Using the Freshsales API to Create or Update Accounts (with PHP examples)
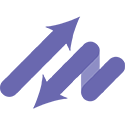
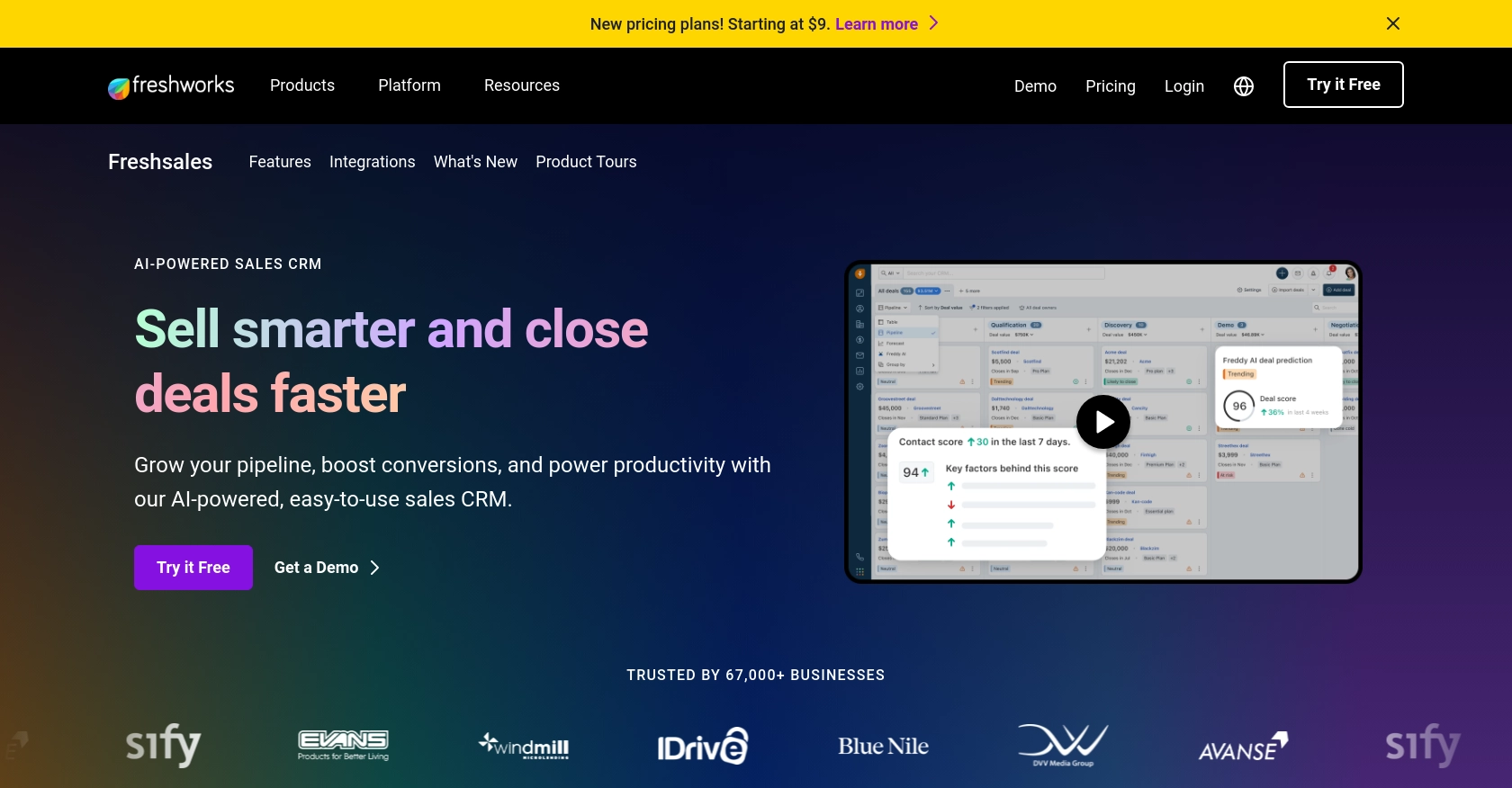
Introduction to Freshsales API
Freshsales is a powerful customer relationship management (CRM) platform designed to help businesses manage their sales processes more effectively. With features such as lead scoring, email tracking, and built-in phone capabilities, Freshsales provides a comprehensive solution for sales teams looking to streamline their operations and improve customer interactions.
