Using the Confluence API to Get Space Pages (with Javascript examples)
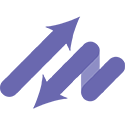
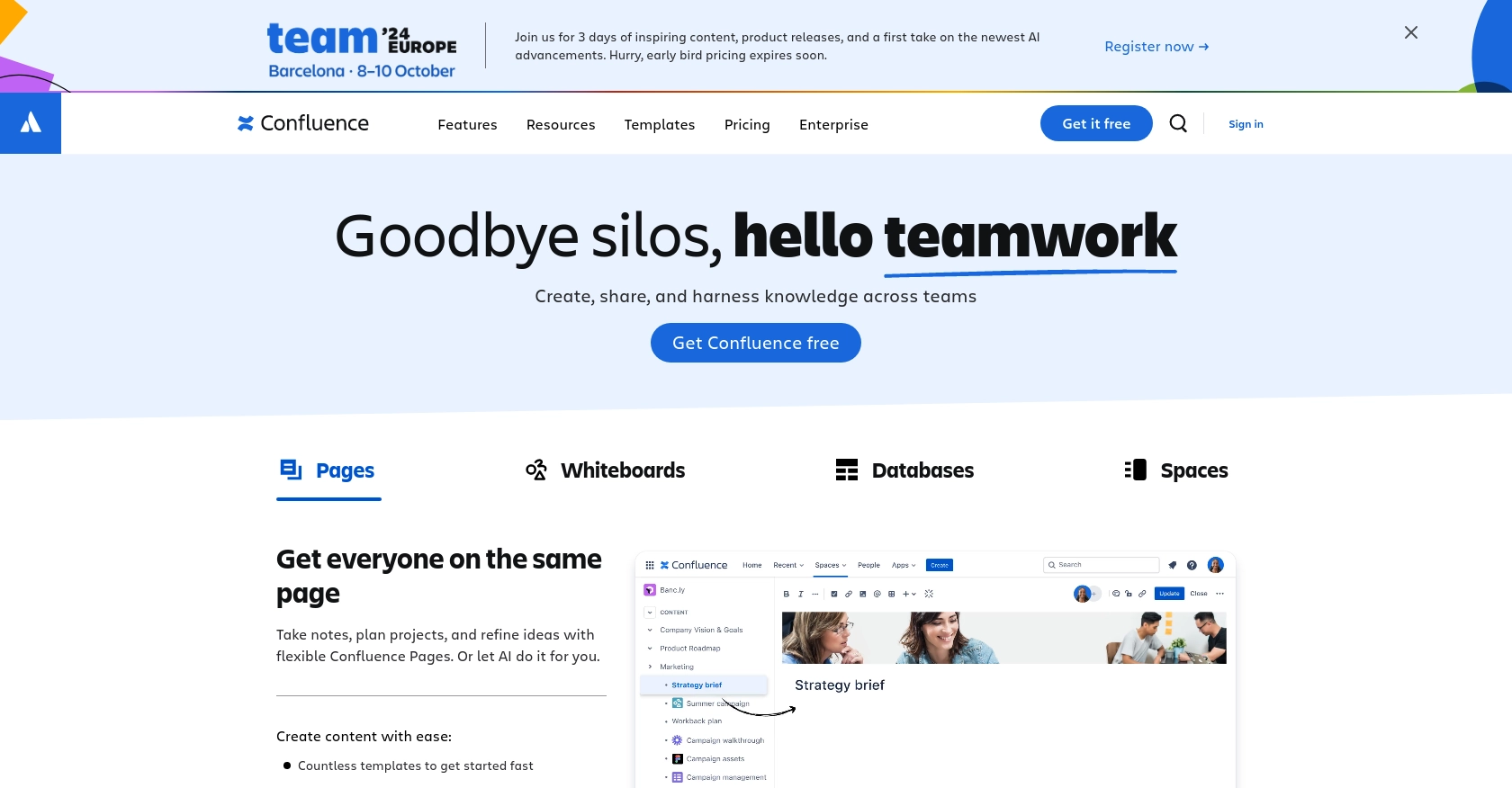
Introduction to Confluence API Integration
Confluence, developed by Atlassian, is a powerful collaboration tool used by teams to create, share, and manage content seamlessly. It serves as a centralized hub for documentation, project planning, and knowledge sharing, making it an essential tool for businesses aiming to enhance productivity and communication.
