How to Create Annotations with the Mixpanel API in Python
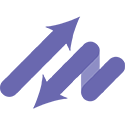
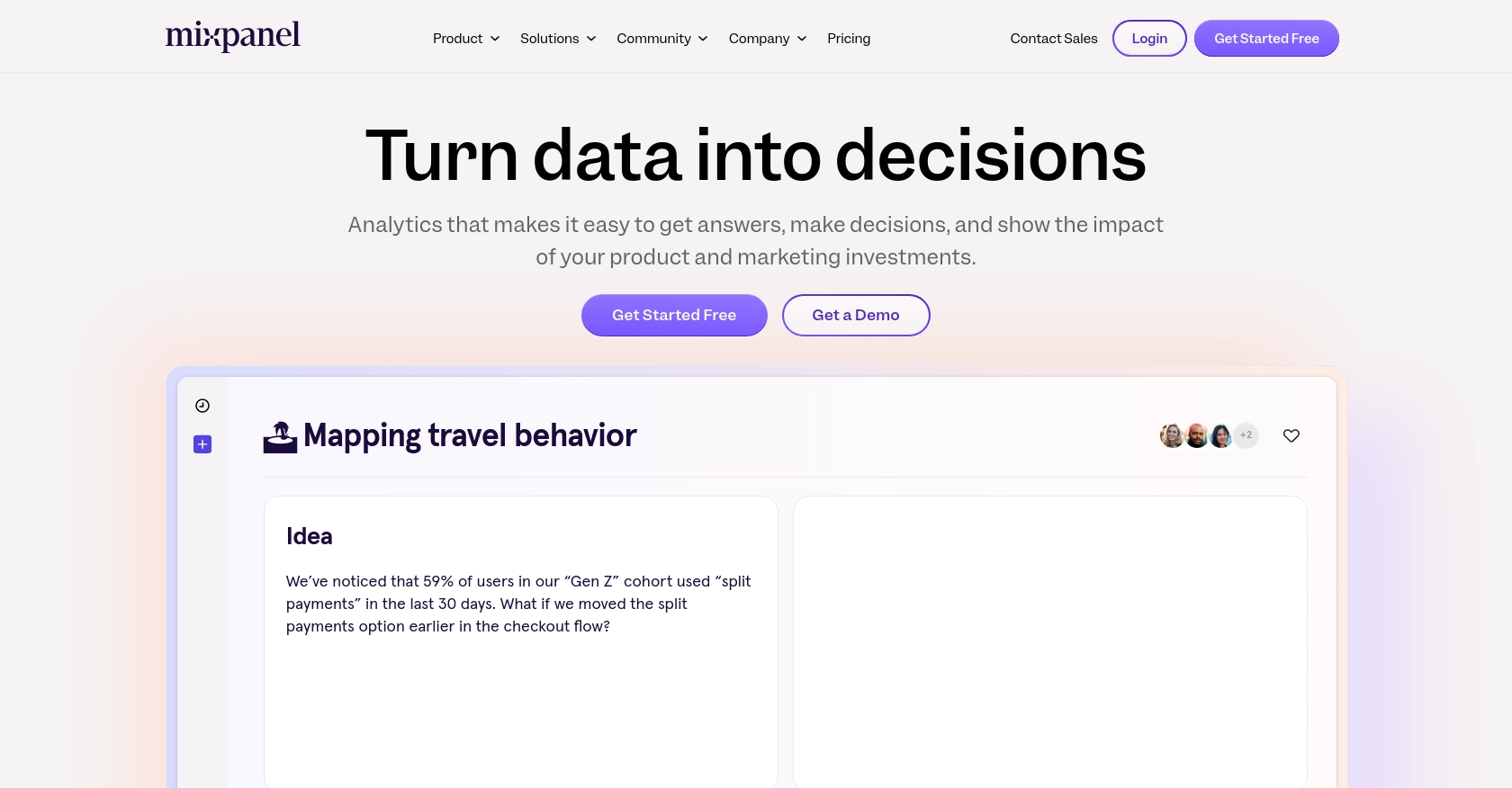
Introduction to Mixpanel API for Creating Annotations
Mixpanel is a powerful analytics platform that helps businesses understand user interactions with their products. By providing deep insights into user behavior, Mixpanel enables companies to make data-driven decisions to enhance user experience and drive growth.