Integrating with the Freshsales API allows developers to automate and enhance CRM functionalities, such as creating or updating accounts programmatically. For example, a developer might use the Freshsales API to automatically update account information from an external data source, ensuring that the CRM always reflects the most current customer data.
Setting Up Your Freshsales Test/Sandbox Account
Before you can start integrating with the Freshsales API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data. Freshsales offers a free trial that you can use to access the API and test its functionalities.
Creating a Freshsales Account
To begin, visit the Freshsales website and sign up for a free trial. Follow the on-screen instructions to create your account. Once your account is set up, you'll have access to the Freshsales dashboard where you can manage your CRM data.
Generating an API Key for Freshsales
Freshsales uses a custom authentication method that requires an API key. Here's how you can generate your API key:
- Log in to your Freshsales account.
- Navigate to the profile icon in the top right corner and select Settings.
- Under the API Settings section, you'll find the option to generate an API key.
- Click on Generate New API Key and copy the key provided. Store it securely as you'll need it for authenticating your API requests.
Creating a Freshsales App for OAuth Authentication
If your integration requires OAuth-based authentication, you'll need to create an app within Freshsales:
- In the Freshsales dashboard, go to Settings and select API Settings.
- Click on Create App and fill in the necessary details such as app name and redirect URL.
- Once the app is created, you'll receive a Client ID and Client Secret. These credentials are essential for OAuth authentication.
With your Freshsales account and API key or OAuth credentials ready, you're all set to start making API calls to create or update accounts programmatically using PHP.
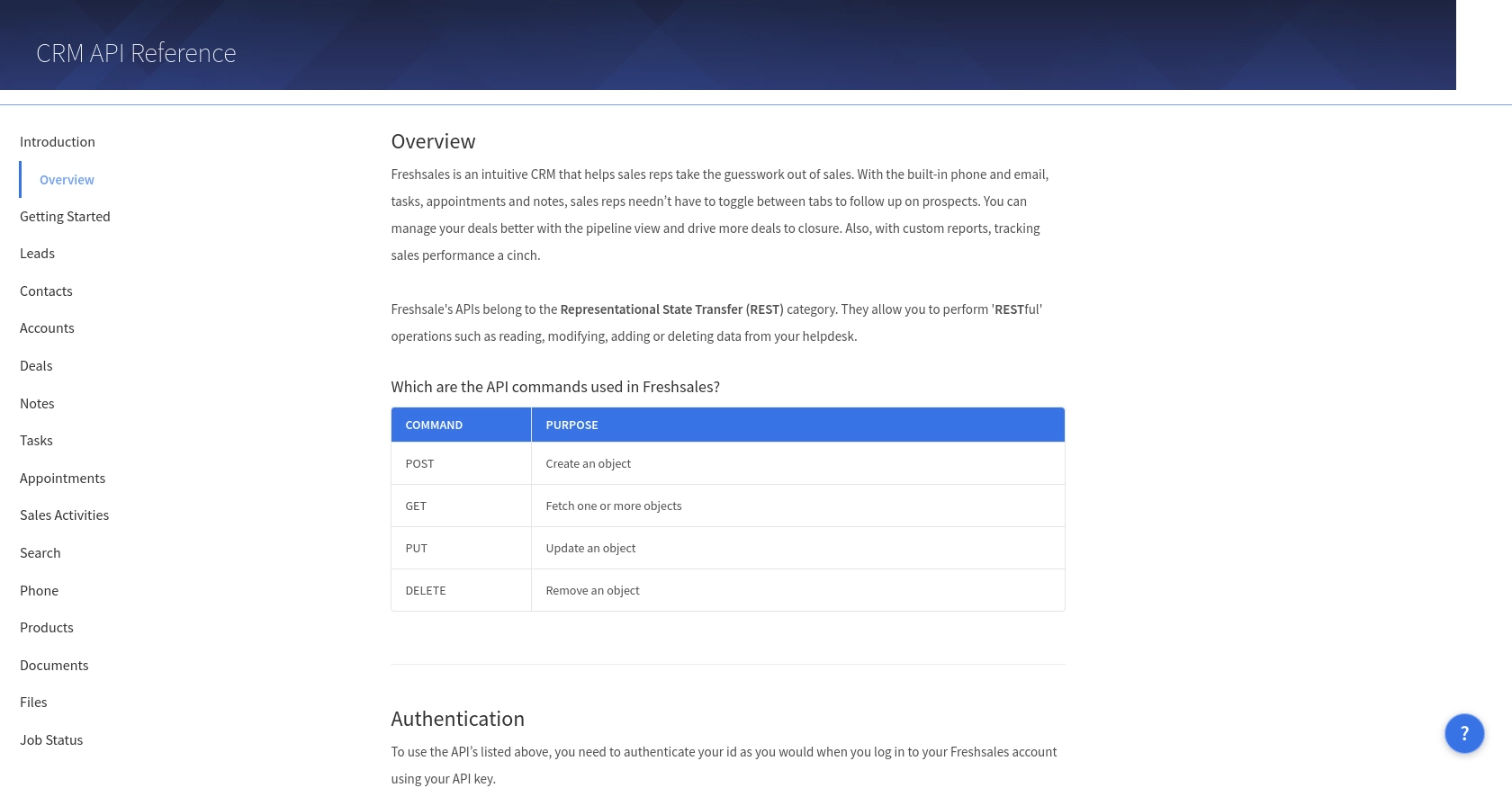
sbb-itb-96038d7
Making API Calls to Freshsales with PHP
To interact with the Freshsales API using PHP, you'll need to ensure you have the correct setup and dependencies. This section will guide you through the process of making API calls to create or update accounts in Freshsales.
Setting Up Your PHP Environment for Freshsales API Integration
Before you begin, make sure you have PHP installed on your machine. You can verify this by running the following command in your terminal:
php -v
If PHP is not installed, you can download it from the official PHP website. Additionally, you'll need the cURL
extension enabled, which is commonly used for making HTTP requests in PHP.
Installing Required PHP Dependencies
To make HTTP requests, you'll use the cURL
library. Ensure it's enabled in your php.ini
file:
extension=curl
Restart your web server to apply changes.
Creating or Updating Accounts in Freshsales Using PHP