Integrating with the Confluence API allows developers to automate and streamline content management tasks. For example, you might want to retrieve all pages within a specific space to generate reports or synchronize content with another system. This capability can significantly enhance workflow efficiency and ensure that team members have access to the most up-to-date information.
Setting Up a Confluence Test or Sandbox Account
Before you can start interacting with the Confluence API, it's essential to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting your live data. Here's how you can get started:
Creating a Confluence Sandbox Account
To begin, you'll need a Confluence account. If you don't have one, you can sign up for a free trial on the Atlassian website. This trial will give you access to Confluence Cloud, where you can test API interactions.
- Visit the Confluence sign-up page.
- Follow the instructions to create your account. You'll receive a confirmation email to verify your account.
- Once verified, log in to your Confluence dashboard.
Setting Up OAuth 2.0 for Confluence API Access
Confluence API uses OAuth 2.0 for authentication, ensuring secure access to your data. Follow these steps to set up OAuth 2.0:
- Navigate to the Atlassian Developer Console and log in with your Atlassian account.
- Create a new app by clicking on Create App. Provide the necessary details such as app name and description.
- Once the app is created, go to the Authorization section and select OAuth 2.0.
- Generate your Client ID and Client Secret. These credentials will be used to authenticate API requests.
- Set the necessary scopes for your app. For accessing pages, ensure you include the
read:page:confluence
scope.
Generating an Access Token
With your OAuth 2.0 credentials, you can now generate an access token to authenticate your API requests:
// Example using Node.js to generate an access token
const axios = require('axios');
async function getAccessToken() {
const response = await axios.post('https://auth.atlassian.com/oauth/token', {
grant_type: 'client_credentials',
client_id: 'Your_Client_ID',
client_secret: 'Your_Client_Secret',
scope: 'read:page:confluence'
});
return response.data.access_token;
}
getAccessToken().then(token => console.log('Access Token:', token));
Replace Your_Client_ID
and Your_Client_Secret
with the credentials you generated earlier. This script will return an access token that you can use in your API calls.
With your Confluence sandbox account and OAuth 2.0 setup, you're ready to start making API requests to retrieve space pages. This setup ensures that your interactions with the Confluence API are secure and efficient.
