Using the Salesloft API to Get Tasks in Python
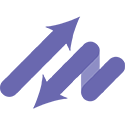
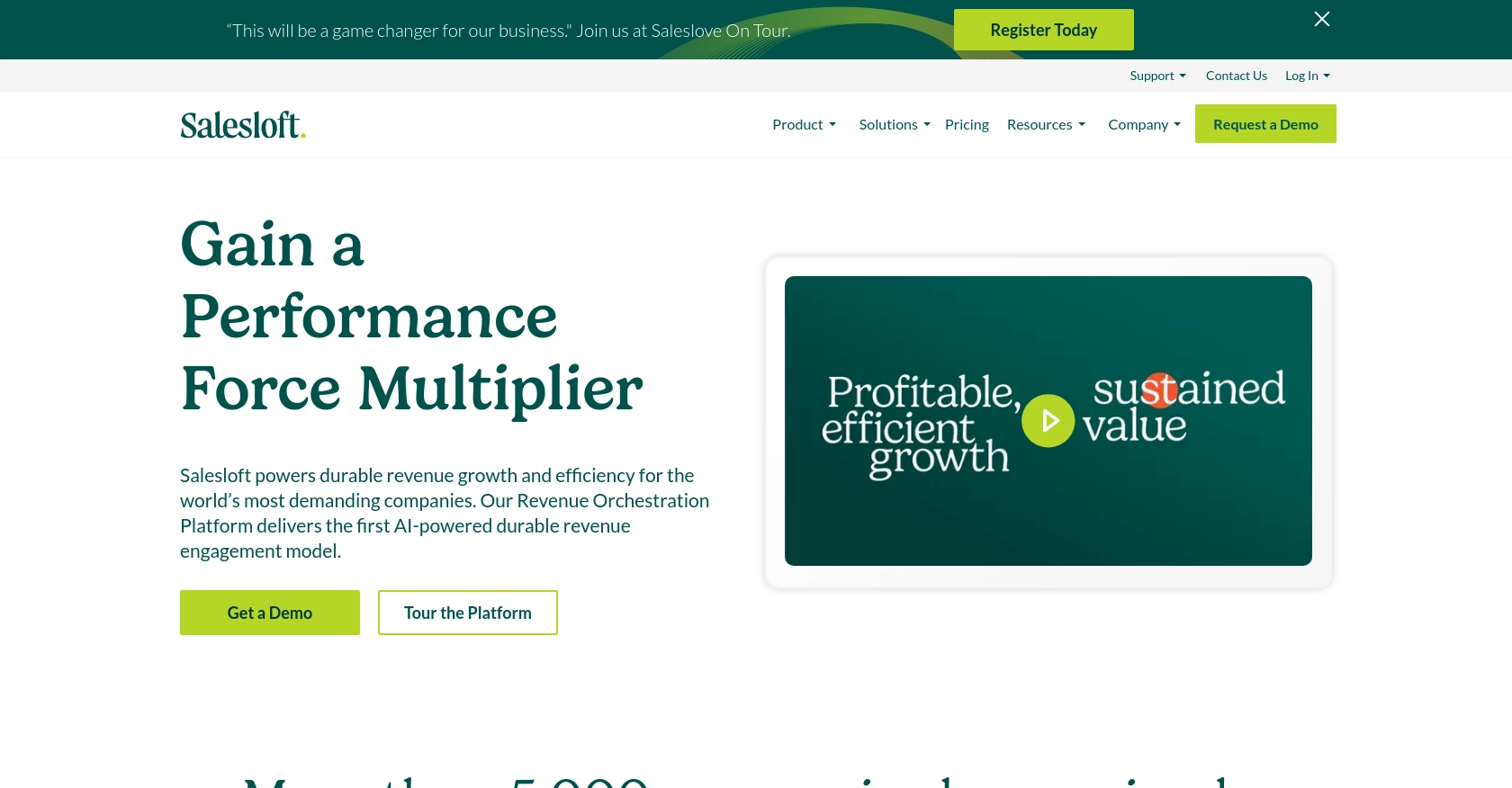
Introduction to Salesloft API
Salesloft is a powerful sales engagement platform designed to enhance the efficiency and effectiveness of sales teams. It offers a suite of tools that streamline the sales process, enabling sales professionals to connect with prospects more effectively and close deals faster.
Integrating with the Salesloft API allows developers to automate and manage sales tasks, improving workflow and productivity. For example, a developer might use the Salesloft API to retrieve task data, enabling the automation of task tracking and reporting within a custom dashboard.
