How to Get Meeting Analytics with the Google Meet API in PHP
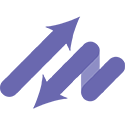
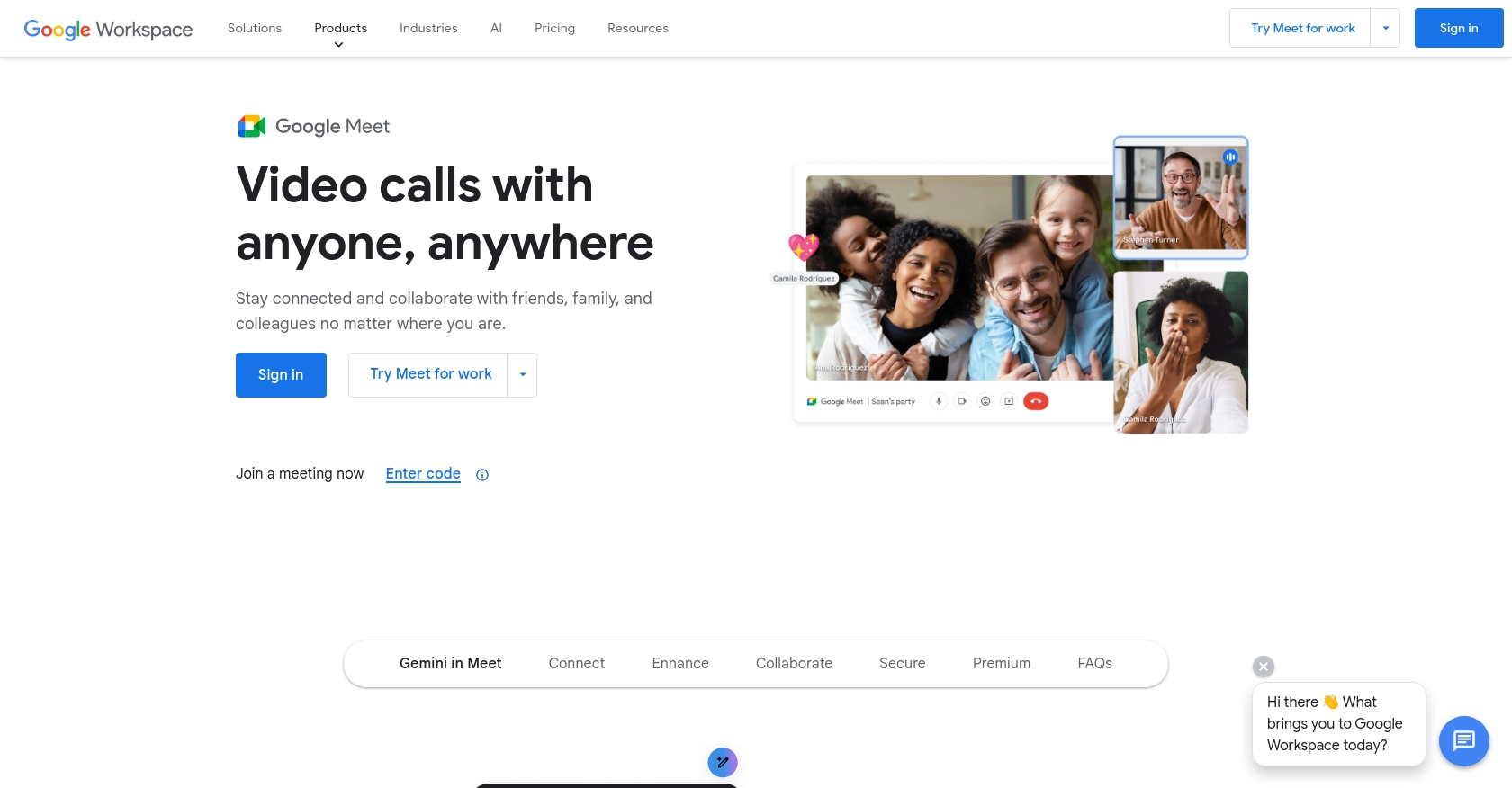
Introduction to Google Meet API
Google Meet is a widely used video conferencing platform that enables seamless communication and collaboration for businesses and individuals. It offers a range of features, including video calls, screen sharing, and real-time captions, making it an essential tool for remote work and virtual meetings.
Integrating with the Google Meet API allows developers to access meeting data and analytics, providing valuable insights into meeting performance and participant engagement. For example, a developer might use the API to retrieve meeting analytics, such as participant count and duration, to generate reports for performance reviews or to optimize meeting schedules.
Setting Up Your Google Meet API Test Account
Before you can start using the Google Meet API to gather meeting analytics, you'll need to set up a Google Cloud project and configure OAuth 2.0 for authentication. This setup will allow you to access the API securely and retrieve the necessary data.
