Using the PipelineCRM API to Get People (with Javascript examples)
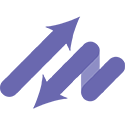
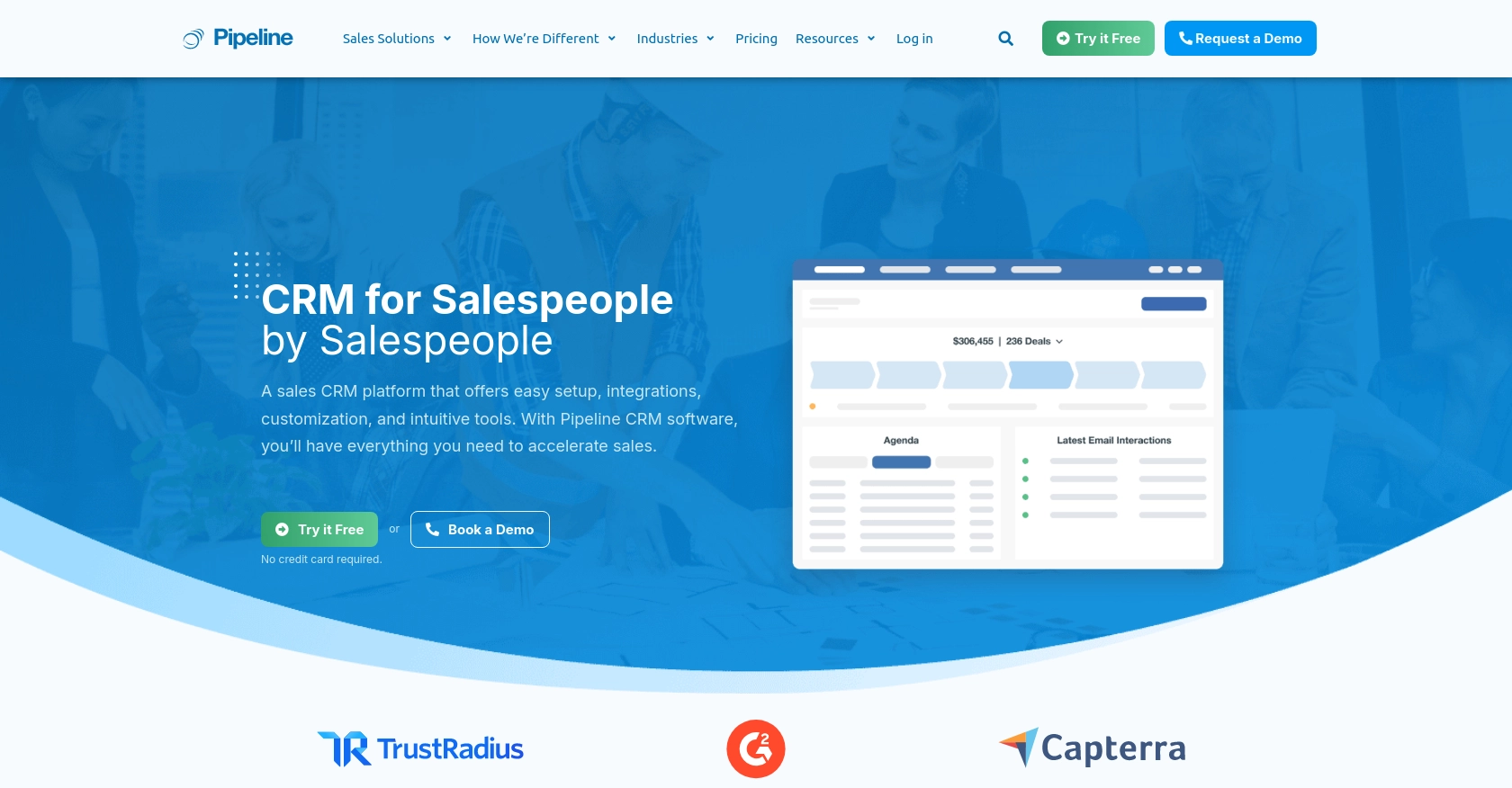
Introduction to PipelineCRM
PipelineCRM is a robust customer relationship management platform designed to help businesses manage their sales processes efficiently. With features like contact management, sales tracking, and reporting, PipelineCRM provides a comprehensive solution for sales teams looking to streamline their workflows and improve customer interactions.
