How to Get Candidates with the Recruitee API in Javascript
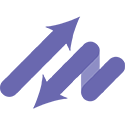
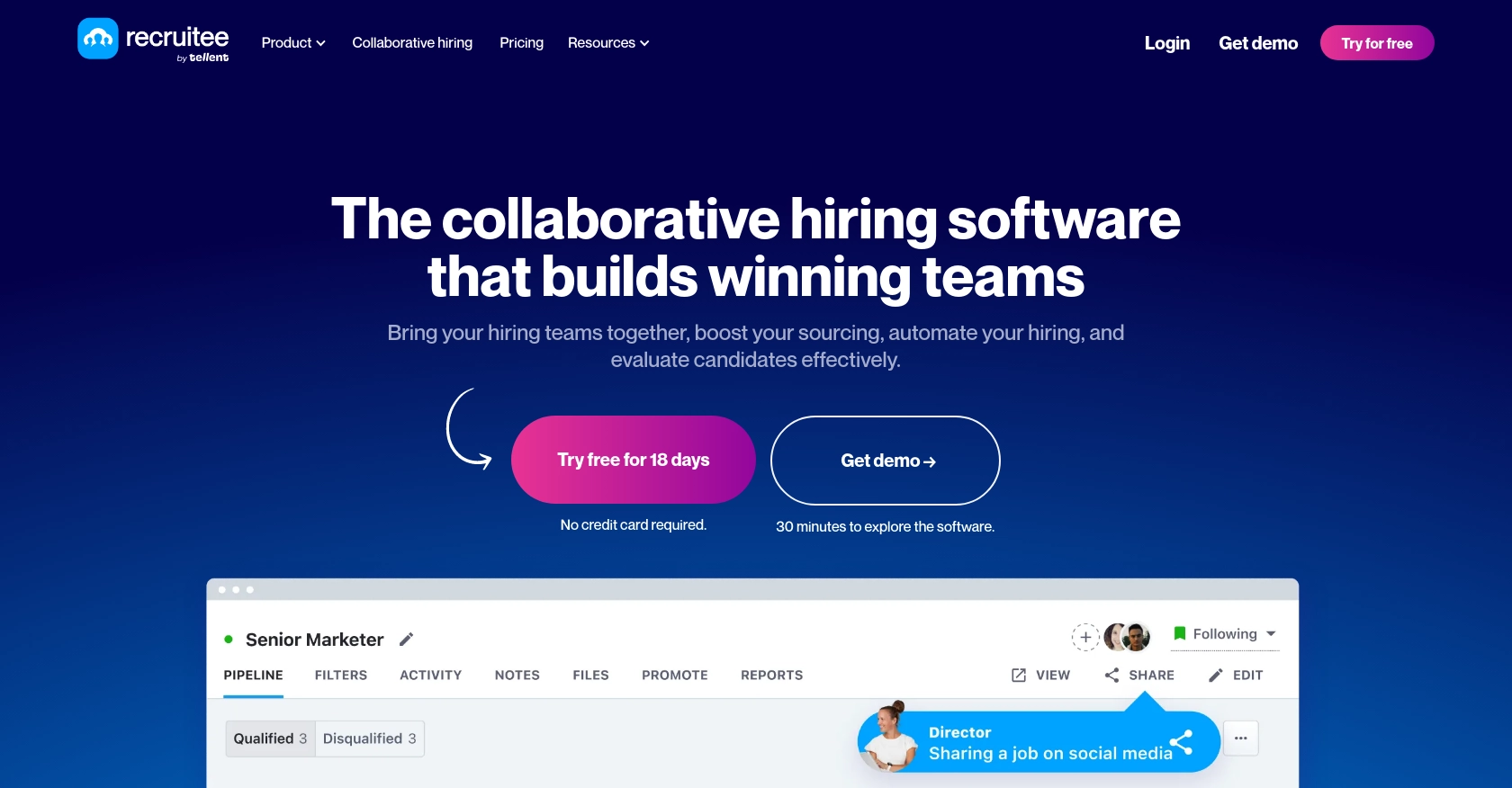
Introduction to Recruitee's ATS API
Recruitee is a powerful applicant tracking system (ATS) that helps businesses streamline their recruitment processes. With features like job posting, candidate management, and team collaboration, Recruitee is a popular choice for companies looking to enhance their hiring efficiency.
