How to Get Users with the Teamwork CRM API in Javascript
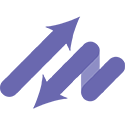
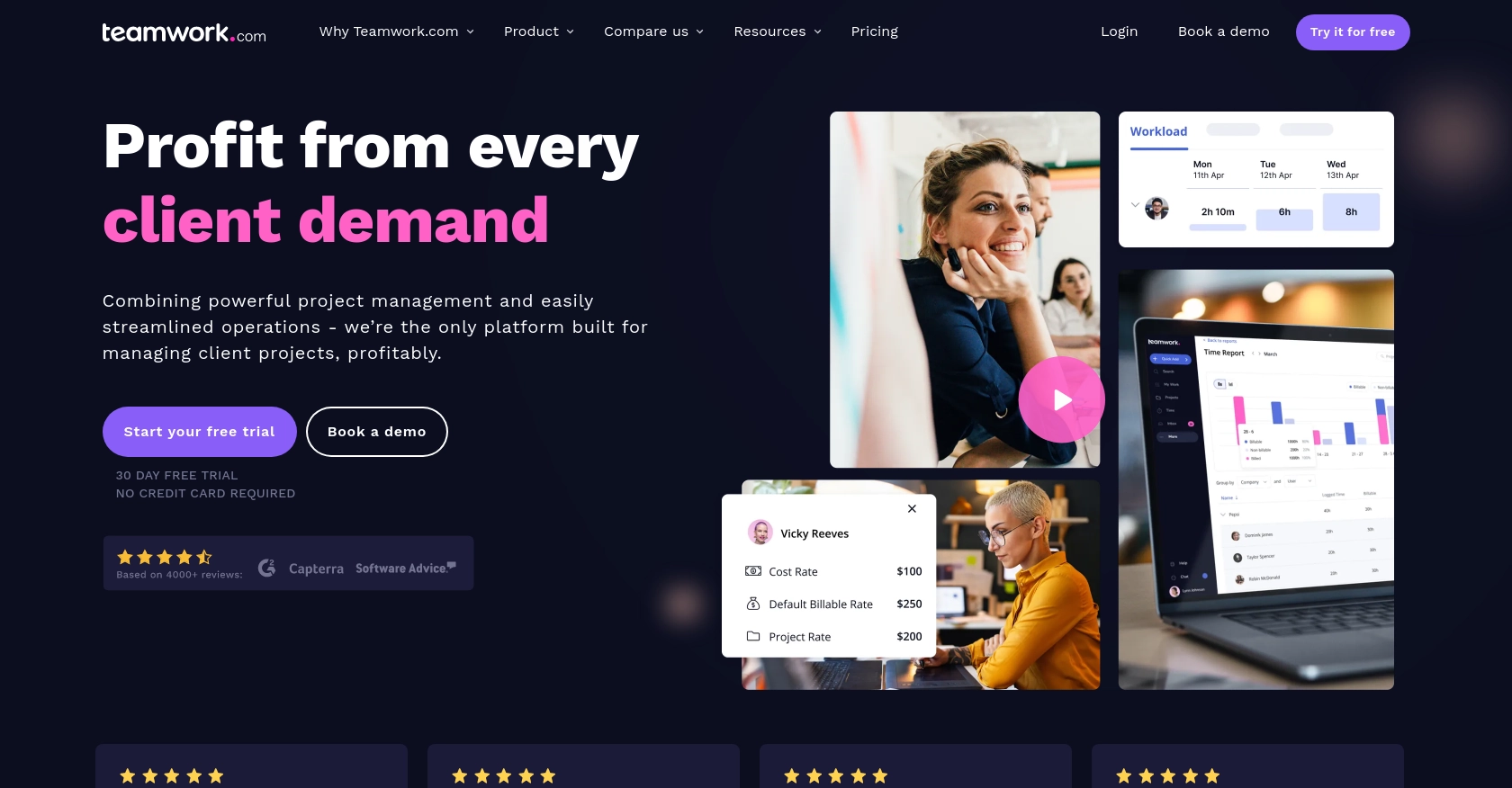
Introduction to Teamwork CRM API Integration
Teamwork CRM is a powerful customer relationship management tool designed to help businesses manage their sales pipelines, track leads, and enhance customer interactions. With its robust features and user-friendly interface, Teamwork CRM is an ideal choice for businesses looking to streamline their sales processes and improve team collaboration.