Setting Up Your Salesloft Test Account for API Integration
Before you begin interacting with the Salesloft API, you need to set up a test account. This will allow you to safely experiment with API calls without affecting live data.
Creating a Salesloft Sandbox Account
If you don't already have a Salesloft account, you can sign up for a free trial or demo account on the Salesloft website. This will provide you with access to the necessary features for testing API interactions.
- Visit the Salesloft website and navigate to the sign-up page.
- Follow the instructions to create your account. Once completed, you'll have access to the Salesloft dashboard.
Generating OAuth Credentials for Salesloft API
Salesloft uses OAuth 2.0 for authentication, which requires you to create an OAuth application to obtain the necessary credentials.
- Log in to your Salesloft account and navigate to Your Applications under the account settings.
- Select OAuth Applications and click on Create New.
- Fill in the required fields and save your application. You will receive your Client ID, Client Secret, and Redirect URI.
These credentials are essential for the OAuth authorization process.
Authorizing Your Application with Salesloft
To authorize your application, you need to obtain an authorization code and exchange it for an access token.
- Direct the user to the following URL, replacing
YOUR_CLIENT_ID
andYOUR_REDIRECT_URI
with your application's details: - Upon user approval, the authorization code will be sent to your redirect URI.
- Exchange the authorization code for an access token by making a POST request to the token endpoint:
- Store the access token securely, as it will be used to authenticate API requests.
https://accounts.salesloft.com/oauth/authorize?client_id=YOUR_CLIENT_ID&redirect_uri=YOUR_REDIRECT_URI&response_type=code
POST https://accounts.salesloft.com/oauth/token
{
"client_id": "YOUR_CLIENT_ID",
"client_secret": "YOUR_CLIENT_SECRET",
"code": "AUTHORIZATION_CODE",
"grant_type": "authorization_code",
"redirect_uri": "YOUR_REDIRECT_URI"
}
For more details on OAuth authentication, refer to the Salesloft OAuth documentation.
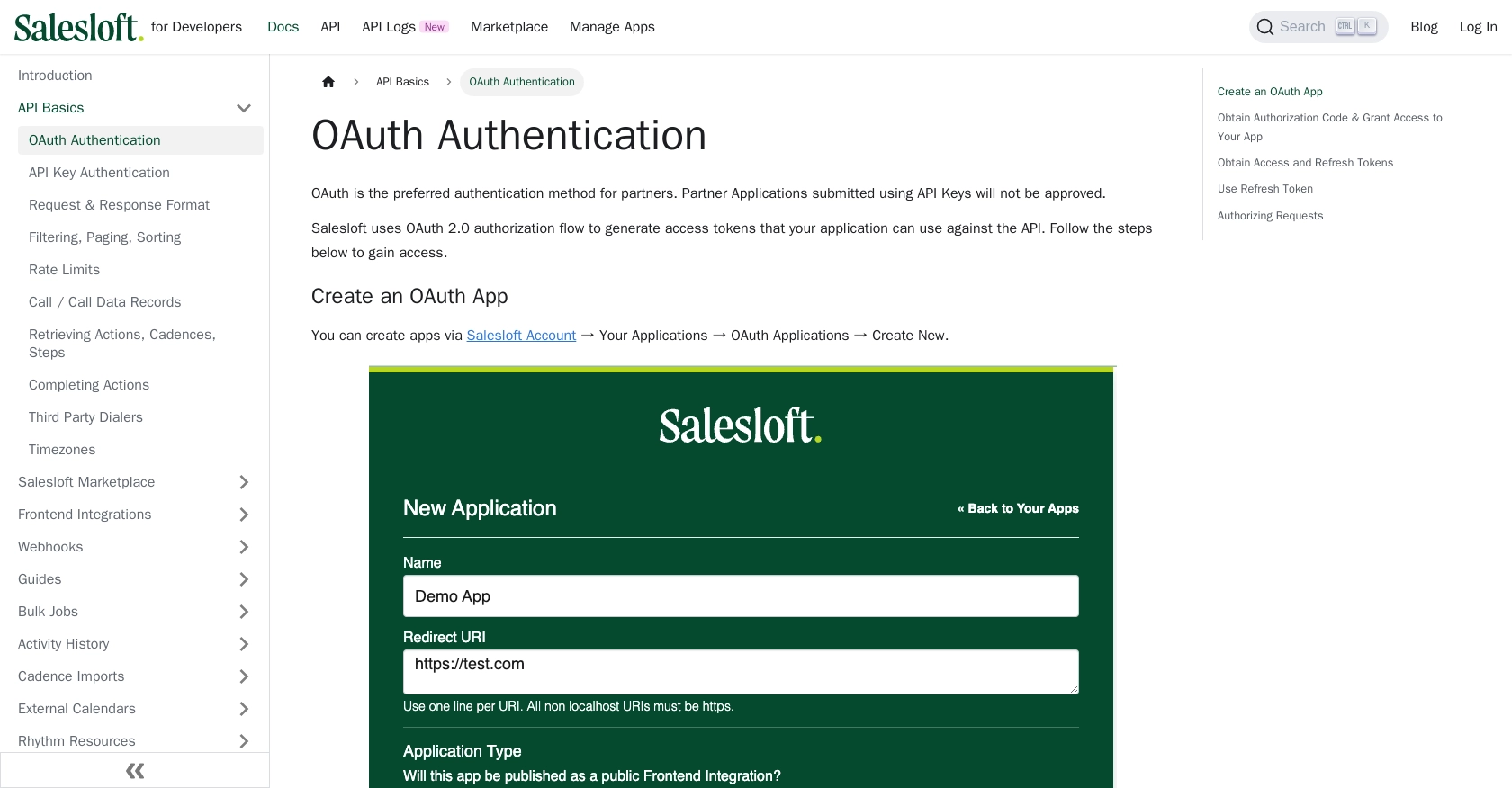
sbb-itb-96038d7
Making API Calls to Retrieve Tasks from Salesloft Using Python
