Using the Pipeliner CRM API to Get Contacts in Python
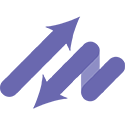
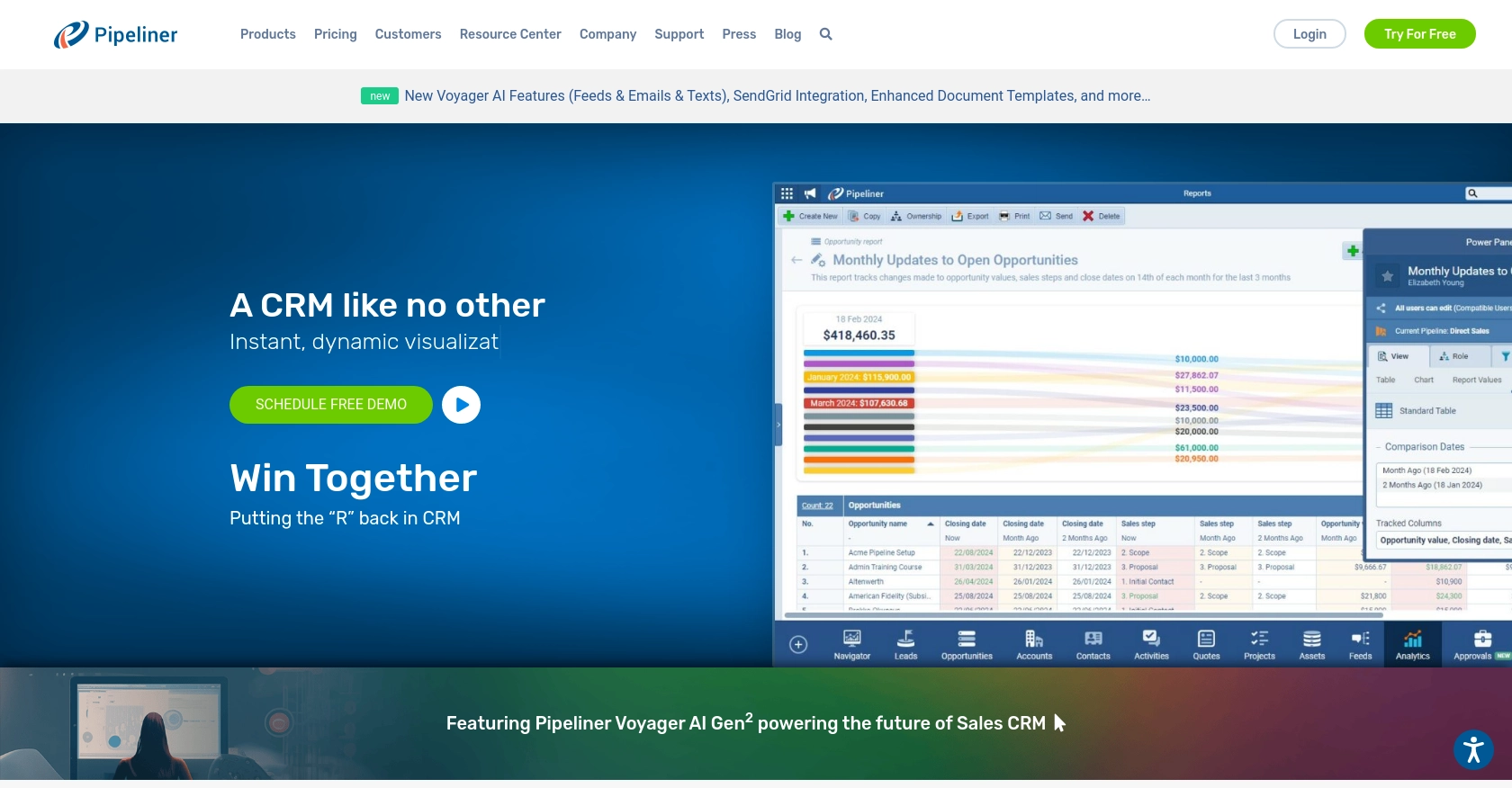
Introduction to Pipeliner CRM
Pipeliner CRM is a powerful customer relationship management platform designed to enhance sales processes and improve team collaboration. With its intuitive interface and robust features, Pipeliner CRM helps businesses manage leads, track sales activities, and analyze performance metrics effectively.
