Using the Intercom API to Create Notes in Javascript
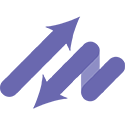
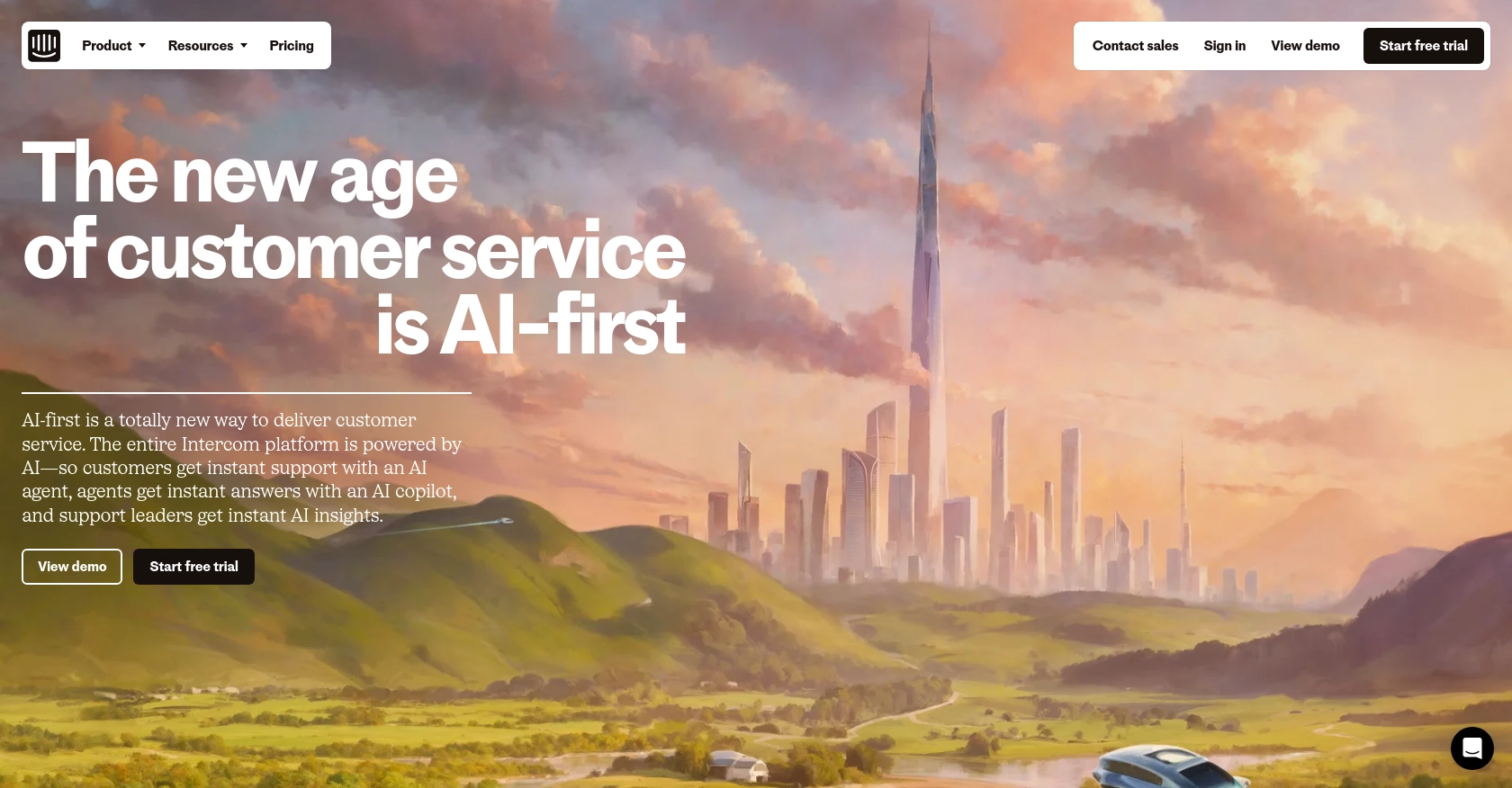
Introduction to Intercom API Integration
Intercom is a powerful customer communication platform that enables businesses to engage with their customers through personalized messaging and support. It offers a suite of tools for managing customer interactions, including live chat, email marketing, and customer feedback.