Integrating with Mixpanel's API allows developers to automate and customize data analysis processes. One valuable feature is the ability to create annotations, which can be used to mark significant events or changes in your data timeline. For example, a developer might want to annotate a spike in user activity following a marketing campaign launch, providing context for future analysis.
Setting Up Your Mixpanel Test Account for API Integration
Before you can start creating annotations with the Mixpanel API, you need to set up a Mixpanel account. This will allow you to access the necessary credentials and permissions to interact with the API.
Creating a Mixpanel Account
If you don't have a Mixpanel account, you can sign up for a free trial on the Mixpanel website. Follow the instructions to create your account and log in to the Mixpanel dashboard.
Generating Service Account Credentials for Mixpanel API
Mixpanel uses service accounts for authentication, which are ideal for non-human entities like scripts or backend services. Follow these steps to create a service account:
- Navigate to your organization settings in the Mixpanel dashboard.
- Go to the Service Accounts tab.
- Click on Create Service Account.
- Select the role and projects you want the service account to access.
- Save the service account username and secret securely, as you won't be able to access the secret again.
For more details, refer to the Mixpanel Service Accounts documentation.
Authenticating with Mixpanel API Using Python
Mixpanel's API uses HTTP Basic Auth for service accounts. Here's how you can authenticate using Python:
import requests
# Replace with your service account credentials
username = 'your_service_account_username'
secret = 'your_service_account_secret'
# Example API call to authenticate
response = requests.get(
'https://mixpanel.com/api/app/me',
auth=(username, secret)
)
print(response.json())
Ensure you replace your_service_account_username
and your_service_account_secret
with your actual credentials.
Verifying Your Setup
After setting up your service account and authenticating, you can verify your setup by making a test API call. If successful, you should receive a response with your account details.
For more information on rate limits and best practices, refer to the Mixpanel Rate Limits documentation.
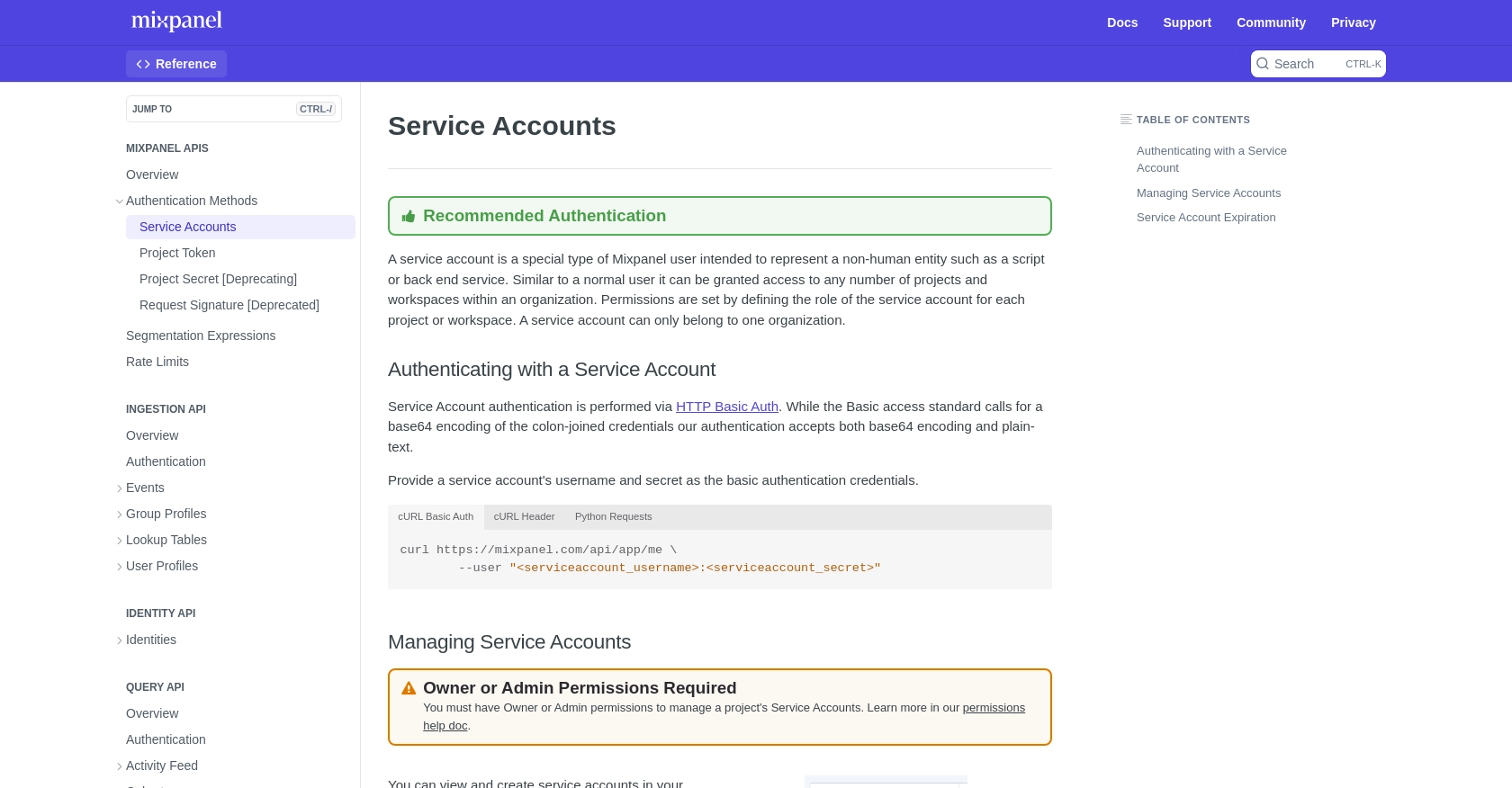
sbb-itb-96038d7