Integrating with the Pipeliner CRM API allows developers to access and manage customer data seamlessly. For example, a developer might use the API to retrieve contact information, enabling the automation of sales outreach or the synchronization of customer data across different platforms.
Setting Up Your Pipeliner CRM Test Account
Before you can start interacting with the Pipeliner CRM API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Follow these steps to get started:
Creating a Pipeliner CRM Account
- Visit the Pipeliner CRM website and sign in using your Pipeliner CRM account credentials. If you don't have an account, you can create one by following the on-screen instructions.
- Once logged in, select your team space to proceed to the administration panel.
Generating Pipeliner CRM API Keys for Authentication
Pipeliner CRM uses Basic Authentication, which requires a username and password generated as API keys. Here's how to obtain them:
- In the administration panel, navigate to the Unit, Users & Roles tab.
- Open the Applications section and create a new application.
- Once the application is created, click on Show API Access to reveal your API username and password. These credentials are generated only once, so make sure to store them securely.
Understanding Pipeliner CRM API Authentication
With your API keys in hand, you can now authenticate your API requests. The username and password will be used in the Basic Authentication header for each API call. Remember to keep these credentials private to ensure the security of your data.
For more detailed information on authentication, you can refer to the Pipeliner CRM Authentication Documentation.
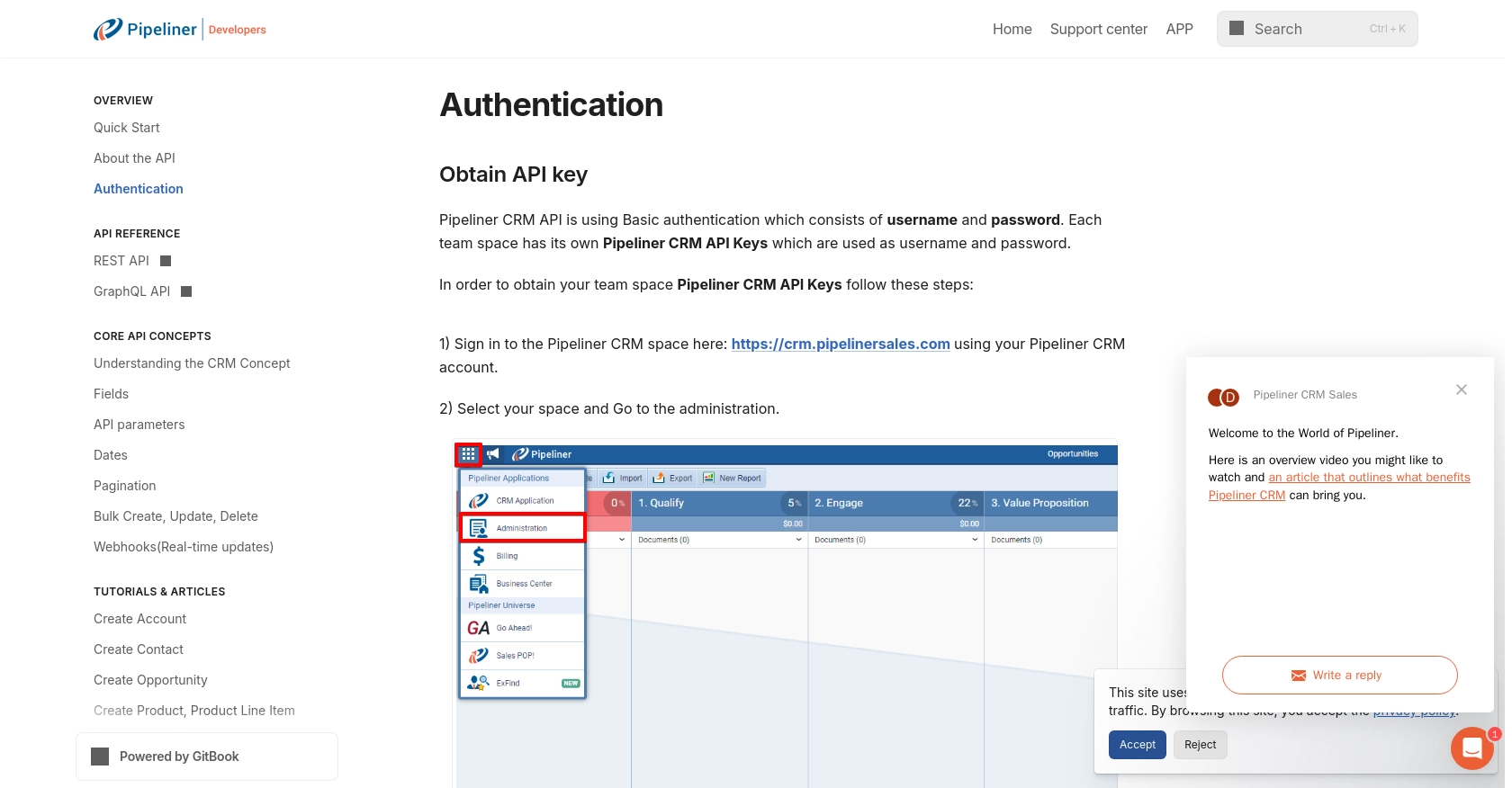
sbb-itb-96038d7
Making API Calls to Retrieve Contacts from Pipeliner CRM Using Python
To interact with the Pipeliner CRM API and retrieve contact information, you'll need to set up your Python environment and make the necessary API calls. This section will guide you through the process, including setting up Python, installing dependencies, and executing the API call to fetch contacts.
