How to Get Accounts with the Microsoft Dynamics 365 API in Python
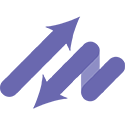
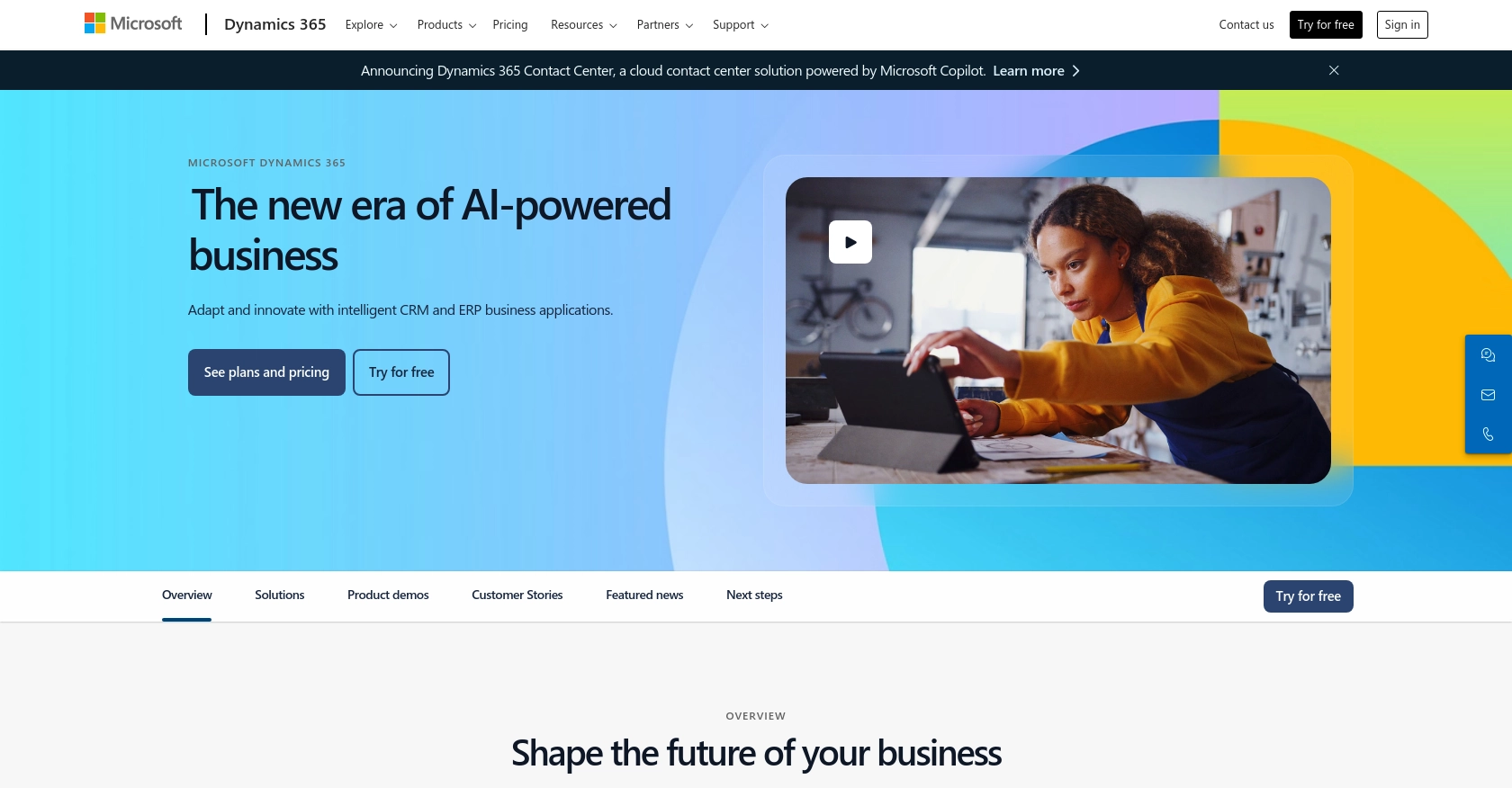
Introduction to Microsoft Dynamics 365 API
Microsoft Dynamics 365 is a comprehensive suite of business applications that combines CRM and ERP capabilities to streamline business processes and improve customer engagement. It offers a range of tools for sales, customer service, finance, and operations, making it a popular choice for businesses looking to enhance their productivity and efficiency.
