Using the Keap API to Get Users in Javascript
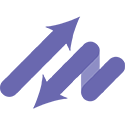
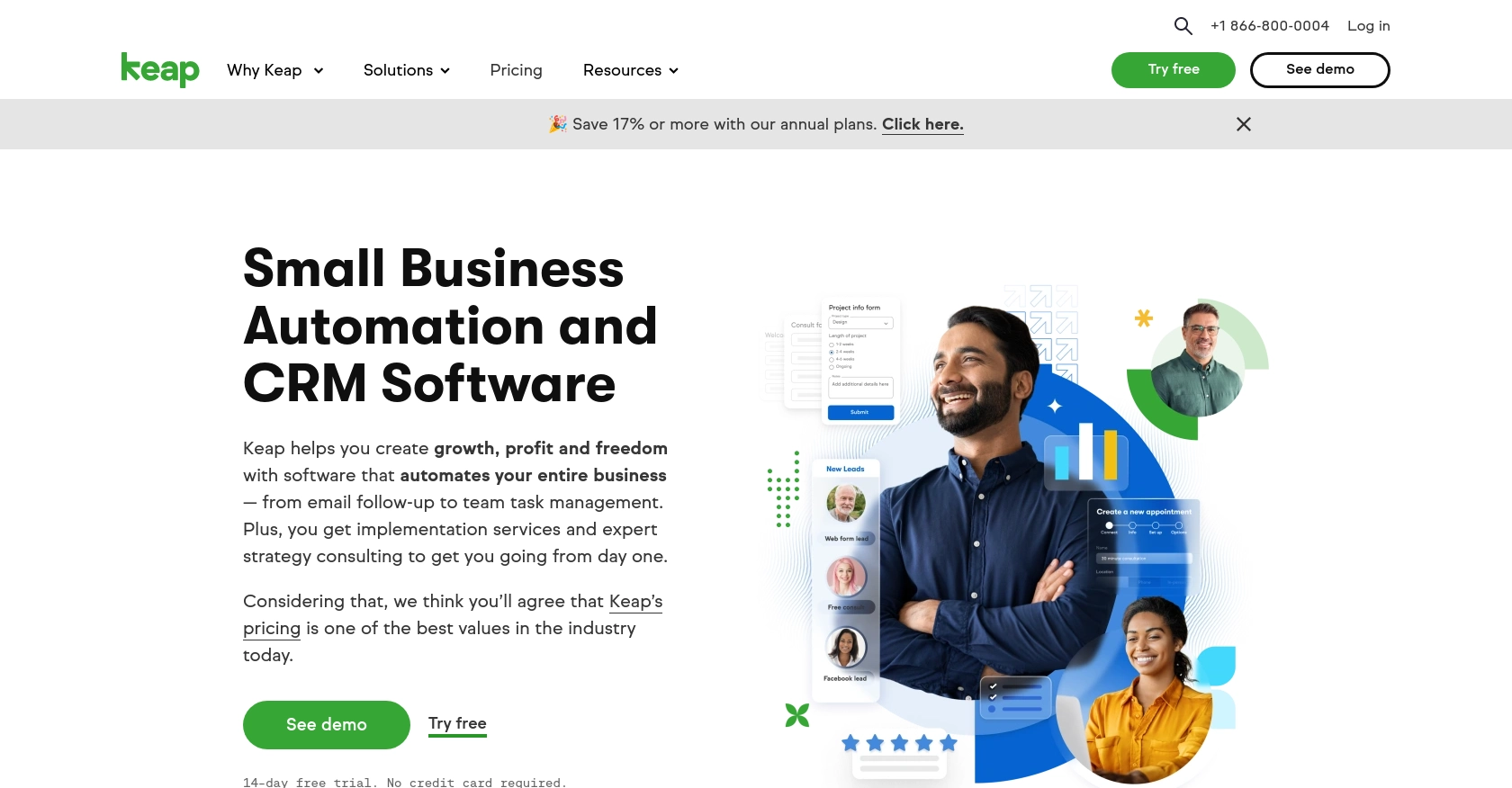
Introduction to Keap API Integration
Keap, formerly known as Infusionsoft, is a powerful CRM and marketing automation platform designed to help small businesses streamline their sales and marketing efforts. With its robust suite of tools, Keap enables businesses to manage customer relationships, automate marketing campaigns, and track sales performance effectively.
