Using the Xero API to Create or Update Invoices (with Javascript examples)
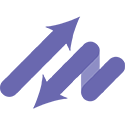
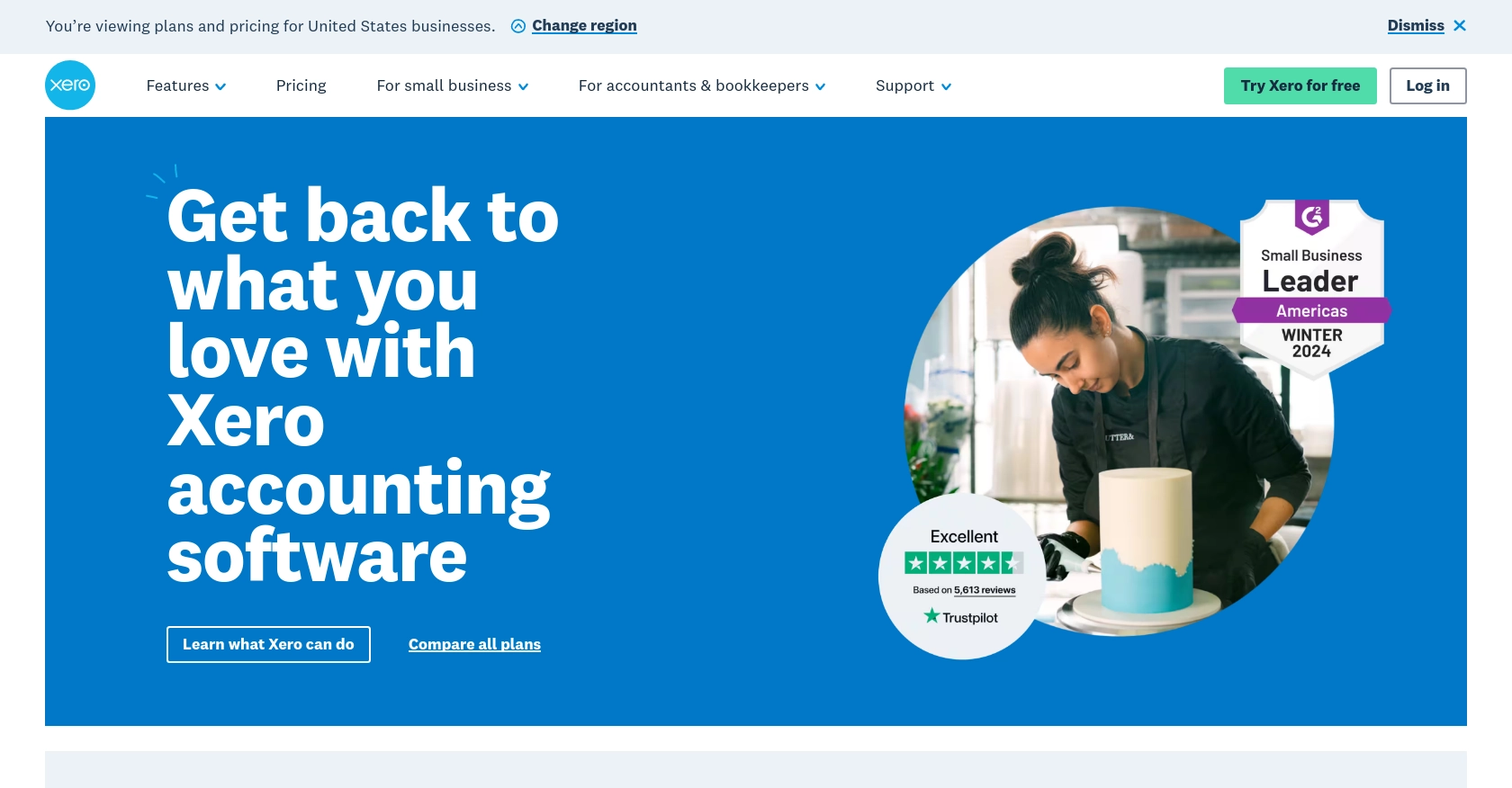
Introduction to Xero API for Invoice Management
Xero is a powerful cloud-based accounting software platform designed to help small and medium-sized businesses manage their finances efficiently. With features like invoicing, bank reconciliation, and expense tracking, Xero provides a comprehensive solution for financial management.
Integrating with Xero's API allows developers to automate and streamline accounting tasks, such as creating or updating invoices. For example, a developer might use the Xero API to automatically generate invoices from an e-commerce platform, ensuring that all sales are accurately recorded in the accounting system.
