Using the Brevo API to Get Contacts in PHP
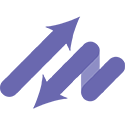
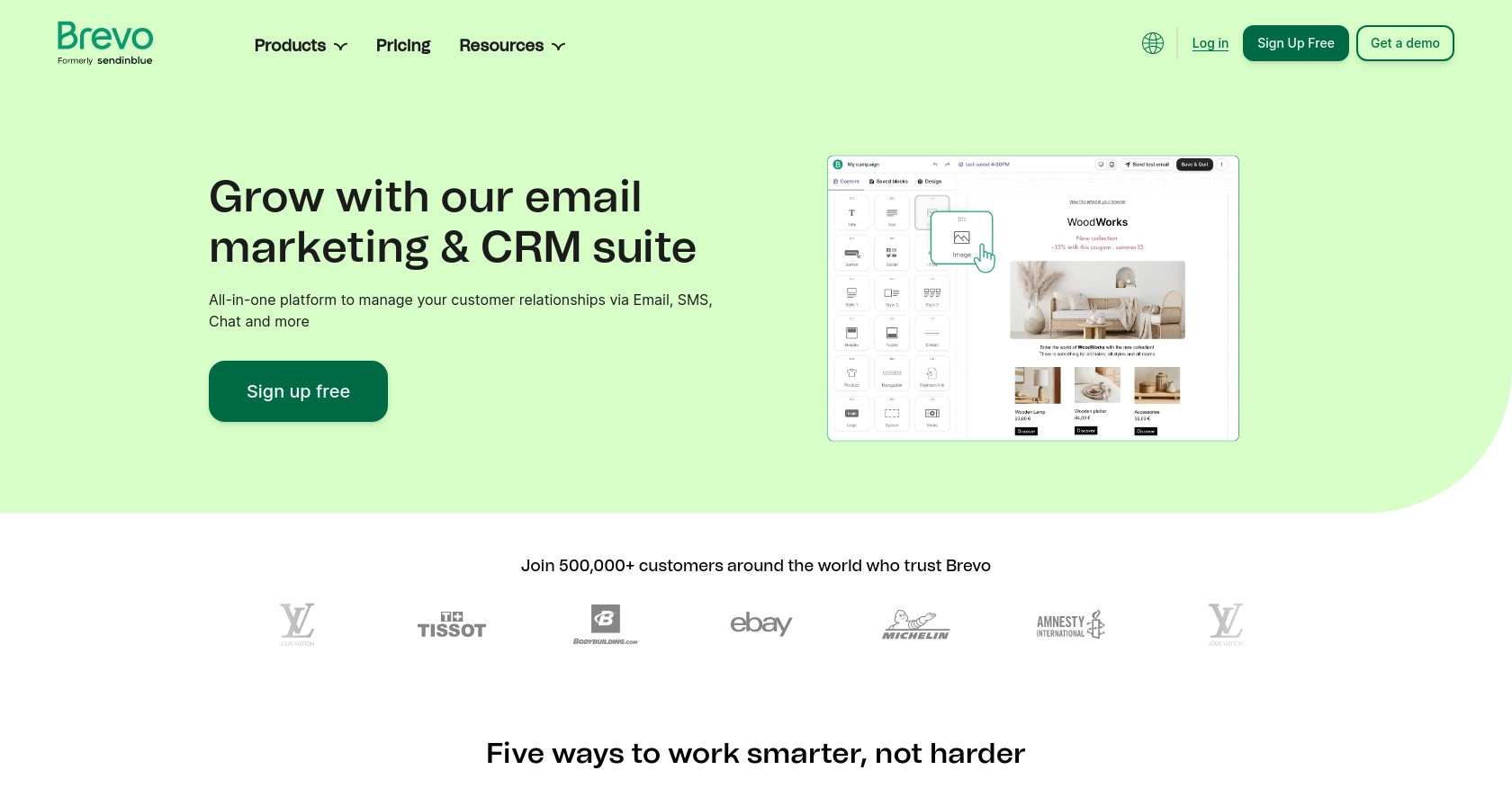
Introduction to Brevo API for Contact Management
Brevo is a versatile platform offering a suite of tools for managing customer relationships through email, SMS, and more. Its robust API allows developers to seamlessly integrate Brevo's functionalities into their applications, enhancing communication strategies and customer engagement.