Integrating with the Microsoft Dynamics 365 API allows developers to access and manage business data programmatically. For example, a developer might want to retrieve account information to generate detailed financial reports or synchronize data with other business systems. This integration can significantly enhance the ability to automate workflows and improve data accuracy across platforms.
Setting Up a Microsoft Dynamics 365 Test/Sandbox Account
Before you can start interacting with the Microsoft Dynamics 365 API, you need to set up a test or sandbox account. This environment allows you to safely develop and test your integrations without affecting live data.
Register for a Microsoft Dynamics 365 Free Trial
To begin, sign up for a free trial of Microsoft Dynamics 365. This trial provides access to the full suite of Dynamics 365 applications, allowing you to explore and test various features.
- Visit the Microsoft Dynamics 365 Free Trial page.
- Follow the on-screen instructions to create your account.
- Once registered, you will receive access to a sandbox environment where you can test API interactions.
Create an App for OAuth Authentication
Microsoft Dynamics 365 uses OAuth for authentication. You need to register an application in your Microsoft Entra ID tenant to obtain the necessary credentials for API access.
- Navigate to the Azure Portal and sign in with your Microsoft account.
- Go to Azure Active Directory > App registrations > New registration.
- Enter a name for your app and select the appropriate account type.
- Set the redirect URI to
https://localhost
for local development. - Click Register to create the app.
Generate Client ID and Client Secret
After registering your app, you need to generate a client ID and client secret to authenticate API requests.
- In the app registration page, navigate to Certificates & secrets.
- Under Client secrets, click New client secret.
- Add a description and select an expiration period, then click Add.
- Copy the client secret value immediately as it will not be displayed again.
Configure API Permissions
To access Microsoft Dynamics 365 data, configure the necessary API permissions for your app.
- Go to API permissions in your app registration.
- Click Add a permission and select Dynamics 365.
- Choose the required permissions, such as
user_impersonation
, and click Add permissions. - Ensure you grant admin consent for the permissions.
With these steps completed, your Microsoft Dynamics 365 test environment is ready, and you can proceed to make API calls using the OAuth credentials.