Setting Up Your Xero Sandbox Account for API Integration
Before diving into the integration process with Xero's API, it's essential to set up a sandbox account. This allows developers to test their applications in a controlled environment without affecting live data. Xero provides a demo company that you can use for testing purposes.
Creating a Xero Developer Account
To begin, you'll need a Xero developer account. Follow these steps to create one:
- Visit the Xero Developer Portal and sign up for a developer account.
- Once registered, log in to your account to access the developer dashboard.
Setting Up a Xero Demo Company
With your developer account ready, you can now set up a demo company:
- Navigate to the My Apps section in the developer dashboard.
- Select the option to create a new app, and fill in the required details such as app name and company URL.
- After creating the app, you'll have access to a demo company, which you can use to test API calls.
Configuring OAuth 2.0 Authentication
Xero's API uses OAuth 2.0 for authentication. Here's how to set it up:
- In your app settings, locate the OAuth 2.0 credentials. You'll need the client ID and client secret for authentication.
- Set up the redirect URI, which is the URL to which users will be redirected after they authorize your app.
- Ensure you have the necessary scopes for invoice management. Refer to the Xero OAuth 2.0 Scopes documentation for more details.
Generating Access Tokens
With OAuth 2.0 configured, you can generate access tokens to authenticate API requests:
- Use the standard authorization code flow to obtain an authorization code. Detailed steps can be found in the Xero OAuth 2.0 Authorization Flow guide.
- Exchange the authorization code for an access token and a refresh token.
- Store these tokens securely, as they will be used to authenticate your API requests.
With your sandbox account and OAuth 2.0 authentication set up, you're ready to start integrating with Xero's API to create or update invoices using JavaScript.
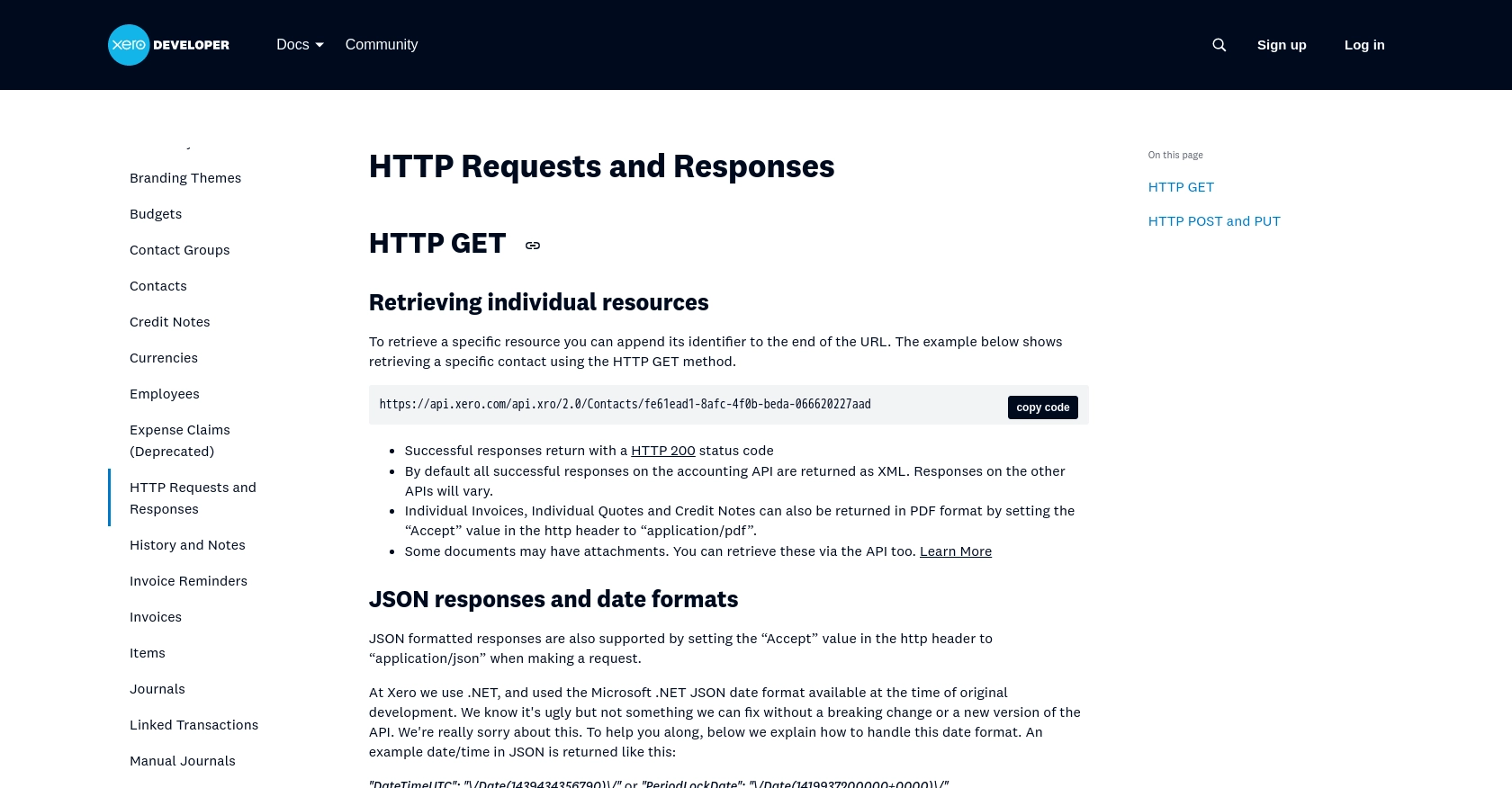
sbb-itb-96038d7
Making API Calls to Xero for Invoice Management Using JavaScript
With your Xero sandbox account and OAuth 2.0 authentication configured, you can now proceed to make API calls to create or update invoices using JavaScript. This section will guide you through the necessary steps, including setting up your development environment, writing the code, and handling responses and errors.