For developers, integrating with the Keap API opens up opportunities to enhance business processes by accessing and managing user data programmatically. For example, you might want to retrieve user information from Keap to personalize marketing communications or synchronize customer data with other applications.
Setting Up Your Keap Developer Account and Sandbox Environment
Before you can start interacting with the Keap API, you'll need to set up a developer account and create a sandbox environment. This will allow you to test your integration without affecting live data.
Register for a Keap Developer Account
To begin, you'll need to register for a Keap developer account. This account will give you access to the necessary tools and resources to build and test your integration.
- Visit the Keap Developer Portal.
- Click on the "Register" button to create a new developer account.
- Fill out the registration form with your details and submit it.
- Once registered, log in to your developer account to access the dashboard.
Create a Sandbox App in Keap
With your developer account set up, the next step is to create a sandbox app. This environment will allow you to test API calls without impacting real user data.
- In your developer dashboard, navigate to the "Sandbox Apps" section.
- Click on "Create a New Sandbox App."
- Provide the necessary information, such as app name and description, and submit the form.
- Your sandbox app will be created, and you'll receive a client ID and client secret, which are essential for OAuth authentication.
Set Up OAuth Authentication for Keap API
Keap uses OAuth 2.0 for authentication. Follow these steps to set up OAuth for your sandbox app:
- In the sandbox app settings, locate the "OAuth Credentials" section.
- Copy the client ID and client secret provided.
- Set up a redirect URI in your application to handle the OAuth callback.
- Use the client ID, client secret, and redirect URI to request an access token from Keap.
For more detailed information on OAuth setup, refer to the Keap REST API documentation.
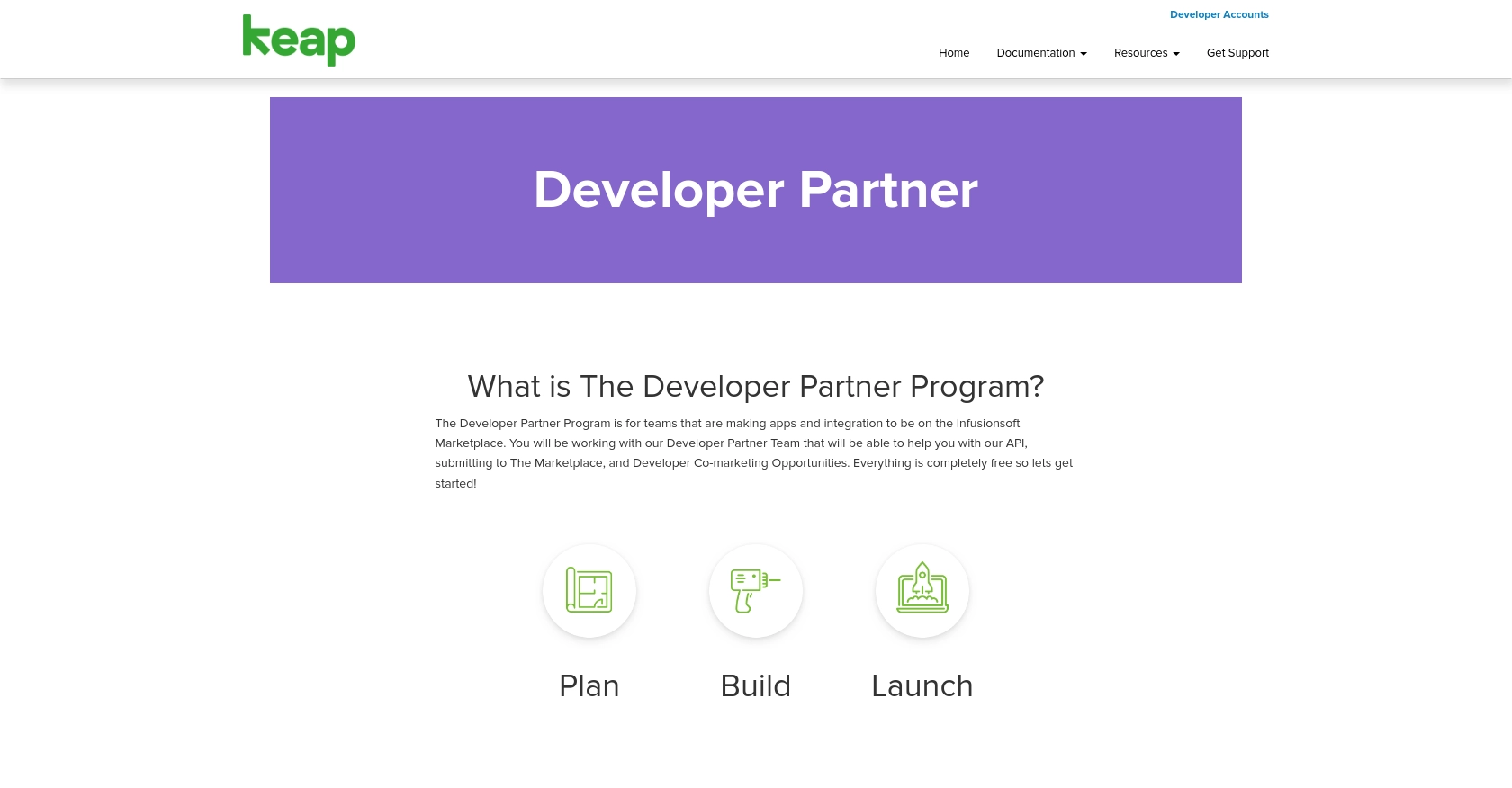
sbb-itb-96038d7
Making API Calls to Retrieve Users from Keap Using JavaScript
To interact with the Keap API and retrieve user data, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the code, and handling potential errors.
Setting Up Your JavaScript Environment for Keap API Integration
Before making API calls, ensure you have a suitable environment for running JavaScript. You'll need Node.js installed on your machine, which comes with npm (Node Package Manager) to manage dependencies.
- Download and install Node.js if you haven't already.
- Open your terminal or command prompt and verify the installation by running
node -v
andnpm -v
. - Create a new directory for your project and navigate into it.
- Initialize a new Node.js project by running
npm init -y
. - Install the Axios library for making HTTP requests by running
npm install axios