Now, let's dive into the code to create or update accounts in Freshsales. You'll use the Freshsales API endpoint for accounts and authenticate using your API key.
<?php
// Freshsales API endpoint for accounts
$endpoint = "https://yourdomain.freshsales.io/api/accounts";
// Your Freshsales API key
$apiKey = "Your_API_Key";
// Data for creating or updating an account
$data = [
"account" => [
"name" => "Example Account",
"website" => "https://example.com",
"industry" => "Technology"
]
];
// Initialize cURL session
$ch = curl_init($endpoint);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
"Authorization: Token token=$apiKey",
"Content-Type: application/json"
]);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
// Execute the request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Request Error:' . curl_error($ch);
} else {
// Decode the response
$responseData = json_decode($response, true);
echo "Account ID: " . $responseData['account']['id'];
}
// Close cURL session
curl_close($ch);
?>
In this code snippet, replace Your_API_Key
with the API key you generated earlier. The $data
array contains the account information you want to create or update. Adjust the fields as needed based on your requirements.
Verifying API Call Success in Freshsales
After running the PHP script, you can verify the success of your API call by checking the Freshsales dashboard. Navigate to the Accounts section to see if the account has been created or updated as expected.
Handling Errors and Troubleshooting
It's crucial to handle potential errors when making API calls. The code above checks for cURL errors and prints them. Additionally, you can inspect the HTTP response code to determine if the request was successful. A 200 status code indicates success, while other codes may require further investigation.
Conclusion and Best Practices for Freshsales API Integration
Integrating with the Freshsales API using PHP allows developers to automate CRM tasks, such as creating or updating accounts, enhancing the efficiency of sales processes. By following the steps outlined in this guide, you can ensure a smooth integration experience.
Best Practices for Secure and Efficient Freshsales API Usage
- Securely Store API Keys: Always store your API keys securely, preferably in environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of Freshsales API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Standardize Data Fields: Ensure that data fields are standardized and validated before making API calls to maintain data integrity within Freshsales.
- Error Handling: Implement robust error handling to manage API call failures and log errors for troubleshooting.
Streamline Your Integrations with Endgrate
While integrating with Freshsales can significantly enhance your CRM capabilities, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Freshsales.
With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers. Visit Endgrate to learn more about how you can streamline your integration processes.
Read More
Ready to get started?