How to Get Companies with the PipelineCRM API in Javascript
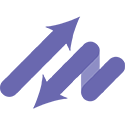
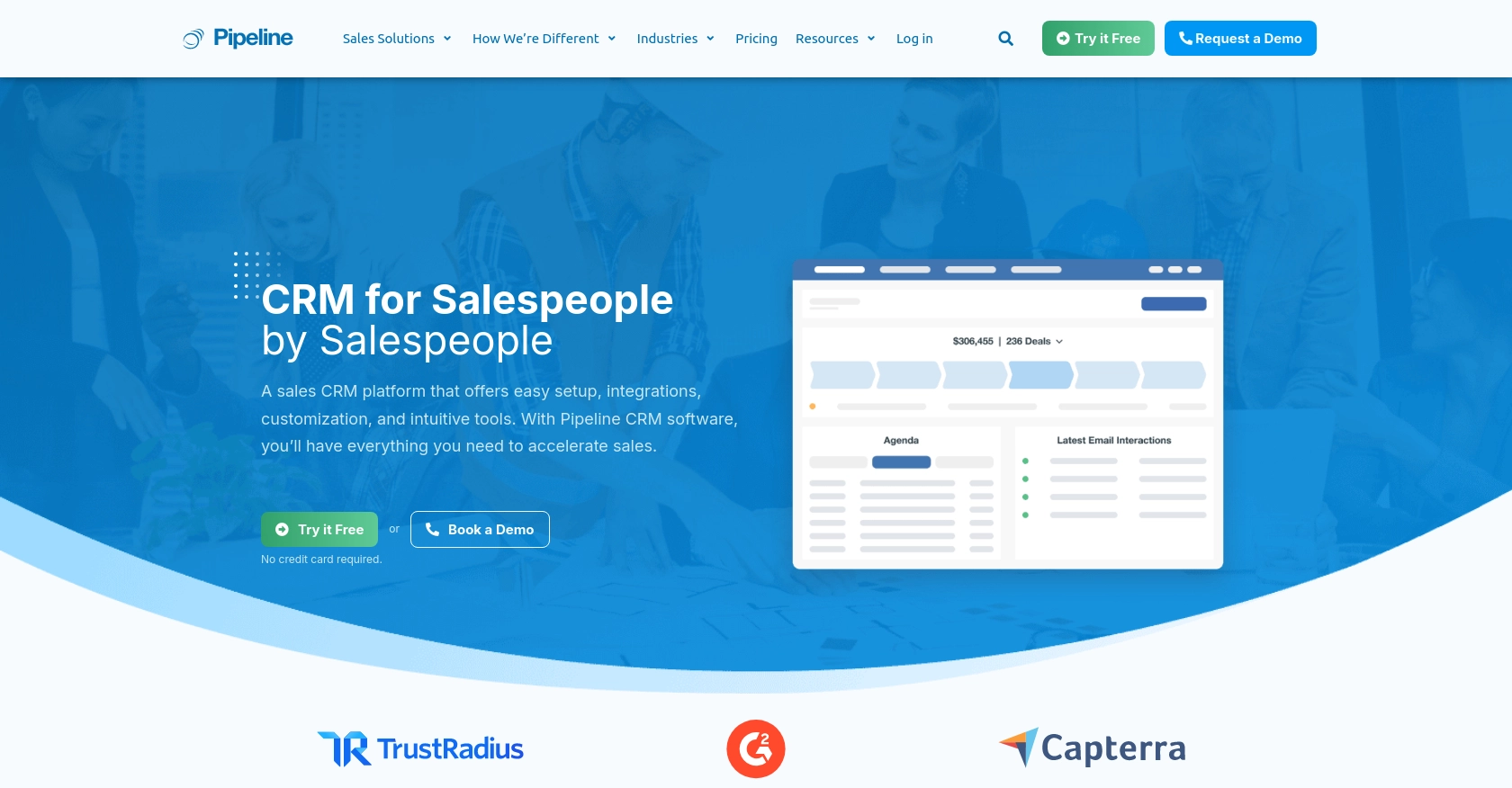
Introduction to PipelineCRM
PipelineCRM is a robust customer relationship management platform designed to enhance sales processes and improve customer interactions for businesses. It offers a comprehensive suite of tools that help sales teams manage leads, track deals, and streamline communication with clients.