Setting Up Your JavaScript Environment for Xero API Integration
Before you start coding, ensure that you have the following prerequisites:
- Node.js installed on your machine. You can download it from the official Node.js website.
- A code editor like Visual Studio Code.
- The
axios
library for making HTTP requests. Install it using the following command:
npm install axios
Creating or Updating Invoices with Xero API
Now, let's write the JavaScript code to interact with the Xero API for invoice management. Create a file named xeroInvoices.js
and add the following code:
const axios = require('axios');
// Set the API endpoint for creating or updating invoices
const endpoint = 'https://api.xero.com/api.xro/2.0/Invoices';
// Set your access token and tenant ID
const accessToken = 'Your_Access_Token';
const tenantId = 'Your_Tenant_ID';
// Define the invoice data
const invoiceData = {
Type: 'ACCREC',
Contact: {
ContactID: 'Your_Contact_ID'
},
LineItems: [
{
Description: 'Consulting services',
Quantity: 10,
UnitAmount: 100,
AccountCode: '200'
}
],
Date: '2023-10-01',
DueDate: '2023-10-15',
Status: 'AUTHORISED'
};
// Configure the request headers
const headers = {
'Authorization': `Bearer ${accessToken}`,
'Xero-tenant-id': tenantId,
'Content-Type': 'application/json'
};
// Make the POST request to create or update the invoice
axios.post(endpoint, invoiceData, { headers })
.then(response => {
console.log('Invoice created/updated successfully:', response.data);
})
.catch(error => {
console.error('Error creating/updating invoice:', error.response.data);
});
Replace Your_Access_Token
, Your_Tenant_ID
, and Your_Contact_ID
with the appropriate values obtained during the OAuth 2.0 setup.
Verifying Successful API Requests and Handling Errors
After running the script, you should verify the success of your API request by checking the response data. If the request is successful, the invoice details will be printed in the console. You can also log in to your Xero demo company to confirm that the invoice has been created or updated.
In case of errors, the error response will be logged in the console. Common error codes include:
- 400 Bad Request: The request was invalid or cannot be served. Check the request parameters.
- 401 Unauthorized: Authentication failed. Verify your access token and tenant ID.
- 429 Too Many Requests: Rate limit exceeded. Refer to the Xero API rate limits documentation for more details.
By following these steps, you can efficiently manage invoices in Xero using JavaScript, ensuring seamless integration with your applications.