Connecting with Brevo's API can empower developers to efficiently manage contact lists, automate marketing campaigns, and personalize customer interactions. For example, a developer might use the Brevo API to retrieve contact information and synchronize it with a CRM system, ensuring that customer data is always up-to-date and accessible.
Setting Up Your Brevo Account for API Access
Before you can start using the Brevo API to manage contacts, you need to set up your Brevo account and obtain an API key. This key is essential for authenticating your requests and ensuring secure access to Brevo's services.
Create a Brevo Account
If you don't already have a Brevo account, follow these steps to create one:
- Visit the Brevo signup page.
- Fill in the required information and complete the registration process.
- Confirm your email address to activate your account.
Generate an API Key in Brevo
Once your account is set up, you need to generate an API key to authenticate your API requests:
- Log in to your Brevo account.
- Click on your profile name at the top-right corner and select SMTP & API.
- Navigate to the API keys tab and click Generate a new API key.
- Name your API key for easy identification and click Generate.
- Copy the generated API key and store it securely, as you'll need it for API requests.
For more detailed instructions, you can refer to the Brevo API documentation.
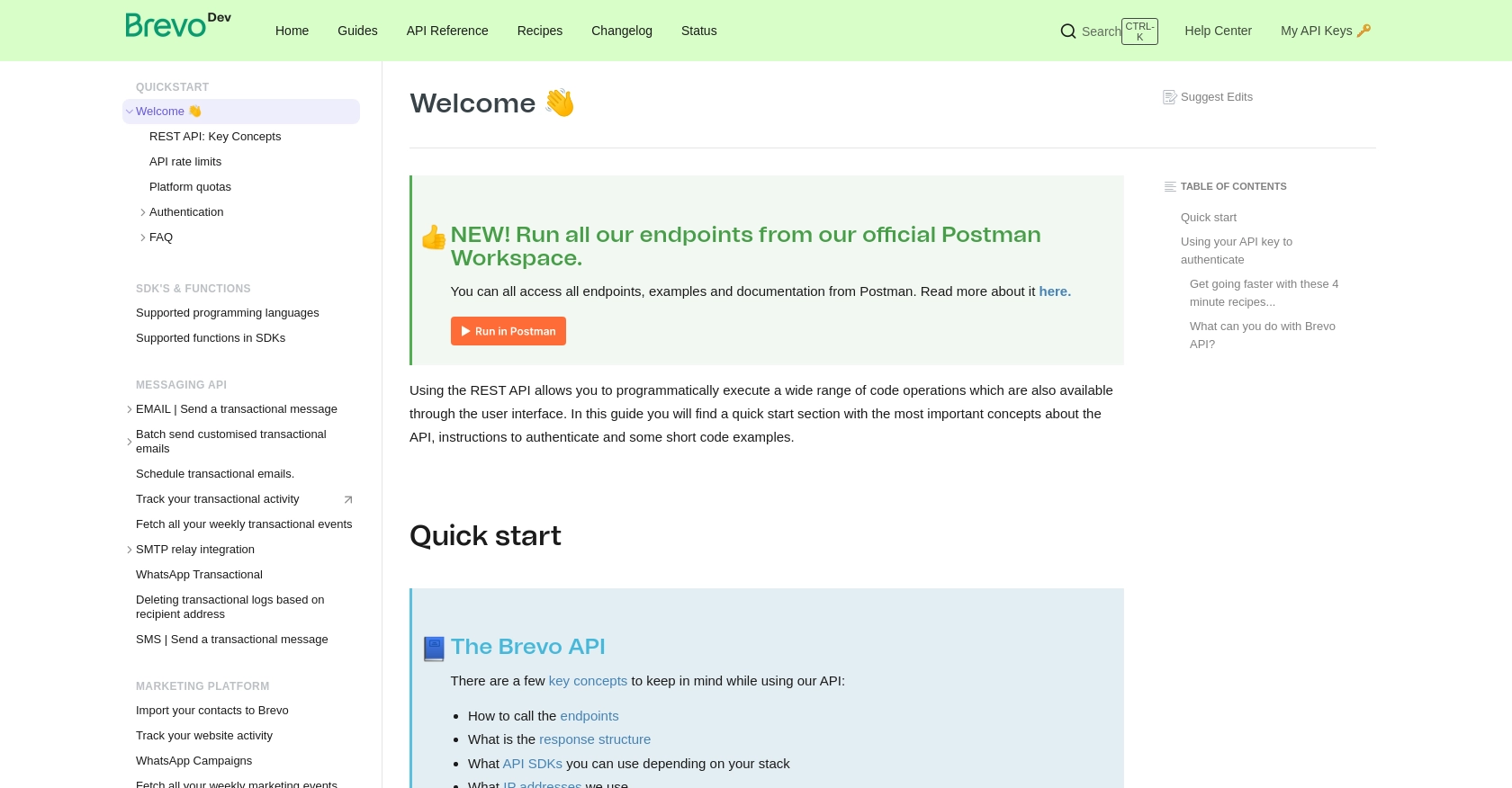
sbb-itb-96038d7
Sure, here's the HTML content for the "How to make the actual API call" section:Making API Calls to Retrieve Contacts Using Brevo API in PHP
To interact with the Brevo API and retrieve contact information, you'll need to set up your PHP environment and make authenticated requests. This section will guide you through the necessary steps, including setting up your PHP environment, writing the code, and handling potential errors.
