Using the Keap API to Get Contacts in PHP
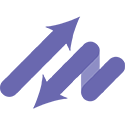
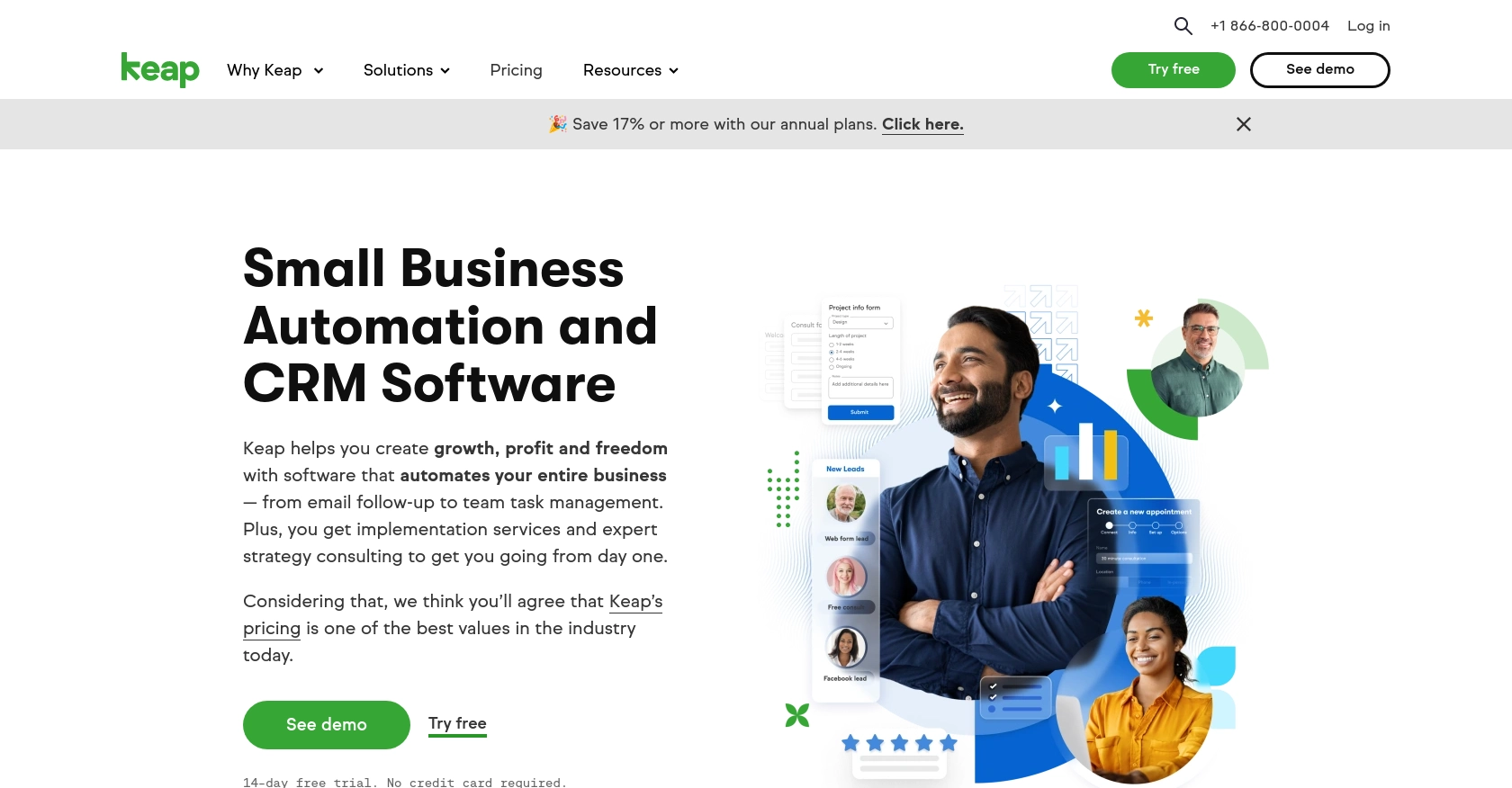
Introduction to Keap CRM
Keap, formerly known as Infusionsoft, is a powerful CRM platform designed to help small businesses manage customer relationships, automate marketing, and streamline sales processes. With its robust set of tools, Keap enables businesses to nurture leads, track customer interactions, and enhance overall productivity.