Setting Up Your Python Environment for Pipeliner CRM API
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1
- The Python package installer, pip
Once these are installed, open your terminal or command prompt and install the requests
library, which will be used to make HTTP requests:
pip install requests
Writing Python Code to Fetch Contacts from Pipeliner CRM
Now that your environment is ready, you can write the Python script to retrieve contacts from Pipeliner CRM. Create a file named get_pipeliner_contacts.py
and add the following code:
import requests
from requests.auth import HTTPBasicAuth
# Set the API endpoint and authentication details
space_id = "your_space_id"
service_url = "your_service_url"
endpoint = f"{service_url}/entities/Contacts"
username = "your_api_username"
password = "your_api_password"
# Make a GET request to the API
response = requests.get(endpoint, auth=HTTPBasicAuth(username, password))
# Check if the request was successful
if response.status_code == 200:
data = response.json()
# Loop through the contacts and print their information
for contact in data["data"]:
print(contact)
else:
print(f"Failed to retrieve contacts: {response.status_code} - {response.text}")
Replace your_space_id
, your_service_url
, your_api_username
, and your_api_password
with the actual values obtained from your Pipeliner CRM account setup.
Executing the Python Script and Handling Responses
Run the script from your terminal or command line using the following command:
python get_pipeliner_contacts.py
If the request is successful, you should see the contact information printed in the console. If there's an error, the script will output the error code and message.
Handling Pagination in Pipeliner CRM API
Pipeliner CRM API supports pagination, allowing you to retrieve large sets of data efficiently. By default, the API returns up to 30 records per request, but you can adjust this limit up to 100. Use the end_cursor
and after
parameters to paginate through the results:
has_next_page = True
after_cursor = None
while has_next_page:
params = {"after": after_cursor} if after_cursor else {}
response = requests.get(endpoint, auth=HTTPBasicAuth(username, password), params=params)
if response.status_code == 200:
data = response.json()
for contact in data["data"]:
print(contact)
has_next_page = data["page_info"]["has_next_page"]
after_cursor = data["page_info"]["end_cursor"]
else:
print(f"Failed to retrieve contacts: {response.status_code} - {response.text}")
break
This loop continues fetching contacts until all pages are retrieved. For more details on pagination, refer to the Pipeliner CRM Pagination Documentation.