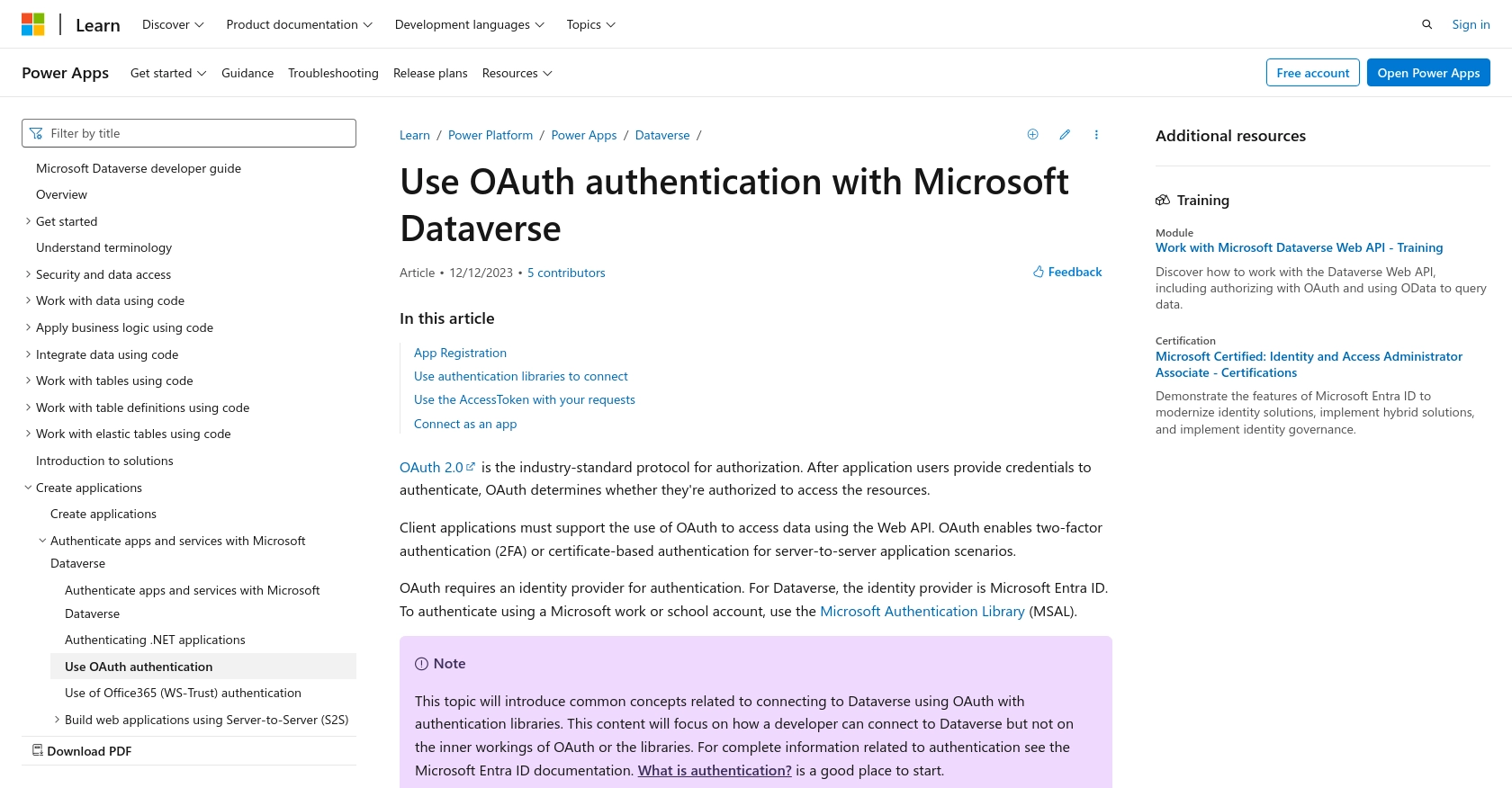
sbb-itb-96038d7
Making API Calls to Retrieve Accounts from Microsoft Dynamics 365 Using Python
To interact with the Microsoft Dynamics 365 API and retrieve account information, you'll need to use Python. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your Python Environment for Microsoft Dynamics 365 API
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
Next, install the required libraries by running the following command in your terminal:
pip install requests msal
Writing Python Code to Retrieve Accounts from Microsoft Dynamics 365
Create a new Python file named get_dynamics_accounts.py
and add the following code:
import requests from msal import ConfidentialClientApplication # Define your credentials and endpoints client_id = 'Your_Client_ID' client_secret = 'Your_Client_Secret' tenant_id = 'Your_Tenant_ID' authority = f"https://login.microsoftonline.com/{tenant_id}" scope = ["https://yourorg.crm.dynamics.com/.default"] endpoint = "https://yourorg.crm.dynamics.com/api/data/v9.2/accounts" # Acquire a token using MSAL app = ConfidentialClientApplication(client_id, authority=authority, client_credential=client_secret) token_response = app.acquire_token_for_client(scopes=scope) # Check if token acquisition was successful if "access_token" in token_response: headers = { "Authorization": f"Bearer {token_response['access_token']}", "OData-MaxVersion": "4.0", "OData-Version": "4.0", "Accept": "application/json" } # Make the GET request to retrieve accounts response = requests.get(endpoint, headers=headers) # Check if the request was successful if response.status_code == 200: accounts = response.json() for account in accounts['value']: print(f"Account Name: {account['name']}, Account ID: {account['accountid']}") else: print(f"Failed to retrieve accounts: {response.status_code} - {response.text}") else: print("Failed to acquire token.")
Replace Your_Client_ID
, Your_Client_Secret
, and Your_Tenant_ID
with your actual credentials.
Verifying API Call Success and Handling Errors
After running the script, you should see a list of accounts printed in your terminal. If the request fails, the script will output the error code and message. Common error codes include:
- 401 Unauthorized: Check your credentials and ensure the token is valid.
- 403 Forbidden: Verify that your app has the necessary permissions.
- 404 Not Found: Ensure the endpoint URL is correct.
For more detailed error handling, refer to the Microsoft Dynamics 365 API documentation.