Integrating with Recruitee's ATS API allows developers to automate and manage candidate data seamlessly. For example, you can use the API to retrieve candidate information and integrate it into your internal systems, enabling a more efficient recruitment workflow.
Setting Up Your Recruitee Test Account
Before you can start interacting with Recruitee's ATS API, you'll need to set up a test account and generate an API token. This will allow you to authenticate your requests and access candidate data securely.
Creating a Recruitee Account
If you don't already have a Recruitee account, you can sign up for a free trial on their website. This will give you access to the necessary features to test the API integration.
- Visit the Recruitee website and click on "Try for free."
- Follow the prompts to create your account by providing the required information.
- Once your account is set up, log in to access the dashboard.
Generating a Personal API Token in Recruitee
To authenticate API requests, you'll need a personal API token. Follow these steps to generate one:
- Navigate to Settings in the Recruitee dashboard.
- Select Apps and plugins from the menu.
- Click on Personal API tokens.
- Click + New token in the top-right corner to generate a new token.
- Copy the generated token and store it securely, as you'll need it for API requests.
For more detailed instructions, refer to the Recruitee API documentation.
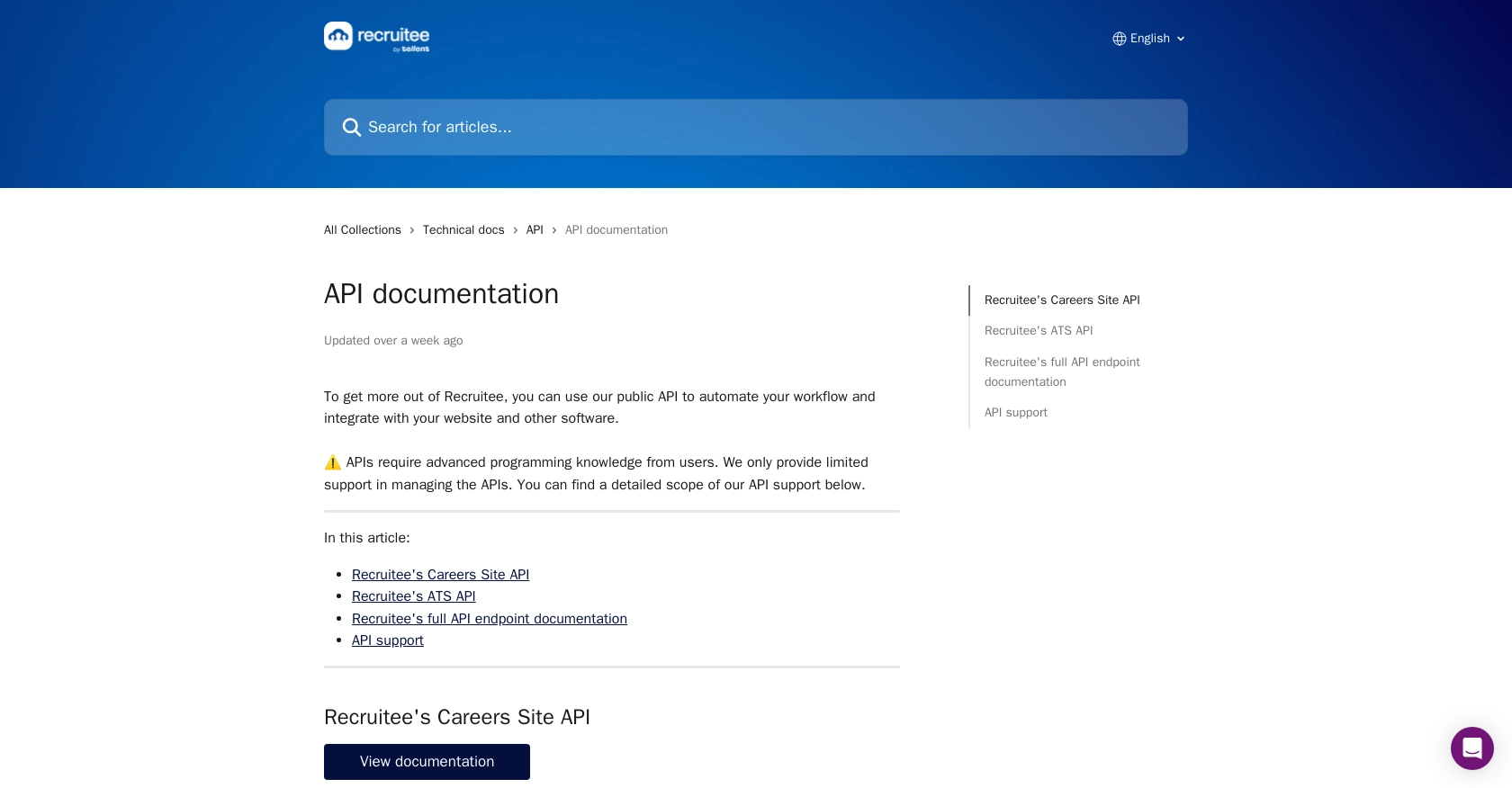
sbb-itb-96038d7
Making API Calls to Retrieve Candidates from Recruitee Using JavaScript
To interact with Recruitee's ATS API and retrieve candidate data, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the code, and handling responses effectively.
Setting Up Your JavaScript Environment for Recruitee API Integration
Before making API calls, ensure you have Node.js installed on your machine. Node.js allows you to run JavaScript code outside of a browser, which is essential for server-side operations like API requests.
- Download and install Node.js from the official website.