How to Make the Mixpanel API Call to Create Annotations Using Python
Now that you have set up your Mixpanel account and authenticated using a service account, you can proceed to create annotations using the Mixpanel API. This section will guide you through the process of making an API call to create annotations with Python.
Setting Up Your Python Environment for Mixpanel API Integration
Before making the API call, ensure that you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you will need the requests
library to handle HTTP requests. You can install it using pip:
pip install requests
Creating Annotations with Mixpanel API in Python
To create an annotation, you need to send a POST request to the Mixpanel API endpoint. Below is a sample Python script to create an annotation:
import requests
import json
# Replace with your service account credentials
username = 'your_service_account_username'
secret = 'your_service_account_secret'
# Mixpanel API endpoint for creating annotations
url = 'https://mixpanel.com/api/app/projects/{projectId}/annotations'.format(projectId='your_project_id')
# Annotation data
data = {
"date": "2023-10-01 12:00:00",
"description": "Marketing campaign launch"
}
# Make the POST request to create an annotation
response = requests.post(
url,
auth=(username, secret),
headers={'Content-Type': 'application/json'},
data=json.dumps(data)
)
# Check if the request was successful
if response.status_code == 201:
print("Annotation created successfully:", response.json())
else:
print("Failed to create annotation:", response.status_code, response.json())
Ensure you replace your_service_account_username
, your_service_account_secret
, and your_project_id
with your actual credentials and project ID.
Understanding the Mixpanel API Response
Upon a successful request, the API will return a status code of 201, along with the details of the created annotation. If the request fails, you will receive an error code, such as 401 for unauthorized access or 403 for forbidden access. Refer to the Mixpanel Create Annotations documentation for more details on error codes.
Verifying the Annotation in Mixpanel
After creating an annotation, you can verify it by checking your Mixpanel dashboard. The annotation should appear in your project's data timeline, providing context for the specified date and description.
For more information on handling errors and best practices, refer to the Mixpanel Rate Limits documentation.