Developers may want to integrate with Keap's API to access and manage customer data, such as contacts, to improve business workflows and customer engagement. For example, a developer could use the Keap API to retrieve contact information and synchronize it with other business applications, ensuring that customer data is always up-to-date across platforms.
Setting Up Your Keap Developer Account and Sandbox Environment
Before you can start interacting with the Keap API, you'll need to set up a developer account and create a sandbox environment. This will allow you to test your integrations without affecting live data.
Register for a Keap Developer Account
To begin, you'll need to register for a Keap developer account. This account will give you access to the necessary tools and resources to build and test your integrations.
- Visit the Keap Developer Portal.
- Click on the "Register" button to create a new account.
- Fill in the required information, including your name, email, and company details.
- Submit the form to complete your registration.
Create a Keap Sandbox App
Once your developer account is set up, the next step is to create a sandbox app. This will provide you with a safe environment to test your API calls.
- Log in to your Keap developer account.
- Navigate to the "Apps" section and click on "Create a New App."
- Provide a name and description for your sandbox app.
- Click "Create" to generate your sandbox app.
Set Up OAuth Authentication for Keap API
The Keap API uses OAuth for authentication, which requires you to create an app to obtain the necessary credentials.
- In your sandbox app, navigate to the "API Keys" section.
- Click on "Create API Key" to generate your client ID and client secret.
- Note down the client ID and client secret, as you'll need these for authentication.
With your developer account, sandbox app, and OAuth credentials set up, you're ready to start making API calls to Keap. This setup ensures that you can safely test your integrations and manage customer data effectively.
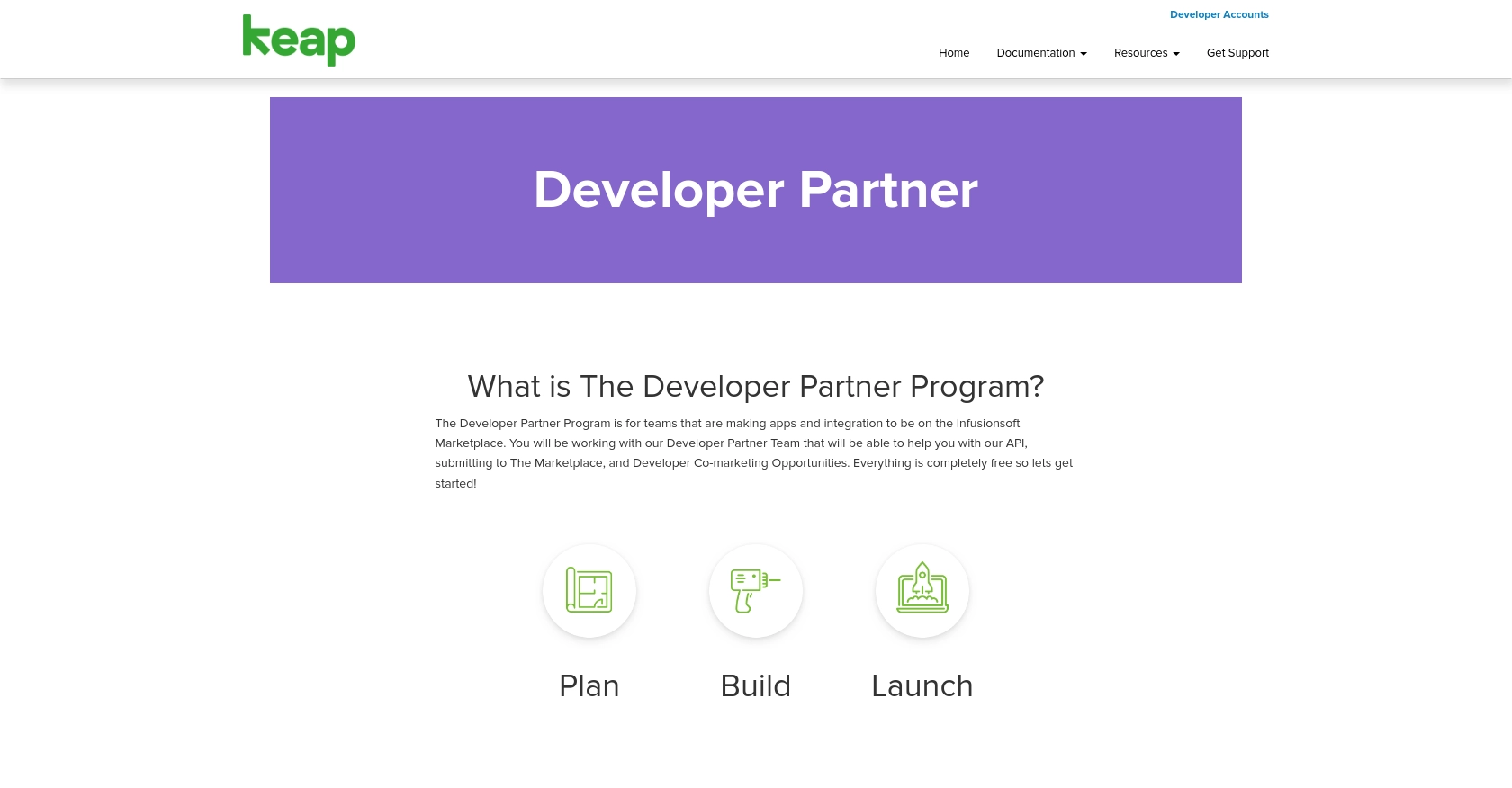
sbb-itb-96038d7
Making API Calls to Retrieve Contacts from Keap Using PHP
To interact with the Keap API and retrieve contact information, you'll need to use PHP, a popular server-side scripting language. This section will guide you through the process of setting up your PHP environment, making the API call, and handling the response.
Setting Up Your PHP Environment for Keap API Integration
Before making API calls, ensure your development environment is ready. You'll need:
- PHP 7.4 or higher
- Composer for dependency management
Install the necessary dependencies using Composer. Run the following command to install the Guzzle HTTP client, which simplifies making HTTP requests:
composer require guzzlehttp/guzzle
Writing PHP Code to Make Keap API Calls
With your environment set up, you can now write the PHP code to retrieve contacts from Keap. Create a file named get_keap_contacts.php
