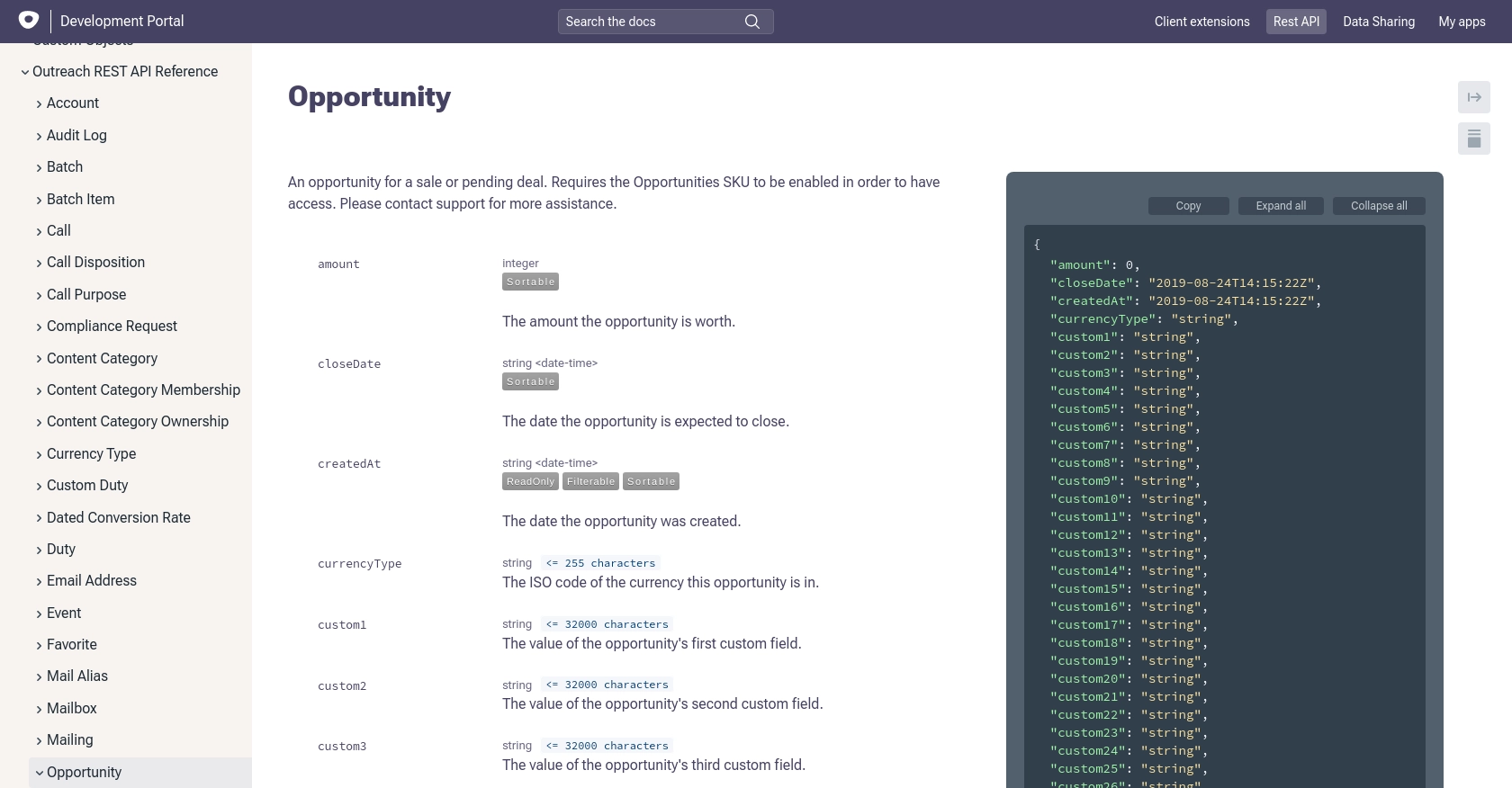
Conclusion and Best Practices for Using Outreach API in Python
Integrating with the Outreach API to create opportunities in Python can significantly enhance your sales processes by automating and streamlining tasks. By following the steps outlined in this guide, you can efficiently manage sales opportunities and improve your team's productivity.
Best Practices for Secure and Efficient API Integration with Outreach
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limiting: The Outreach API has a rate limit of 10,000 requests per hour. Implement logic to handle rate limit responses and retry requests after the specified time. For more details, refer to the Outreach API documentation.
- Refresh Tokens Regularly: Access tokens expire after two hours. Use the refresh token to obtain new access tokens and ensure continuous API access.
- Validate API Responses: Always check the status codes and response data to ensure successful API interactions. Implement error handling to manage unexpected responses.
- Optimize Data Handling: Standardize and transform data fields as needed to align with your application's requirements and ensure consistency across integrations.
Enhance Your Integration Strategy with Endgrate
While integrating with the Outreach API can be highly beneficial, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process by providing a single endpoint to connect with multiple platforms, including Outreach.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. Build once for each use case and enjoy an intuitive integration experience for your customers. Explore how Endgrate can streamline your integration strategy by visiting Endgrate.
Read More
Ready to get started?