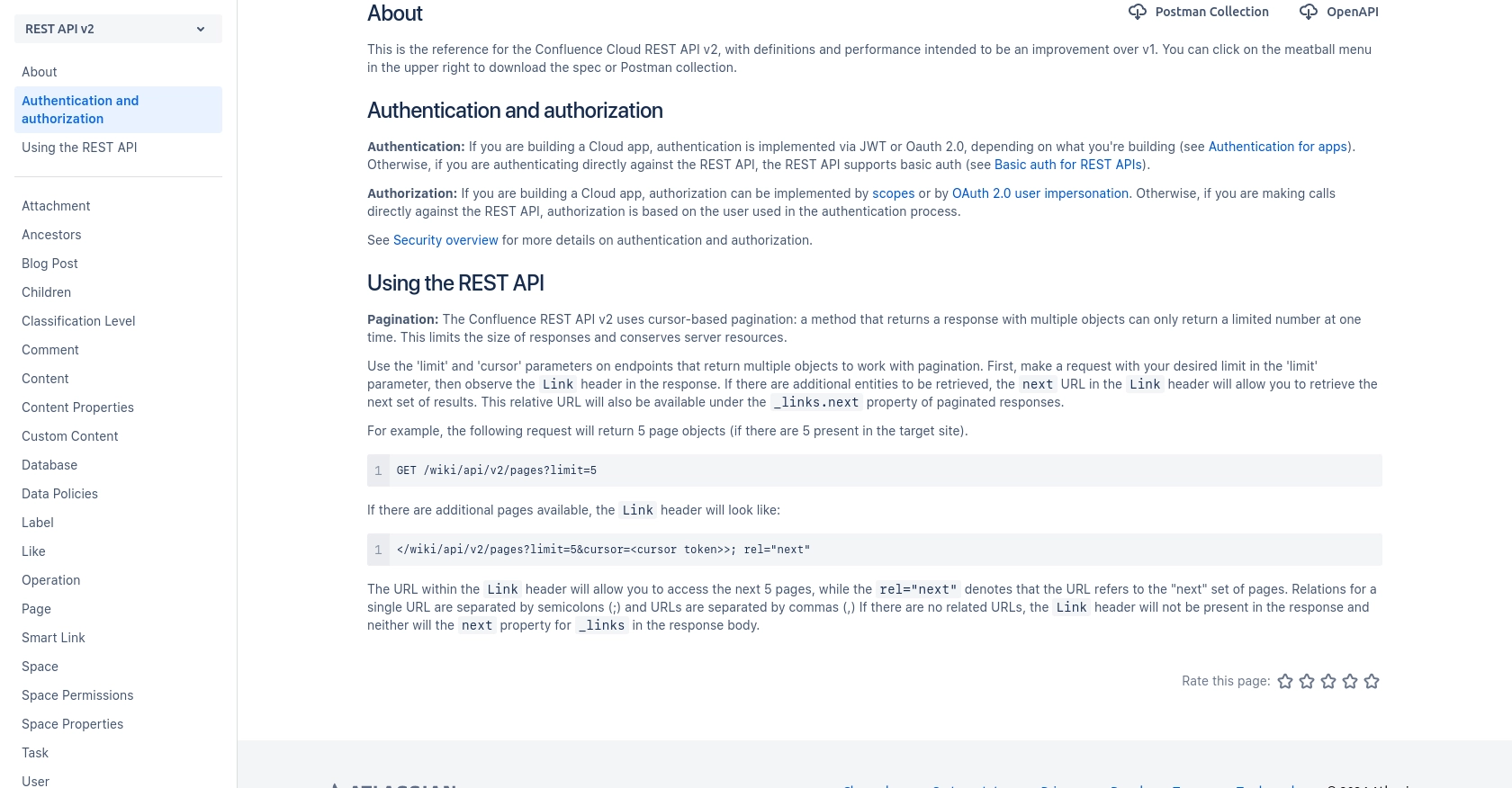
sbb-itb-96038d7
Making API Calls to Retrieve Confluence Space Pages Using JavaScript
Now that you have set up your Confluence sandbox account and obtained an access token, it's time to make API calls to retrieve pages within a specific space. This section will guide you through the process using JavaScript, ensuring you can efficiently interact with the Confluence API.
Prerequisites for JavaScript API Integration with Confluence
Before proceeding, ensure you have the following installed on your machine:
- Node.js (version 14 or higher)
- npm (Node Package Manager)
Additionally, you'll need the axios
library to make HTTP requests. Install it using the following command:
npm install axios
Example Code to Retrieve Confluence Space Pages
Below is a JavaScript example demonstrating how to retrieve pages from a specific Confluence space using the Confluence API:
const axios = require('axios');
async function getConfluencePages(spaceId) {
try {
const response = await axios.get(`https://your-domain.atlassian.net/wiki/api/v2/spaces/${spaceId}/pages`, {
headers: {
'Authorization': 'Bearer Your_Access_Token',
'Accept': 'application/json'
}
});
const pages = response.data.results;
pages.forEach(page => {
console.log(`Page ID: ${page.id}, Title: ${page.title}`);
});
} catch (error) {
console.error('Error fetching pages:', error.response ? error.response.data : error.message);
}
}
getConfluencePages('Your_Space_ID');
Replace Your_Access_Token
with the token you generated earlier and Your_Space_ID
with the ID of the space you wish to query. This script will output the ID and title of each page in the specified space.
Verifying API Call Success in Confluence
To verify that your API call was successful, check the console output for the list of pages. You can also log in to your Confluence dashboard and navigate to the specified space to ensure the pages match the retrieved data.
Handling Errors and Understanding Confluence API Error Codes
When making API calls, it's crucial to handle potential errors gracefully. The Confluence API may return various error codes, such as:
- 400 Bad Request: The request was invalid or cannot be served.
- 401 Unauthorized: Authentication failed or user does not have permissions.
- 404 Not Found: The requested resource could not be found.
Ensure your error handling logic captures these scenarios to provide meaningful feedback and troubleshooting information.
For more information on error codes, refer to the Confluence API documentation.