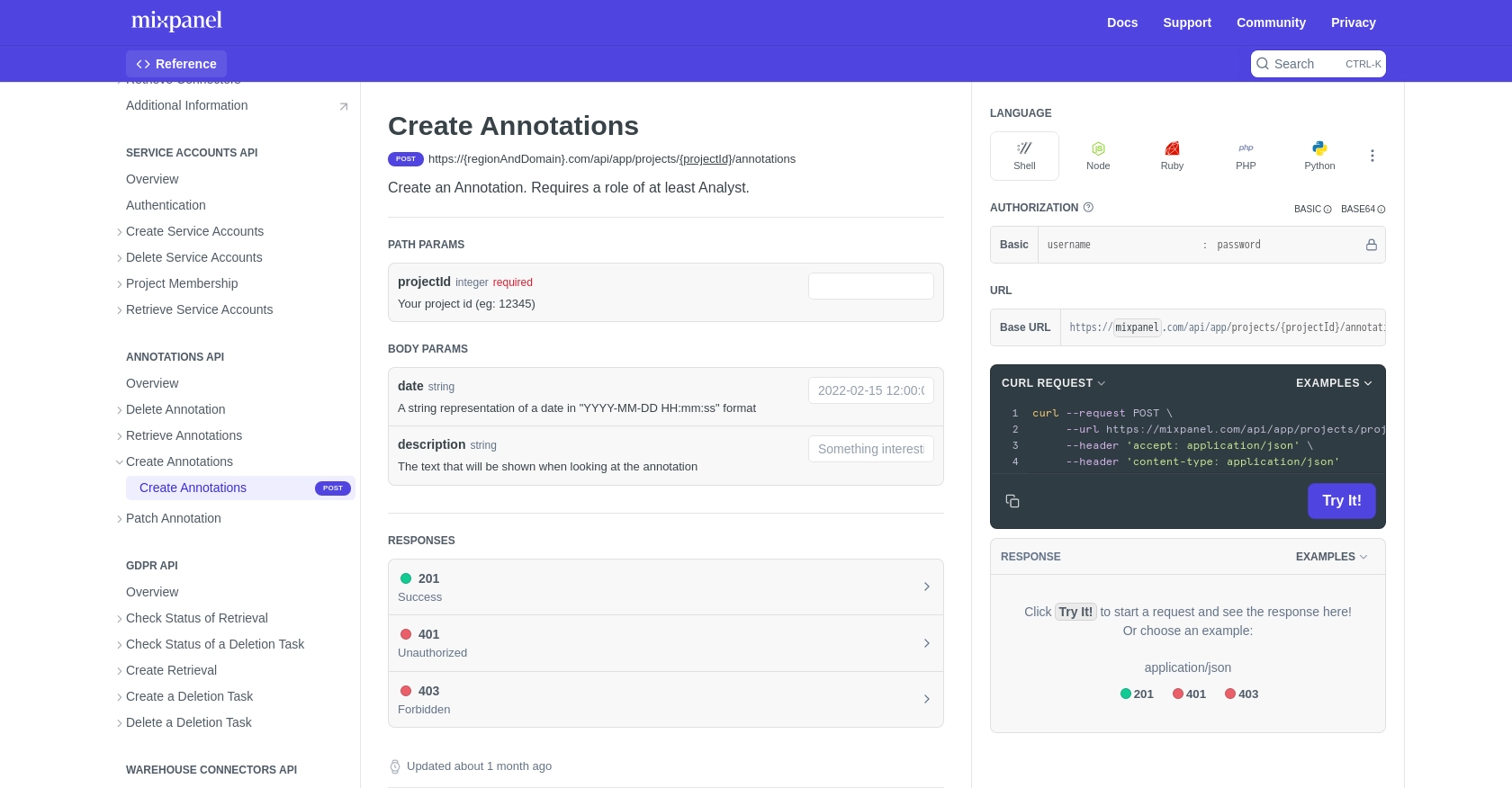
Conclusion and Best Practices for Using Mixpanel API to Create Annotations
Creating annotations with the Mixpanel API in Python offers a powerful way to enhance your data analysis by marking significant events directly on your data timeline. This capability allows developers to provide context for data spikes, feature launches, or any other noteworthy occurrences, thereby improving the interpretability of analytics.
Best Practices for Secure and Efficient Mixpanel API Integration
- Secure Storage of Credentials: Always store your service account credentials securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handling Rate Limits: Be mindful of Mixpanel's rate limits to avoid disruptions. The Query API allows a maximum of 5 concurrent queries and 60 queries per hour. Plan your API calls accordingly to prevent hitting these limits. For more details, refer to the Mixpanel Rate Limits documentation.
- Error Handling: Implement robust error handling in your code to manage potential issues such as unauthorized access (401) or forbidden requests (403). This ensures your application can gracefully handle API errors.
- Data Standardization: When creating annotations, ensure that date formats and descriptions are consistent to maintain clarity and accuracy in your data timeline.
Enhancing Integration Efficiency with Endgrate
While integrating with Mixpanel's API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Mixpanel. This allows developers to focus on core product development while outsourcing integration complexities.
By leveraging Endgrate, you can build once for each use case and avoid the need to develop multiple integrations for different platforms. This not only saves time and resources but also enhances the integration experience for your customers.
Explore how Endgrate can streamline your integration processes by visiting Endgrate and discover the benefits of a seamless integration solution.
Read More
Ready to get started?