.
Writing JavaScript Code to Fetch Users from Keap API
With your environment set up, you can now write the JavaScript code to fetch users from the Keap API. The following example demonstrates how to make a GET request to retrieve user data.
const axios = require('axios');
// Define the API endpoint and headers
const endpoint = 'https://api.keap.com/crm/rest/v1/users';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json'
};
// Function to get users from Keap
async function getUsers() {
try {
const response = await axios.get(endpoint, { headers });
const users = response.data;
console.log('Retrieved Users:', users);
} catch (error) {
console.error('Error fetching users:', error.response ? error.response.data : error.message);
}
}
// Call the function to fetch users
getUsers();
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth authentication process. This token is essential for authorizing your API requests.
Verifying Successful API Requests and Handling Errors
After running the script, you should see the user data printed in the console if the request is successful. To verify, log in to your Keap sandbox account and check the user data to ensure it matches the retrieved information.
In case of errors, the script will log the error message. Common issues include invalid tokens or incorrect endpoint URLs. Refer to the Keap REST API documentation for detailed error code explanations and troubleshooting tips.
Best Practices for Keap API Integration and Error Handling
When integrating with the Keap API, it's crucial to follow best practices to ensure a smooth and efficient process. Here are some key recommendations:
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be aware of Keap's API rate limits to avoid exceeding the allowed number of requests. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Standardize Data Fields: Ensure that data fields retrieved from Keap are standardized and transformed as needed to match your application's data schema. This will help maintain consistency across different systems.
- Error Handling: Implement robust error handling to manage different types of errors, such as network issues or invalid requests. Log errors for troubleshooting and use descriptive error messages to aid in debugging.
Streamline Your Integrations with Endgrate
Building and maintaining integrations can be time-consuming and complex. With Endgrate, you can simplify this process by leveraging a single API endpoint that connects to multiple platforms, including Keap.
Endgrate allows you to focus on your core product by outsourcing integrations, saving time and resources. With its intuitive interface, you can build once for each use case and provide a seamless integration experience for your customers.
Explore how Endgrate can enhance your integration capabilities by visiting Endgrate's website and discover how it can help you scale your integrations efficiently.
Read More
Ready to get started?