- Verify the installation by running
node -v
andnpm -v
in your terminal. - Initialize a new Node.js project by running
npm init -y
in your project directory. - Install the Axios library for making HTTP requests:
npm install axios
.
Writing JavaScript Code to Fetch Candidates from Recruitee
With your environment set up, you can now write the JavaScript code to interact with the Recruitee API. The following example demonstrates how to fetch candidate data using Axios.
const axios = require('axios');
// Replace 'Your_Token' and 'Your_Company_ID' with your actual API token and company ID
const apiToken = 'Your_Token';
const companyId = 'Your_Company_ID';
// Set the API endpoint
const endpoint = `https://api.recruitee.com/c/${companyId}/candidates`;
// Configure the request headers
const headers = {
'Authorization': `Bearer ${apiToken}`
};
// Make a GET request to the Recruitee API
axios.get(endpoint, { headers })
.then(response => {
// Handle the response data
const candidates = response.data;
console.log('Candidates:', candidates);
})
.catch(error => {
// Handle errors
console.error('Error fetching candidates:', error.response ? error.response.data : error.message);
});
In this code, you start by importing Axios and setting up your API token and company ID. You then define the API endpoint and configure the request headers with your token. The axios.get
method is used to make the request, and the response data is logged to the console.
Verifying Successful API Requests and Handling Errors
After running the code, you should see a list of candidates printed in the console. To verify the request's success, you can cross-check the data with your Recruitee dashboard.
It's crucial to handle potential errors gracefully. The example code includes error handling that logs any issues encountered during the request. Common errors might include invalid tokens or network issues.
For more detailed information on error codes and handling, refer to the Recruitee API documentation.