Integrating with PipelineCRM's API allows developers to automate and optimize various sales operations, such as retrieving company data. For example, a developer might use the PipelineCRM API to fetch a list of companies and their details, enabling seamless integration with other business applications and enhancing data-driven decision-making.
Setting Up Your PipelineCRM Test Account
Before you can start interacting with the PipelineCRM API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting any live data.
Creating a PipelineCRM Account
If you don't already have a PipelineCRM account, you can sign up for a free trial on their website. This trial will give you access to the necessary features to test API interactions.
- Visit the PipelineCRM website.
- Click on the "Sign Up" button and fill out the registration form with your details.
- Once registered, log in to your new PipelineCRM account.
Generating an API Key for Authentication
PipelineCRM uses API key-based authentication to secure API requests. Follow these steps to generate your API key:
- Navigate to the "Settings" section of your PipelineCRM dashboard.
- Locate the "API Keys" tab and click on it.
- Click on "Generate New API Key" and provide a name for your key to easily identify it later.
- Copy the generated API key and store it securely, as you'll need it to authenticate your API requests.
With your API key in hand, you're now ready to start making API calls to PipelineCRM. In the next section, we'll explore how to use JavaScript to interact with the PipelineCRM API and retrieve company data.
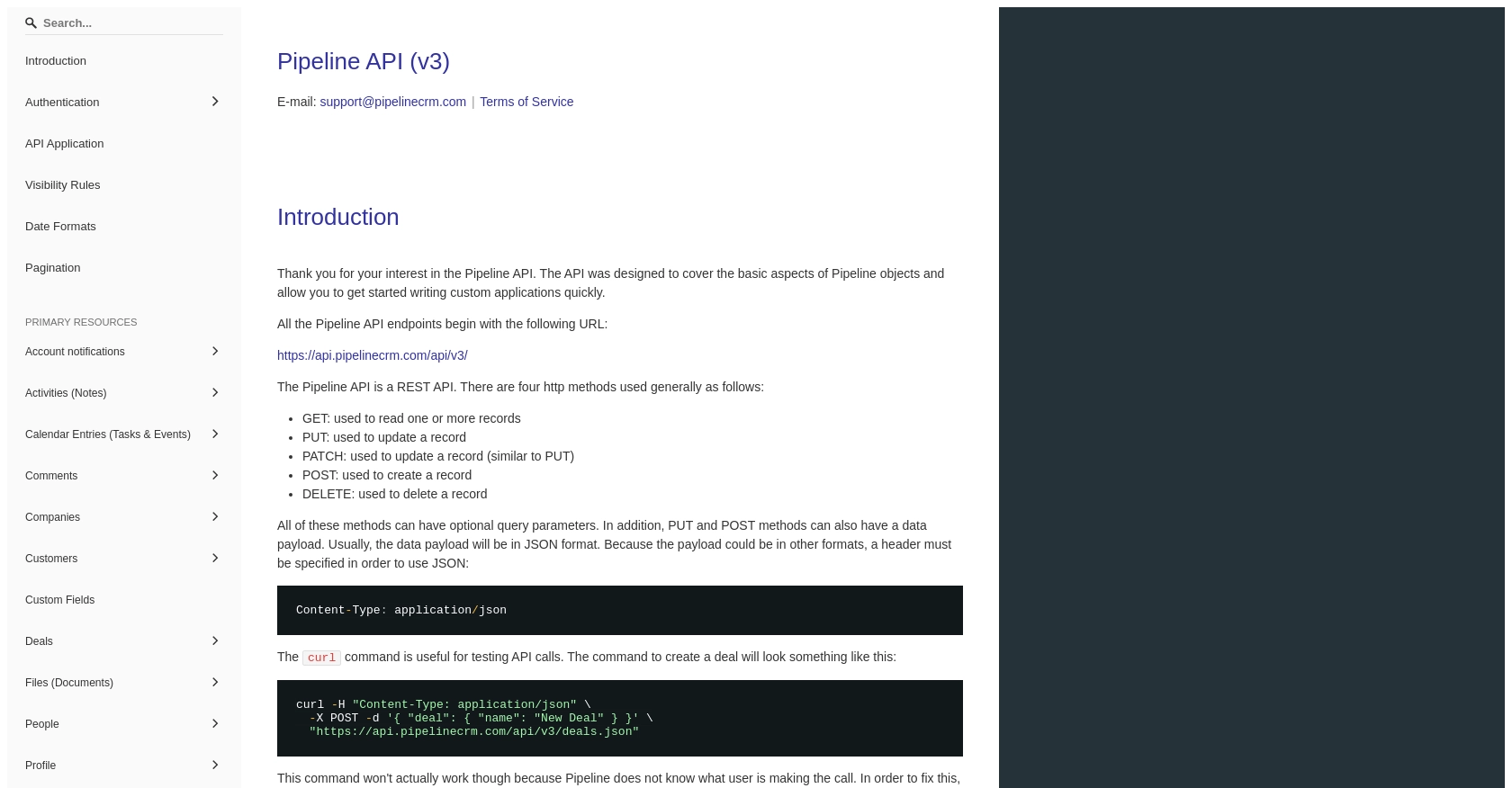
sbb-itb-96038d7
Making API Calls to Retrieve Companies with PipelineCRM in JavaScript
To interact with the PipelineCRM API using JavaScript, you'll need to set up your development environment and write code to make HTTP requests. This section will guide you through the process of fetching company data from PipelineCRM using JavaScript.