To interact with the Salesloft API and retrieve tasks, you'll need to use Python. This section will guide you through the process of setting up your environment, making the API call, and handling the response.
Setting Up Your Python Environment for Salesloft API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Install Python 3.11.1 from the official Python website if you haven't already.
- Use pip to install the
requests
library by running the following command in your terminal:
pip install requests
Executing the Salesloft API Call to Fetch Tasks
With your environment set up, you can now proceed to make the API call to retrieve tasks from Salesloft. Follow these steps:
- Create a new Python file named
get_salesloft_tasks.py
and add the following code: - Replace
YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth process. - Run the script using the following command:
import requests
# Define the API endpoint and headers
endpoint = "https://api.salesloft.com/v2/tasks"
headers = {
"Authorization": "Bearer YOUR_ACCESS_TOKEN",
"Accept": "application/json"
}
# Make a GET request to the Salesloft API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
tasks = response.json().get('data', [])
for task in tasks:
print(f"Task ID: {task['id']}, Subject: {task['subject']}, Due Date: {task['due_date']}")
else:
print(f"Failed to retrieve tasks: {response.status_code} - {response.text}")
python get_salesloft_tasks.py
Handling API Responses and Errors from Salesloft
Upon executing the script, you should see a list of tasks printed in the console. If the request fails, the script will output the error code and message. Common HTTP status codes include:
- 200 OK: The request was successful.
- 403 Forbidden: Access is denied. Check your access token.
- 404 Not Found: The endpoint is incorrect.
- 422 Unprocessable Entity: There was an issue with the request parameters.
For more details on handling errors, refer to the Salesloft Request & Response Format documentation.
Verifying Task Retrieval in Salesloft Sandbox
After running the script, you can verify the retrieved tasks by logging into your Salesloft sandbox account and checking the tasks section. Ensure the tasks match the output from your script.
For additional information on API calls, refer to the Salesloft API documentation.
