Using the Pendo API to Get Page Parameters in PHP
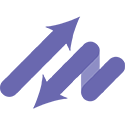
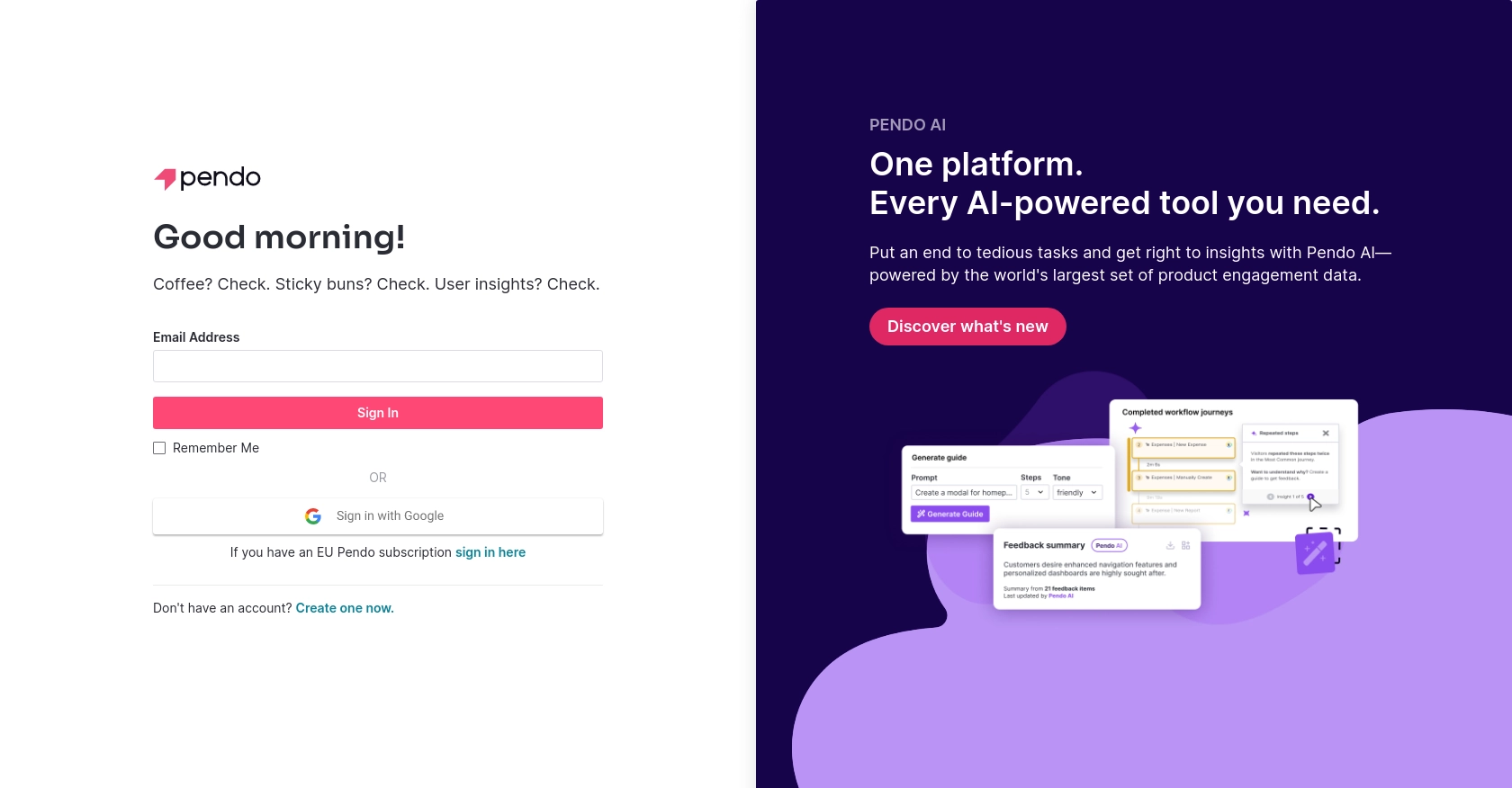
Introduction to Pendo API
Pendo is a powerful platform designed to help businesses understand and guide user experiences within their applications. By providing insights into user behavior and offering tools for in-app messaging and feedback, Pendo enables companies to enhance their product offerings and improve customer satisfaction.