Setting Up Your JavaScript Environment
Before making API calls, ensure you have Node.js installed on your machine. Node.js allows you to run JavaScript code outside of a browser, making it ideal for server-side applications.
- Download and install Node.js from the official website.
- Verify the installation by running
node -v
andnpm -v
in your terminal to check the versions.
Installing Required Dependencies
You'll need the axios
library to make HTTP requests. Install it using npm:
npm install axios
Writing JavaScript Code to Fetch Companies
Create a new JavaScript file named getCompanies.js
and add the following code:
const axios = require('axios');
// Your PipelineCRM API key
const apiKey = 'Your_API_Key';
// Set the API endpoint
const endpoint = 'https://api.pipelinecrm.com/v3/companies';
// Configure the request headers
const headers = {
'Content-Type': 'application/json',
'Authorization': `Bearer ${apiKey}`
};
// Function to fetch companies
async function getCompanies() {
try {
const response = await axios.get(endpoint, { headers });
const companies = response.data;
console.log('Companies:', companies);
} catch (error) {
console.error('Error fetching companies:', error.response ? error.response.data : error.message);
}
}
// Execute the function
getCompanies();
Replace Your_API_Key
with the API key you generated earlier. This code uses axios
to send a GET request to the PipelineCRM API endpoint for companies. It logs the retrieved company data to the console.
Running Your JavaScript Code
Execute the script using Node.js:
node getCompanies.js
If successful, you should see a list of companies printed in the console. This confirms that your API call was successful and that you have retrieved the company data from PipelineCRM.
Handling Errors and Verifying API Responses
It's crucial to handle potential errors when making API calls. The code above includes a try-catch
block to catch and log any errors that occur during the request. Check the error.response
object for more details on the error if the request fails.
Verify the retrieved data by comparing it with the data in your PipelineCRM test account. This ensures that the API call is correctly fetching the intended information.
Conclusion and Best Practices for Using PipelineCRM API with JavaScript
Integrating with the PipelineCRM API using JavaScript can significantly enhance your sales operations by automating data retrieval and management processes. By following the steps outlined in this guide, you can efficiently fetch company data and integrate it with other business applications.
Best Practices for Secure and Efficient API Integration
- Secure API Key Storage: Always store your API keys securely. Avoid hardcoding them in your source code. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be aware of any rate limits imposed by the PipelineCRM API. Implement logic to handle rate limit responses, such as retrying requests after a delay.
- Data Standardization: Ensure that the data retrieved from the API is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement robust error handling to manage API call failures gracefully. Log errors for debugging and monitor API usage to identify potential issues.
Streamlining Integrations with Endgrate
If managing multiple integrations is becoming a challenge, consider using Endgrate to simplify the process. Endgrate provides a unified API endpoint that connects to various platforms, including PipelineCRM, allowing you to focus on your core product while outsourcing integration complexities. By building once for each use case, you can save time and resources, providing an intuitive integration experience for your customers.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website and discover the benefits of a streamlined integration process.
Read More
Ready to get started?