Integrating with the PipelineCRM API allows developers to access and manipulate customer data programmatically. For example, a developer might use the API to retrieve a list of people from the CRM system, enabling seamless integration with other business applications or custom reporting tools.
Setting Up Your PipelineCRM Test Account
Before you can start interacting with the PipelineCRM API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Follow these steps to get started:
Create a PipelineCRM Account
- Visit the PipelineCRM website and sign up for a free trial or demo account.
- Follow the on-screen instructions to complete the registration process.
- Once your account is created, log in to access the PipelineCRM dashboard.
Generate Your API Key for PipelineCRM
PipelineCRM uses API key-based authentication to secure API requests. Here's how to generate your API key:
- Navigate to the account settings in the PipelineCRM dashboard.
- Locate the API section and click on it to access API settings.
- Click on the option to generate a new API key.
- Copy the generated API key and store it securely, as you will need it to authenticate your API requests.
With your API key ready, you can now proceed to make API calls to PipelineCRM. Ensure that you keep your API key confidential to prevent unauthorized access to your account.
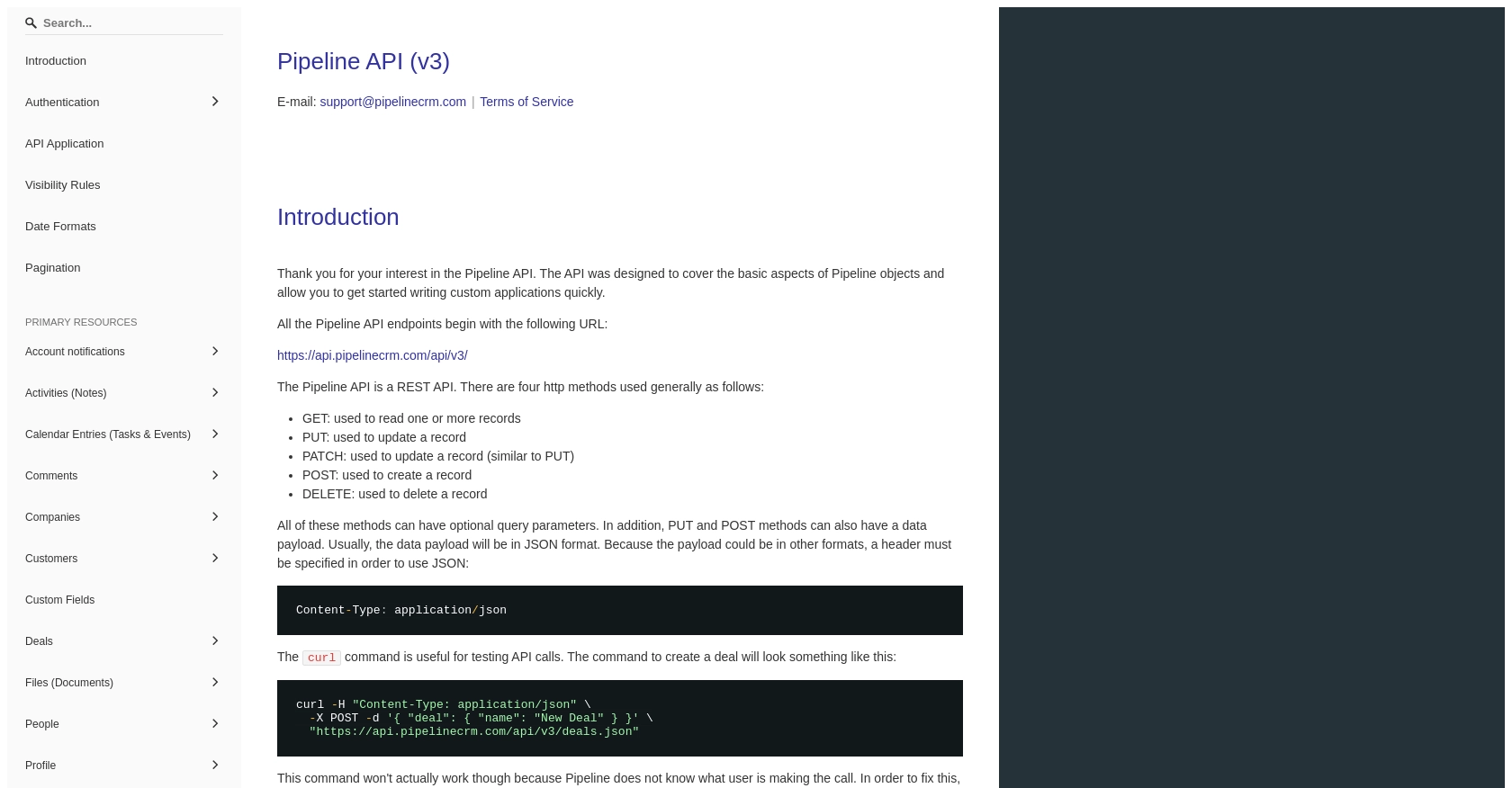
sbb-itb-96038d7
Making API Calls to Retrieve People from PipelineCRM Using JavaScript
To interact with the PipelineCRM API and retrieve a list of people, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your JavaScript Environment
Before you begin, ensure you have the following prerequisites installed on your machine:
- Node.js (version 14 or later)
- NPM (Node Package Manager)
Once you have these installed, open your terminal and create a new project directory:
mkdir pipelinecrm-api-example
cd pipelinecrm-api-example
npm init -y
Next, install the Axios library, which will be used to make HTTP requests:
npm install axios
Writing JavaScript Code to Fetch People from PipelineCRM
Create a new file named getPeople.js
and add the following code:
const axios = require('axios');
// Replace 'YOUR_API_KEY' with your actual PipelineCRM API key
const apiKey = 'YOUR_API_KEY';
// Set the API endpoint
const endpoint = 'https://api.pipelinecrm.com/api/v3/people';
// Configure the request headers
const headers = {
'Content-Type': 'application/json',
'Authorization': `Bearer ${apiKey}`
};
// Function to get people from PipelineCRM
async function getPeople() {
try {
const response = await axios.get(endpoint, { headers });
const people = response.data.data;
console.log('Retrieved People:', people);
} catch (error) {
console.error('Error fetching people:', error.response ? error.response.data : error.message);