Setting Up Your PHP Environment for Brevo API Integration
Before making API calls, ensure your PHP environment is ready. You'll need PHP installed on your machine along with Composer, a dependency manager for PHP.
- Ensure PHP is installed by running
php -v
in your terminal. - Install Composer by following the instructions on the Composer download page.
Once Composer is installed, you can use it to manage the Brevo API client library.
Installing Required Dependencies for Brevo API
Use Composer to install the Brevo API client library:
composer require sendinblue/api-v3-sdk
Writing the PHP Code to Retrieve Contacts from Brevo
With the dependencies installed, you can now write the PHP code to make an API call to Brevo and retrieve contacts:
<?php
require_once(__DIR__ . '/vendor/autoload.php');
$config = SendinBlue\Client\Configuration::getDefaultConfiguration()->setApiKey('api-key', 'YOUR_API_KEY');
$apiInstance = new SendinBlue\Client\Api\ContactsApi(
new GuzzleHttp\Client(),
$config
);
$limit = 50;
$offset = 0;
$modifiedSince = new \DateTime('2021-09-07T19:20:30+01:00');
try {
$result = $apiInstance->getContacts($limit, $offset, $modifiedSince);
print_r($result);
} catch (Exception $e) {
echo 'Exception when calling ContactsApi->getContacts: ', $e->getMessage(), PHP_EOL;
}
?>
Replace YOUR_API_KEY
with the API key you generated earlier. This script initializes the API client, sets the parameters for the request, and prints the retrieved contacts.
Handling Errors and Verifying API Call Success
After running the script, verify the output to ensure the API call was successful. If there are any errors, the catch block will display the error message. Common errors include:
- 400 Bad Request: Check your request parameters.
- 401 Unauthorized: Verify your API key.
- 429 Too Many Requests: Brevo API has a rate limit; ensure you're not exceeding it.
For more details on error codes, refer to the Brevo API documentation.
