Using the FTP API to Export Data (with Python examples)
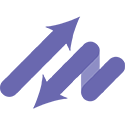
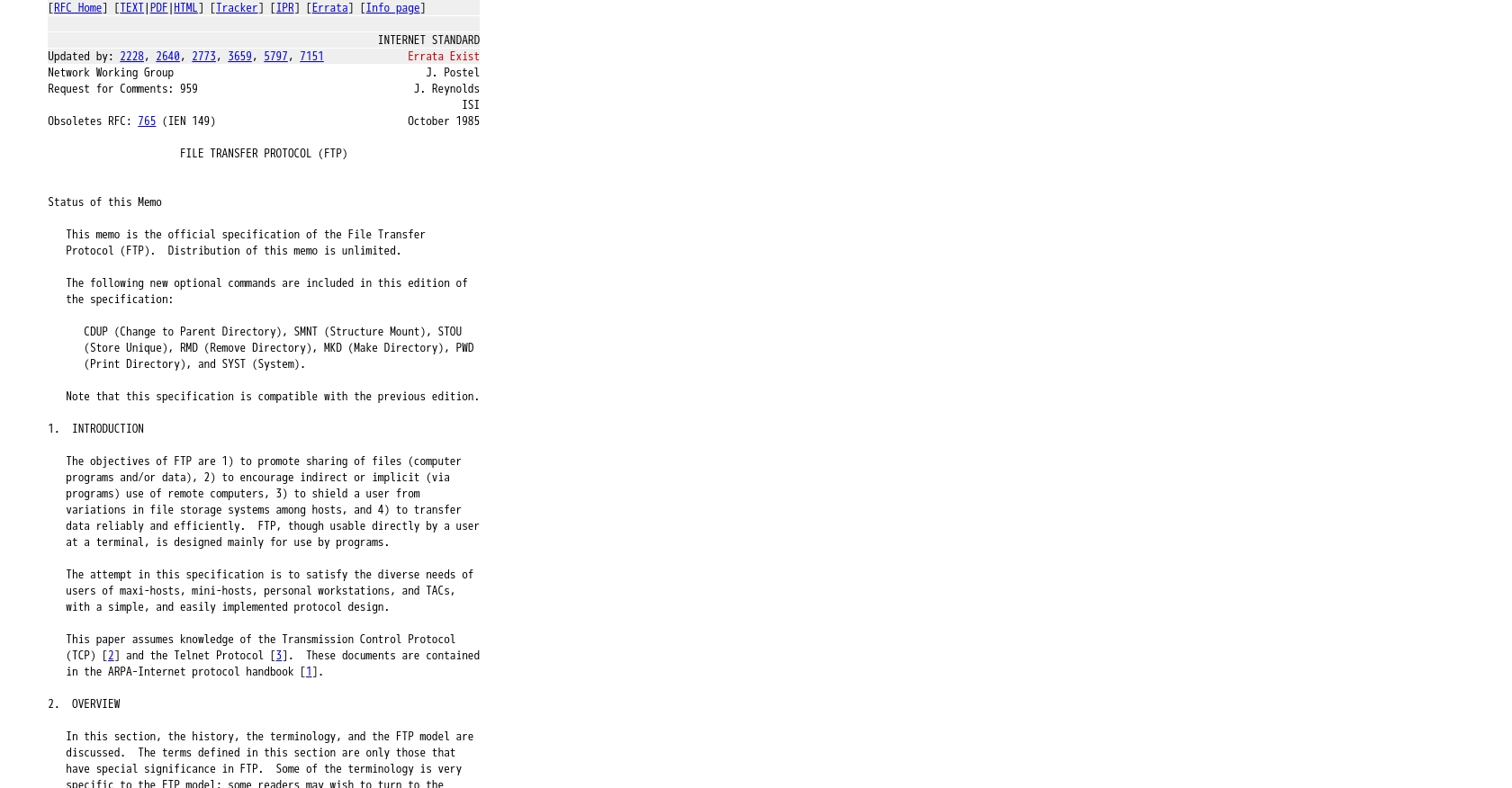
Introduction to FTP and Data Export
File Transfer Protocol (FTP) is a standard network protocol used to transfer files between a client and server on a computer network. It is widely used for exchanging and managing files over the internet, making it a vital tool for developers who need to handle large volumes of data efficiently.
Integrating with an FTP server allows developers to automate the process of exporting data, which can be particularly useful for businesses that need to regularly back up data or share large datasets with partners. For example, a developer might use the FTP API to export sales data from a SaaS application to an external server for further analysis or reporting.
Setting Up Your FTP Server for Data Export
Before you can start exporting data using the FTP API, you need to set up access to an FTP server. This involves creating an account on an FTP server and obtaining the necessary credentials to connect and authenticate your requests.