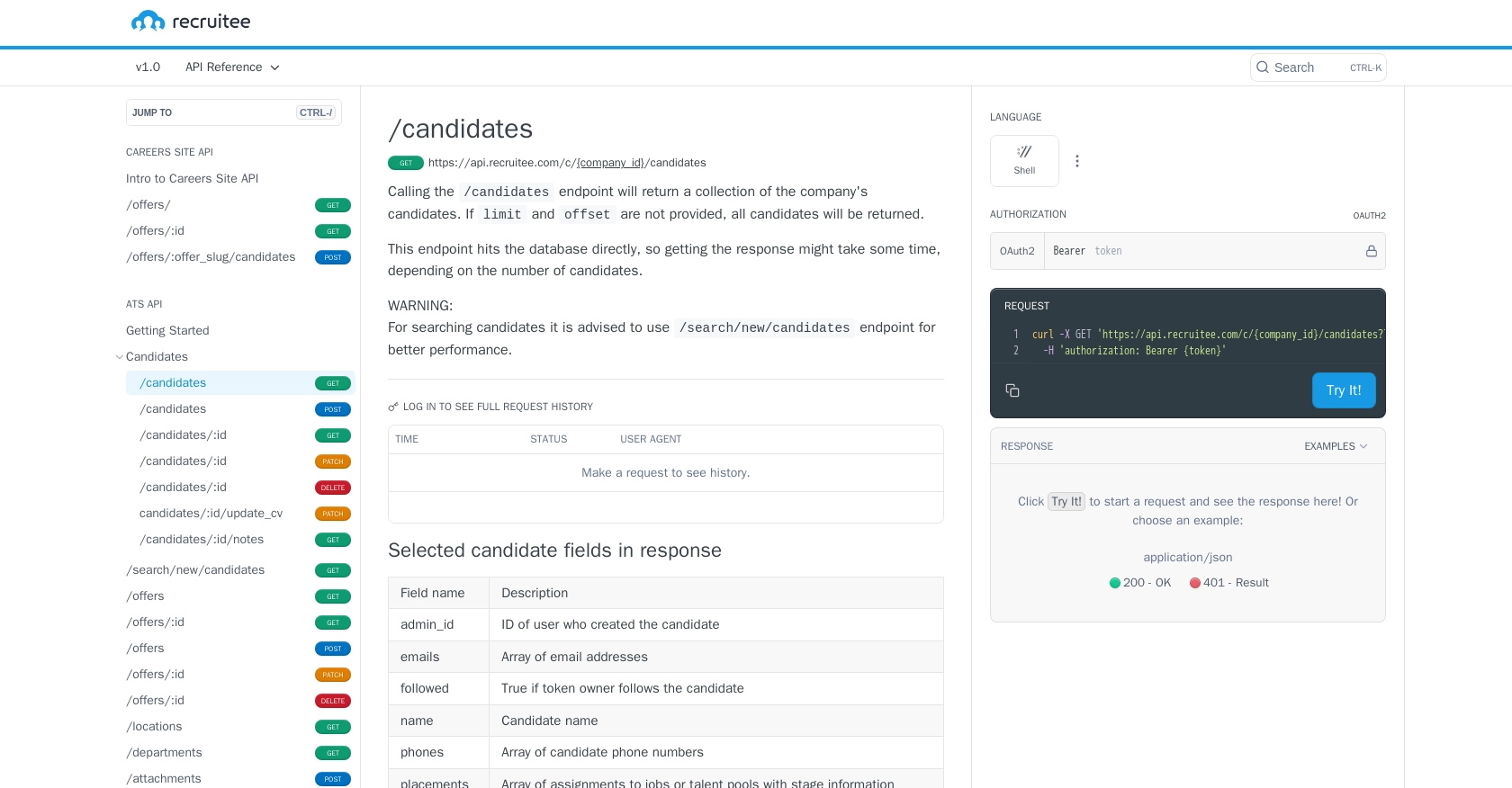
Conclusion: Best Practices for Using Recruitee's ATS API in JavaScript
Integrating with Recruitee's ATS API using JavaScript can significantly enhance your recruitment processes by automating candidate data management. To ensure a smooth integration, consider the following best practices:
Securely Storing API Credentials
Always store your API tokens securely. Avoid hardcoding them in your source files. Instead, use environment variables or secure vaults to manage sensitive information.
Handling Recruitee API Rate Limits
While the Recruitee API documentation does not specify rate limits, it's a good practice to implement error handling for rate limit responses. This ensures your application can gracefully handle any potential throttling by the API.
Transforming and Standardizing Candidate Data
When integrating candidate data into your systems, ensure that data fields are standardized and transformed as needed. This will help maintain consistency across different platforms and improve data quality.
Utilizing Endgrate for Efficient Integrations
If managing multiple integrations becomes overwhelming, consider using Endgrate. Endgrate provides a unified API endpoint that simplifies the integration process across various platforms, including Recruitee. This allows you to focus on your core product while outsourcing complex integrations.
By following these best practices and leveraging tools like Endgrate, you can streamline your recruitment workflows and enhance your overall hiring efficiency.
Read More
Ready to get started?