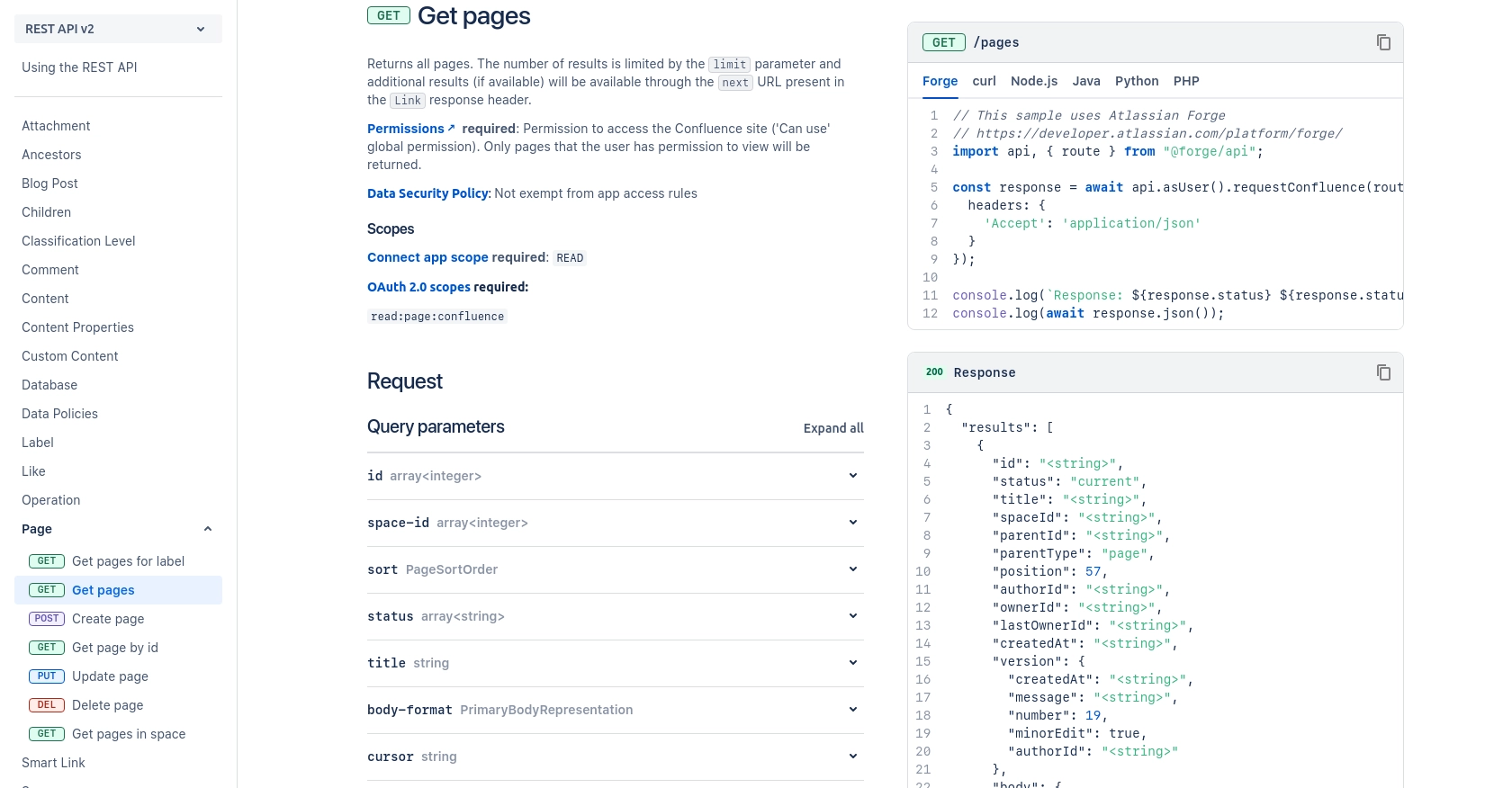
Best Practices for Using Confluence API in JavaScript
When working with the Confluence API, it's essential to follow best practices to ensure secure and efficient integration. Here are some recommendations:
- Securely Store Credentials: Always store your OAuth 2.0 credentials securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of Confluence API's rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Standardize Data Fields: When synchronizing data between Confluence and other systems, ensure consistent data formatting and field mapping to maintain data integrity.
Enhance Your Integration Strategy with Endgrate
Integrating with multiple APIs can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Confluence. By leveraging Endgrate, you can:
- Save Time and Resources: Focus on your core product development while Endgrate handles the intricacies of API integrations.
- Build Once, Use Everywhere: Develop a single integration solution that works across multiple platforms, reducing redundancy and maintenance efforts.
- Deliver a Seamless Experience: Offer your customers an intuitive and efficient integration experience with minimal effort.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?