Developers often integrate with Intercom's API to enhance customer engagement and streamline communication processes. For example, creating notes on customer profiles can help support teams keep track of interactions and provide personalized service. This article will guide you through using the Intercom API to create notes in JavaScript, offering a practical solution for developers looking to optimize their customer support workflows.
Setting Up Your Intercom Developer Account and OAuth Authentication
Before you can start creating notes using the Intercom API, you'll need to set up a developer account and configure OAuth authentication. This process ensures that your application can securely interact with Intercom's services.
Creating an Intercom Developer Account
If you don't already have an Intercom developer account, follow these steps to create one:
- Visit the Intercom Developer Hub and sign up for a free account.
- Once registered, log in to your account to access the Developer Hub.
- Create a new development workspace, which will allow you to build and test your integration.
Configuring OAuth for Intercom API Access
Intercom uses OAuth for authentication, which allows your application to access user data securely. Follow these steps to set up OAuth:
- In the Developer Hub, navigate to the "Authentication" section.
- Select "Use OAuth" to enable OAuth for your application.
- Provide the necessary information, including:
- Redirect URLs: Specify the URLs where users will be redirected after authorizing your app. Ensure these URLs use HTTPS.
- Permissions: Choose the scopes your application needs. For creating notes, ensure you have the necessary permissions to read and write user data.
- Save your settings to generate your client ID and client secret.
Obtaining an Access Token
With your OAuth configuration complete, you can now obtain an access token to authenticate API requests:
- Redirect users to the following URL to obtain an authorization code:
- Once the user authorizes your app, they will be redirected to your specified URL with a
code
parameter. - Exchange this authorization code for an access token by making a POST request to:
code
: The authorization code received.client_id
: Your app's client ID.client_secret
: Your app's client secret.- Upon success, you'll receive an access token, which you can use to authenticate API requests.