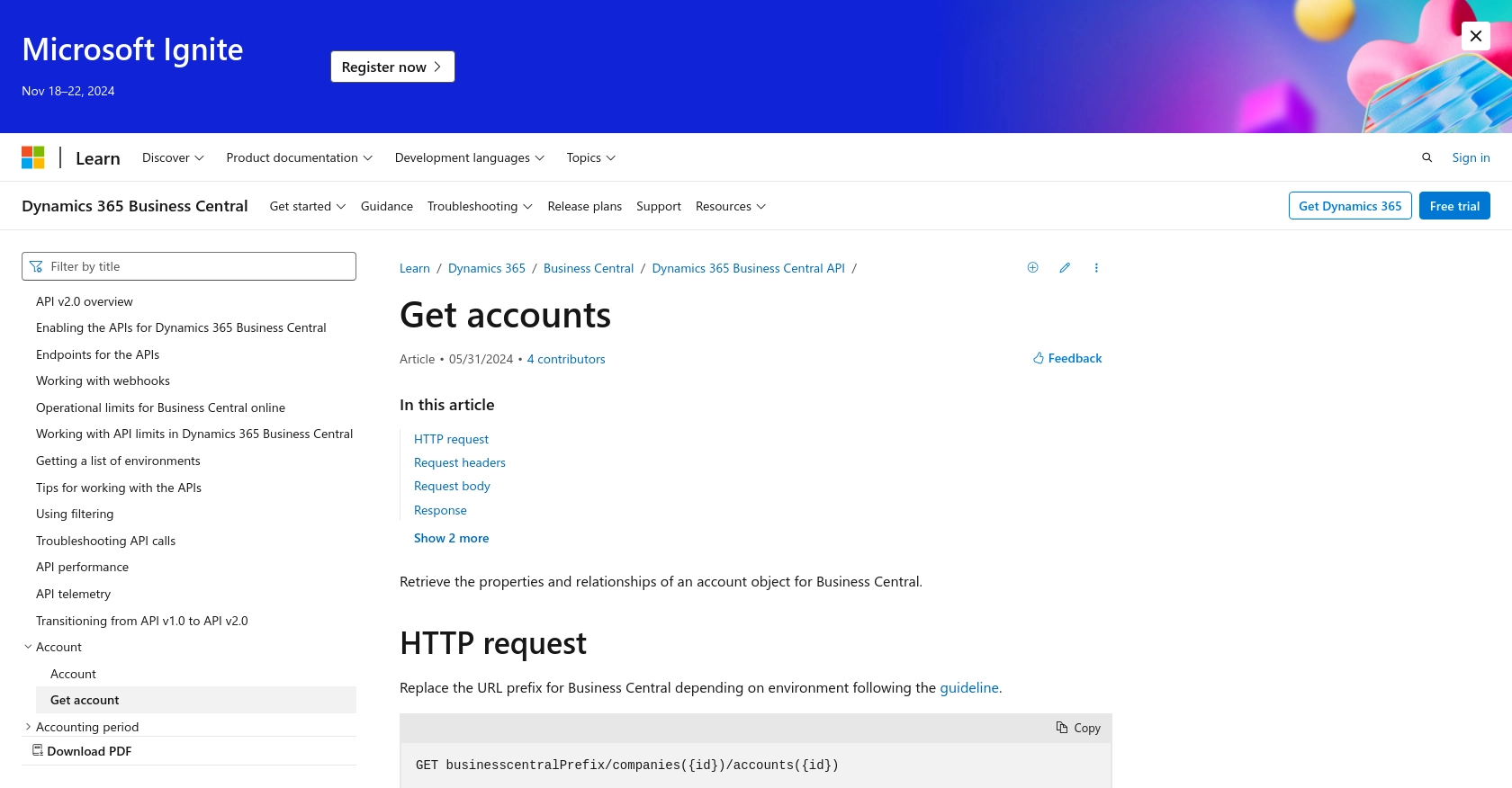
Conclusion and Best Practices for Using Microsoft Dynamics 365 API in Python
Integrating with the Microsoft Dynamics 365 API using Python offers a powerful way to automate and streamline business processes. By following the steps outlined in this guide, developers can efficiently retrieve account information and leverage it for various business applications.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your client ID, client secret, and other sensitive information securely. Consider using environment variables or secure vaults to manage these credentials.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Microsoft Dynamics 365 API. Implement retry logic and exponential backoff to handle rate limit errors gracefully.
- Data Transformation and Standardization: Ensure that data retrieved from the API is transformed and standardized to fit your application's requirements. This can help maintain data consistency across systems.
- Monitor and Log API Requests: Implement logging to monitor API requests and responses. This can help in troubleshooting issues and understanding usage patterns.
Enhance Your Integration Strategy with Endgrate
While building integrations with Microsoft Dynamics 365 can be rewarding, it can also be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to multiple platforms, including Microsoft Dynamics 365. By leveraging Endgrate, developers can focus on their core product while outsourcing integrations, saving time and resources.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate and discover a more efficient way to manage your integrations.
Read More
- https://endgrate.com/provider/microsoftdynamics365
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/authenticate-oauth
- https://learn.microsoft.com/en-us/graph/use-the-api
- https://learn.microsoft.com/en-us/dynamics365/business-central/dev-itpro/api-reference/v2.0/api/dynamics_account_get
Ready to get started?