Developers might want to integrate with the Pendo API to access detailed analytics and user interaction data. For example, retrieving page parameters using the Pendo API in PHP can help developers analyze user engagement on specific pages, allowing for more targeted improvements and personalized user experiences.
Setting Up Your Pendo Test Account and API Key
Before you can start using the Pendo API to retrieve page parameters in PHP, you'll need to set up a Pendo account and obtain an API key. This key will allow you to authenticate your requests and access the necessary data.
Creating a Pendo Account
If you don't already have a Pendo account, you can sign up for a free trial on the Pendo website. This will give you access to the platform's features and allow you to explore its capabilities.
- Visit the Pendo website and click on the "Sign Up" button.
- Fill out the registration form with your details and submit it to create your account.
- Once your account is created, log in to access the Pendo dashboard.
Generating Your Pendo API Key
To interact with the Pendo API, you'll need an API key. Follow these steps to generate one:
- Log in to your Pendo account and navigate to the "Settings" section.
- Under "Integrations," find the "API Keys" option.
- Click on "Create API Key" and provide a name for your key to help you identify it later.
- Once the key is generated, copy it and store it securely. You'll need it to authenticate your API requests.
With your Pendo account set up and your API key in hand, you're ready to start making API calls to retrieve page parameters using PHP.
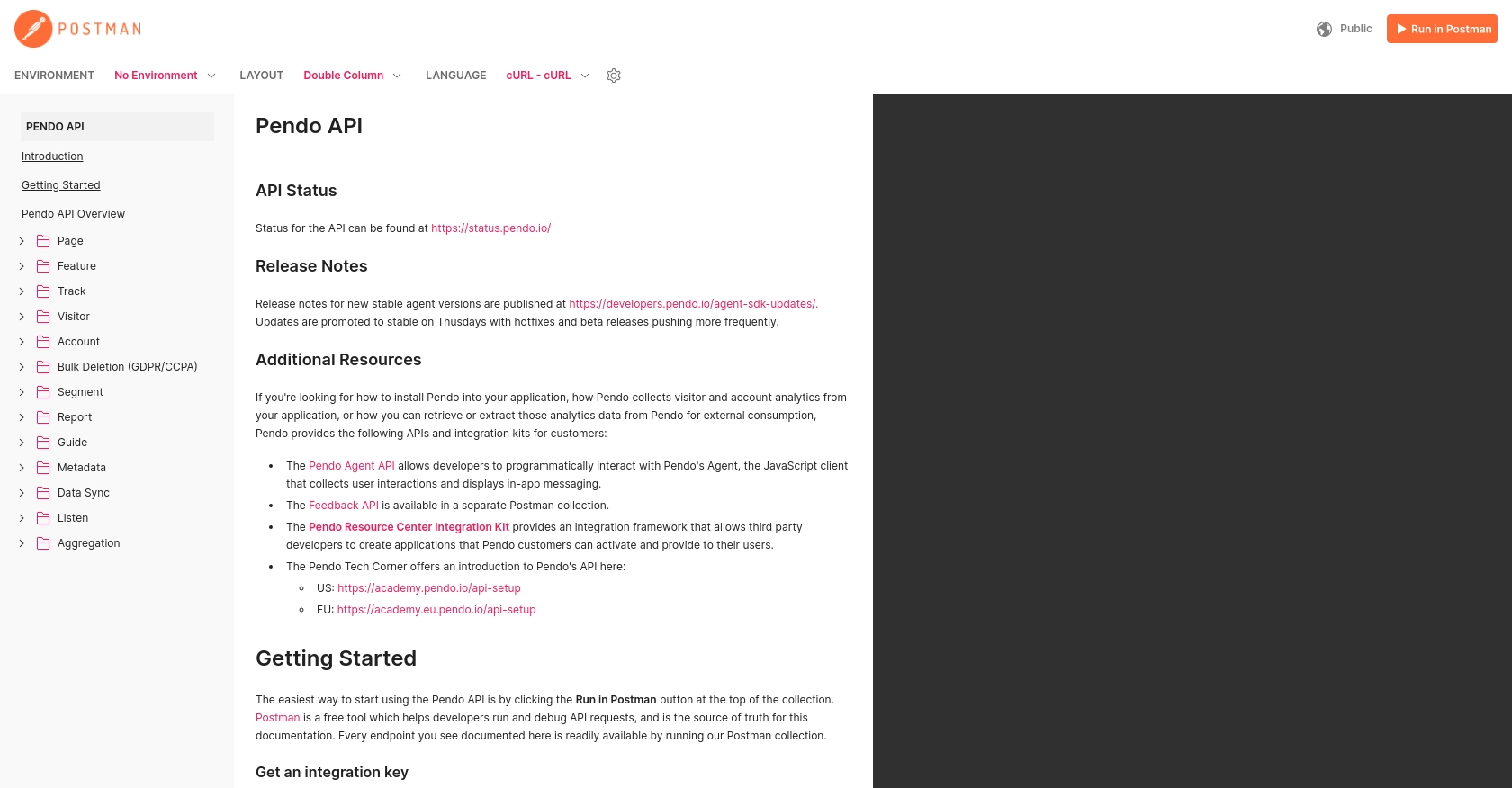
sbb-itb-96038d7
Making API Calls to Retrieve Pendo Page Parameters Using PHP
To effectively interact with the Pendo API and retrieve page parameters using PHP, you'll need to ensure your development environment is properly set up. This includes having the correct version of PHP and installing necessary dependencies.
Setting Up Your PHP Environment
Before diving into the code, make sure you have the following prerequisites:
- PHP 7.4 or later installed on your machine.
- Composer, the PHP package manager, to manage dependencies.
Once you have these installed, you can proceed to install the Guzzle HTTP client, which simplifies making HTTP requests in PHP. Run the following command in your terminal:
composer require guzzlehttp/guzzle
Writing PHP Code to Call the Pendo API
With your environment ready, you can now write the PHP code to make an API call to Pendo and retrieve page parameters. Create a new PHP file named get_pendo_page_parameters.php