Integrating with the Teamwork CRM API allows developers to automate and enhance various CRM functionalities, such as retrieving user data. For example, a developer might want to access user information to synchronize it with another application or to generate detailed sales reports. This integration can significantly improve efficiency and data accuracy across platforms.
Setting Up a Teamwork CRM Test Account for API Integration
Before you can start interacting with the Teamwork CRM API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Follow these steps to get started:
Create a Teamwork CRM Account
- Visit the Teamwork CRM website and sign up for a free trial or demo account.
- Follow the on-screen instructions to complete the registration process.
- Once your account is created, log in to the Teamwork CRM dashboard.
Generate API Credentials
To authenticate your API requests, you'll need to generate API credentials. Teamwork CRM supports both Basic Authentication and OAuth 2.0. For this guide, we'll focus on Basic Authentication:
- Navigate to the API section within your Teamwork CRM account settings.
- Locate the option to generate an API key. This key will be used to authenticate your API requests.
- Copy the API key and store it securely, as you'll need it for making API calls.
Configure OAuth 2.0 (Optional)
If you prefer using OAuth 2.0 for authentication, follow these steps:
- Access the Developer Portal from your Teamwork CRM account.
- Register your application to obtain a client ID and client secret.
- Implement the OAuth 2.0 App Login Flow to acquire a bearer token.
- Use the bearer token in the Authorization header for your API requests.
For more detailed information on authentication methods, refer to the Teamwork CRM authentication documentation.
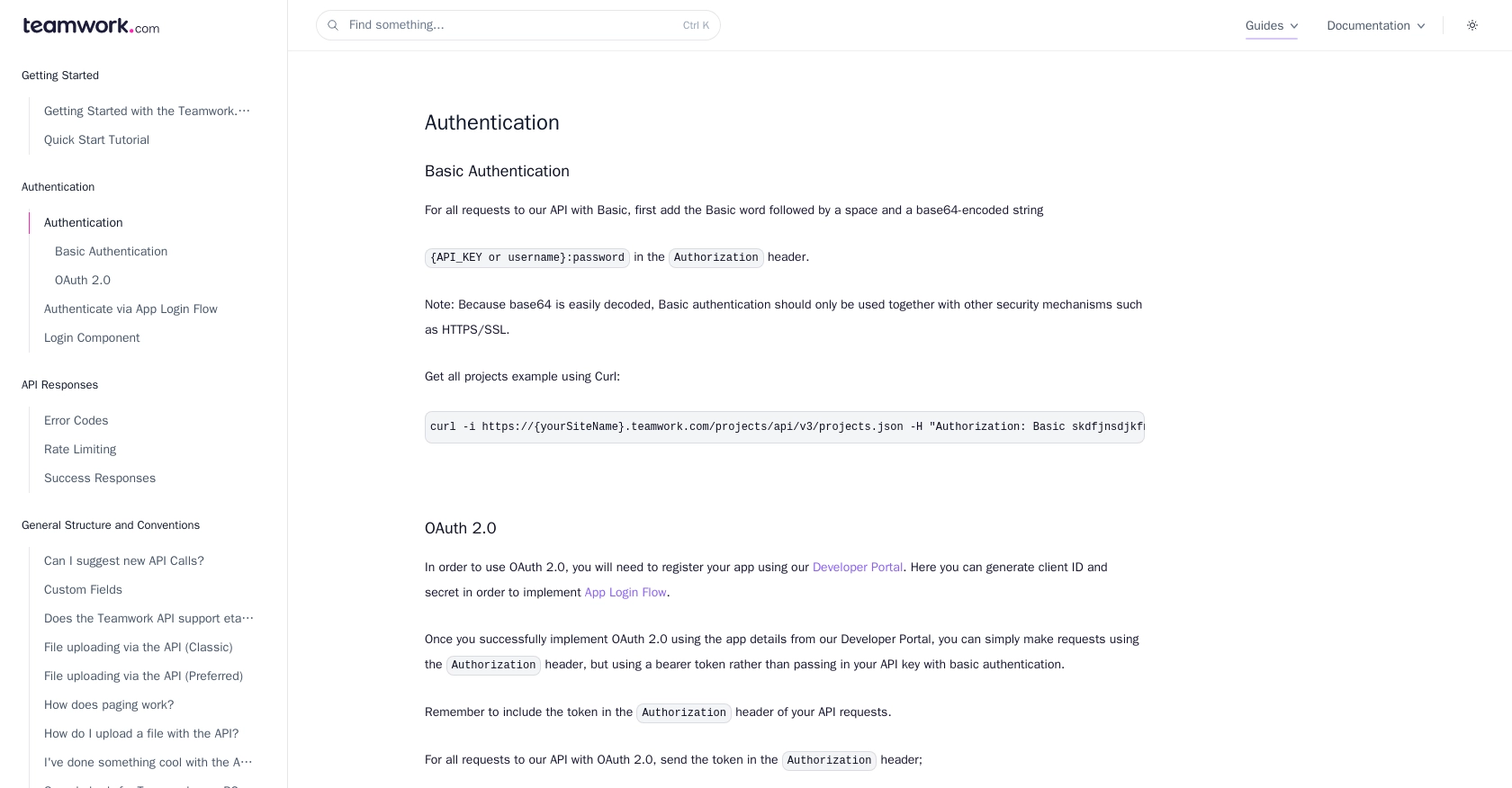
sbb-itb-96038d7
Making API Calls to Retrieve Users with Teamwork CRM in JavaScript
To interact with the Teamwork CRM API and retrieve user data, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the necessary code, and handling responses effectively.