https://app.intercom.com/oauth?client_id=YOUR_CLIENT_ID&state=YOUR_STATE
https://api.intercom.io/auth/eagle/token
Include the following parameters in the request body:
For more detailed information, refer to the Intercom OAuth documentation.
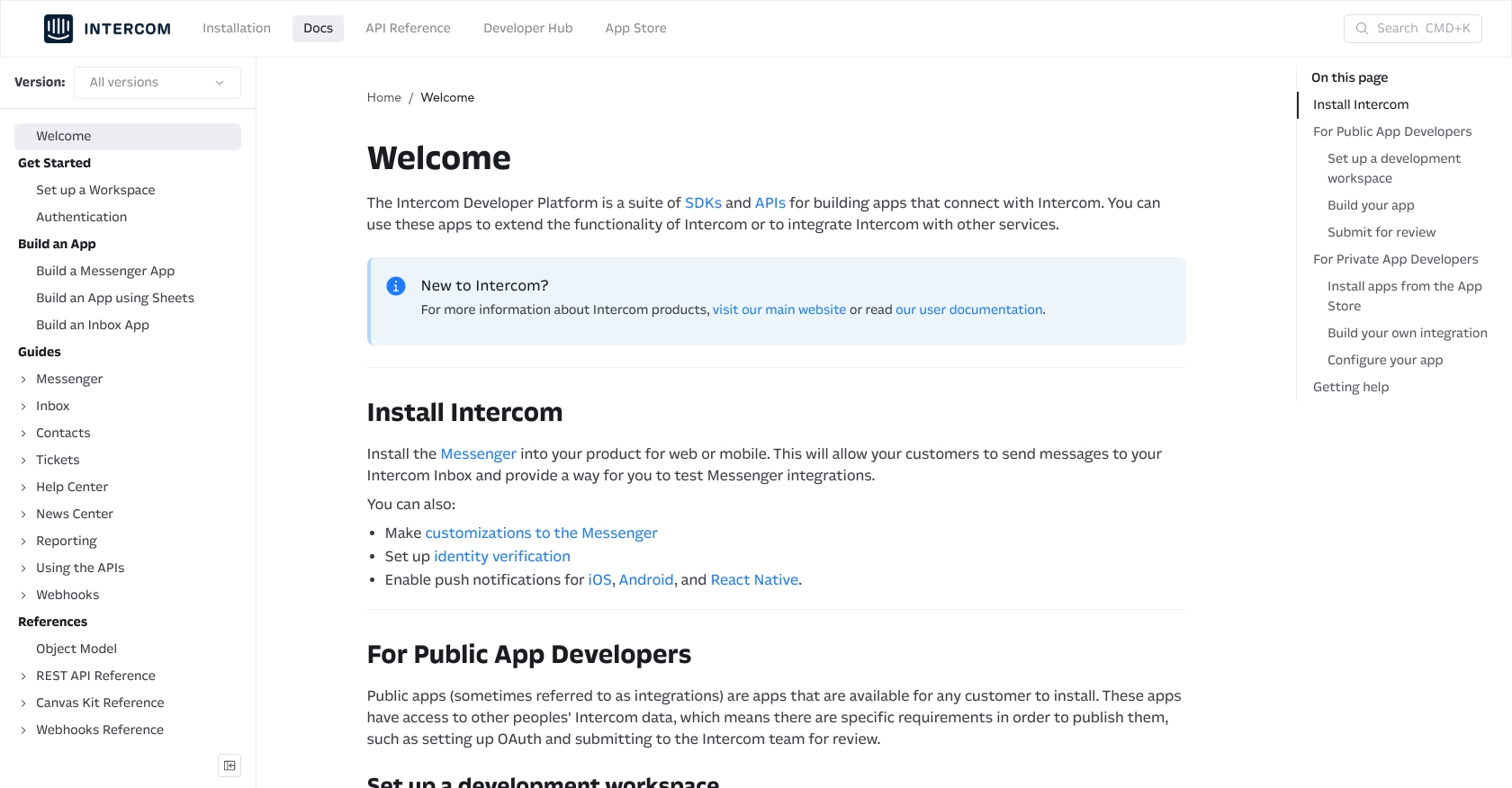
sbb-itb-96038d7
Making API Calls to Create Notes in Intercom Using JavaScript
To interact with the Intercom API and create notes, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the necessary code, and handling responses from the API.
Setting Up Your JavaScript Environment for Intercom API Integration
Before making API calls, ensure you have Node.js installed on your machine. You will also need the axios
library to handle HTTP requests. Install it using npm:
npm install axios
Writing JavaScript Code to Create Notes in Intercom
With your environment ready, you can now write the code to create a note for a contact in Intercom. Here's a step-by-step guide:
- Create a new JavaScript file, for example,
createNote.js
. - Import the
axios
library and set up your API endpoint and headers:
const axios = require('axios');
const endpoint = 'https://api.intercom.io/contacts/{contact_id}/notes';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json',
'Intercom-Version': '2.11'
};
Replace YOUR_ACCESS_TOKEN
with the access token obtained during OAuth setup and {contact_id}
with the specific contact ID.
- Define the note data and make a POST request to create the note:
const noteData = {
body: 'This is a new note',
admin_id: 'YOUR_ADMIN_ID'
};
axios.post(endpoint, noteData, { headers })
.then(response => {
console.log('Note created successfully:', response.data);
})
.catch(error => {
console.error('Error creating note:', error.response.data);
});
Replace YOUR_ADMIN_ID
with the admin ID responsible for the note.
Verifying Successful Note Creation in Intercom
After running your script, you should see a confirmation message in the console. To verify the note creation, log in to your Intercom account and check the contact's profile for the new note.
Handling Errors and Common Issues with Intercom API
When making API calls, it's essential to handle potential errors. The Intercom API may return various error codes, such as 401 for unauthorized access or 404 if the contact is not found. Ensure your access token is valid and the contact ID is correct.
For more details on error handling, refer to the Intercom API documentation.