Create a Google Cloud Project for Google Meet API
- Go to the Google Cloud Console.
- Navigate to Menu > IAM & Admin > Create a Project.
- Enter a descriptive name for your project and click Create.
Enable Google Meet API in Your Project
- In the Google Cloud Console, go to Menu > APIs & Services > Library.
- Search for "Google Meet API" and click on it.
- Click Enable to activate the API for your project.
Configure OAuth Consent Screen for Google Meet API
- In the Google Cloud Console, go to Menu > APIs & Services > OAuth consent screen.
- Select the user type for your app and click Create.
- Fill out the app registration form and click Save and Continue.
Create OAuth 2.0 Credentials for Google Meet API
- Go to Menu > APIs & Services > Credentials.
- Click Create Credentials > OAuth client ID.
- Select Web application as the application type.
- Enter a name for the credential and add authorized redirect URIs.
- Click Create to generate your client ID and client secret.
Ensure you securely store your client ID and client secret, as they will be used to authenticate your API requests.
For more detailed instructions, refer to the official Google documentation: Create a Google Cloud Project, Enable Google Workspace APIs, Configure OAuth Consent, and Create Credentials.
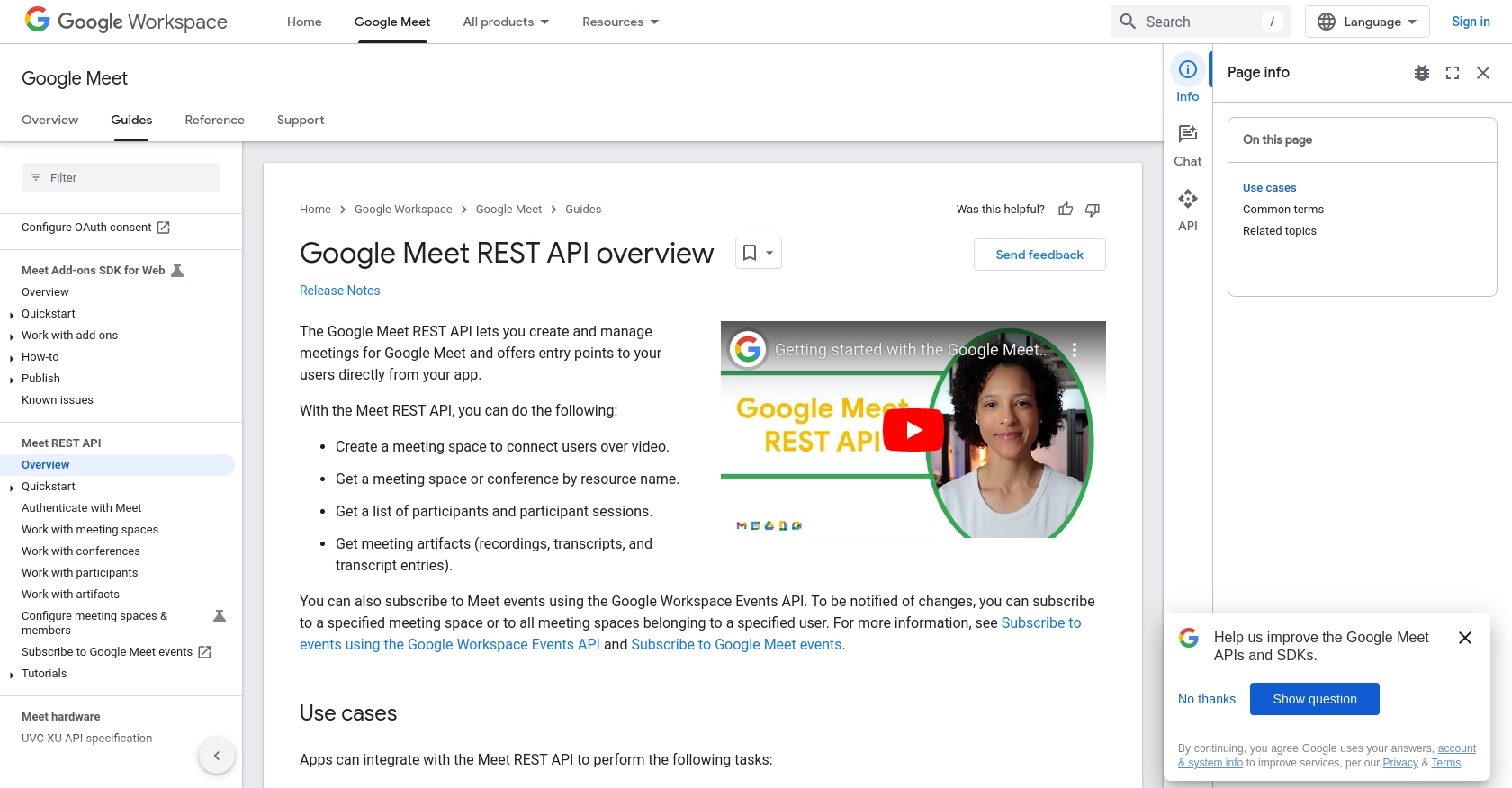
sbb-itb-96038d7
Making API Calls to Retrieve Meeting Analytics from Google Meet Using PHP
To interact with the Google Meet API and retrieve meeting analytics using PHP, you'll need to set up your environment with the necessary tools and libraries. This section will guide you through the process of making API calls to access meeting data, such as participant count and meeting duration, using PHP.