Setting Up Your JavaScript Environment for Teamwork CRM API Integration
Before making API calls, ensure you have a working JavaScript environment. You can use Node.js or a browser-based environment. For this guide, we'll use Node.js. Make sure you have Node.js installed on your machine.
- Download and install Node.js if you haven't already.
- Initialize a new Node.js project by running
npm init -y
in your terminal. - Install the
axios
library to simplify HTTP requests by runningnpm install axios
.
Writing JavaScript Code to Retrieve Users from Teamwork CRM
Now that your environment is set up, you can write the JavaScript code to make an API call to Teamwork CRM and retrieve user data. Here's a step-by-step guide:
const axios = require('axios');
// Define the API endpoint and your API key
const apiEndpoint = 'https://{yourSiteName}.teamwork.com/crm/api/v1/users.json';
const apiKey = 'Your_API_Key';
// Create a function to get users
async function getUsers() {
try {
const response = await axios.get(apiEndpoint, {
headers: {
'Authorization': `Basic ${Buffer.from(apiKey + ':').toString('base64')}`
}
});
// Log the user data
console.log(response.data);
} catch (error) {
console.error('Error fetching users:', error.response ? error.response.data : error.message);
}
}
// Call the function to retrieve users
getUsers();
In this code:
- We use the
axios
library to make HTTP GET requests to the Teamwork CRM API endpoint. - The API key is base64-encoded for Basic Authentication, as required by Teamwork CRM.
- The
getUsers
function fetches user data and logs it to the console. - Error handling is implemented to catch and display any issues during the API call.
Verifying Successful API Requests and Handling Errors
After running your code, you should see the user data printed in your console. If the request is successful, you'll receive a 200 OK status code. If there's an error, refer to the following common error codes:
- 401 Unauthorized: Check your API key and ensure it's correctly set up.
- 404 Not Found: Verify the API endpoint URL.
- 429 Too Many Requests: You have exceeded the rate limit of 150 requests per minute. Wait a while before retrying.
For more details on error codes, refer to the Teamwork CRM error codes documentation.
Conclusion and Best Practices for Teamwork CRM API Integration
Integrating with the Teamwork CRM API using JavaScript can significantly enhance your application's capabilities by automating CRM functionalities and improving data synchronization. By following the steps outlined in this guide, you can efficiently retrieve user data and handle API responses effectively.
Best Practices for Secure and Efficient API Usage
- Secure API Credentials: Always store your API keys and tokens securely. Avoid hardcoding them in your source code. Consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of the rate limit of 150 requests per minute. Implement retry logic with exponential backoff to handle HTTP 429 errors gracefully. For more details, refer to the Teamwork CRM rate limiting documentation.
- Data Transformation: Standardize and transform data fields as needed to ensure consistency across different platforms and applications.
- Error Handling: Implement comprehensive error handling to manage different HTTP status codes and provide meaningful feedback to users or logs.
Enhance Your Integration Strategy with Endgrate
While integrating with individual APIs like Teamwork CRM can be beneficial, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies integration processes across various platforms.
By using Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations.
- Provide an intuitive integration experience for your customers, enhancing user satisfaction and engagement.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate's website and discover the benefits of a unified API approach.
Read More
Ready to get started?