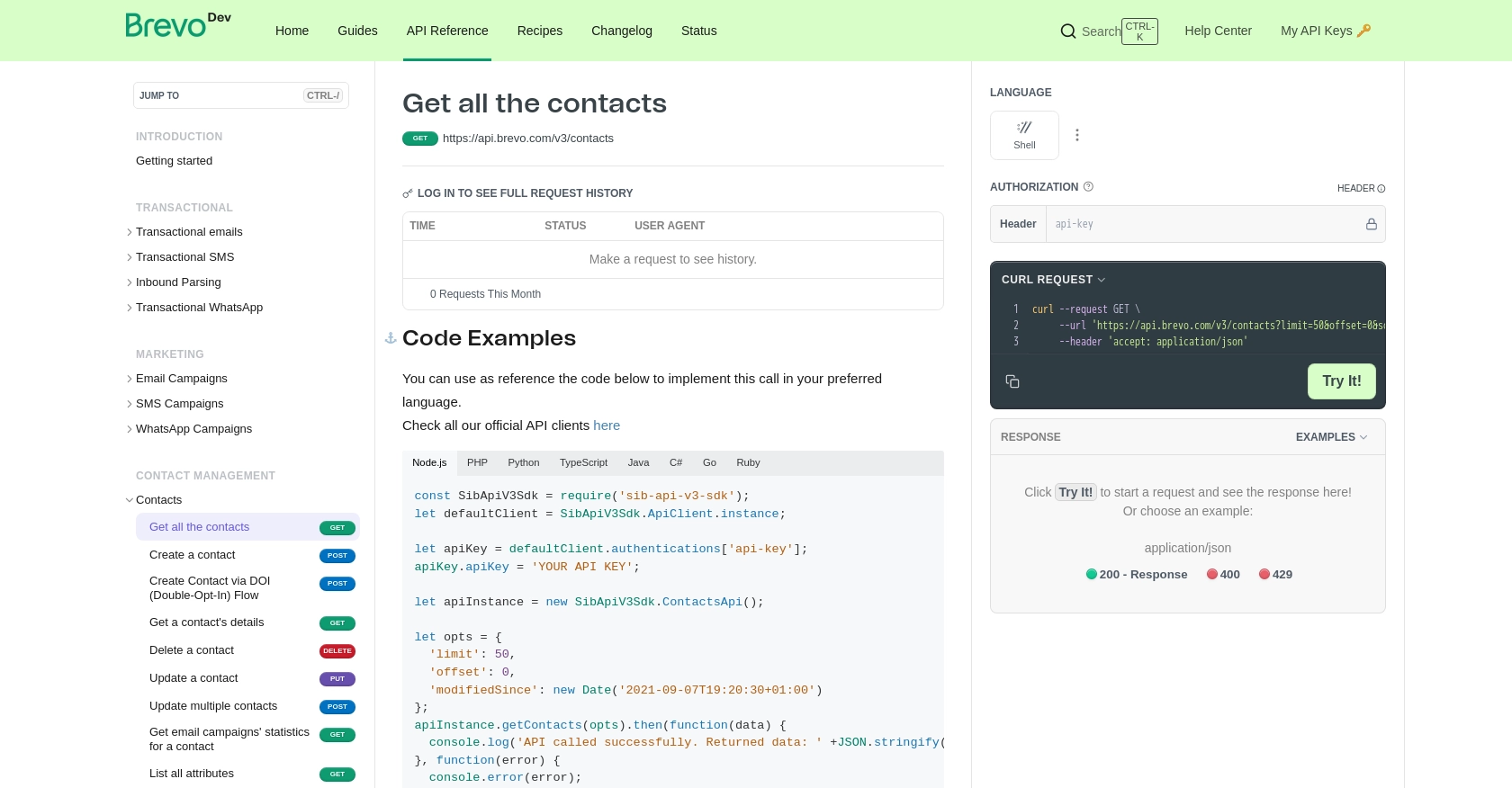
Conclusion and Best Practices for Using Brevo API in PHP
Integrating with the Brevo API using PHP allows developers to efficiently manage contacts and enhance their communication strategies. By following the steps outlined in this guide, you can seamlessly retrieve and manage contact data, ensuring your applications remain synchronized and up-to-date.
Best Practices for Secure and Efficient Brevo API Integration
- Secure API Key Storage: Always store your API keys securely. Consider using environment variables or a secure vault to prevent unauthorized access.
- Handle Rate Limits: Brevo API imposes rate limits. Implement logic to handle
429 Too Many Requests
errors by retrying requests after a delay. - Data Transformation: Standardize and transform data fields as needed to ensure compatibility with your CRM or other systems.
- Error Handling: Implement robust error handling to gracefully manage API call failures and provide meaningful feedback to users.
Streamline Your Integrations with Endgrate
While integrating with Brevo API can be straightforward, managing multiple integrations can become complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Brevo. This allows you to focus on your core product while outsourcing integration complexities.
Explore how Endgrate can save you time and resources by offering an intuitive integration experience for your customers. Visit Endgrate to learn more about how you can streamline your integration processes.
Read More
Ready to get started?