}
}
// Execute the function
getPeople();
In this code, you use Axios to send a GET request to the PipelineCRM API endpoint for retrieving people. Make sure to replace 'YOUR_API_KEY'
with the API key you generated earlier.
Running the JavaScript Code and Verifying Results
To execute the code, run the following command in your terminal:
node getPeople.js
If successful, you should see a list of people retrieved from your PipelineCRM test account printed in the console. This confirms that the API call was successful.
Handling Errors and Understanding Error Codes
When making API calls, it's essential to handle potential errors gracefully. The code above includes a try-catch
block to catch and log any errors that occur during the request. Common error codes you might encounter include:
- 401 Unauthorized: Indicates that the API key is missing or invalid.
- 404 Not Found: The requested resource could not be found.
- 500 Internal Server Error: An error occurred on the server side.
For more detailed information on error codes, refer to the PipelineCRM API documentation.
Conclusion: Best Practices for Using the PipelineCRM API with JavaScript
Integrating with the PipelineCRM API using JavaScript can significantly enhance your ability to manage customer data efficiently. By following the steps outlined in this article, you can seamlessly retrieve and manipulate data, enabling better integration with other business applications.
Best Practices for Secure and Efficient API Integration
- Secure Your API Key: Always store your API key securely and avoid hardcoding it in your source files. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the API. Implement retry logic and exponential backoff strategies to handle rate limit errors gracefully.
- Standardize Data Fields: When integrating with multiple systems, ensure that data fields are standardized to maintain consistency across platforms.
- Monitor API Usage: Regularly monitor your API usage to identify any anomalies or potential issues. This can help in optimizing your integration and ensuring smooth operation.
Streamline Your Integrations with Endgrate
While integrating with individual APIs like PipelineCRM can be beneficial, managing multiple integrations can become complex and time-consuming. This is where Endgrate can help. By providing a unified API endpoint, Endgrate allows you to connect with multiple platforms effortlessly, saving you time and resources.
With Endgrate, you can focus on your core product while outsourcing the complexities of integration. Build once for each use case and enjoy an intuitive integration experience for your customers. Visit Endgrate to learn more about how you can simplify your integration processes.
Read More
Ready to get started?