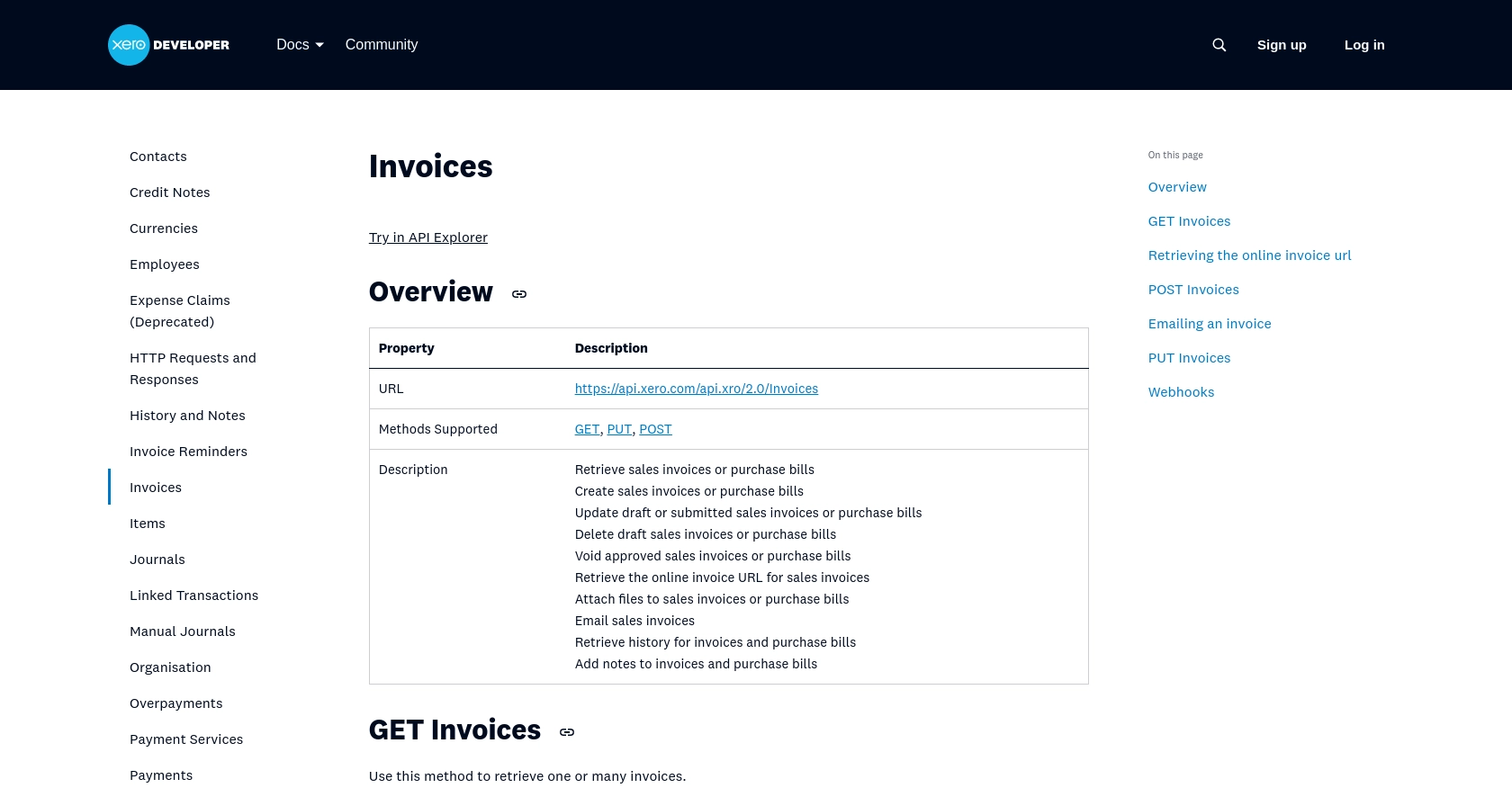
Conclusion: Best Practices for Xero API Integration and Next Steps
Integrating with the Xero API to manage invoices using JavaScript can significantly enhance your application's financial management capabilities. By automating invoice creation and updates, you can streamline accounting processes and ensure accurate financial records.
Best Practices for Secure and Efficient Xero API Usage
- Secure Token Storage: Always store your access and refresh tokens securely. Consider using environment variables or secure vault services to protect sensitive information.
- Handle Rate Limits: Be mindful of Xero's rate limits to avoid disruptions. Implement retry logic with exponential backoff to manage requests efficiently. For more details, refer to the Xero API rate limits documentation.
- Data Standardization: Ensure that data fields are consistent and standardized across your application to prevent errors and maintain data integrity.
Enhance Your Integration Strategy with Endgrate
While integrating with Xero's API offers powerful capabilities, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint for various platforms, including Xero.
By leveraging Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing you to focus on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an intuitive integration experience for your customers, enhancing user satisfaction.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate's website and discover a more efficient way to manage your API integrations.
Read More
- https://endgrate.com/provider/xero
- https://developer.xero.com/documentation/api/accounting/requests-and-responses
- https://developer.xero.com/documentation/guides/oauth2/limits/
- https://developer.xero.com/documentation/guides/oauth2/overview
- https://developer.xero.com/documentation/guides/oauth2/scopes
- https://developer.xero.com/documentation/guides/oauth2/auth-flow
- https://developer.xero.com/documentation/guides/oauth2/tenants
- https://developer.xero.com/documentation/api/accounting/invoices
Ready to get started?