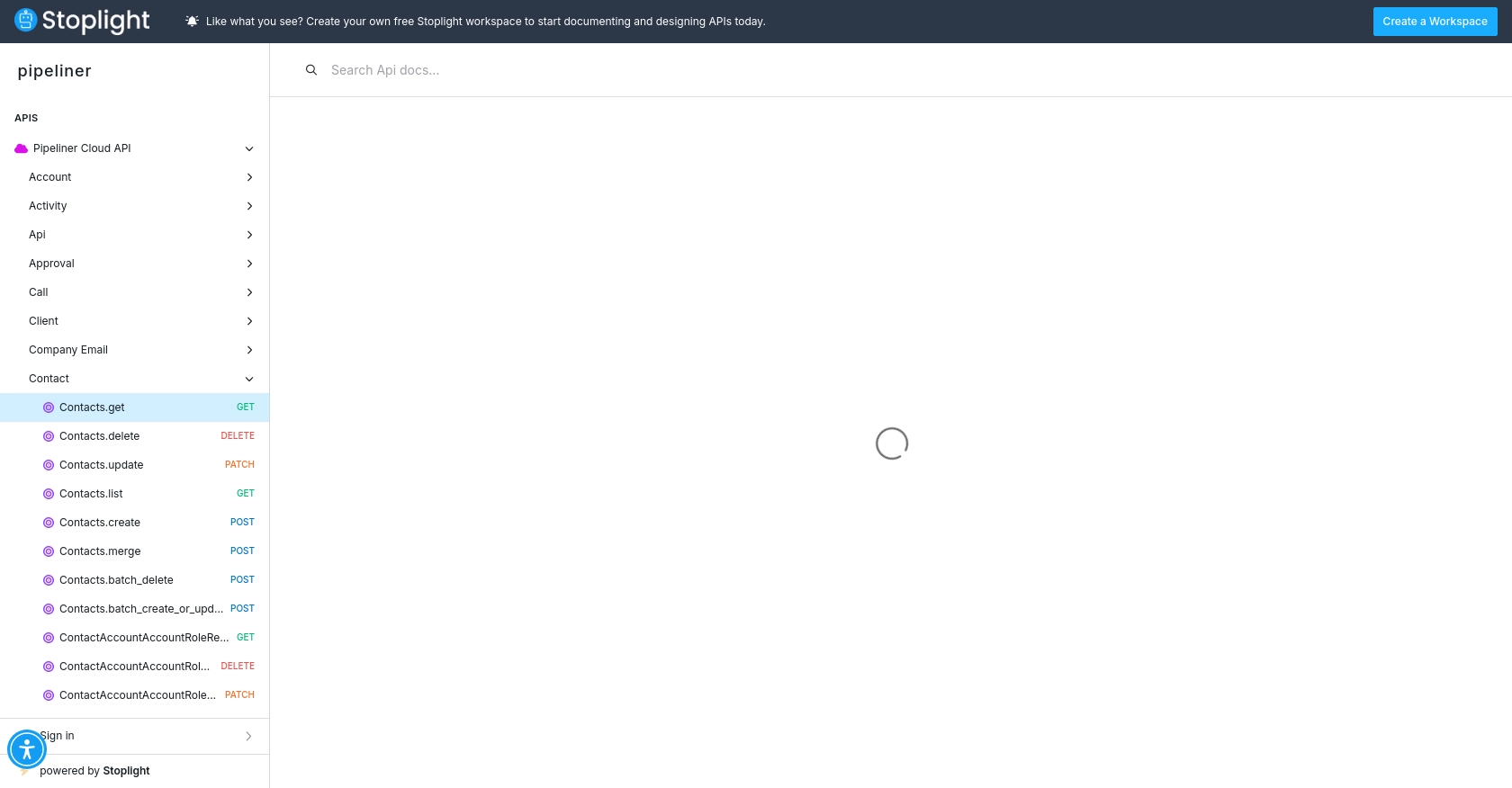
Conclusion and Best Practices for Using Pipeliner CRM API in Python
Integrating with the Pipeliner CRM API using Python provides a powerful way to manage and automate customer relationship processes. By following the steps outlined in this guide, you can efficiently retrieve and handle contact data, ensuring seamless synchronization across platforms.
Best Practices for Secure and Efficient API Integration
- Secure Storage of Credentials: Always store your API credentials securely. Consider using environment variables or secure vaults to keep your username and password safe.
- Handling Rate Limits: Be mindful of any rate limits imposed by the API. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Transformation and Standardization: Ensure that the data retrieved from Pipeliner CRM is transformed and standardized to fit your application's data model. This will help maintain consistency and accuracy across systems.
- Error Handling: Implement robust error handling to manage different HTTP status codes and API responses. This will help in diagnosing issues quickly and maintaining a smooth integration experience.
Streamlining Integrations with Endgrate
While building integrations with Pipeliner CRM can be rewarding, it can also be time-consuming and complex, especially when managing multiple integrations. Endgrate offers a streamlined solution by providing a unified API endpoint that connects to various platforms, including Pipeliner CRM.
By using Endgrate, you can save time and resources, allowing you to focus on your core product development. With its intuitive integration experience, Endgrate simplifies the process, enabling you to build once for each use case and easily manage multiple integrations.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate and discover the benefits of a unified integration platform.
Read More
Ready to get started?