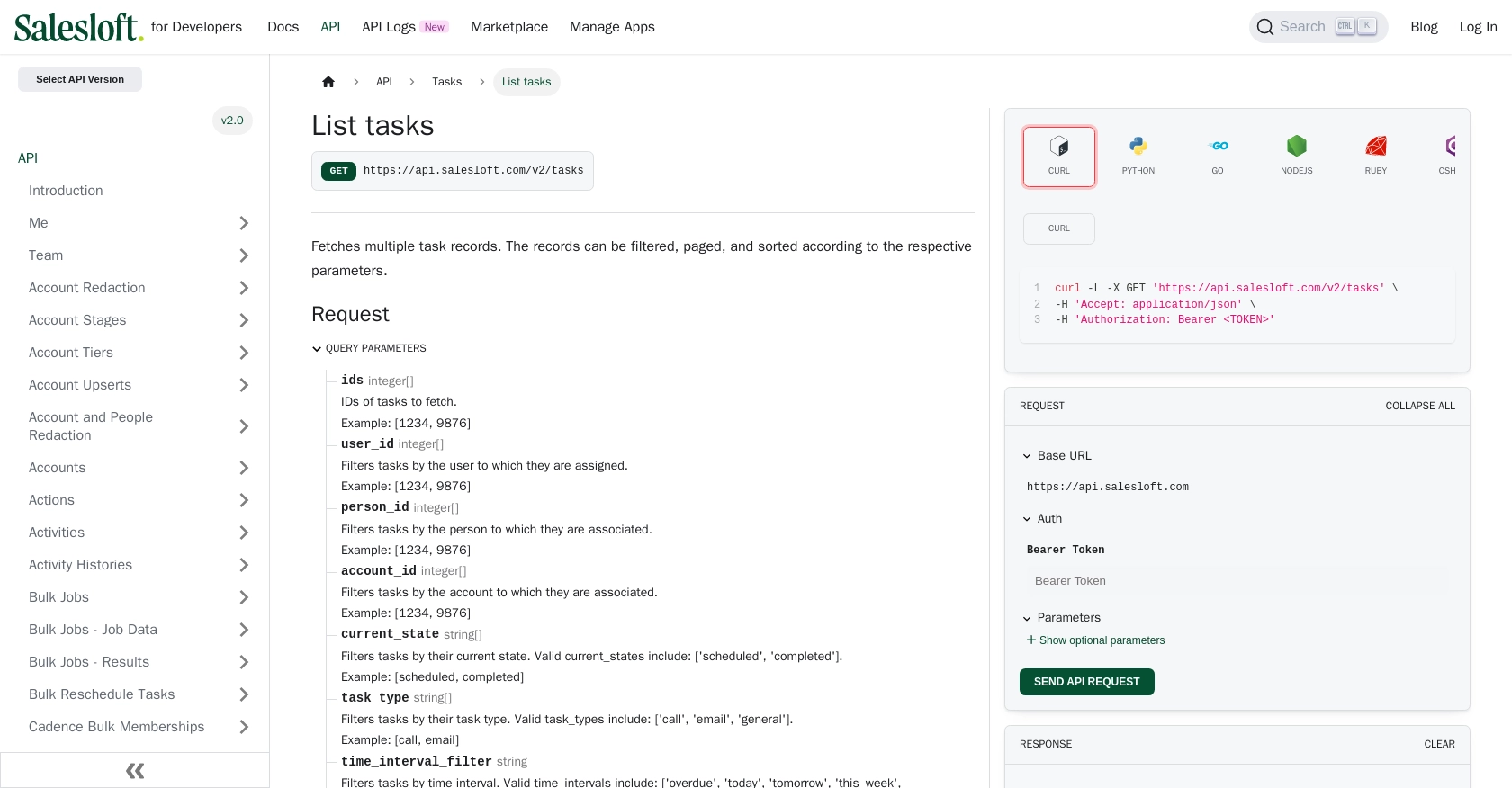
Conclusion and Best Practices for Using Salesloft API in Python
Integrating with the Salesloft API to retrieve tasks using Python can significantly enhance your sales team's efficiency by automating task management and reporting. By following the steps outlined in this guide, you can seamlessly connect to Salesloft and leverage its powerful features.
Best Practices for Secure and Efficient Salesloft API Integration
- Securely Store Credentials: Always store your OAuth credentials, including the access token and refresh token, securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limits: Be mindful of Salesloft's rate limits, which are set at 600 requests per minute. Implement logic to handle rate limit responses and retry requests if necessary. For more details, refer to the Salesloft Rate Limits documentation.
- Implement Error Handling: Ensure your application gracefully handles errors by checking HTTP status codes and implementing retry logic where appropriate. This will improve the reliability of your integration.
- Optimize Data Handling: When retrieving large datasets, consider using pagination to manage data efficiently. Salesloft supports pagination parameters to help you control the volume of data returned in each request.
Enhancing Your Integration Strategy with Endgrate
While building custom integrations with Salesloft can be rewarding, it can also be time-consuming and complex. Endgrate offers a streamlined solution for managing multiple integrations across various platforms. By using Endgrate, you can:
- Save time and resources by outsourcing integration development, allowing you to focus on your core product.
- Build once for each use case instead of multiple times for different integrations, simplifying your development process.
- Provide an intuitive integration experience for your customers, enhancing user satisfaction and engagement.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
- https://endgrate.com/provider/salesloft
- https://developers.salesloft.com/docs/platform/api-basics/oauth-authentication/
- https://developers.salesloft.com/docs/platform/api-basics/request-response-format/
- https://developers.salesloft.com/docs/platform/api-basics/filtering-paging-sorting/
- https://developers.salesloft.com/docs/platform/api-basics/rate-limits/
- https://developers.salesloft.com/docs/api/tasks-index/
Ready to get started?