and add the following code:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$apiKey = 'Your_API_Key'; // Replace with your actual API key
try {
$response = $client->request('GET', 'https://engageapi.pendo.io/api/v1/pages', [
'headers' => [
'Authorization' => 'api_key ' . $apiKey,
'Content-Type' => 'application/json'
]
]);
$data = json_decode($response->getBody(), true);
foreach ($data['pages'] as $page) {
echo 'Page Name: ' . $page['name'] . '<br>';
echo 'Page ID: ' . $page['id'] . '<br>';
echo 'Parameters: ' . json_encode($page['parameters']) . '<br><br>';
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Replace Your_API_Key
with the API key you generated earlier. This script uses Guzzle to send a GET request to the Pendo API endpoint for pages. It then parses the JSON response to display the page name, ID, and parameters.
Running the PHP Script and Verifying the Output
To execute the script, run the following command in your terminal:
php get_pendo_page_parameters.php
If successful, you should see a list of page names, IDs, and their parameters printed to the console. This confirms that your API call was successful and that you have retrieved the desired data from Pendo.
Handling Errors and Troubleshooting
While making API calls, you may encounter errors. Common issues include incorrect API keys or network problems. The script includes a try-catch block to handle exceptions and display error messages. Ensure your API key is correct and that your network connection is stable.
For more detailed error information, refer to the Pendo API documentation at Pendo API Documentation.
Conclusion and Best Practices for Using the Pendo API in PHP
Integrating with the Pendo API to retrieve page parameters using PHP can significantly enhance your ability to analyze user engagement and improve user experiences. By following the steps outlined in this article, you can efficiently set up your environment, authenticate your requests, and handle data retrieval.
Best Practices for Secure and Efficient API Integration
- Secure API Key Storage: Always store your API keys securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Pendo API to avoid throttling. Implement exponential backoff strategies to manage retries effectively.
- Data Transformation: Consider transforming and standardizing data fields to ensure consistency across different systems and applications.
Streamlining Integrations with Endgrate
While integrating with the Pendo API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product development. By using Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers.
Explore how Endgrate can help you save time and resources by visiting Endgrate today.
Read More
Ready to get started?