and add the following code:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
// Set the API endpoint and headers
$endpoint = 'https://api.infusionsoft.com/crm/rest/v1/contacts';
$headers = [
'Authorization' => 'Bearer YOUR_ACCESS_TOKEN',
'Accept' => 'application/json'
];
try {
// Make a GET request to the API
$response = $client->request('GET', $endpoint, ['headers' => $headers]);
// Parse the JSON data from the response
$data = json_decode($response->getBody(), true);
// Loop through the contacts and print their information
foreach ($data['contacts'] as $contact) {
echo 'Name: ' . $contact['given_name'] . ' ' . $contact['family_name'] . '<br>';
echo 'Email: ' . $contact['email_addresses'][0]['email'] . '<br><br>';
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Replace YOUR_ACCESS_TOKEN
with the access token obtained from the OAuth authentication process.
Running the PHP Script to Fetch Contacts from Keap
Execute the script from the command line or a web server:
php get_keap_contacts.php
If successful, the script will output the contact names and emails from your Keap sandbox environment.
Handling Errors and Verifying API Call Success
To ensure your API call is successful, check the HTTP status code returned by the API. A status code of 200 indicates success, while other codes may indicate errors. Handle exceptions in your code to manage errors gracefully.
Verify the retrieved contacts by cross-referencing them with the data in your Keap sandbox account. This ensures the API call is fetching the correct information.
Conclusion and Best Practices for Using Keap API with PHP
Integrating with the Keap API using PHP allows developers to efficiently manage and synchronize customer data across various platforms. By following the steps outlined in this guide, you can set up a robust integration that enhances business workflows and customer engagement.
Best Practices for Keap API Integration
- Securely Store Credentials: Always store your OAuth credentials, such as client ID and client secret, securely. Use environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be aware of Keap's rate limits to avoid exceeding the allowed number of requests. Implement exponential backoff strategies to handle rate limit errors gracefully.
- Standardize Data Fields: Ensure that data retrieved from Keap is transformed and standardized to match the format required by your application or other integrated systems.
- Error Handling: Implement robust error handling to manage exceptions and API errors. Log errors for debugging and provide meaningful feedback to users.
Streamline Your Integrations with Endgrate
While integrating with Keap API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product development.
With Endgrate, you can build once for each use case and leverage a single API endpoint to connect with multiple platforms, including Keap. This not only saves time and resources but also provides an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
Ready to get started?