Creating an FTP Account
To begin, you need access to an FTP server. If you don't have one, you can set up a local FTP server or use a cloud-based service that offers FTP access. Many hosting providers offer FTP services as part of their packages.
- Choose a hosting provider or set up a local FTP server.
- Create an FTP account by following the provider's instructions.
- Ensure you have the following details: FTP server address, username, and password.
Authenticating with the FTP Server
FTP uses a simple username and password authentication method. You'll need these credentials to connect to the server and perform data export operations.
Here's how you can authenticate using Python:
from ftplib import FTP
# Connect to the FTP server
ftp = FTP('ftp.example.com')
ftp.login(user='your_username', passwd='your_password')
# Verify connection
print("Connection successful: ", ftp.getwelcome())
Replace ftp.example.com
, your_username
, and your_password
with your actual FTP server details.
Testing Your FTP Connection
Once authenticated, it's a good practice to test your connection by listing the files in the server's root directory:
# List files in the root directory
files = ftp.nlst()
print("Files in root directory:", files)
If the connection is successful, you should see a list of files and directories available on the server.
With your FTP server set up and authenticated, you're ready to start exporting data using the FTP API. In the next section, we'll explore how to perform these operations using Python.
Making FTP API Calls for Data Export Using Python
To effectively export data using the FTP API, you'll need to leverage Python's capabilities to interact with the FTP server. This section will guide you through the process of making API calls to export data efficiently.
Setting Up Your Python Environment for FTP API Integration
Before diving into the code, ensure that your Python environment is properly set up. You'll need Python 3.x installed on your machine, along with the ftplib
library, which is included in Python's standard library.
Verify your Python installation by running the following command in your terminal:
python --version
If Python is installed, you should see the version number. If not, download and install Python from the official website.
Exporting Data from FTP Server Using Python
Once your environment is ready, you can start writing the code to export data from the FTP server. Below is a step-by-step guide to performing this operation:
from ftplib import FTP
# Connect to the FTP server
ftp = FTP('ftp.example.com')
ftp.login(user='your_username', passwd='your_password')
# Define the file you want to download
filename = 'data.csv'
# Open a local file to store the downloaded data
with open(filename, 'wb') as local_file:
# Use FTP's RETR command to download the file
ftp.retrbinary(f'RETR {filename}', local_file.write)
# Close the FTP connection
ftp.quit()
print(f"Data exported successfully to {filename}")
Replace ftp.example.com
, your_username
, and your_password
with your actual FTP server details. The data.csv
should be replaced with the name of the file you wish to export.
Verifying Successful Data Export from FTP Server
After running the script, check your local directory for the downloaded file. If the file is present and contains the expected data, the export was successful. You can further verify by opening the file and inspecting its contents.
Handling Errors and Exceptions in FTP Data Export
While interacting with the FTP server, you may encounter errors such as connection issues or file not found errors. It's crucial to handle these exceptions gracefully:
try:
# Connect and login to the FTP server
ftp = FTP('ftp.example.com')
ftp.login(user='your_username', passwd='your_password')
# Attempt to download the file
with open(filename, 'wb') as local_file:
ftp.retrbinary(f'RETR {filename}', local_file.write)
print(f"Data exported successfully to {filename}")
except Exception as e:
print(f"An error occurred: {e}")
finally:
# Ensure the FTP connection is closed
ftp.quit()
This code snippet includes a try-except-finally
block to catch and handle any exceptions, ensuring the FTP connection is closed properly.
By following these steps, you can efficiently export data from an FTP server using Python, automating your data management processes and enhancing productivity.
Conclusion and Best Practices for FTP Data Export Using Python
Exporting data using the FTP API with Python can significantly streamline your data management processes, allowing for efficient and automated data transfers. By following the steps outlined in this guide, you can set up a robust system for exporting data from an FTP server, ensuring that your data is always accessible and up-to-date.
Best Practices for Secure and Efficient FTP Data Transfers
- Secure Credentials: Always store your FTP credentials securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limiting: Be mindful of any rate limits imposed by your FTP server provider. Implement retry logic and exponential backoff to handle rate limiting gracefully.
- Data Standardization: Ensure that exported data is standardized and transformed as needed to maintain consistency across different systems.
- Error Handling: Implement comprehensive error handling to manage connection issues, authentication failures, and file transfer errors effectively.
Enhancing Integration Capabilities with Endgrate
While managing FTP integrations manually can be effective, leveraging a tool like Endgrate can further enhance your integration capabilities. Endgrate provides a unified API endpoint that simplifies the process of connecting to multiple platforms, including FTP servers. By using Endgrate, you can save time and resources, allowing you to focus on your core product development.
Explore how Endgrate can streamline your integration processes and provide a seamless experience for your customers. Visit Endgrate to learn more about how you can optimize your integration strategy and achieve faster, more reliable data exports.
Read More
Ready to get started?