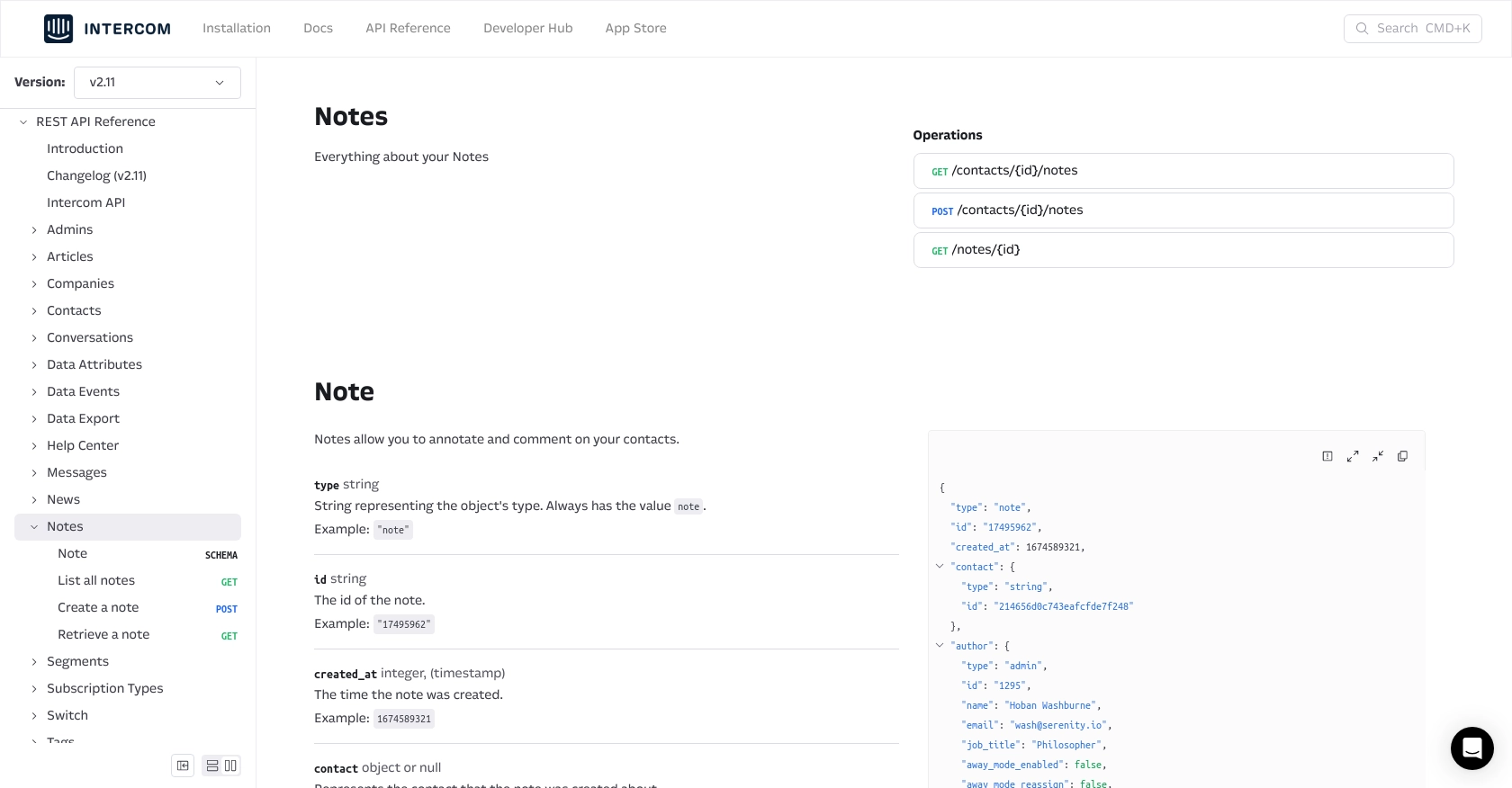
Conclusion and Best Practices for Intercom API Integration
Integrating with the Intercom API to create notes in JavaScript can significantly enhance your customer support workflows by allowing seamless documentation of interactions. By following the steps outlined in this guide, you can efficiently set up OAuth authentication, make API calls, and handle potential errors.
Best Practices for Secure and Efficient Intercom API Usage
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Avoid hardcoding them in your source code. Consider using environment variables or a secure vault.
- Handle Rate Limiting: Be mindful of Intercom's rate limits to avoid throttling. Implement retry logic with exponential backoff to manage rate limit errors gracefully.
- Data Standardization: Ensure that the data you send and receive is standardized. This helps maintain consistency across different systems and improves data integrity.
Streamlining Integrations with Endgrate
While building integrations manually can be rewarding, it can also be time-consuming and complex, especially when dealing with multiple platforms. Endgrate offers a streamlined solution by providing a unified API endpoint that connects to various platforms, including Intercom.
By using Endgrate, you can save time and resources, allowing you to focus on your core product development. With its intuitive integration experience, you can build once for each use case and effortlessly manage multiple integrations.
Explore how Endgrate can simplify your integration processes by visiting Endgrate today.
Read More
Ready to get started?