Setting Up Your PHP Environment for Google Meet API
Before making API calls, ensure your PHP environment is properly configured. You'll need PHP version 7.4 or higher and the Composer dependency manager to install required libraries.
- Ensure PHP is installed on your system. You can check your PHP version by running
php -v
in your terminal. - Install Composer by following the instructions on the Composer website.
- Use Composer to install the Google API Client Library for PHP by running the following command:
composer require google/apiclient:^2.0
Authenticating with Google Meet API Using OAuth 2.0 in PHP
To authenticate your requests, you'll use OAuth 2.0 credentials. Ensure you have your client ID and client secret from the previous setup steps.
require_once 'vendor/autoload.php';
$client = new Google_Client();
$client->setAuthConfig('path/to/your/client_credentials.json');
$client->addScope('https://www.googleapis.com/auth/meetings.space.readonly');
// Redirect to Google's OAuth 2.0 server
if (!isset($_GET['code'])) {
$authUrl = $client->createAuthUrl();
header('Location: ' . filter_var($authUrl, FILTER_SANITIZE_URL));
} else {
$client->authenticate($_GET['code']);
$_SESSION['access_token'] = $client->getAccessToken();
}
Replace path/to/your/client_credentials.json
with the path to your downloaded credentials file. This script will redirect users to Google's OAuth 2.0 server for authentication.
Executing API Calls to Fetch Meeting Analytics Data
Once authenticated, you can make API calls to retrieve meeting analytics. Below is an example of how to fetch meeting data using the Google Meet API.
if (isset($_SESSION['access_token']) && $_SESSION['access_token']) {
$client->setAccessToken($_SESSION['access_token']);
$service = new Google_Service_Meet($client);
$results = $service->meetings->listMeetings();
foreach ($results->getItems() as $meeting) {
echo 'Meeting ID: ' . $meeting->getId() . '<br>';
echo 'Participant Count: ' . $meeting->getParticipantCount() . '<br>';
echo 'Duration: ' . $meeting->getDuration() . ' minutes<br>';
}
} else {
echo 'No access token found. Please authenticate first.';
}
This code snippet initializes the Google Meet service and retrieves a list of meetings, displaying each meeting's ID, participant count, and duration.
Handling Errors and Verifying API Call Success
It's important to handle potential errors when making API calls. Check the response status and handle exceptions appropriately to ensure robust error management.
try {
$results = $service->meetings->listMeetings();
// Process results
} catch (Exception $e) {
echo 'An error occurred: ' . $e->getMessage();
}
Verify the success of your API calls by checking the returned data in your Google Cloud Console or by logging the output for further analysis.
Conclusion and Best Practices for Using Google Meet API in PHP
Integrating with the Google Meet API using PHP provides developers with powerful tools to access and analyze meeting data, enhancing the ability to optimize virtual meeting experiences. By following the steps outlined in this guide, you can efficiently set up your environment, authenticate using OAuth 2.0, and execute API calls to retrieve valuable meeting analytics.
Best Practices for Secure and Efficient API Integration
- Secure Storage of Credentials: Always store your client ID and client secret securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limiting: Be aware of Google Meet API rate limits and implement retry logic or exponential backoff strategies to handle rate limit errors gracefully.
- Data Standardization: Ensure that the data retrieved from the API is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement robust error handling to manage exceptions and ensure smooth operation even when issues arise.
Streamlining Integrations with Endgrate
For developers looking to simplify and scale their integration efforts, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Endgrate's unified API provides an intuitive integration experience, enabling you to build once for each use case and connect to multiple platforms seamlessly.
Explore how Endgrate can enhance your integration capabilities by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/googlemeet
- https://developers.google.com/meet/api/guides/overview
- https://developers.google.com/meet/api/guides/authenticate-authorize
- https://developers.google.com/workspace/guides/create-project
- https://developers.google.com/workspace/guides/enable-apis
- https://developers.google.com/workspace/guides/configure-oauth-consent
- https://developers.google.com/workspace/guides/create-credentials
Ready to get started?